The Proxy API Overview
Proxy Public Beta is not available to new customers
Proxy Public Beta is currently closed for new customers. Please consider using Twilio Conversations and Programmable Voice directly if you are building your masking application.
Public Beta for customers already using Proxy
Twilio's Proxy API is currently available as a Public Beta product. Some features are not yet implemented and others may be changed before the product is declared as Generally Available.
Public Beta products are not covered by a Twilio SLA.
Twilio Proxy is exposed as a REST API that allows you to create masked/anonymized text and voice conversations between users.
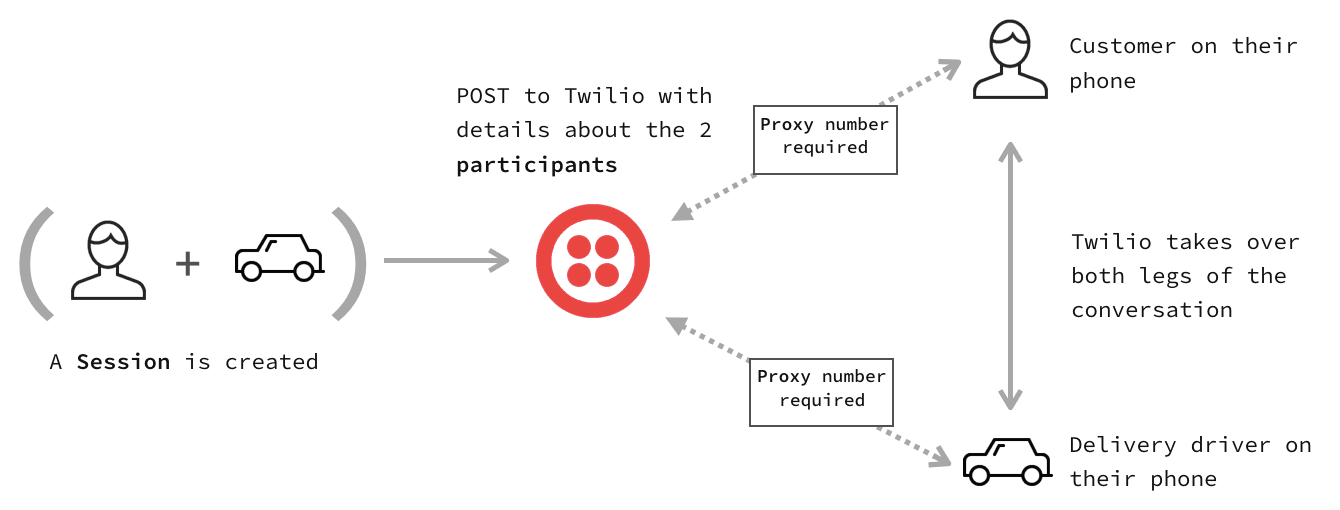
The Proxy REST API allows you to create and manage:
Additionally, you can add phone numbers with Voice or SMS capabilities to a phone number pool.
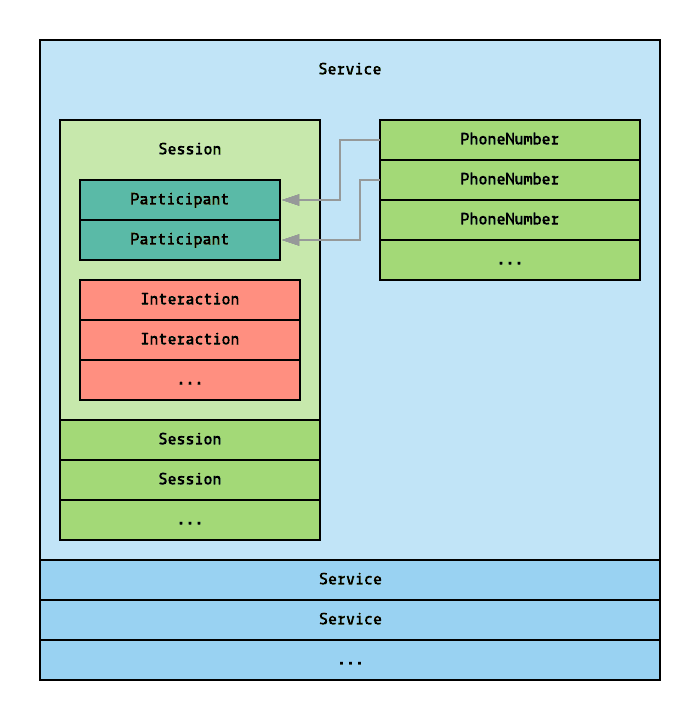
All URLs in the reference documentation use the following base URL:
https://proxy.twilio.com/v1
All requests to the Proxy REST API are served over HTTPS. Unencrypted HTTP is not supported.
To authenticate requests to the Twilio APIs, Twilio supports HTTP Basic authentication. Use your API key as the username and your API key secret as the password. You can create an API key either in the Twilio Console or using the API.
Note: Twilio recommends using API keys for authentication in production apps. For local testing, you can use your Account SID as the username and your Auth token as the password. You can find your Account SID and Auth Token in the Twilio Console.
Learn more about Twilio API authentication.
If you use one of our helper libraries for C#, Java, Node.js, PHP, Python, or Ruby; you needn't worry about the URL for the API or how to do HTTP Basic authentication. The helper libraries take care of it for you.
Refer to our quickstart guide for a step-by-step introduction to Proxy, or browse the Proxy resources listed in the left-hand navigation for API Reference material.