Retrieve Call Logs with Node.js
In this guide we'll show you how to how to retrieve information about in progress and completed calls from your Twilio account. The code snippets in this guide are written using modern JavaScript language features in Node.js version 6 or higher and make use of the Twilio Node.js SDK.
Let's get started!
The first thing we will need are your account credentials.
First, you'll need to get your Twilio account credentials. They consist of your AccountSid and your Auth Token. They can be found on the home page of the console.
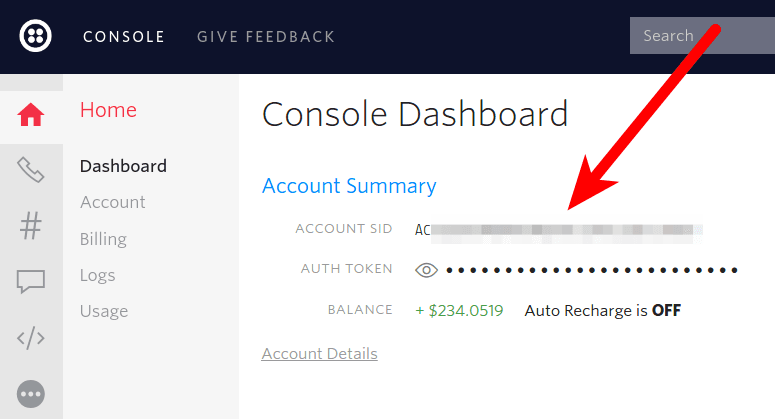
With our account credentials, we can us the Twilio Node.js module to retrieve Twilio call logs.
You can use Twilio's REST API to retrieve logs about the phone calls to and from your Twilio account. If you just want to check a couple logs, however, you should try looking at the voice logs in your Twilio console first.
To list all phone calls for your Twilio account, just call client.calls.list()
.
Info
The list
method automatically handles paging for you, eagerly fetching all records and paging under the hood. For more information, visit the Node.js Helper Library page.
1// Download the helper library from https://www.twilio.com/docs/node/install2const twilio = require("twilio"); // Or, for ESM: import twilio from "twilio";34// Find your Account SID and Auth Token at twilio.com/console5// and set the environment variables. See http://twil.io/secure6const accountSid = process.env.TWILIO_ACCOUNT_SID;7const authToken = process.env.TWILIO_AUTH_TOKEN;8const client = twilio(accountSid, authToken);910async function listCall() {11const calls = await client.calls.list({ limit: 20 });1213calls.forEach((c) => console.log(c.sid));14}1516listCall();
Response
Note: This shows the raw API response from Twilio. Responses from SDKs (Java, Python, etc.) may look a little different.1{2"calls": [3{4"account_sid": "ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",5"annotation": "billingreferencetag1",6"answered_by": "machine_start",7"api_version": "2010-04-01",8"caller_name": "callerid1",9"date_created": "Fri, 18 Oct 2019 17:00:00 +0000",10"date_updated": "Fri, 18 Oct 2019 17:01:00 +0000",11"direction": "outbound-api",12"duration": "4",13"end_time": "Fri, 18 Oct 2019 17:03:00 +0000",14"forwarded_from": "calledvia1",15"from": "+13051416799",16"from_formatted": "(305) 141-6799",17"group_sid": "GPdeadbeefdeadbeefdeadbeefdeadbeef",18"parent_call_sid": "CAdeadbeefdeadbeefdeadbeefdeadbeef",19"phone_number_sid": "PNdeadbeefdeadbeefdeadbeefdeadbeef",20"price": "-0.200",21"price_unit": "USD",22"sid": "CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",23"start_time": "Fri, 18 Oct 2019 17:02:00 +0000",24"status": "completed",25"subresource_uris": {26"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Notifications.json",27"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Recordings.json",28"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Payments.json",29"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Events.json",30"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Siprec.json",31"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Streams.json",32"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Transcriptions.json",33"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessageSubscriptions.json",34"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessages.json"35},36"to": "+13051913581",37"to_formatted": "(305) 191-3581",38"trunk_sid": "TKdeadbeefdeadbeefdeadbeefdeadbeef",39"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json",40"queue_time": "1000"41},42{43"account_sid": "ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",44"annotation": "billingreferencetag2",45"answered_by": "human",46"api_version": "2010-04-01",47"caller_name": "callerid2",48"date_created": "Fri, 18 Oct 2019 16:00:00 +0000",49"date_updated": "Fri, 18 Oct 2019 16:01:00 +0000",50"direction": "inbound",51"duration": "3",52"end_time": "Fri, 18 Oct 2019 16:03:00 +0000",53"forwarded_from": "calledvia2",54"from": "+13051416798",55"from_formatted": "(305) 141-6798",56"group_sid": "GPdeadbeefdeadbeefdeadbeefdeadbeee",57"parent_call_sid": "CAdeadbeefdeadbeefdeadbeefdeadbeee",58"phone_number_sid": "PNdeadbeefdeadbeefdeadbeefdeadbeee",59"price": "-0.100",60"price_unit": "JPY",61"sid": "CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0",62"start_time": "Fri, 18 Oct 2019 16:02:00 +0000",63"status": "completed",64"subresource_uris": {65"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Notifications.json",66"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Recordings.json",67"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Payments.json",68"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Events.json",69"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Siprec.json",70"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Streams.json",71"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Transcriptions.json",72"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/UserDefinedMessageSubscriptions.json",73"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/UserDefinedMessages.json"74},75"to": "+13051913580",76"to_formatted": "(305) 191-3580",77"trunk_sid": "TKdeadbeefdeadbeefdeadbeefdeadbeef",78"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0.json",79"queue_time": "1000"80}81],82"end": 1,83"first_page_uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=0",84"next_page_uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=1&PageToken=PACAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0",85"page": 0,86"page_size": 2,87"previous_page_uri": null,88"start": 0,89"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=0"90}
You can also filter the results. This example only returns phone calls to the phone number "+15558675309" which had a call status of "busy" but you can filter on other call properties as well.
1// Download the helper library from https://www.twilio.com/docs/node/install2const twilio = require("twilio"); // Or, for ESM: import twilio from "twilio";34// Find your Account SID and Auth Token at twilio.com/console5// and set the environment variables. See http://twil.io/secure6const accountSid = process.env.TWILIO_ACCOUNT_SID;7const authToken = process.env.TWILIO_AUTH_TOKEN;8const client = twilio(accountSid, authToken);910async function listCall() {11const calls = await client.calls.list({12status: "busy",13to: "+15558675310",14limit: 20,15});1617calls.forEach((c) => console.log(c.sid));18}1920listCall();
Response
Note: This shows the raw API response from Twilio. Responses from SDKs (Java, Python, etc.) may look a little different.1{2"calls": [3{4"account_sid": "ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",5"annotation": "billingreferencetag1",6"answered_by": "machine_start",7"api_version": "2010-04-01",8"caller_name": "callerid1",9"date_created": "Fri, 18 Oct 2019 17:00:00 +0000",10"date_updated": "Fri, 18 Oct 2019 17:01:00 +0000",11"direction": "outbound-api",12"duration": "4",13"end_time": "Fri, 18 Oct 2019 17:03:00 +0000",14"forwarded_from": "calledvia1",15"from": "+13051416799",16"from_formatted": "(305) 141-6799",17"group_sid": "GPdeadbeefdeadbeefdeadbeefdeadbeef",18"parent_call_sid": "CAdeadbeefdeadbeefdeadbeefdeadbeef",19"phone_number_sid": "PNdeadbeefdeadbeefdeadbeefdeadbeef",20"price": "-0.200",21"price_unit": "USD",22"sid": "CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",23"start_time": "Fri, 18 Oct 2019 17:02:00 +0000",24"status": "completed",25"subresource_uris": {26"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Notifications.json",27"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Recordings.json",28"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Payments.json",29"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Events.json",30"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Siprec.json",31"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Streams.json",32"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Transcriptions.json",33"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessageSubscriptions.json",34"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessages.json"35},36"to": "+13051913581",37"to_formatted": "(305) 191-3581",38"trunk_sid": "TKdeadbeefdeadbeefdeadbeefdeadbeef",39"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json",40"queue_time": "1000"41},42{43"account_sid": "ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",44"annotation": "billingreferencetag2",45"answered_by": "human",46"api_version": "2010-04-01",47"caller_name": "callerid2",48"date_created": "Fri, 18 Oct 2019 16:00:00 +0000",49"date_updated": "Fri, 18 Oct 2019 16:01:00 +0000",50"direction": "inbound",51"duration": "3",52"end_time": "Fri, 18 Oct 2019 16:03:00 +0000",53"forwarded_from": "calledvia2",54"from": "+13051416798",55"from_formatted": "(305) 141-6798",56"group_sid": "GPdeadbeefdeadbeefdeadbeefdeadbeee",57"parent_call_sid": "CAdeadbeefdeadbeefdeadbeefdeadbeee",58"phone_number_sid": "PNdeadbeefdeadbeefdeadbeefdeadbeee",59"price": "-0.100",60"price_unit": "JPY",61"sid": "CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0",62"start_time": "Fri, 18 Oct 2019 16:02:00 +0000",63"status": "completed",64"subresource_uris": {65"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Notifications.json",66"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Recordings.json",67"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Payments.json",68"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Events.json",69"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Siprec.json",70"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Streams.json",71"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/Transcriptions.json",72"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/UserDefinedMessageSubscriptions.json",73"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0/UserDefinedMessages.json"74},75"to": "+13051913580",76"to_formatted": "(305) 191-3580",77"trunk_sid": "TKdeadbeefdeadbeefdeadbeefdeadbeef",78"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0.json",79"queue_time": "1000"80}81],82"end": 1,83"first_page_uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=0",84"next_page_uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=1&PageToken=PACAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa0",85"page": 0,86"page_size": 2,87"previous_page_uri": null,88"start": 0,89"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls.json?Status=completed&To=%2B123456789&From=%2B987654321&StartTime=2008-01-02&ParentCallSid=CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa&EndTime=2009-01-02&PageSize=2&Page=0"90}
Finally, if you just want to retrieve information about a specific call you can get that CallSid directly.
1// Download the helper library from https://www.twilio.com/docs/node/install2const twilio = require("twilio"); // Or, for ESM: import twilio from "twilio";34// Find your Account SID and Auth Token at twilio.com/console5// and set the environment variables. See http://twil.io/secure6const accountSid = process.env.TWILIO_ACCOUNT_SID;7const authToken = process.env.TWILIO_AUTH_TOKEN;8const client = twilio(accountSid, authToken);910async function fetchCall() {11const call = await client.calls("CA42ed11f93dc08b952027ffbc406d0868").fetch();1213console.log(call.to);14}1516fetchCall();
Response
Note: This shows the raw API response from Twilio. Responses from SDKs (Java, Python, etc.) may look a little different.1{2"account_sid": "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",3"annotation": "billingreferencetag",4"answered_by": "machine_start",5"api_version": "2010-04-01",6"caller_name": "callerid",7"date_created": "Fri, 18 Oct 2019 17:00:00 +0000",8"date_updated": "Fri, 18 Oct 2019 17:01:00 +0000",9"direction": "outbound-api",10"duration": "4",11"end_time": "Fri, 18 Oct 2019 17:03:00 +0000",12"forwarded_from": "calledvia",13"from": "+13051416799",14"from_formatted": "(305) 141-6799",15"group_sid": "GPdeadbeefdeadbeefdeadbeefdeadbeef",16"parent_call_sid": "CAdeadbeefdeadbeefdeadbeefdeadbeef",17"phone_number_sid": "PNdeadbeefdeadbeefdeadbeefdeadbeef",18"price": "-0.200",19"price_unit": "USD",20"sid": "CA42ed11f93dc08b952027ffbc406d0868",21"start_time": "Fri, 18 Oct 2019 17:02:00 +0000",22"status": "completed",23"subresource_uris": {24"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Notifications.json",25"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Recordings.json",26"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Payments.json",27"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Events.json",28"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Siprec.json",29"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Streams.json",30"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Transcriptions.json",31"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessageSubscriptions.json",32"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessages.json"33},34"to": "+13051913581",35"to_formatted": "(305) 191-3581",36"trunk_sid": "TKdeadbeefdeadbeefdeadbeefdeadbeef",37"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json",38"queue_time": "1000"39}
We just learned how to retrieve Twilio call logs using JavaScript. Check out our tutorials to see full implementations of Twilio Voice in Node.js with Express.