Record Phone Calls in Node.js
In this guide we'll show you how to use Programmable Voice to record phone calls with your Node.js web application. You can tell Twilio to record part of a phone call or the entire thing. The code snippets in this guide are written using modern JavaScript language features in Node.js version 6 or higher, and make use of the following modules:
Let's get started!
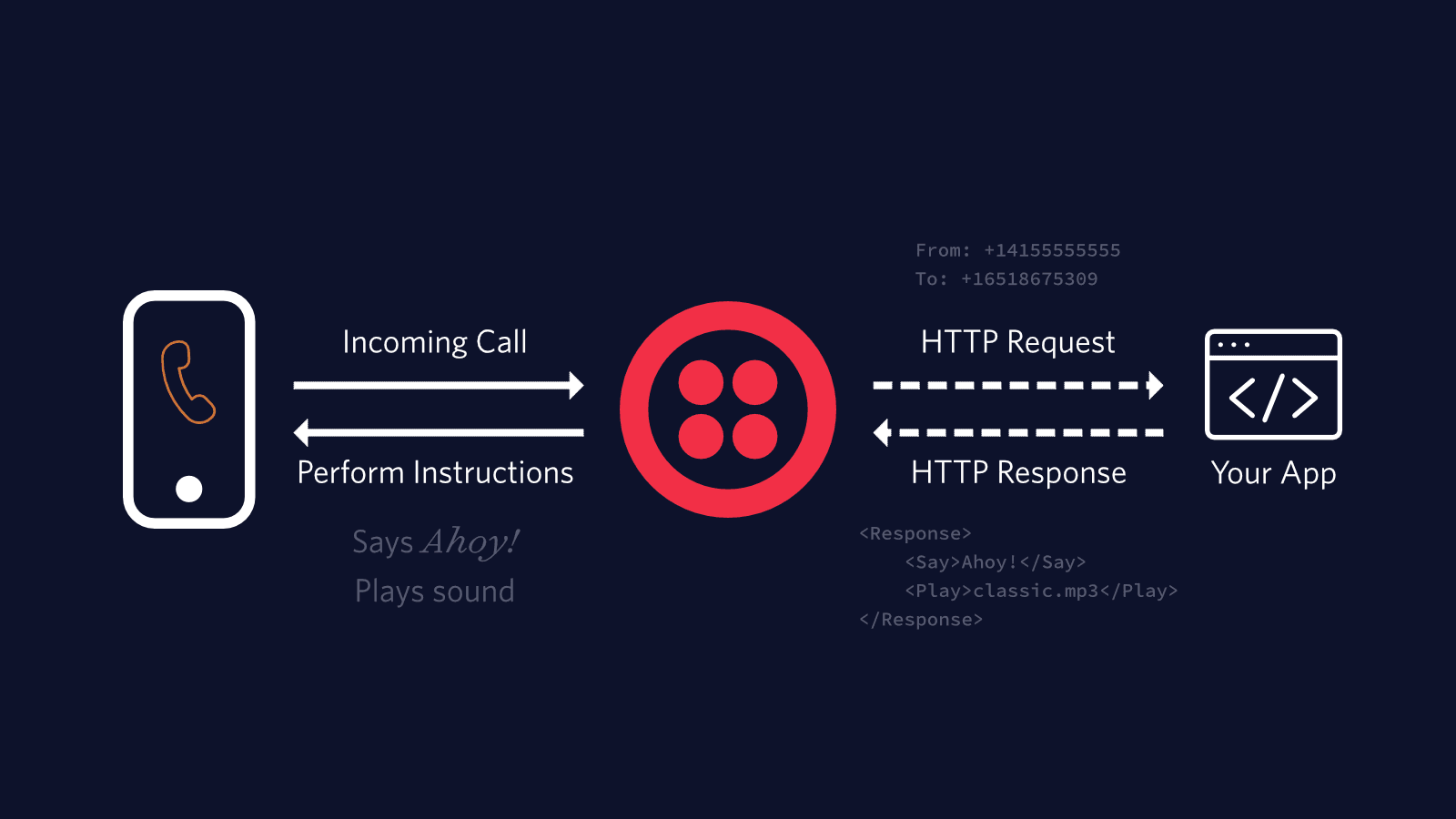
When a phone number you have bought through Twilio receives an incoming call, Twilio will send an HTTP request to a server you control, asking for instructions on how to handle the call. Your server will respond with an XML document containing TwiML that tells Twilio to read out a message, play an MP3 file, make a recording, and much more.
To start answering phone calls, you must:
- Buy and configure a Twilio-powered phone number capable of making and receiving phone calls, and point it at your web application
- Write web application code to tell Twilio how to handle the incoming call (using TwiML)
- Make your web application accessible on the Internet so Twilio can send you a webhook request when you receive a call
Warning
If you are sending SMS messages to the U.S. or Canada, before proceeding further, be aware of updated restrictions on the use of Toll-Free numbers for messaging, including TF numbers obtained by purchasing them. These restrictions do not apply to Voice or other uses outside of SMS messaging. See this support article for details.
In the console, you can search for and buy phone numbers in dozens of different countries, capable of calling (and being called by) just about every phone on the planet.
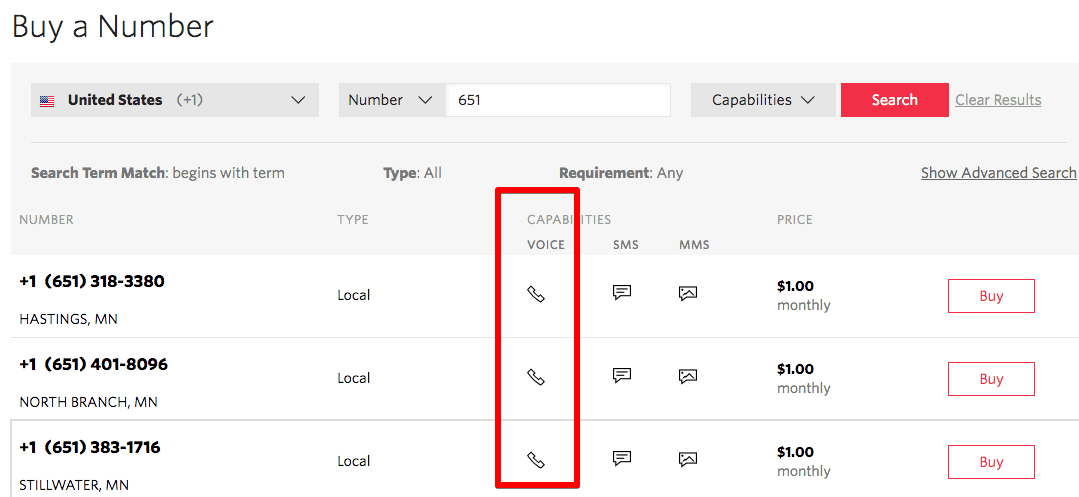
Once you purchase a number, you'll need to configure that number to send a request to your web application. This callback mechanism is called a webhook. This can be done in the number's configuration page.
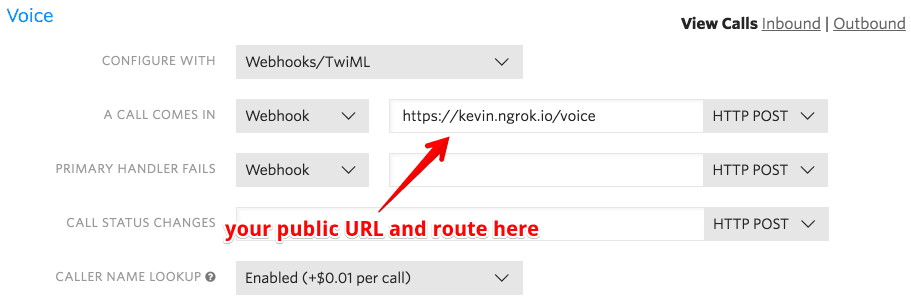
A webhook is a callback mechanism that allows two systems to communicate events to one another over the Internet using HTTP requests. In this case, Twilio is sending a webhook request to your web application whenever a phone number you control receives an incoming call. You'll see this webhook mechanism used in many Twilio APIs for handling event notifications like this.
Not working on a server with a public URL? We'll show you how to expose your local development machine to the public Internet later in this guide. Next, you'll need to write some server-side code that will be executed when an incoming call comes in.
Now comes the fun part - writing code that will handle an incoming HTTP request from Twilio!
In this example we'll use the Express web framework for Node.js to respond to Twilio's request and we'll use TwiML to tell Twilio how to handle the call.
1const express = require('express');2const VoiceResponse = require('twilio').twiml.VoiceResponse;34const app = express();56// Returns TwiML which prompts the caller to record a message7app.post('/record', (request, response) => {8// Use the Twilio Node.js SDK to build an XML response9const twiml = new VoiceResponse();10twiml.say('Hello. Please leave a message after the beep.');1112// Use <Record> to record the caller's message13twiml.record();1415// End the call with <Hangup>16twiml.hangup();1718// Render the response as XML in reply to the webhook request19response.type('text/xml');20response.send(twiml.toString());21});2223// Create an HTTP server and listen for requests on port 300024app.listen(3000);
TwiML is a set of XML tags that tell Twilio how to handle an incoming call (or SMS). In this example we tell Twilio to read some instructions to the caller and then record whatever the caller says next.
You can listen to your recordings in your Twilio Console or access them directly through Twilio's REST API.
You can also tell Twilio to transcribe a recording, giving you a text representation of what the caller said.
1const express = require('express');2const VoiceResponse = require('twilio').twiml.VoiceResponse;34const app = express();56// Returns TwiML which prompts the caller to record a message7app.post('/record', (request, response) => {8// Use the Twilio Node.js SDK to build an XML response9const twiml = new VoiceResponse();10twiml.say('Hello. Please leave a message after the beep.');1112// Use <Record> to record and transcribe the caller's message13twiml.record({ transcribe: true, maxLength: 30 });1415// End the call with <Hangup>16twiml.hangup();1718// Render the response as XML in reply to the webhook request19response.type('text/xml');20response.send(twiml.toString());21});2223// Create an HTTP server and listen for requests on port 300024app.listen(3000);
Here we add "transcribe: true" to our response to tell Twilio to transcribe the recording after it's complete. We also pass a "maxLength" argument to limit the length of the recording (it defaults to an hour).
Check out the <Record> reference docs to see all the parameters you can use to customize your recordings.
When you make outgoing calls with the Twilio REST API, you can tell Twilio to record the entire call from beginning to end.
First, you'll need to get your Twilio account credentials. They can be found on the home page of the console.
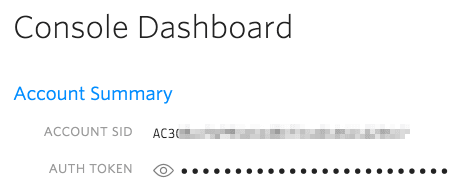
Just pass an extra "record" argument to "client.calls.create()" and Twilio will record the entire phone call.
1// Download the helper library from https://www.twilio.com/docs/node/install2const twilio = require("twilio"); // Or, for ESM: import twilio from "twilio";34// Find your Account SID and Auth Token at twilio.com/console5// and set the environment variables. See http://twil.io/secure6const accountSid = process.env.TWILIO_ACCOUNT_SID;7const authToken = process.env.TWILIO_AUTH_TOKEN;8const client = twilio(accountSid, authToken);910async function createCall() {11const call = await client.calls.create({12from: "+15017122661",13record: true,14to: "+14155551212",15url: "http://demo.twilio.com/docs/voice.xml",16});1718console.log(call.sid);19}2021createCall();
Response
Note: This shows the raw API response from Twilio. Responses from SDKs (Java, Python, etc.) may look a little different.1{2"account_sid": "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",3"answered_by": null,4"api_version": "2010-04-01",5"caller_name": null,6"date_created": "Tue, 31 Aug 2010 20:36:28 +0000",7"date_updated": "Tue, 31 Aug 2010 20:36:44 +0000",8"direction": "inbound",9"duration": "15",10"end_time": "Tue, 31 Aug 2010 20:36:44 +0000",11"forwarded_from": "+141586753093",12"from": "+15017122661",13"from_formatted": "(415) 867-5308",14"group_sid": null,15"parent_call_sid": null,16"phone_number_sid": "PNaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",17"price": "-0.03000",18"price_unit": "USD",19"sid": "CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",20"start_time": "Tue, 31 Aug 2010 20:36:29 +0000",21"status": "completed",22"subresource_uris": {23"notifications": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Notifications.json",24"recordings": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Recordings.json",25"payments": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Payments.json",26"events": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Events.json",27"siprec": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Siprec.json",28"streams": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Streams.json",29"transcriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Transcriptions.json",30"user_defined_message_subscriptions": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessageSubscriptions.json",31"user_defined_messages": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/UserDefinedMessages.json"32},33"to": "+14155551212",34"to_formatted": "(415) 867-5309",35"trunk_sid": null,36"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Calls/CAaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json",37"queue_time": "1000"38}
Once the call is complete, you can listen to your recordings in your Twilio Console or access them directly through Twilio's REST API.
You can also gain access to the recording as soon as the call is complete by including a recordingStatusCallback
with your client.calls.create
method. When your recording is ready, Twilio will send a request to the URL you specified, and that request will include a link to the recording's audio file.
You can learn more about the RecordingStatusCallback
parameter in the Call Resource API documentation.
If this guide was helpful, you might also want to check out these tutorials for Programmable Voice and Node.js. Tutorials walk through full sample applications, implementing Twilio use cases like these:
Happy hacking!