Programmable Voice Quickstart for C# / .NET
With just a few lines of code, your .NET application can make and receive phone calls with Twilio Programmable Voice.
This quickstart teaches you how to do this using Twilio's REST API, the Twilio C# / .NET Helper Library, and the ASP.NET framework to ease development.
In this quickstart, you will learn how to:
- Buy a voice-enabled Twilio phone number
- Set up your development environment
- Make an outbound phone call that plays an MP3
- Receive and respond to an inbound phone call that reads a message to the caller using Text-to-Speech
Prefer to get started by watching a video? Check out our video on how to place and receive phone calls with C# on YouTube.
If you don't already have a Twilio Account, create one by filling out the form on the Try Twilio page.
If you already have a Voice-enabled phone number, skip to the Set up your development environment section.
Warning
If you are sending SMS to the U.S. or Canada, please be aware of updated restrictions on the use of Toll-Free numbers for messaging purposes. These restrictions do not apply to Voice, or to other non-SMS uses of Twilio phone numbers. But if you obtain a Toll-Free Number for non-SMS purposes and then wish to use it as well for Messaging, please read this first.
Follow the steps below to purchase a Twilio Phone Number with Voice capabilities.
- Log in to your Twilio Console.
- Navigate to the Buy a Number Console page: Search for "Buy a number" in the search bar at the top of your Console, or in the navigation sidebar, click on Phone Numbers > Manage > Buy a number.
- Under Capabilities, make sure that the Voice checkbox is checked.
- Under Search criteria, enter any criteria you want in your phone number and click the Search button.
- Choose a number from the list and click its associated Buy button.
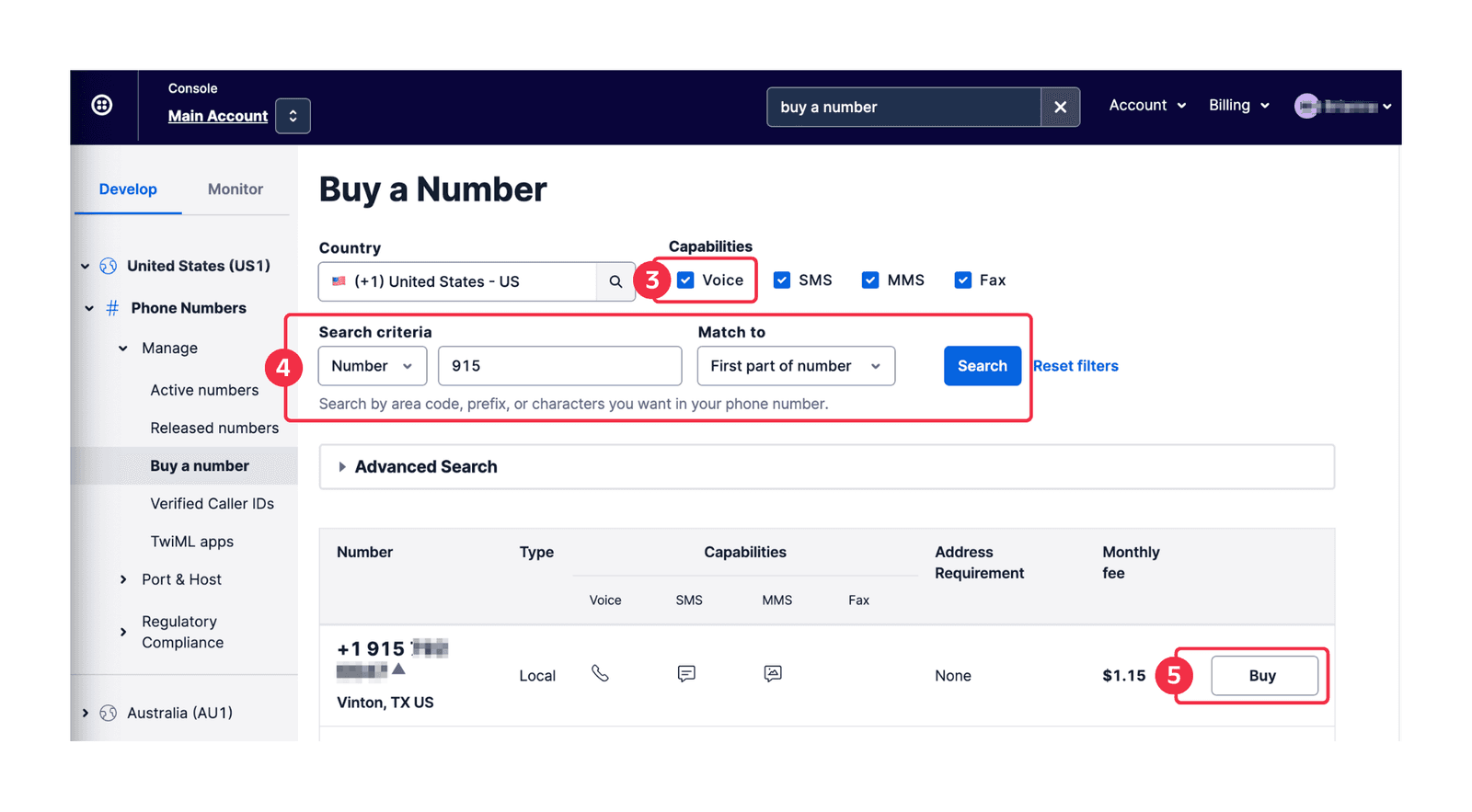
- On the Review Phone Number pop-up, click the Buy (XXX) XXX-XXXX button to confirm your purchase.
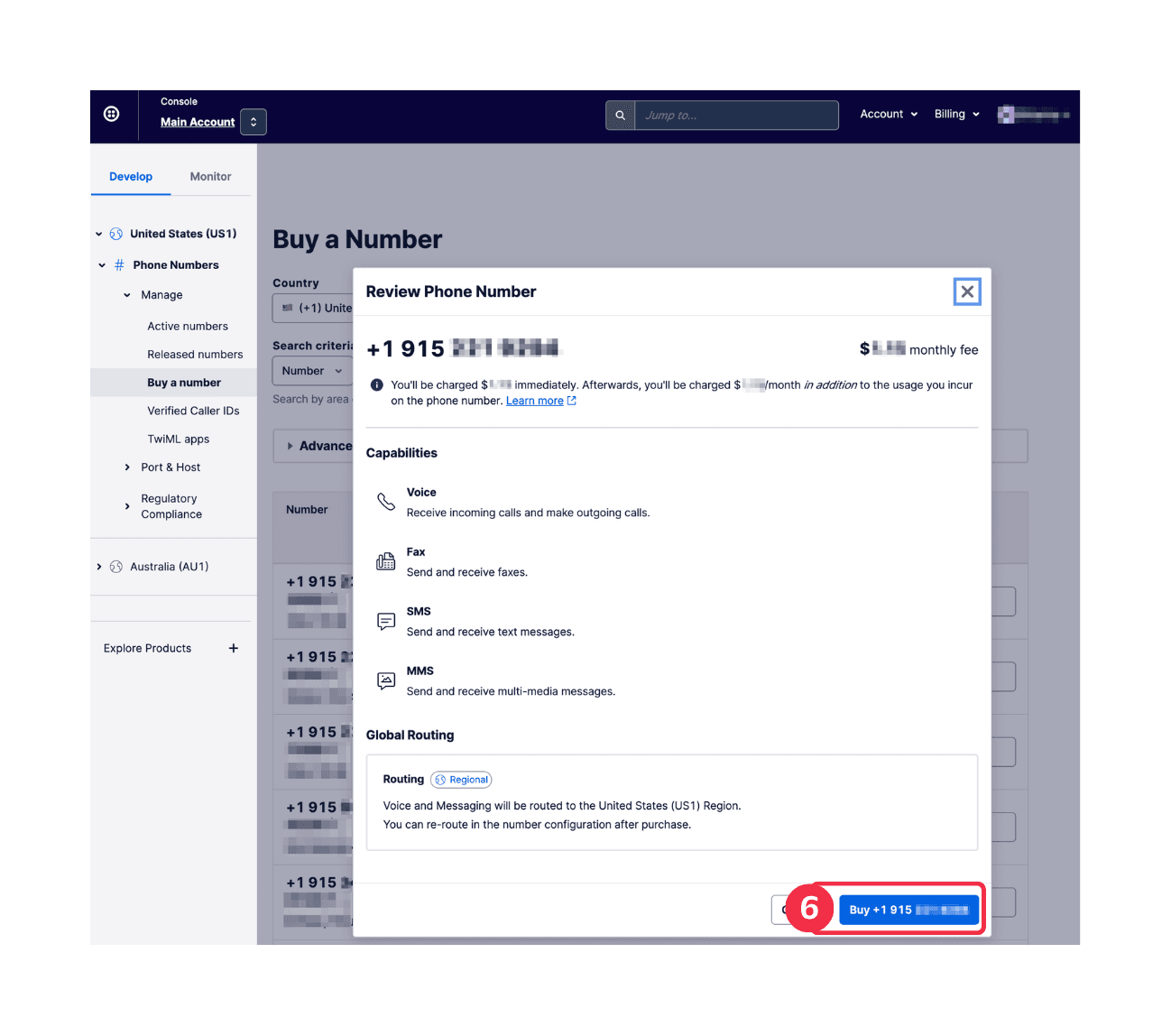
To make your first phone call, you'll need to have .NET and the Twilio .NET package installed.
If you have Visual Studio installed, you can move to step 2.2.
If you plan to use .NET Core with an editor other than Visual Studio, you can check if you already have .NET Core installed on your machine by opening up a terminal and running the following command:
dotnet --version
If you have .NET installed, you should see something like the following:
1$ dotnet --version22.1.4
If you don't have .NET Core already installed and will not be using Visual Studio, you can download .NET Core from Microsoft.
Info
The Twilio .NET Package supports the .NET Framework 3.5 and above as well as any framework that supports .NET Standard 1.4.
The easiest way to install the library is using NuGet.
If you are using Visual Studio, select the File menu and choose New > Project > Console App (either .NET Core or .NET Framework will work).
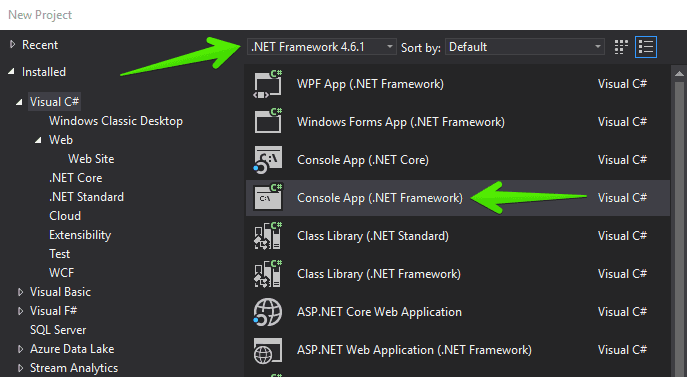
Then, from the main menu in Visual studio, select Tools > NuGet Package Manager > Package Manager Console. Type this command:
Install-Package Twilio
If you are using the dotnet
command line tool, run these commands to create a new .NET project:
1mkdir twilio-test2cd twilio-test3dotnet new console4dotnet add package Twilio
Now that you have .NET and the Twilio .NET library installed, you need to add code to your project that makes an API request to Twilio.
Open the Program.cs file in your new project and replace the existing code with the following code sample:
1// Download the twilio-csharp library from twilio.com/docs/libraries/csharp2using System;3using Twilio;4using Twilio.Rest.Api.V2010.Account;5using Twilio.Types;67namespace YourNewConsoleApp8{9class Program10{11static void Main(string[] args)12{13// To set up environmental variables, see https://twil.io/secure14const string accountSid = Environment.GetEnvironmentVariable("TWILIO_ACCOUNT_SID");15const string authToken = Environment.GetEnvironmentVariable("TWILIO_AUTH_TOKEN");16TwilioClient.Init(accountSid, authToken);1718var to = new PhoneNumber("+14155551212");19var from = new PhoneNumber("+15017122661");20var call = CallResource.Create(to, from,21url: new Uri("http://demo.twilio.com/docs/voice.xml"));2223Console.WriteLine(call.Sid);24}25}26}
This code starts a phone call between the two phone numbers that we pass as arguments. The 'from' number is our Twilio number, and the 'to' number is who we want to call.
The url
argument points to some TwiML (Twilio Markup Language), which tells Twilio what to do next when our recipient answers their phone. This TwiML tells Twilio to read a message using text to speech and then play an MP3.
Before this code will work, though, we need to edit it a little to work with your Twilio account.
Swap the placeholder values for accountSid
and authToken
with your personal Twilio credentials. Go to https://www.twilio.com/console and log in. On this page, you'll find your unique Account SID and Auth Token, which you'll need any time you send messages through the Twilio client like this. You can reveal your auth token by clicking on the eyeball icon:
Edit Program.cs
and replace the values for accountSid
and authToken
with your unique values.
Danger
It's okay to hardcode your credentials when getting started, but you should use configuration to keep them secret before deploying to production. ASP.NET applications should use the built-in configuration system for ASP.NET on the .NET Framework or ASP.NET Core. Other types of .NET applications could use environment variables.
Remember that voice-enabled phone number you bought just a few minutes ago? Go ahead and replace the existing from
number with that one, making sure to use E.164 formatting:
[+][country code][phone number including area code]
Next, replace the to phone number with your mobile phone number. This can be any phone number that can receive calls, but it's a good idea to test with your phone so that you can see the magic happen! As above, you should use E.164 formatting for this value.
Save your changes and run this code either in Visual Studio or from your terminal:
dotnet run
That's it! Your phone should ring with a call from your Twilio number, and you'll hear our short message for you. 😉
Warning
If you're using a Twilio trial account, outgoing phone calls are limited to phone numbers you have verified with Twilio. Phone numbers can be verified via your Twilio Console's Verified Caller IDs. For other trial account restrictions and limitations, check out our guide on how to work with your free Twilio trial account.
When your Twilio number receives an incoming call, Twilio will send an HTTP request to a server you control. This callback mechanism is known as awebhook. When Twilio sends your application a request, it expects a response in theTwiML XML format telling it how to respond to the message. Let's see how we would build this in C# using ASP.NET MVC for .NET Framework 4.6.1. If you prefer using ASP.NET Core,check out this article. If you need to use ASP.NET Web API,we have an article for that, as well.
In Visual Studio, select the "File" menu and choose "New" then "Project..." and select "ASP.NET Web Application (.NET Framework)."
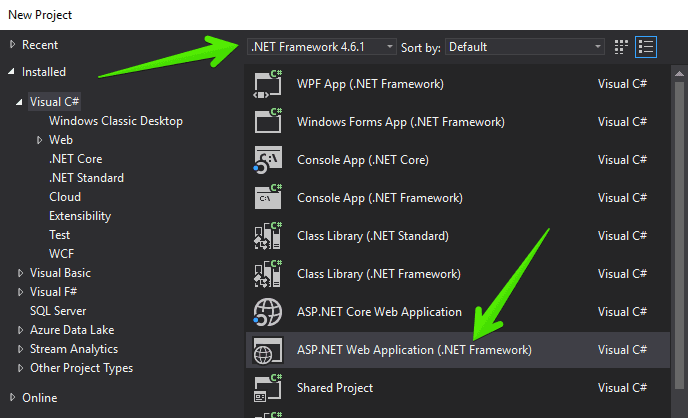
Next, choose the "MVC" template.
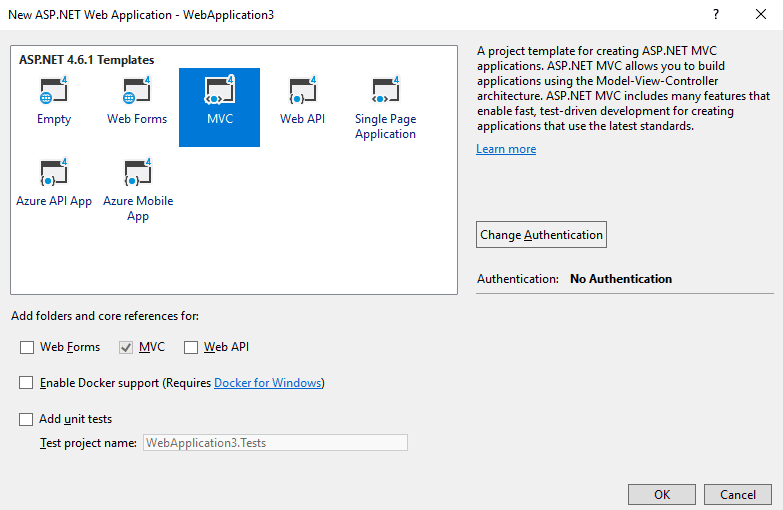
Select "Tools," "NuGet Package Manager," and "Package Manager Console" from the main menu in Visual Studio and type the following command:
Install-Package Twilio.AspNet.Mvc -DependencyVersion HighestMinor
In the directory named Controllers
, create a new Controller called VoiceController.cs
and use the following code to create a server that can handle incoming calls.
1// In Package Manager, run:2// Install-Package Twilio.AspNet.Mvc -DependencyVersion HighestMinor34using System.Web.Mvc;5using Twilio.AspNet.Mvc;6using Twilio.TwiML;78namespace YourNewWebProject.Controllers9{10public class VoiceController : TwilioController11{12[HttpPost]13public ActionResult Index()14{15var response = new VoiceResponse();16response.Say("Thank you for calling! Have a great day.");1718return TwiML(response);19}20}21}
Run the project from Visual Studio. You should see the ASP.NET MVC home page running on localhost
and a random port number.
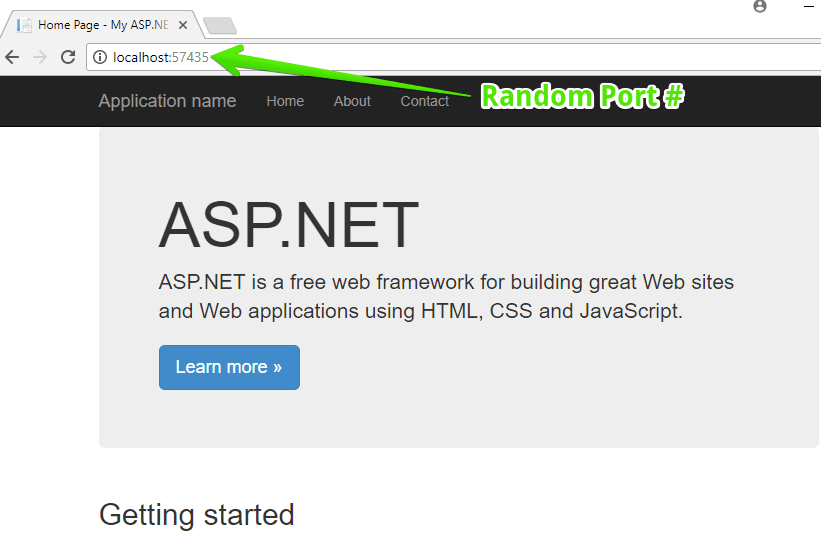
You'll need to make your application accessible over the internet. We'll show you how to set that up next so your app can receive calls.
We've just built a small ASP.NET MVC application to accept incoming phone calls. Before it will work, we need to make sure that Twilio can reach our application.
Most Twilio services use webhooks to communicate with your application. When Twilio receives a phone call, for example, it reaches out to a URL in your application for instructions on how to handle the message.
When you're working on your ASP.NET application in your development environment, your app is only reachable by other programs on your computer, so Twilio won't be able to talk to it. We need to solve this problem by making your application accessible over the internet.
While there are a lot of ways to do this, like deploying your application to Azure or AWS, you'll probably want a less laborious way to test your Twilio application. For a lightweight way to make your app available on the internet, we recommend a tool calledngrok. Ngrok listens on the same port that your local web server is running on and provides a unique URL on the ngrok.io domain, forwarding incoming requests to your local development environment. It works something like this:
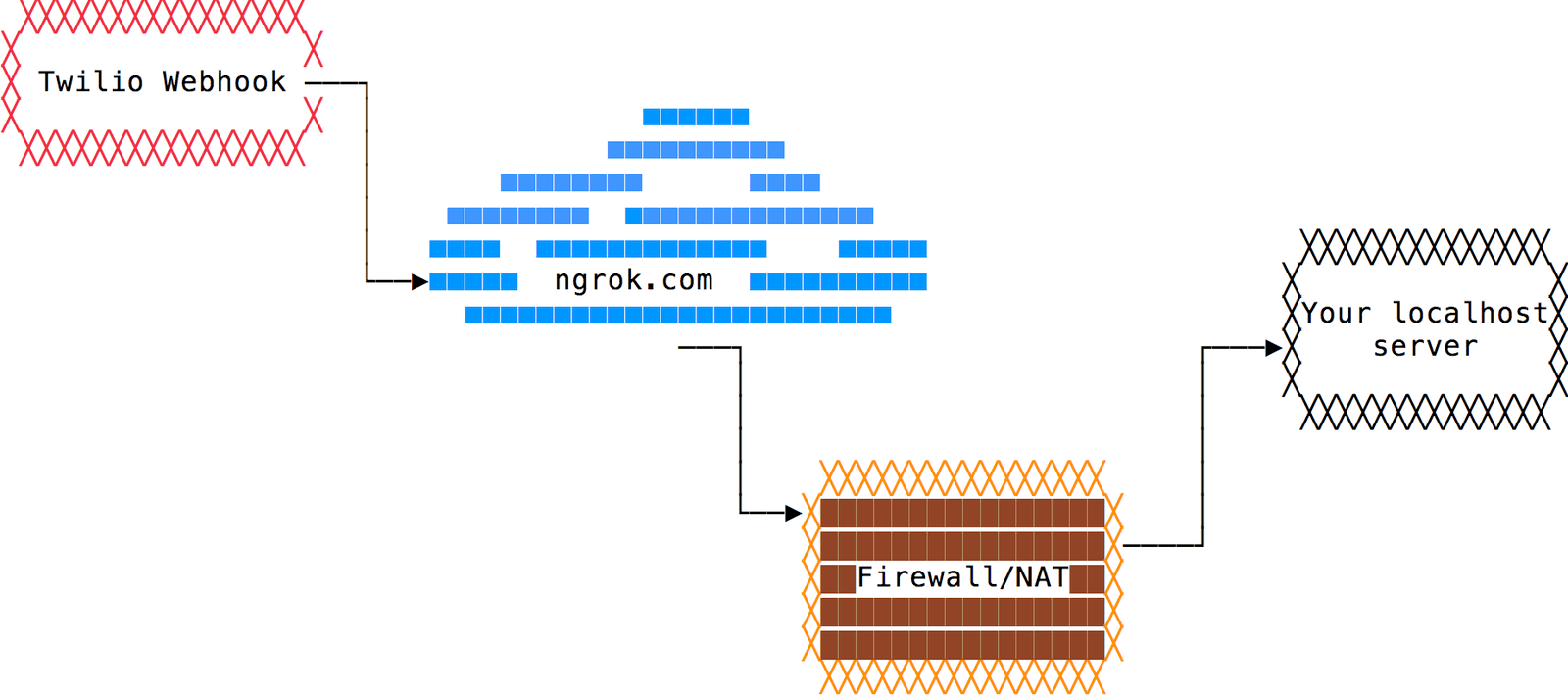
If you haven't done so already, install ngrok Extensions for Visual Studio. For more info on ngrok, including some great tips and tricks, check out this in-depth blog post.
After installing the Visual Studio extension, you will need to restart Visual Studio and reopen your project. Start your project again to bring up the home page. Then, while this is running, select "Start ngrok Tunnel" from the "Tools" menu.
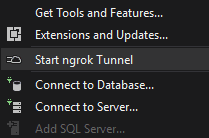
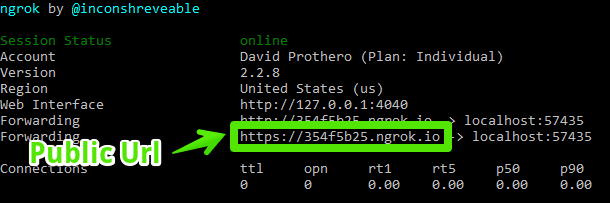
Now we have a new external URL.
For Twilio to know where to look, you need to configure your Twilio phone number to call your webhook URL whenever a call comes in.
- Log into twilio.com and go to the Numbers page in the Console.
- Click on your voice-enabled phone number.
- Find the Voice & Fax section. The default "CONFIGURE WITH" is what you'll need: "Webhooks, TwiML Bins, [etc.]"
- In the "A CALL COMES IN" section, select "Webhook" and paste in your URL: in this quickstart step above, it would be:
https://354f5b25.ngrok.io/voice
- be sure to add/voice
at the end, as this is the route to yourVoiceController
class.
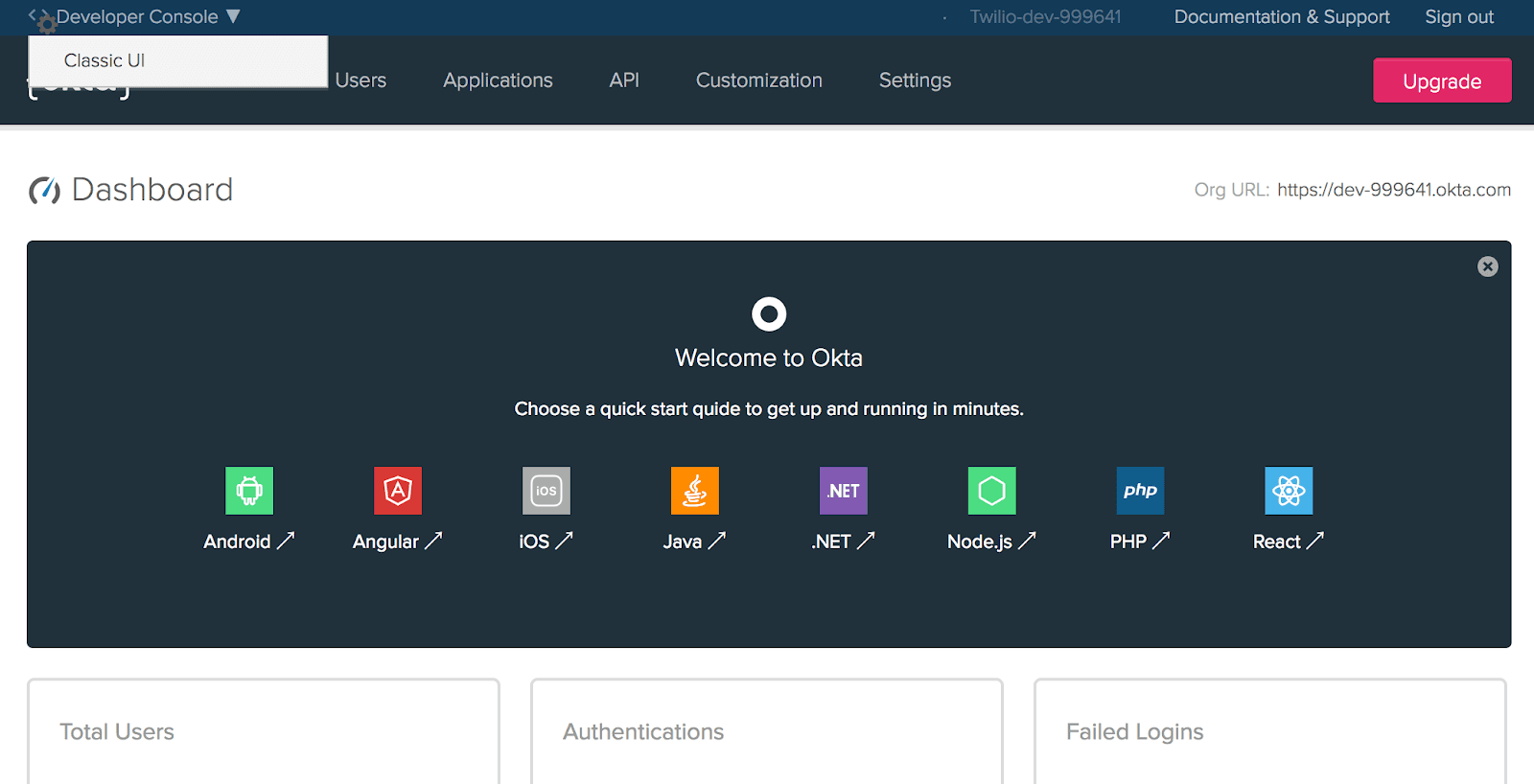
Save your changes - you're ready!
Make sure you are running your project in Visual Studio, and your ngrok tunnel is running. If you restarted ngrok, you will have to update your webhook in the console to use the right URL.
With both of those servers running, we're ready for the fun part - testing our new ASP.NET voice application!
Make a phone call from your mobile phone to your Twilio phone number. You should see an HTTP request in your ngrok console. Your ASP.NET app will process the incoming request and respond with your TwiML. Then you'll hear your message once the call connects.
Now you know the basics of making and responding to phone calls with C#.
Our ASP.NET app here only used the <Say> TwiML verb to read a message to the caller using text to speech, but you can do much more with different TwiML verbs like <Record>, <Gather>, and <Conference>.
Check out these pages to learn more:
- Gather user input via keypad (DTMF tones) in C#
- Learn how to record incoming and outgoing Twilio Voice phone calls using C#
- Create conference calls in C#
- Dive into the API Reference documentation for Twilio Programmable Voice
- Learn how to modify calls in progress with C#
- Looking to make a call from your browser or mobile application? Use a Twilio Voice SDK to integrate high-quality VoIP calling.
- Retrieve information about in-progress and completed calls from your Twilio account using C#.
- Check out our full sample application tutorial on how to transfer calls from one support agent to another with C# and ASP.NET MVC
Let's build something amazing.