Set up your C# and ASP.NET MVC development environment
In this guide, we'll cover how to set up your ASP.NET development environment for an MVC project. We'll also talk about a couple of helpful tools that we recommend for all C# applications that use Twilio: ngrok and the Twilio C# SDK.
Warning
This tutorial is intended for Visual Studio on Windows with .NET Framework. For a cross-platform tutorial using .NET Core, please check out our SMS developer quickstart.
For Windows, we recommend using Visual Studio Community Edition, which is a free IDE provided by Microsoft. It will install all the necessary components as well as the .NET Framework. You can also follow along with this tutorial using Visual Studio Professional or Enterprise.
First, let's create a simple ASP.NET MVC project. Visual Studio can create everything for you in a few simple clicks.
- In the Menu click File > New > Project
- This will open a dialog, in the Templates for Visual C# choose ASP.NET Web Application (.NET Framework)
- At the bottom enter the name for the project such as WebApplicationTwilio and click Ok
- In the next dialog select MVC, uncheck the box Host in Azure and click Ok
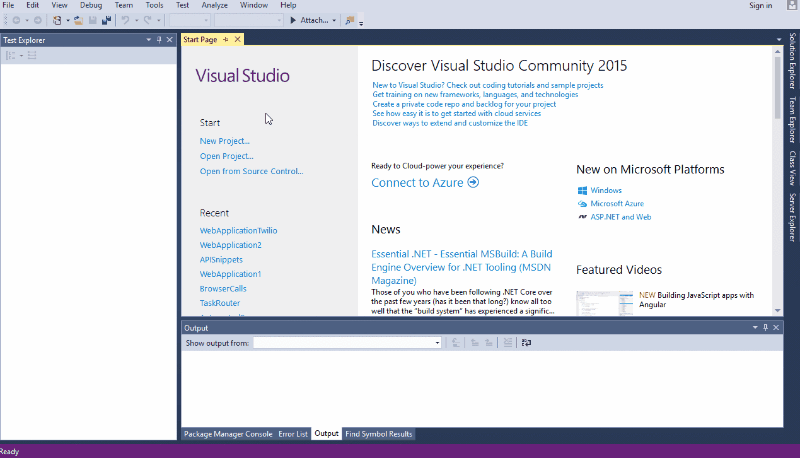
Now you should have a fully set up ASP.NET MVC project ready to go. You can click in the menu bar on Debug > Start Debugging (or press F5) it will automatically build, run the application and open your web browser to it. (Don't forget to stop the debugging before the next step).
Now we will add the Twilio C# SDK and show a simple example of how to use it with an MVC project.
- In the Solution Explorer right click on your Solution and select Manage NuGet Packages for Solution
- This will open a new tab showing you the installed packages of your Solution. Click on Browse
- Select Twilio by Twilio, on the right-hand pane check the box for your project choose the latest version and click Install
- Repeat this for the Twilio.AspNet.Mvc package by Twilio
The next step will be to modify the HomeController which handles what the application returns when you first connect to it. Right now it returns a basic HTML webpage, we will change that to return some TwiML.
Replace the content of Controllers\HomeController.cs with the following
1using System.Web.Mvc;2using Twilio.AspNet.Mvc;3using Twilio.TwiML;45namespace WebApplicationTwilio.Controllers6{7public class HomeController : TwilioController8{9public ActionResult Index()10{11var response = new VoiceResponse();12response.Say("hello world");13return TwiML(response);14}15}16}17
And that's it. You can press F5 and you should see a page displaying
1<Response>2<Say>hello world</Say>3</Response>
Now that you have a simple "Hello World!" in TwiML, your development environment is ready to go. But for most Twilio projects you'll want to install one more helpful tool: ngrok.
Most Twilio services use webhooks to communicate with your application. When Twilio receives an incoming phone call, for example, it reaches out to a URL in your application for instructions on how to handle the call.
When you're working on your application in your development environment, your app is only reachable by other programs on the same computer, so Twilio won't be able to talk to it.
ngrok is our favorite tool for solving this problem. Once started, it provides a unique URL on the ngrok.io domain which will forward incoming requests to your local development environment.
To start, head over to the ngrok download page and grab the binary for your operating system. (For more details on installing ngrok, read our ngrok guide.)
Once downloaded, run your ASP.NET MVC application and take note of the URL in the browser to find the port number your application is running on:

Once you have the port number, start ngrok using this command:
./ngrok.exe http -host-header="localhost:5678" 5678
Where 5678 is the port number. You should see output similar to this:
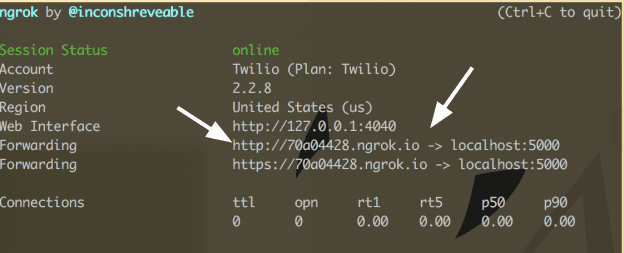
Look at the "Forwarding" line to see your unique ngrok domain name (ours is "aaf29606.ngrok.io") and then point your browser to that domain name.
If everything's working correctly, you should see your "Hello World!" message displayed at your new ngrok URL.
Any time you're working on your Twilio application and need a URL for a webhook you should use ngrok to get a publicly accessible URL like this one.
For the final step, head up towards the phone number section in Twilio's console.
- Set the "Configure with" to Voice Webhook/TwiML
- Fill in your ngrok URL
- At the bottom click on the Save button
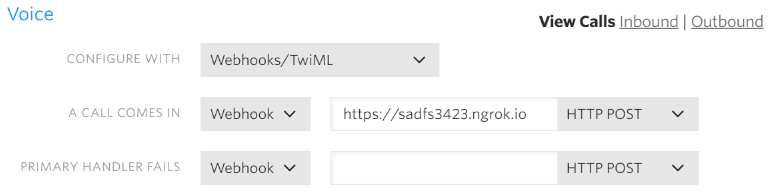
That's it, you can take your phone and call your Twilio Number, and you should hear your "Hello World!"
You're now ready to build out your ASP.NET MVC application. Learn more with the following resources: