Environment Variables
Environment Variables are key/value pairs that you can add to a specific Environment. Use these for storing configuration like API keys rather than hardcoding them into your Functions. Environment Variables are encrypted, so they are the preferred way to store API keys, passwords, and any other secrets that your Function needs to use.
They also prove useful because when an Environment Variable is updated, the new value will instantly reflect in subsequent Function executions without the need for deploying new code. This allows you to adjust configurations on the fly without potentially interrupting your service due to deployments.
To view and modify the Environment Variables for a given Service, open the Service using the Twilio Console. Once the Functions Editor is open for your Service, in the Settings menu, click on Environment Variables.
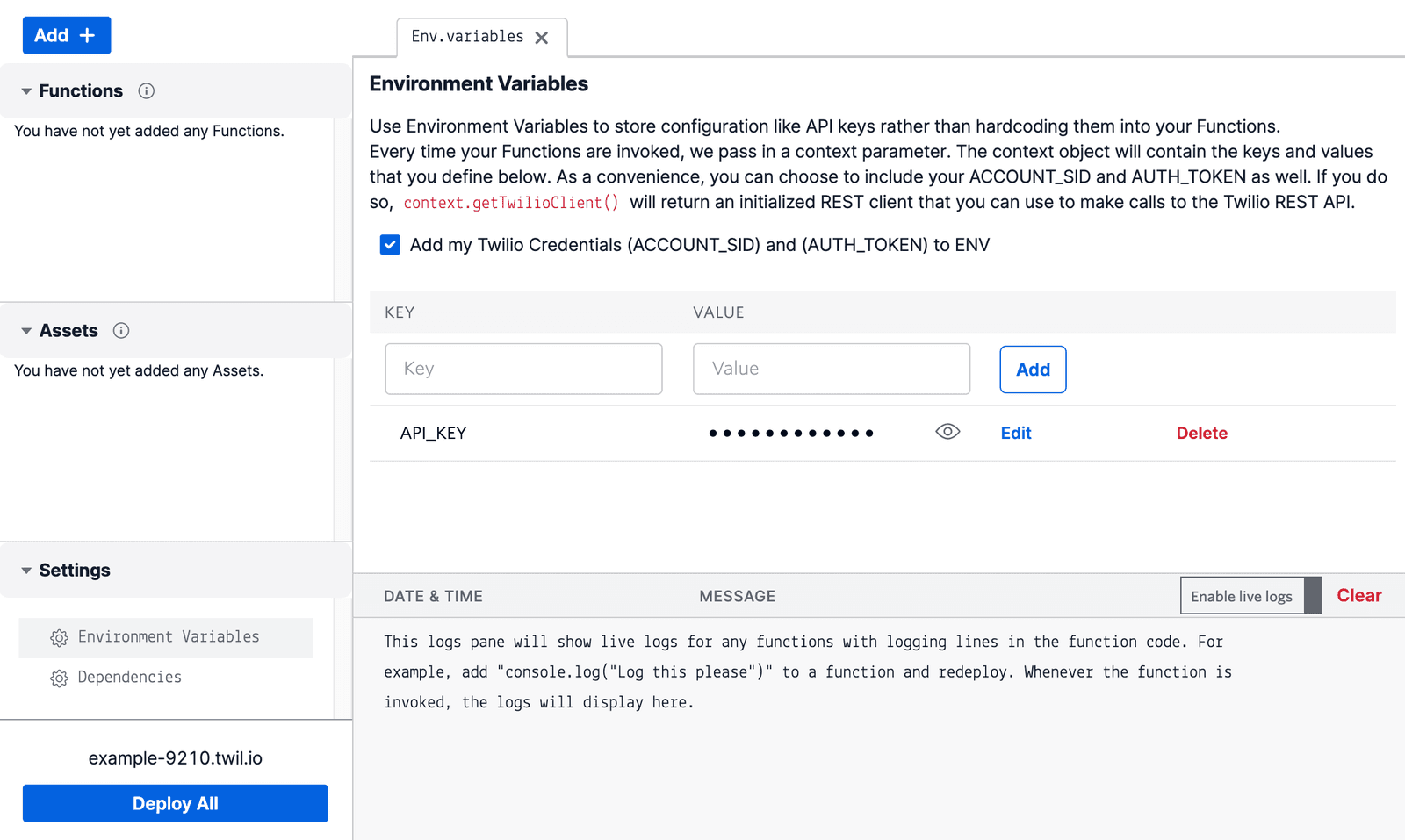
The resulting UI allows you to add, remove, and update Environment Variables.
Additionally, the Add my Twilio Credentials (ACCOUNT_SID) and (AUTH_TOKEN) to ENV checkbox allows you to choose if you would like for your ACCOUNT SID and AUTH TOKEN to be automatically added to your Function's context. This means both of these values will be accessible as Environment Variables from context
, and also that calling context.getTwilioClient()
will return an initialized Twilio REST client for making calls to Twilio's API.
Info
If you're using the Serverless Toolkit, you will instead set your Environment Variables using .env files.
If you're using multiple Environments in your application, such as dev, stage, and production, it's common to have the same Environment Variables present in each Environment, but with different values so that each version of your application is connecting to the appropriate resources. These could be various API keys with different levels of access or rate limits for the same service, credentials for different versions of your database, and more.
Using the Console UI, you can switch between which Environment Variables you are adjusting by clicking on your application URL, directly above the Deploy All button. This will render a menu showing your various Environments, and selecting one will put you in the context of that Environment.
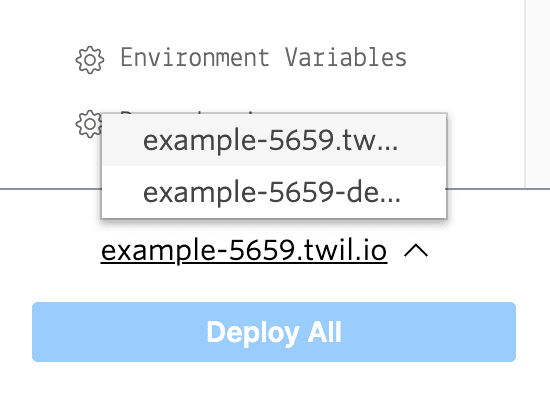
Any modifications to Environment Variables that follow will only apply to the selected Environment and not affect any others.
Info
If you're developing using the Serverless Toolkit, check out the specific documentation on how to scope environment variables.
Any Environment Variables that have been set will be accessible in your Function as properties of the context
object by name. For example, if you set an Environment Variable named API_KEY, it can be retrieved as context.API_KEY
in your Function's code.
Suppose an IVR tree you're designing requires some logic to determine if a branch of your business is open that day based on local temperatures. Using the OpenWeather Weather API and an API Key that you've set to an Environment Variable, you could securely retrieve that key from context.API_KEY
and make validated requests for weather data to complete your business logic. You can also store a common support phone number as an Environment Variable to share between your Functions.
1const axios = require("axios");2const querystring = require("querystring");34exports.handler = async (context, event, callback) => {5// Environment Variables can be accessed from the context object6const apiKey = context.API_KEY;7const supportNumber = context.SUPPORT_PHONE_NUMBER;89// Query parameters and the request body can be accessed10// from the event object11const city = event.city || "Seattle";1213// The Weather API accepts the city and apiKey as query parameters14const query = querystring.stringify({ q: city, appid: apiKey });15// Make our OpenWeather API request, and be sure to await it!16const { data } = await axios.get(17`https://api.openweathermap.org/data/2.5/weather?${query}`18);19// Do some math to convert the returned temperature from Kelvin to F20const tempInFahrenheit = (data.main.temp - 273.15) * 1.8 + 32;21// If its too hot, relay this information and the support number22if (tempInFahrenheit >= 100) {23return callback(null, {24isOpen: false,25message:26"Due to extreme temperatures and to protect the health " +27"of our employees, we're closed today. If you'd like to " +28`speak to our support team, please call ${supportNumber}`,29});30}31// Otherwise, business as usual32return callback(null, { isOpen: true, message: "We're open!" });33};
The context
object provides you with several Environment Variables by default:
Property | Type | Description |
---|---|---|
ACCOUNT_SID | string|null | If you have chosen to include your account credentials in your Function, this will return the SID identifying the Account that owns this Function. If you have not chosen to include account credentials in your Function, this value will be null . |
AUTH_TOKEN | string|null | If you have chosen to include your account credentials in your Function, this will return the Auth Token associated with the owning Account. If you have not chosen to include account credentials in your Function, this value will be null . |
DOMAIN_NAME | string | The Domain that is currently serving your Twilio Function. |
PATH | string | The path of Twilio Function that is currently being executed. |
SERVICE_SID | string | The SID of the Service which the current Function is contained in. |
ENVIRONMENT_SID | string | The SID of the Environment which the current Function is hosted in. |
Warning
For a small number of customers, SERVICE_SID
and ENVIRONMENT_SID
are not enabled due to the combined size of environment variables in use being too high and approaching the allowed limit of 3kb. In this case, these variables will return undefined
.
If you believe you are affected by this issue and wish to enable these variables, please reach out to our support team for assistance.
There are limitations on the size of individual Environment Variables depending on your method of deployment. A variable can be no longer than:
- 255 characters if set using the current V2 Console
- 150 characters if set using the legacy, Functions(Classic) Console
- 450 bytes if set using the Serverless Toolkit or the Serverless API
Additionally, there is a maximum limit of approximately 3kb on the combined size of your Environment Variables after they have been JSON encoded.
Danger
If any Environment Variable exceeds the individual limit or all Variables combined exceed the maximum limit, then your deployments will fail until your Variables have been resized.
If you must store an extremely long API key or other credential, such as an RSA key, which will cause you to exceed these limits, we suggest that you instead store the value in a private Asset and ingest it in your code using the Runtime.getAssets helper.
Given these constraints and a large RSA key that you need to store securely, you could store the text of the key in an Asset named credentials.json
, and set the Asset's Privacy to private.
1{2"myRsaKey": "xxxxxx..."3}
You could then access the RSA key (or any other stored credentials) in your Function using this code pattern.
1exports.handler = (context, event, callback) => {2// The open method returns the file's contents assuming utf8 encoding.3// Use JSON.parse to parse the file back into a JavaScript object4const credentials = JSON.parse(5Runtime.getAssets()["/credentials.json"].open()6);78// Reference the key from the credentials object9const { myRsaKey } = credentials;1011// Perform any API calls that require this key!...12};