Migrating from Facebook Account Kit to Twilio Verify
Facebook announced that they are deprecating Account Kit and services will no longer be available starting in March 2020. Twilio Verify offers end to end functionality to quickly and easily replace your application's dependency on Account Kit for SMS login. This tutorial will cover implementing Twilio Verify in web applications.
- Check out a sample application on Glitch
- Look at the finished code on my GitHub.
- Compare before and after code: FB account kit vs Twilio Verify
- Skip ahead to the tutorial
With a similar workflow to Facebook's Account Kit, you can use your existing UI/UX with minimal code changes to integrate Verify. Verify offers:
- global coverage
- fast performance
- high scale
- token management
...all out of the box.
Like Account Kit, Verify manages sending and checking verification tokens for you so there are limited backend changes required to move to this API.
With 99.999% of API uptime, you can rely on Verify to automatically handle delivery using a robust global SMS and voice network covering 200+ countries. Verify email delivery channel is expected to be in Public Beta in November 2019 and GA in early 2020. Build WhatsApp channel separately with Twilio's WhatsApp API. WhatsApp support through Verify is not currently available; if this is something that would be useful to your company please let us know. Twilio Verify does not support instant verification but does support a voice channel.
To code along with this tutorial, you'll need:
- A Twilio account
- A Verify Service which you can create in the Twilio console. I named mine "Account Kit Migration Example"
Make note of your Service SID (starts with VA)
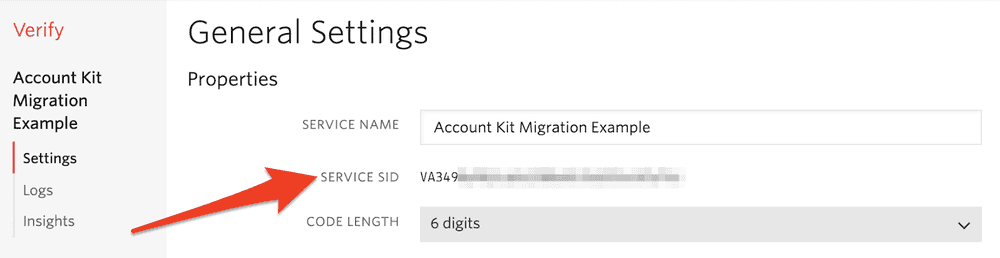
We don't want to store our API Keys directly in our front-end application, so we'll need a backend server that will talk to the Verify API. Account Kit handled some of this with their JavaScript SDK, we'll do this with Twilio Functions.
Info
Twilio Functions provide a serverless environment to build and run Twilio applications so you can get to production faster. Your first 10,000 invocations/month are free. Learn more about our runtime products to build, deploy, and operate apps at scale.
Head over to your Twilio Function configuration and:
- Check the box to Enable ACCOUNT_SID and AUTH_TOKEN
- Add VERIFY_SERVICE_SID as an environment variable (create a service here if you haven't already)
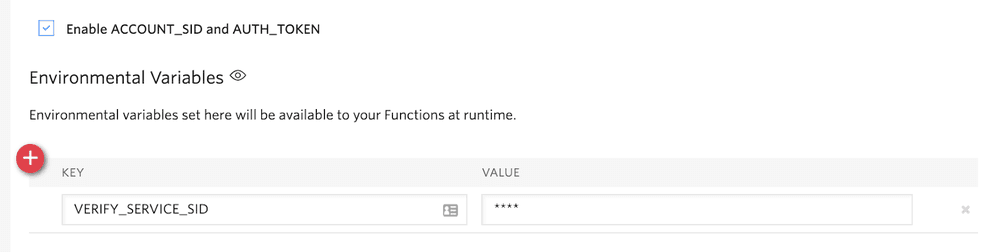
Then create two Twilio Functions, one to start and one to check verifications. For each function:
-
Choose "Blank"
-
Replace the code with the code in the template file:
-
In properties, give your function a name and add a relevant path (start or check)
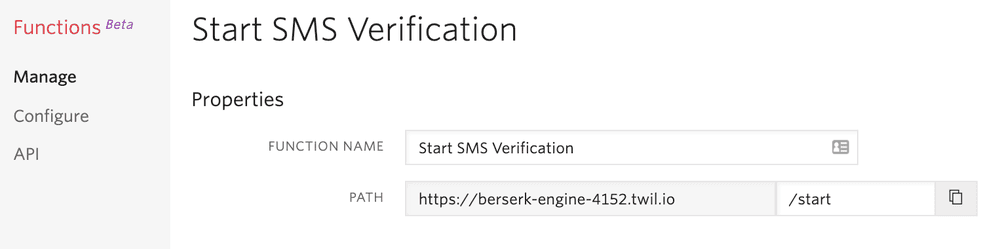
Make note of your function path, we'll need that soon.
Start with a basic HTML page like this or use your existing web application.
Import Bootstrap and jQuery by adding these lines in your <head>
tag. These will be used for some basic styling and adding a modal so we can check the verification code. You can skip the Bootstrap CSS if you have your own styling.
1<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" integrity="sha384-BVYiiSIFeK1dGmJRAkycuHAHRg32OmUcww7on3RYdg4Va+PmSTsz/K68vbdEjh4u" crossorigin="anonymous">2<script src="https://code.jquery.com/jquery-3.4.1.min.js" integrity="sha256-CSXorXvZcTkaix6Yvo6HppcZGetbYMGWSFlBw8HfCJo=" crossorigin="anonymous"></script>3<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js" integrity="sha384-Tc5IQib027qvyjSMfHjOMaLkfuWVxZxUPnCJA7l2mCWNIpG9mGCD8wGNIcPD7Txa" crossorigin="anonymous"></script>
1<div class="container">2<p>Enter your phone number to receive a verification code:</p>3<form class="form-inline">4<input value="+1" id="country_code" class="form-control"/>5<input placeholder="phone number" id="phone_number" class="form-control"/>6<input type="button" onclick="smsLoginTwilio();" class="btn btn-primary" value="Login via SMS" />7</form>8</div>
Add the following code to your file. Replace the URL in the fetch
call with the URL to your Twilio Function and a path of /start
.
1<script>2var cc = null;3var pn = null;45function flashStatus(alertType, message) {6var content = $(".result-message");7content.empty();8content.append(`<div class="alert alert-${alertType}" role="alert">${message}</div>`);9}1011function smsLoginTwilio() {12var countryCode = $("#country_code").val().replace(/\W/g, ''); // strip special characters13var phoneNumber = $("#phone_number").val().replace(/\W/g, ''); // strip special characters1415// save for checking16cc = countryCode;17pn = phoneNumber;1819// Twilio functions do not accept multipart/form-data20const data = new URLSearchParams();21data.append("country_code", countryCode);22data.append("phone_number", phoneNumber);2324// TODO REPLACE THIS25fetch("your-twilio-function-start-verify-url/start", {26method: 'POST',27body: data28})29.then(response => {30return response.json()31})32.then((json) => {33console.log(json);34if (json.success) {35console.log("Successfully sent token.")36} else {37console.log("Error sending token.")38}39})40.catch((err) => {41console.log(err);42});43}44</script>
Open up developer tools and open your file in the browser. Input your phone number in the phone number field and hit "Login via SMS". You should receive an SMS with a 6 digit code and see the following output in the console.
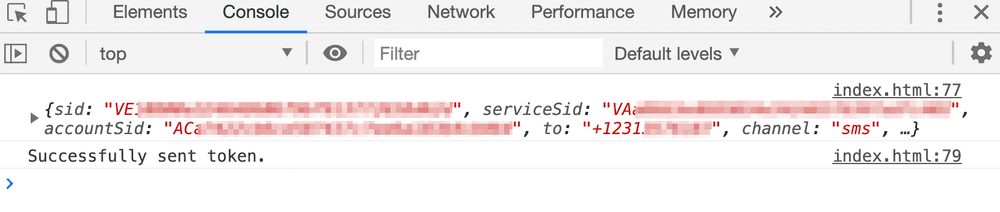
Add the following modal HTML in your body tag so we can verify the token sent to the user. In your implementation, this could also redirect to a new page.
1<div class="modal" id="verification_modal">2<div class="modal-dialog" role="document">3<div class="modal-content">4<div class="modal-body">5<div class="row">6<div class="col-lg-12">7<div class="result-message">8</div>9<div class="input-group input-group-lg">10<input type="text" class="form-control input-lg" id="verification_code" placeholder="Enter the token sent to your device">11<span class="input-group-btn">12<button onclick="smsVerifyTwilio();" class="btn btn-primary btn-lg" type="submit">Verify</button>13</span>14</div>15</div>16</div>17</div>18</div><!-- /.modal-content -->19</div><!-- /.modal-dialog -->20</div><!-- /.modal -->
Then update your smsWithTwilio()
function to open the modal if the json response indicates success:
1if (json.success) {2$(".modal").modal("show");3console.log("Successfully sent token.");4}
Now if you click "Login with SMS" you should see the verification modal:
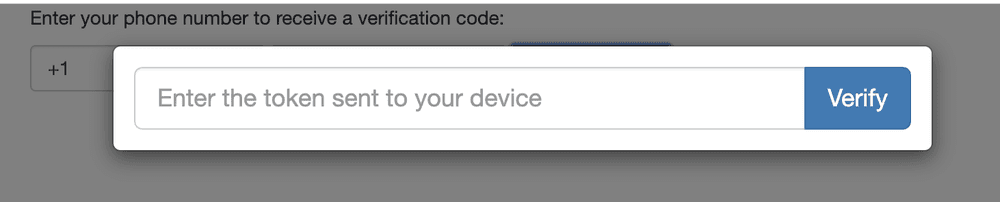
Finally, add a function to verify the token in your script tag. Replace the URL in the fetch
call with the URL to your Twilio function and a path of /check
.
1function smsVerifyTwilio() {2var code = $("#verification_code").val();34// Twilio functions do not accept multipart/form-data5const data = new URLSearchParams();6data.append("country_code", cc);7data.append("phone_number", pn);8data.append("verification_code", code);910// TODO REPLACE THIS11fetch("your-twilio-function-start-verify-url/check", {12method: 'POST',13body: data14})15.then(response => response.json())16.then((json) => {17if (json.success) {18flashStatus("success", "Success!");19console.log("Successfully verified the token.");20} else {21flashStatus("danger", json.message);22$("#verification_code").val("");23console.log("Incorrect token.")24}25})26.catch((err) => {27var content = $(".result-message");28console.log(err);29});30}
Click 'Login with SMS' once more and now if you enter the token sent to your phone you'll see if it was correct!
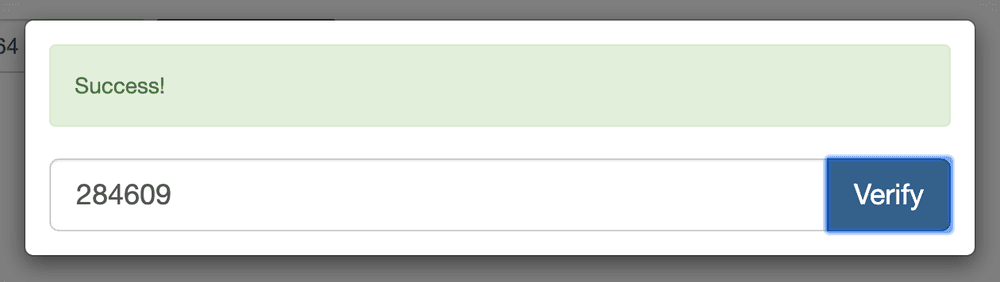
With just two API calls and one web page, you've now validated that a user has a real phone number and access to that number, which will help prevent a lot of fraudulent accounts and ensure that your users can receive other text or voice notifications from your app.
More links that may be helpful:
- Check out a sample application on Glitch
- Look at the finished code on my GitHub.
- Compare code: FB account kit vs Twilio Verify
- Talk to Sales about a solution for your company
If you have any questions about phone verification or account security, please leave a comment or contact me on Twitter.