Set up your Ruby and Sinatra development environment
In this guide, we will cover how to set up your Ruby development environment for a Sinatra project. We will also talk about a couple helpful tools that we recommend for all Ruby applications that use Twilio: Ngrok and the Twilio Ruby SDK.
1# Check your Ruby version2$ ruby --version3ruby 2.3.1p112 (2016-04-26 revision 54768)
If Ruby is already installed on your system, you can check its version by running ruby --version
.
How you install Ruby varies depending on your operating system.
Operating System | Instructions |
---|---|
OS X | The easiest way to install Ruby on OS X is to use the official installer from ruby-lang.org. You can also use Homebrew if you prefer. |
Windows | The easiest way to install Ruby on Windows is the official installer from RubyInstaller. You can also use Chocolatey if you prefer. |
Linux | The exact instructions to install Ruby vary by distribution. Find instructions for yours here. |
Ruby Version Manager or RVM, is a Unix-like software platform designed to manage multiple installations of Ruby on the same device.
1gpg --keyserver hkp://keys.gnupg.net --recv-keys 409B6B1796C275462A1703113804BB82D39DC0E32curl -sSL https://get.rvm.io | bash -s stable --ruby
Bundler is a dependency manager that provides a consistent environment for Ruby projects by tracking and installing the exact gems and versions that are needed.
We have to install Bundler to manage the dependencies for the project.
gem install bundler
Before we can start our Ruby project we'll need something to write it with.
If you already have a code-writing tool of choice, you can stick with it for developing your Ruby application. If you're looking for something new, we recommend trying out a few options:
- Atom is an IDE built with HTML, JavaScript, CSS, and Node.js integration. It runs on Electron, a framework for building cross-platform apps using web technologies.
- Sublime Text is a text editor popular for its ease of use and extensibility. Start here if you're eager to get coding and don't think you'll want a lot of frills in your development environment.
- RubyMine is a fully Integrated Development Environment (IDE) for Ruby. It takes longer to set up but comes with more helpful tools already installed.
- Vim is a perennial favorite text editor among advanced users
If you're new to programming, we recommend giving Atom and Sublime Text each a try before you settle on your favorite.
We're almost ready to start writing our Sinatra web application, but first, we need to install the Sinatra library.
First, you need a Gemfile with the following content on it.
1# Gemfile2source 'https://rubygems.org'34gem 'sinatra'5gem 'twilio-ruby'
Ruby projects use Bundler to manage dependencies, so the command to pull Sinatra and the Twilio SDK into our development environment is bundle install
.
1$ bundle install2Using builder 3.2.23Using jwt 1.5.64Using multi_json 1.12.15Using rack 1.6.56Using tilt 2.0.57Using bundler 1.13.38Using twilio-ruby 4.13.09Using rack-protection 1.5.310Using sinatra 1.4.711Bundle complete! 2 Gemfile dependencies, 9 gems now installed.12Use `bundle show [gemname]` to see where a bundled gem is installed.
We can test that our development environment is configured correctly by creating a simple Sinatra application. We'll grab the example from Sinatra's documentation and drop it in a new file called index.rb
.
1# index.rb2require 'sinatra'3require 'twilio-ruby'45get '/' do6content_type 'text/xml'78Twilio::TwiML::VoiceResponse.new do | response |9response.say(message: 'Hello World')10end.to_s11end
We can then try running our new Sinatra application with the command ruby index.rb -p 3000
. You can then open http://localhost:3000
in your browser and you should see the <?xml version="1.0" encoding="UTF-8"?><Response><Say>Hello World</Say></Response>
response.
Once you see your sample Sinatra application's "<?xml version="1.0" encoding="UTF-8"?><Response><Say>Hello World</Say></Response>" message, your development environment is ready to go. But for most Twilio projects you'll want to install one more helpful tool: ngrok.
Most Twilio services use webhooks to communicate with your application. When Twilio receives an incoming phone call, for example, it reaches out to a URL in your application for instructions on how to handle the call.
When you're working on your Sinatra application in your development environment, your app is only reachable by other programs on the same computer, so Twilio won't be able to talk to it.
Ngrok is our favorite tool for solving this problem. Once started, it provides a unique URL on the ngrok.io domain which will forward incoming requests to your local development environment.
To start, head over to the ngrok download page and grab the binary for your operating system: https://ngrok.com/download
Once downloaded, make sure your Sinatra application is running and then start ngrok using this command: "./ngrok http 3000". You should see output similar to this:
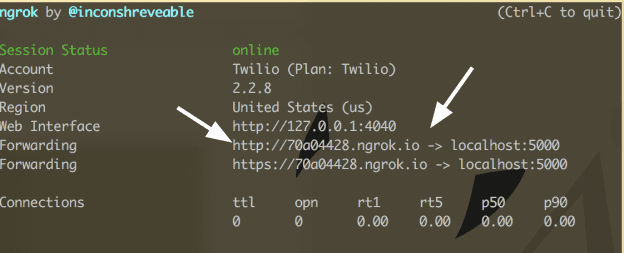
Look at the "Forwarding" line to see your unique Ngrok domain name (ours is "aaf29606.ngrok.io") and then point your browser at that domain name.
If everything's working correctly, you should see your Sinatra application's "<?xml version="1.0" encoding="UTF-8"?><Response><Say>Hello World</Say></Response>" message displayed at your new Ngrok URL.
Anytime you're working on your Twilio application and need a URL for a webhook you should use Ngrok to get a publicly accessible URL like this one.
You're ready to build out your Sinatra application. Learn more with the following resources: