IVR: Phone Tree with Python and Flask
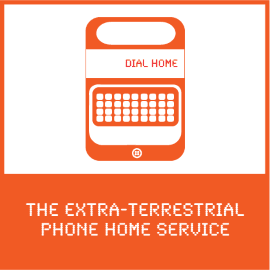
This Python Flask sample application is modeled after a typical call center experience, but with more Reese's Pieces.
Stranded aliens can call a phone number and receive instructions on how to get out of earth safely, or call their home planet directly. In this tutorial, we'll show you the critical bits of code that make this work.
Info
To run this sample app yourself, download the code and follow the instructions on GitHub. You can also look at this GitHub repository to see how we've structured our application's file structure.
Read how Livestream and others built Interactive Voice Response with Twilio. Find source code for many web frameworks on our IVR tutorial page.
To initiate the phone tree, we need to configure one of our Twilio numbers to send our web application an HTTP request when we get an incoming call.
Click on one of your numbers and configure the Voice URL to point to our app. In our code, the route will be /ivr/welcome
.
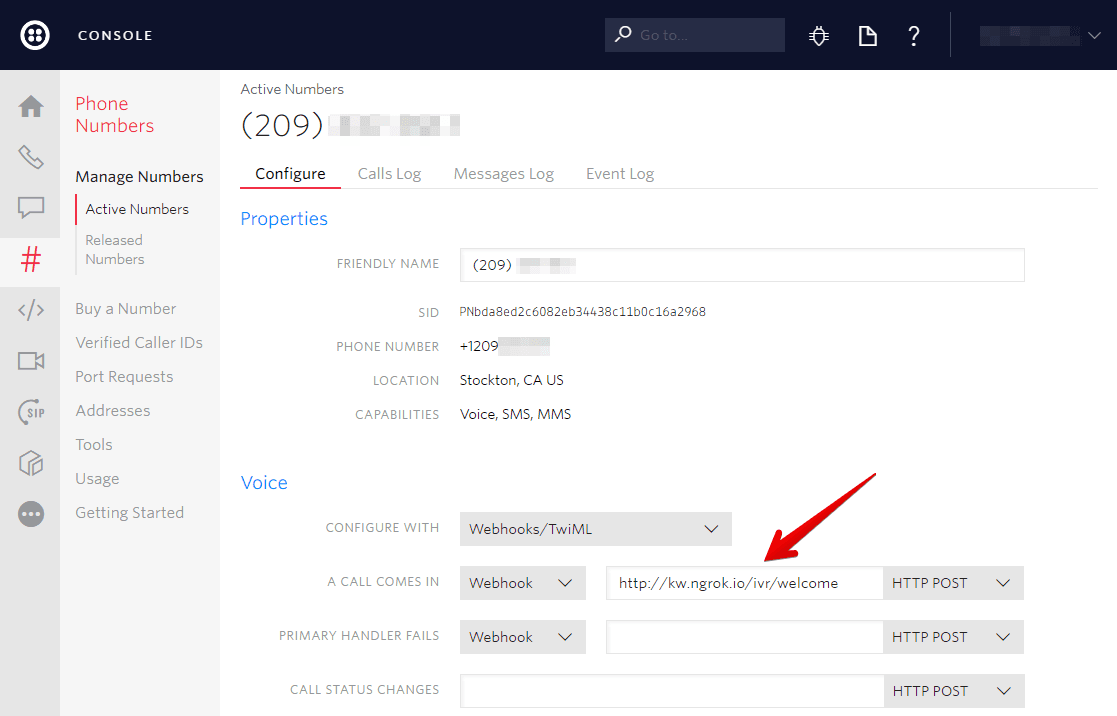
If you don't already have a server configured to use as your webhook, ngrok is an excellent tool for testing webhooks locally.
With our Twilio number configured, we are prepared to respond to the Twilio request.
Our Twilio number is now configured to send HTTP requests to this controller method on any incoming voice calls. Our app responds with TwiML to tell Twilio what to do in response to the message.
In this case, we tell Twilio to Gather
the input from the caller and then Play
a welcome message.
You may have noted we're using an unknown method, ``` TwiML` ``. This method comes from a custom view helper that takes a TwiML Response and transforms it into a valid HTTP Response. Check out the implementation:
1import flask23def twiml(resp):4resp = flask.Response(str(resp))5resp.headers['Content-Type'] = 'text/xml'6return resp
ivr_phone_tree_python/views.py
1from flask import (2flash,3render_template,4redirect,5request,6session,7url_for,8)9from twilio.twiml.voice_response import VoiceResponse1011from ivr_phone_tree_python import app12from ivr_phone_tree_python.view_helpers import twiml131415@app.route('/')16@app.route('/ivr')17def home():18return render_template('index.html')192021@app.route('/ivr/welcome', methods=['POST'])22def welcome():23response = VoiceResponse()24with response.gather(25num_digits=1, action=url_for('menu'), method="POST"26) as g:27g.say(message="Thanks for calling the E T Phone Home Service. " +28"Please press 1 for directions." +29"Press 2 for a list of planets to call.", loop=3)30return twiml(response)313233@app.route('/ivr/menu', methods=['POST'])34def menu():35selected_option = request.form['Digits']36option_actions = {'1': _give_instructions,37'2': _list_planets}3839if option_actions.has_key(selected_option):40response = VoiceResponse()41option_actions[selected_option](response)42return twiml(response)4344return _redirect_welcome()454647@app.route('/ivr/planets', methods=['POST'])48def planets():49selected_option = request.form['Digits']50option_actions = {'2': "+19295566487",51'3': "+17262043675",52"4": "+16513582243"}5354if selected_option in option_actions:55response = VoiceResponse()56response.dial(option_actions[selected_option])57return twiml(response)5859return _redirect_welcome()606162# private methods6364def _give_instructions(response):65response.say("To get to your extraction point, get on your bike and go " +66"down the street. Then Left down an alley. Avoid the police" +67" cars. Turn left into an unfinished housing development." +68"Fly over the roadblock. Go past the moon. Soon after " +69"you will see your mother ship.",70voice="Polly.Amy", language="en-GB")7172response.say("Thank you for calling the E T Phone Home Service - the " +73"adventurous alien's first choice in intergalactic travel")7475response.hangup()76return response777879def _list_planets(response):80with response.gather(81numDigits=1, action=url_for('planets'), method="POST"82) as g:83g.say("To call the planet Broh doe As O G, press 2. To call the " +84"planet DuhGo bah, press 3. To call an oober asteroid " +85"to your location, press 4. To go back to the main menu " +86" press the star key.",87voice="Polly.Amy", language="en-GB", loop=3)8889return response909192def _redirect_welcome():93response = VoiceResponse()94response.say("Returning to the main menu", voice="Polly.Amy", language="en-GB")95response.redirect(url_for('welcome'))9697return twiml(response)
To see the full layout of this app, you can check out its file structure in the GitHub repository.
After playing the audio and retrieving the caller's input, Twilio will send this input to our application.
The gather's action
parameter takes an absolute or relative URL as a value - in our case, this is the menu
endpoint.
When the caller finishes entering digits, Twilio will make a GET
or POST
request to this URL and include a Digits
parameter with the number our caller chose.
After making its request, Twilio will continue the current call using the TwiML received in your response. Note that any TwiML verbs occurring after a <Gather>
are unreachable unless the caller does not enter any digits.
ivr_phone_tree_python/views.py
1from flask import (2flash,3render_template,4redirect,5request,6session,7url_for,8)9from twilio.twiml.voice_response import VoiceResponse1011from ivr_phone_tree_python import app12from ivr_phone_tree_python.view_helpers import twiml131415@app.route('/')16@app.route('/ivr')17def home():18return render_template('index.html')192021@app.route('/ivr/welcome', methods=['POST'])22def welcome():23response = VoiceResponse()24with response.gather(25num_digits=1, action=url_for('menu'), method="POST"26) as g:27g.say(message="Thanks for calling the E T Phone Home Service. " +28"Please press 1 for directions." +29"Press 2 for a list of planets to call.", loop=3)30return twiml(response)313233@app.route('/ivr/menu', methods=['POST'])34def menu():35selected_option = request.form['Digits']36option_actions = {'1': _give_instructions,37'2': _list_planets}3839if option_actions.has_key(selected_option):40response = VoiceResponse()41option_actions[selected_option](response)42return twiml(response)4344return _redirect_welcome()454647@app.route('/ivr/planets', methods=['POST'])48def planets():49selected_option = request.form['Digits']50option_actions = {'2': "+19295566487",51'3': "+17262043675",52"4": "+16513582243"}5354if selected_option in option_actions:55response = VoiceResponse()56response.dial(option_actions[selected_option])57return twiml(response)5859return _redirect_welcome()606162# private methods6364def _give_instructions(response):65response.say("To get to your extraction point, get on your bike and go " +66"down the street. Then Left down an alley. Avoid the police" +67" cars. Turn left into an unfinished housing development." +68"Fly over the roadblock. Go past the moon. Soon after " +69"you will see your mother ship.",70voice="Polly.Amy", language="en-GB")7172response.say("Thank you for calling the E T Phone Home Service - the " +73"adventurous alien's first choice in intergalactic travel")7475response.hangup()76return response777879def _list_planets(response):80with response.gather(81numDigits=1, action=url_for('planets'), method="POST"82) as g:83g.say("To call the planet Broh doe As O G, press 2. To call the " +84"planet DuhGo bah, press 3. To call an oober asteroid " +85"to your location, press 4. To go back to the main menu " +86" press the star key.",87voice="Polly.Amy", language="en-GB", loop=3)8889return response909192def _redirect_welcome():93response = VoiceResponse()94response.say("Returning to the main menu", voice="Polly.Amy", language="en-GB")95response.redirect(url_for('welcome'))9697return twiml(response)
Now that we have told Twilio where to send the caller's input, we can look at how to process that input.
If our callers choose to call their home planet, we will read them the planet directory. Our planet directory is similar to a typical "company directory" feature of most IVRs.
In our TwiML response, we again use a Gather
verb to get our caller's input. This time, the action
verb points to the planets
route, which will map our response to what the caller chooses.
Let's look at that route next. The TwiML response we return for that route uses a Dial
verb with the appropriate phone number to connect our caller to their home planet.
ivr_phone_tree_python/views.py
1from flask import (2flash,3render_template,4redirect,5request,6session,7url_for,8)9from twilio.twiml.voice_response import VoiceResponse1011from ivr_phone_tree_python import app12from ivr_phone_tree_python.view_helpers import twiml131415@app.route('/')16@app.route('/ivr')17def home():18return render_template('index.html')192021@app.route('/ivr/welcome', methods=['POST'])22def welcome():23response = VoiceResponse()24with response.gather(25num_digits=1, action=url_for('menu'), method="POST"26) as g:27g.say(message="Thanks for calling the E T Phone Home Service. " +28"Please press 1 for directions." +29"Press 2 for a list of planets to call.", loop=3)30return twiml(response)313233@app.route('/ivr/menu', methods=['POST'])34def menu():35selected_option = request.form['Digits']36option_actions = {'1': _give_instructions,37'2': _list_planets}3839if option_actions.has_key(selected_option):40response = VoiceResponse()41option_actions[selected_option](response)42return twiml(response)4344return _redirect_welcome()454647@app.route('/ivr/planets', methods=['POST'])48def planets():49selected_option = request.form['Digits']50option_actions = {'2': "+19295566487",51'3': "+17262043675",52"4": "+16513582243"}5354if selected_option in option_actions:55response = VoiceResponse()56response.dial(option_actions[selected_option])57return twiml(response)5859return _redirect_welcome()606162# private methods6364def _give_instructions(response):65response.say("To get to your extraction point, get on your bike and go " +66"down the street. Then Left down an alley. Avoid the police" +67" cars. Turn left into an unfinished housing development." +68"Fly over the roadblock. Go past the moon. Soon after " +69"you will see your mother ship.",70voice="Polly.Amy", language="en-GB")7172response.say("Thank you for calling the E T Phone Home Service - the " +73"adventurous alien's first choice in intergalactic travel")7475response.hangup()76return response777879def _list_planets(response):80with response.gather(81numDigits=1, action=url_for('planets'), method="POST"82) as g:83g.say("To call the planet Broh doe As O G, press 2. To call the " +84"planet DuhGo bah, press 3. To call an oober asteroid " +85"to your location, press 4. To go back to the main menu " +86" press the star key.",87voice="Polly.Amy", language="en-GB", loop=3)8889return response909192def _redirect_welcome():93response = VoiceResponse()94response.say("Returning to the main menu", voice="Polly.Amy", language="en-GB")95response.redirect(url_for('welcome'))9697return twiml(response)
Again, we show some options to the caller and instruct Twilio to collect the caller's choice.
In this route, we grab the caller's digit selection from the HTTP request and store it in a variable called selected_option
. We then use a Dial
verb with the appropriate phone number to connect our caller to their home planet.
The current numbers are hardcoded, but you could update this code to read phone numbers from a database or a file.
ivr_phone_tree_python/views.py
1from flask import (2flash,3render_template,4redirect,5request,6session,7url_for,8)9from twilio.twiml.voice_response import VoiceResponse1011from ivr_phone_tree_python import app12from ivr_phone_tree_python.view_helpers import twiml131415@app.route('/')16@app.route('/ivr')17def home():18return render_template('index.html')192021@app.route('/ivr/welcome', methods=['POST'])22def welcome():23response = VoiceResponse()24with response.gather(25num_digits=1, action=url_for('menu'), method="POST"26) as g:27g.say(message="Thanks for calling the E T Phone Home Service. " +28"Please press 1 for directions." +29"Press 2 for a list of planets to call.", loop=3)30return twiml(response)313233@app.route('/ivr/menu', methods=['POST'])34def menu():35selected_option = request.form['Digits']36option_actions = {'1': _give_instructions,37'2': _list_planets}3839if option_actions.has_key(selected_option):40response = VoiceResponse()41option_actions[selected_option](response)42return twiml(response)4344return _redirect_welcome()454647@app.route('/ivr/planets', methods=['POST'])48def planets():49selected_option = request.form['Digits']50option_actions = {'2': "+19295566487",51'3': "+17262043675",52"4": "+16513582243"}5354if selected_option in option_actions:55response = VoiceResponse()56response.dial(option_actions[selected_option])57return twiml(response)5859return _redirect_welcome()606162# private methods6364def _give_instructions(response):65response.say("To get to your extraction point, get on your bike and go " +66"down the street. Then Left down an alley. Avoid the police" +67" cars. Turn left into an unfinished housing development." +68"Fly over the roadblock. Go past the moon. Soon after " +69"you will see your mother ship.",70voice="Polly.Amy", language="en-GB")7172response.say("Thank you for calling the E T Phone Home Service - the " +73"adventurous alien's first choice in intergalactic travel")7475response.hangup()76return response777879def _list_planets(response):80with response.gather(81numDigits=1, action=url_for('planets'), method="POST"82) as g:83g.say("To call the planet Broh doe As O G, press 2. To call the " +84"planet DuhGo bah, press 3. To call an oober asteroid " +85"to your location, press 4. To go back to the main menu " +86" press the star key.",87voice="Polly.Amy", language="en-GB", loop=3)8889return response909192def _redirect_welcome():93response = VoiceResponse()94response.say("Returning to the main menu", voice="Polly.Amy", language="en-GB")95response.redirect(url_for('welcome'))9697return twiml(response)
That's it! We've just implemented an IVR phone tree that will help get ET back home.
If you're a Python/Flask developer working with Twilio, you might want to check out these other tutorials:
Use Twilio to automate the process of reaching out to your customers in advance of an upcoming appointment.
Two-Factor Authentication with Authy
Use Twilio and Twilio-powered Authy OneTouch to implement two-factor authentication (2FA) in your web app.