Customize the Email in Flex UI
Not a HIPAA Eligible Service
Email in Twilio Flex is not a HIPAA Eligible Service and should not be used in workflows that are subject to HIPAA.
Email in Flex uses the same programmable model as other parts of the Flex UI. This means that you can add, replace, and remove email editor components or invoke various actions related to email in Flex UI. This section describes the dedicated components and actions in Email in Flex, as well as some ideas for how to use them.
Flex UI has two main components for email: the email editor and the email messages themselves.
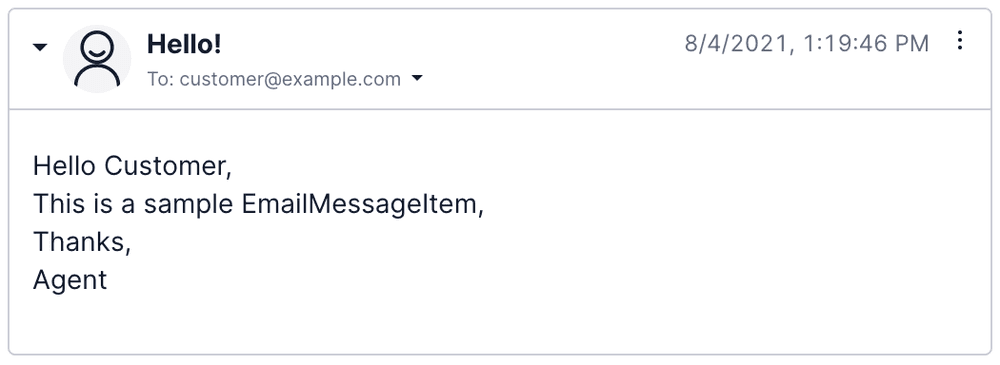
EmailMessageItem
contains an accordion that displays the email message. You can replace it with a custom component or add components before or after it.
1const CustomMessage = (props: EmailMessageItemChildrenProps) => {2return <div>custom message</div>;3};45Flex.EmailMessageItem.Content.replace(<CustomMessage key="custom" />);67
The replaced or added components receive the following props:
1export interface EmailMessageItemChildrenProps {2message: MessageState;3defaultOpen?: boolean;4avatarUrl: string;5conversationSid: string;6}
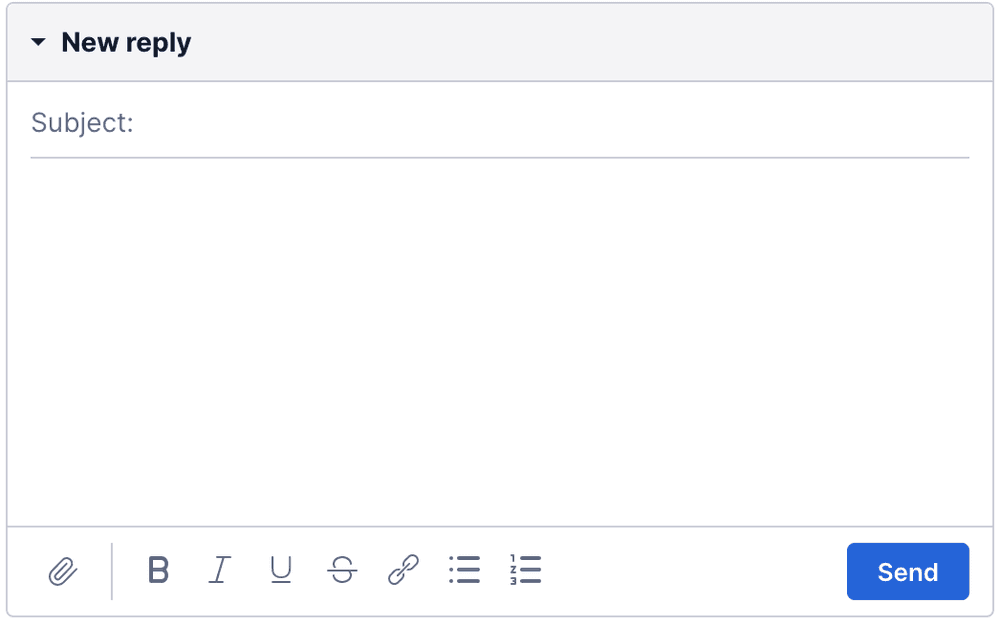
The EmailEditor contains an accordion. You can replace the accordion with custom components, or add components before or after it.
1const CustomEditor = (props: EmailEditorChildrenProps) => {2return <textarea>custom content</textarea>;3};45Flex.EmailEditor.Content.replace(<CustomMessage key="custom" />);
The replaced or added components receive the following props:
1export interface EmailEditorChildrenProps {2disabledReason: string;3charLimit: number;4conversationSid: string;5conversation: ConversationState;6messageState: MessageState;7connectionState: string;8}
Email in Flex uses the Actions Framework to allow you to modify UI interactions. The following actions are standard for sending messages in Flex:
-
SetInputText
- This action supports HTML in input text.
-
SendMessage
The actions for Email in Flex are:
Attaches files to the message the agent is drafting.
1Flex.Actions.invokeAction("AttachFiles", {2files: [file1, file2],3conversationSid: "CHxxxxxxxxx",4conversationType: "email"5});
Property | Description |
---|---|
files | Required. An array of files to be attached. |
conversationSID | Required. The SID for the Conversation to which the media files should be attached. |
conversationType | Required Type: Email. Enforces validation against Conversations limits (max amount of attached files, max size). |
Downloads media files attached to a message.
1Flex.Actions.invokeAction("DownloadMedia", {2media: media,3conversationSid: "CHxxxxxxxxx",4conversationType: "email"5});
Property | Description |
---|---|
media | Required. The Conversations media object to be downloaded. |
conversationSID | Required. The SID for the Conversation from which the media will be downloaded.. |
conversationType | Required Type: Email. Enforces validation against Conversations limits (e.g., max size). |
Starts a new task for the worker. The only required argument is destination
.
1Flex.Actions.invokeAction("StartOutboundEmailTask", {2destination: "customer@address.com",3queueSid: "WQxxxx",4from: "contactCenter@address.com",5fromName: "My contact center name",6taskAttributes: {}7});
Arguments
Property | Description |
---|---|
destination | Required. The address to which the email should be sent. |
queueSid | Optional. The queue that the new email task should be added to. Falls back to default email outbound queue. |
from | Optional. The from email address. Falls back to default email address. |
fromName | Optional. The name associated with the From email address. Falls back to the default email name. |
taskAttributes | Optional. TaskAttributes for the created Task. Defaults to an empty object. |
Flex UI 2.x.x uses a similar programmable interface for adding, removing, and updating components and for invoking actions. See the Flex Developer documentation to learn more about working with components and actions.
Use the SetInputText action to dynamically update the content of the editor. In the following example, the email signature is set using the worker's attributes before they've even written their email.
1const { full_name, email } = Twilio.Flex.Manager.getInstance().workerClient.attributes;23Twilio.Flex.Actions.invokeAction("SetInputText", {4body: <div><p>Your message here</p><p style='margin-top:20px; border-top: 2px dashed; color: tomato; padding-top:20px'>${full_name} - ${email}</p><p style='color:#333333;'>Engineer</p></div>,5conversationSid: "CH049a1c27f2f14bc5b8eba3244a846a05"6});
Editor output:
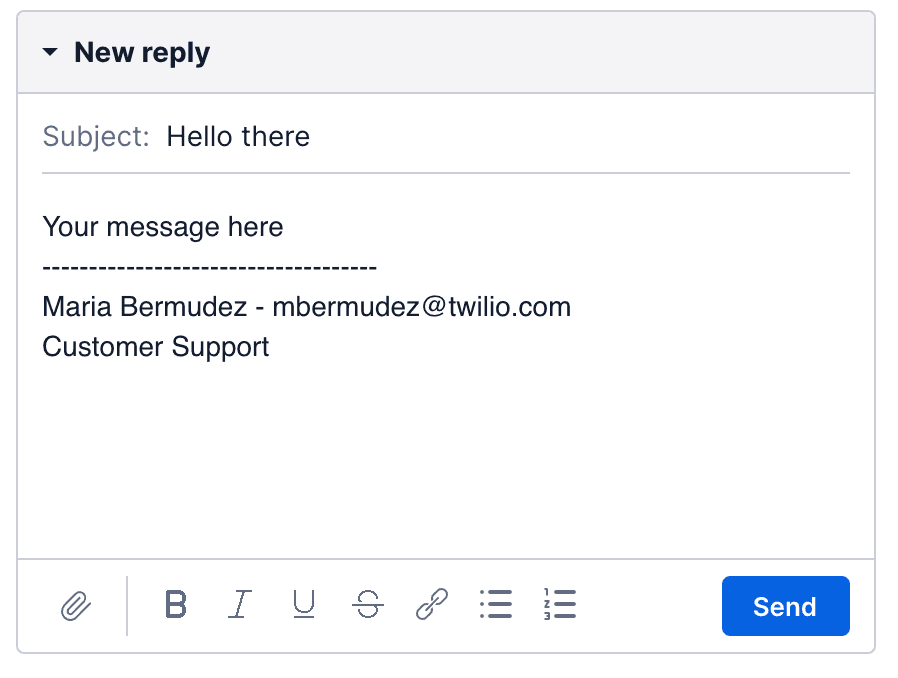
You can go further and include a default response in the text editor so that agents have a strong template to work from. In this example, the SetInputText action is used alongside the Flex TaskHelper method to populate the response with the customer's name.
1const conversationSid = "CH..."2const task = Twilio.Flex.TaskHelper.getTaskFromConversationSid(conversationSid);34Twilio.Flex.Actions.invokeAction("SetInputText", {5body: <div><p>Hi ${task.attributes.customerName}, thanks for your email. </p><p>Regards, Owl Customer Centre</p></div>,6conversationSid,7})
Editor output:
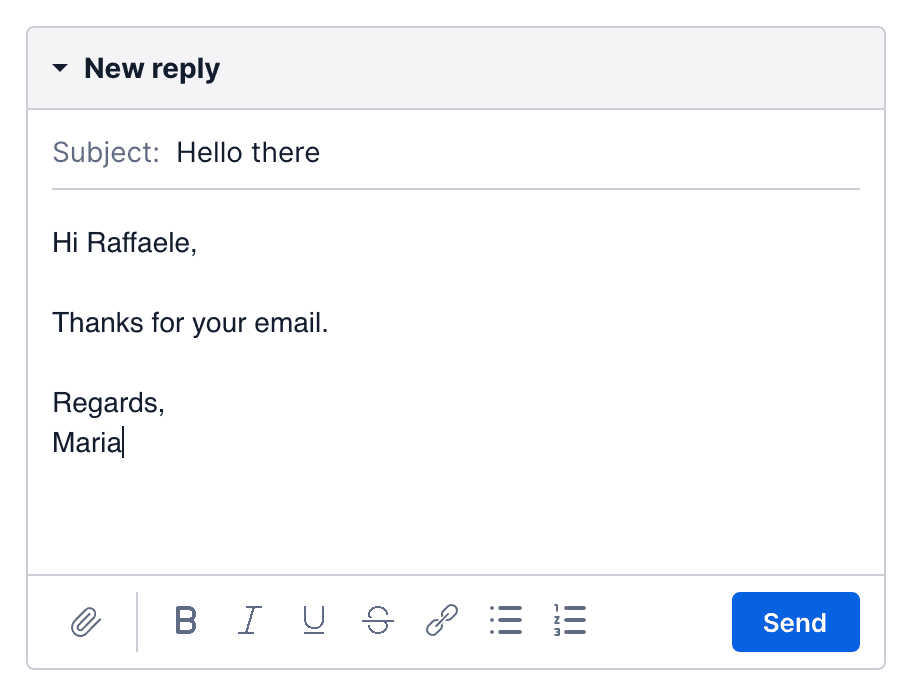
You can automatically inject text after (or before) the agent message by adding the beforeSendMessage listener to Flex Actions. In this example, the code appends a confidentiality disclaimer before sending the email. The agent won't see this change in their HTML editor, but they will see it in the sent message.
1Twilio.Flex.Actions.addListener("beforeSendMessage", (payload) => {2payload.htmlBody += <p style="font-size:10px; color:#666; margin-top:20px;">CONFIDENTIAL: This email and any files transmitted [....]</p>;3});
Message in agent's editor:
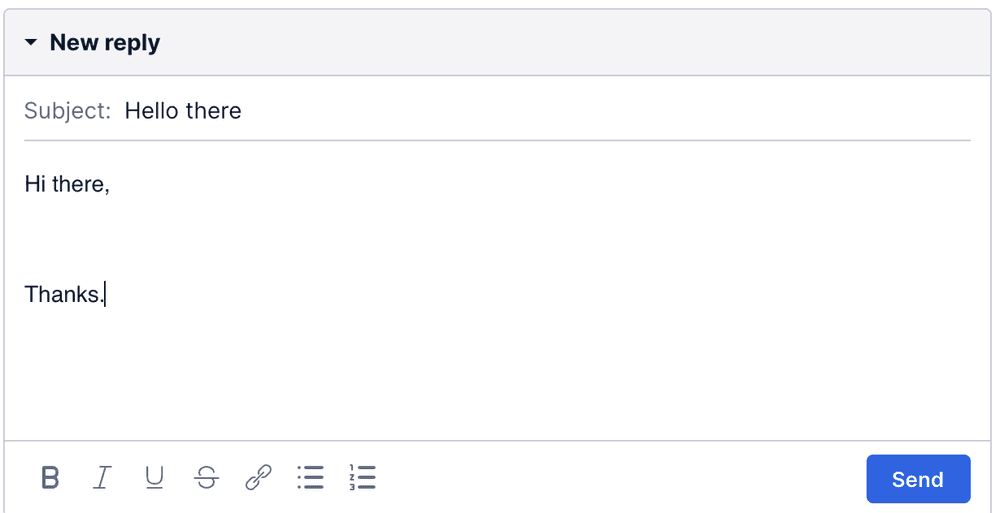
Output message:
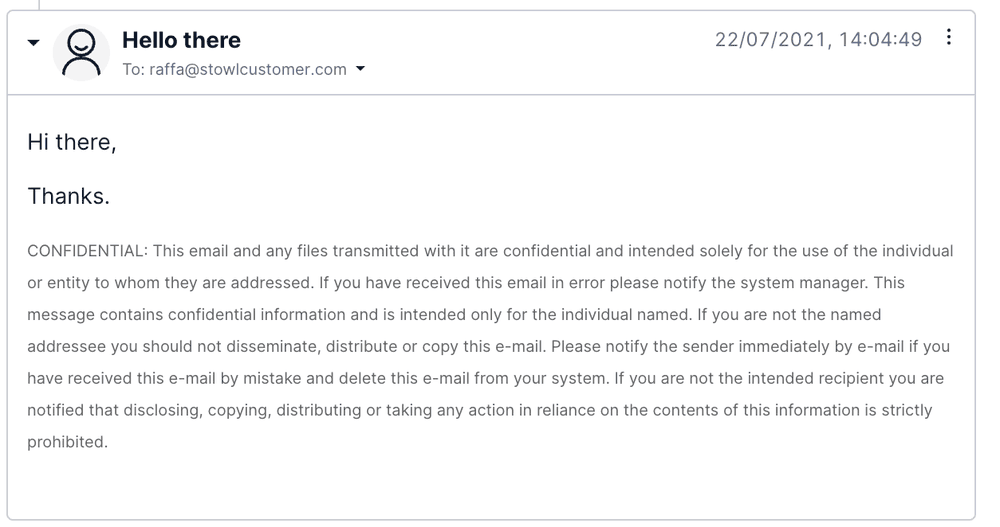
You can create message templates by adding the beforeSendMessage
listener to Flex Actions. In this example, Flex replaces the $CUSTOMER_NAME variable with the actual name of the customer. The agent won't see this change in their HTML editor, but they will see it in the sent message.
1Twilio.Flex.Actions.addListener("beforeSendMessage", (payload) => {2const task = Twilio.Flex.TaskHelper.getTaskFromConversationSid(payload.conversationSid);3payload.htmlBody = payload.htmlBody.replace(/\$CUSTOMER_NAME\$/g, task.attributes.customerName);4})
Message in agent's editor:
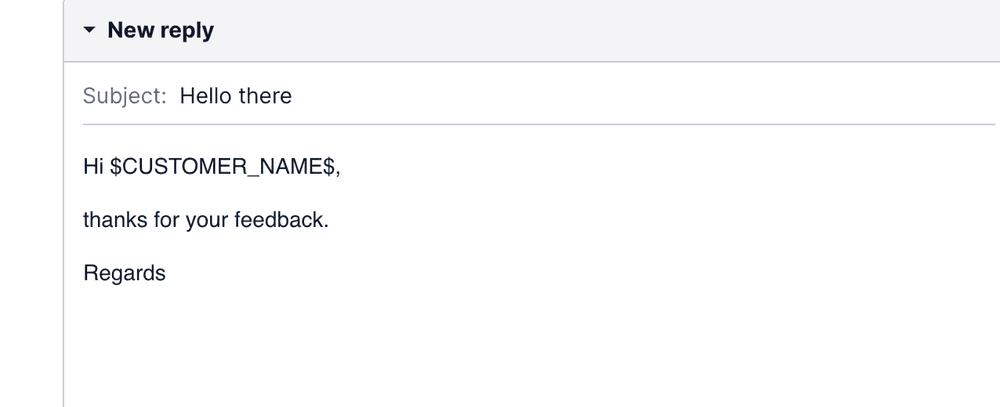
Output message:
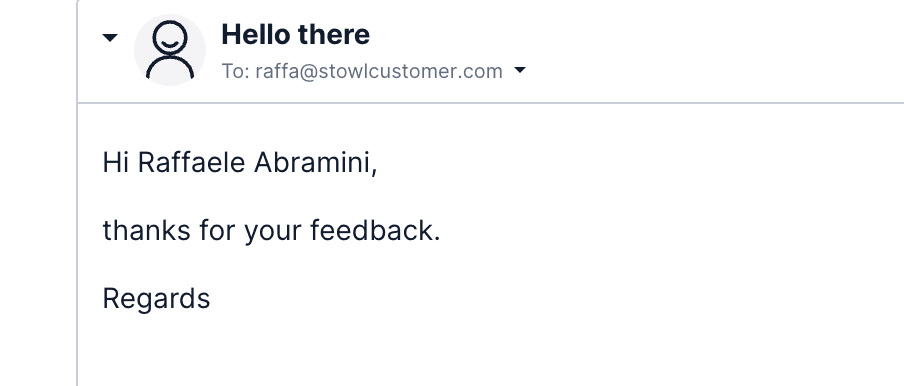