Build a Simple Phone Verification System with Twilio, PHP, MySQL, and jQuery
Time to read: 4 minutes
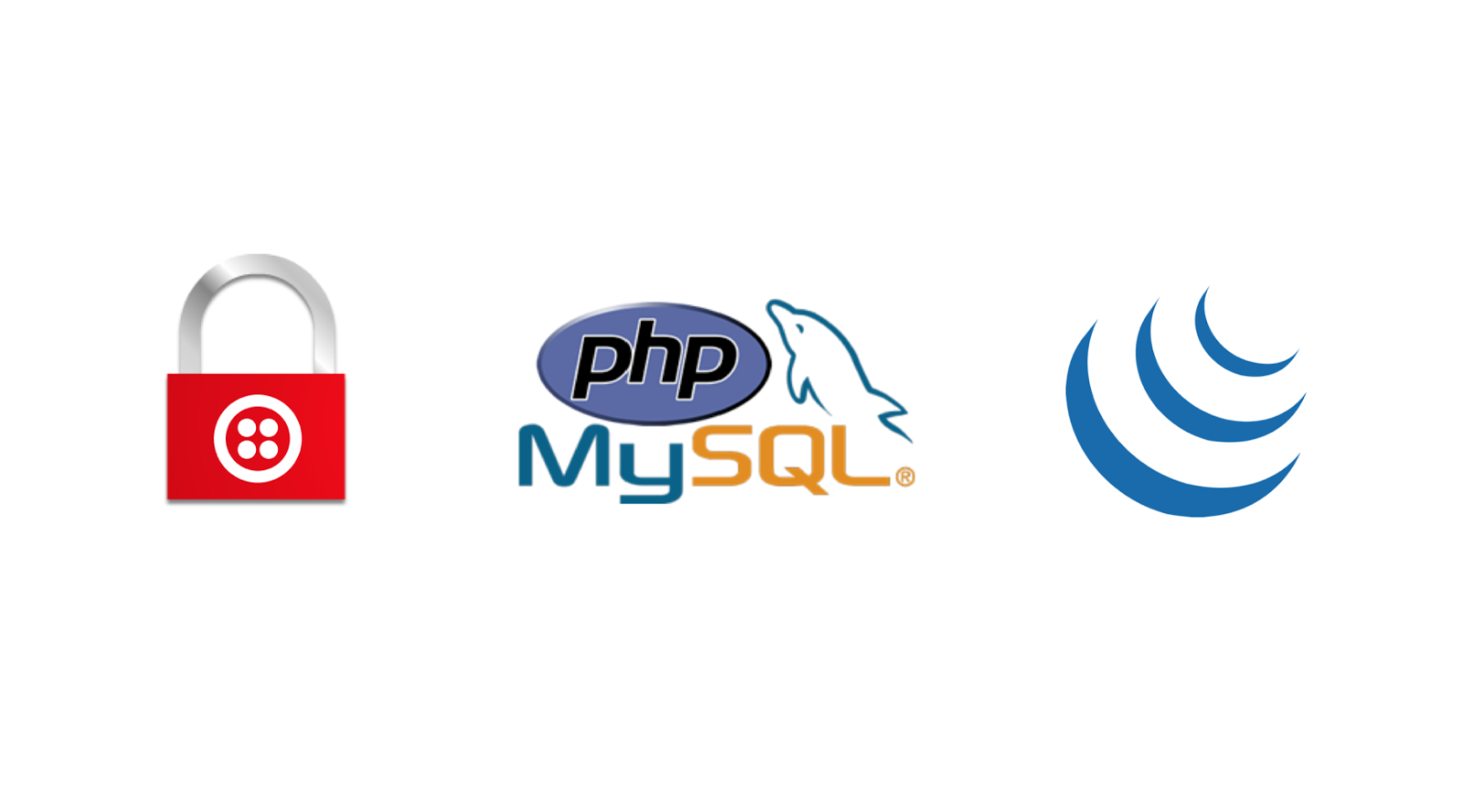
Verifying the phone numbers of your users is a fast, effective way to tamp down on fraud and spam accounts. This post will help you build such a system using Twilio Voice, PHP 7, MySQL 5.x and jQuery.
Below we’ll walk through the code but if you have an existing MAMP environment or equivalent you can download the complete example here and get started. To run this example on your own server, update the database settings in database.php
and your Twilio account settings in call.php
. This example also makes use of our Twilio PHP Helper library installed via Composer.
Basic steps
- User visits verification web page and enters phone number.
- Random verification code is generated and user is called and prompted to enter code.
- If code is entered incorrectly, re-prompt to enter code.
- If code is entered correctly, update database.
- Update web page with status message.
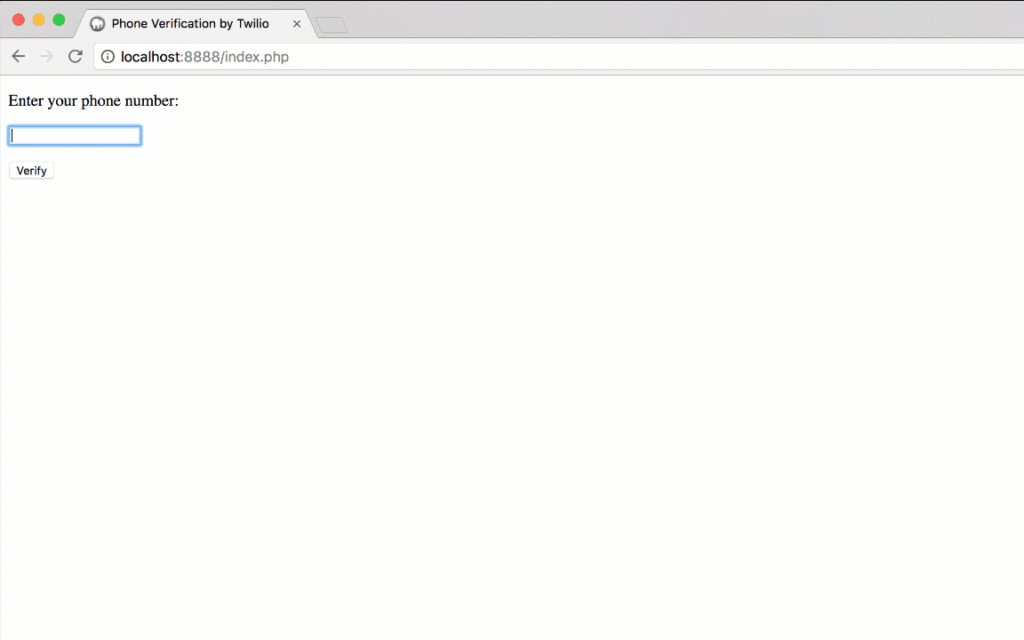
Step Zero: MySQL Database setup
If you already have MySQL setup, skip this step. We’ll be managing our MySQL database with MAMP. Once you’ve installed and setup the GUI, you should be able to reach your server on localhost:8888
or whatever port MAMP has directed you to.
It looks something like this:
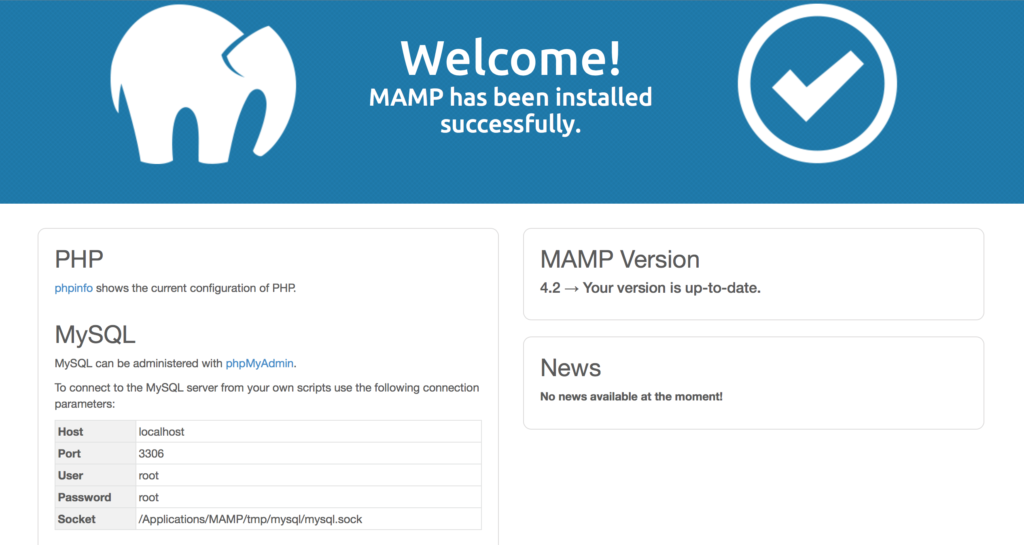
Keep the
Host
, User
and Password
values handy for later. You can then access the phpMyAdmin interface by clicking it from there where we will take the next steps.
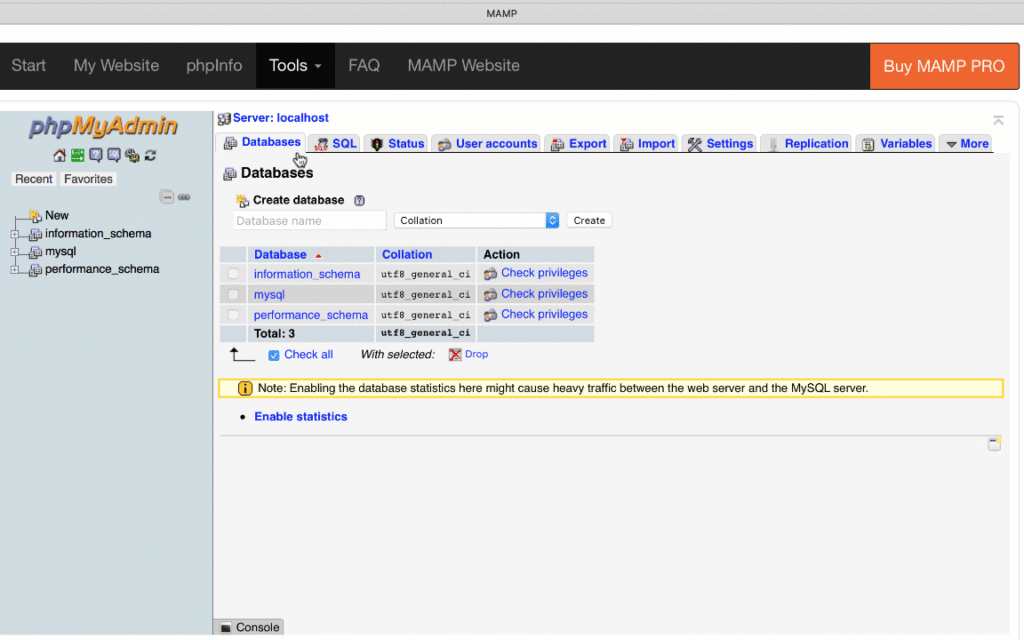
On this page you will want to create a new database, I’ve called it
verify
and set the Collation to utf8_general_ci
. We will then setup the database schema with one table called numbers
.
We’ll be storing the phone number along with the generated verification code in a MySQL database so that we can update the verified user info an retrieve it later. For this example there is a simple table definition which you can store as dbschema.sql
and can be added directly to the phpMyAdmin interface as well. Copy and paste the code below in the SQL tab as the following clip shows.
You will want to be sure to save all of the files we create for this post in the MAMP folder htdocs
. The full path should look like Applications/MAMP/htdocs
.
If you’ve chosen not to download the completed project, create a file called database.php
and place the following code in it remembering to update it with your database info if it does not match the default values.
Step One: The verification web page
We’ll start with the HTML the user will see. Create a file called index.php and add the following code to the :
When the user visits the page they’ll be presented with the form asking them for their phone number. Please note that while we don’t verify the phone number in this example, in a production environment you would want to make sure the phone number is E.164 formatted. Once entered, we’ll hide the form and show them their verification code. Here’s the jQuery needed to make that transition as referenced in the html above in main.js
:
Our initiateCall()
function kicks off an AJAX request to generate the random code and initiate the phone call, which we will address later. Once we’ve got the code, we’ll update the UI to show it to the user and start polling our database for status updates. The code to poll the server looks like this in status.php
:
Once we’ve got confirmation from the server that the number has been verified, we update the UI to let the user know.
Step Two: Generate and display random code
Step two in our walkthrough overlaps with step one since it is initiated when the user submits the form with their phone number. We’ll pick up in our initiateCall()
function which makes the AJAX POST request to call.php
. Inside call.php
we generate the random 6-digit verification code and start the phone call with the Twilio REST API using the PHP Helper Library. If you haven’t already, now would be a good time to run the following command in your project directory:
You will also want to replace the $accountSid , $authToken , $outgoingNumber , and $endPoint variables with your own information.
Once we have the verification code we’ll send it back as JSON for the browser to parse and display.
Steps Three and Four: Collect and verify code via phone call
When initiating the call we direct Twilio to use the twiml.php
file which generates the necessary <Gather> TwiML to prompt the caller to enter their code. The first time this file is requested we’ll ask the caller to enter their code. Once they’ve entered 6 digits, Twilio will make another post to the same URL with the Digits POST
parameter now included. With this information we look up the caller’s phone number in the database and check for a match. If the code entered is incorrect, we’ll re-prompt them to enter it again. Once they’ve entered the correct code we update the database and thank them for calling.
Step Five: Update the web page when the number is verified
Back in Step One we created the jQuery needed to begin polling the server for status updates. Our checkStatus()
function makes a POST
to status.php
which looks up the phone number in the database and sends back some JSON containing the verification status.
Read more about Phone Verification
Documentation: Authy for Two Factor Authentication
Blog post: SMS Phone Verification in Rails 4 with AJAX and Twilio
Blog post: How to Build a Phone-Based Two-Factor Authentication
Blog post: Phone-Based Two-factor Authentication Is A Better Way to Stay Secure
Blog post: The Key to Phone Verification is a Good User Experience
That’s all folks!
We did it. Simple phone verification mission accomplished. Many thanks to Jonathan Gottfried for his inspiration from this post in its original incarnation. And shoutout to my colleague Margaret Staples for lending her PHP expertise.
If you have any questions or ideas for your next project, please find us on twitter or email mspeir@twilio.com.
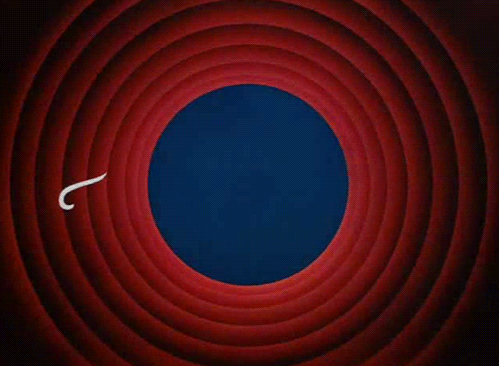
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.