Account and Key Management with API Keys
Twilio Editions feature
Public Key Client Validation is available to Twilio Enterprise Edition and Twilio Security Edition customers. Learn more about Editions.
To manage API Keys and Accounts via the API after enforcing Public Key Client Validation, a Main API Key is required. Once Public Key Client Validation is enforced, requests with Auth Tokens will not be successful anymore and by default, API Keys are not permitted to manage Accounts or Keys.
The required keys can be created in the Console by selecting Main
as the Key Type.
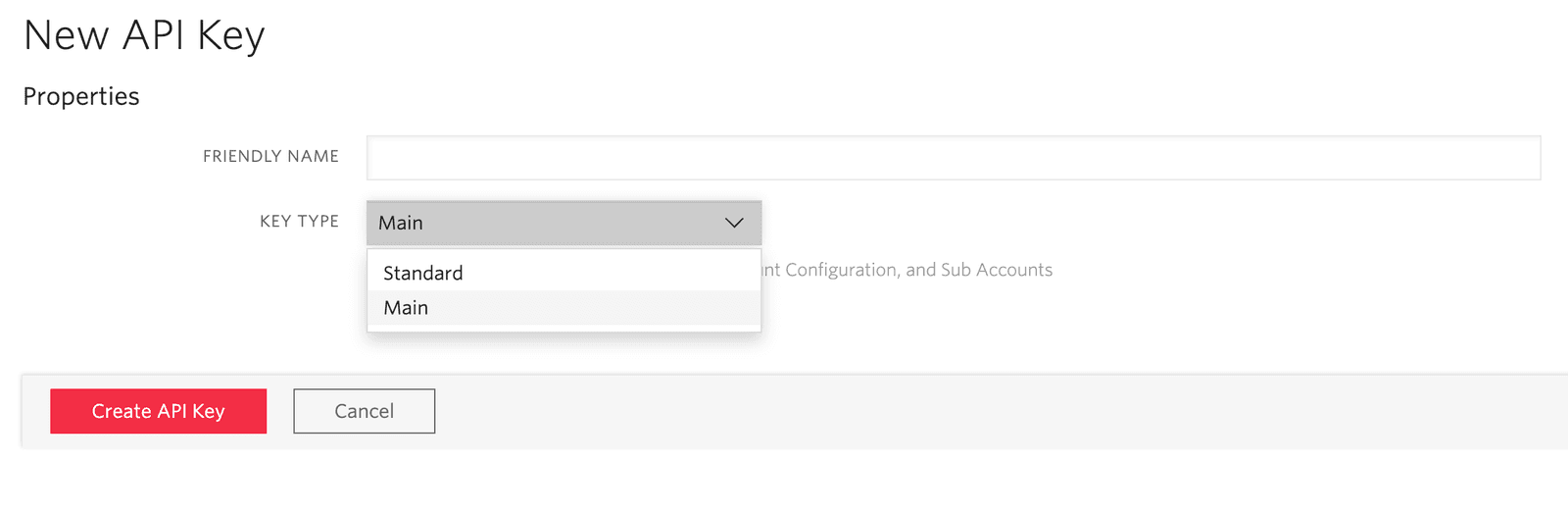
To create a new Subaccount and make a successful API request, the newly created account needs to be primed with its own API Key and Public Key. Only Main API Keys have the permissions to execute the required steps below.
- Create a new Subaccount with the key created above.
- Seed the new account with an API Key
- Seed the new account with a Public Key
- Request with new account credentials
1import com.twilio.rest.accounts.v1.credential.PublicKey;2import com.twilio.rest.api.v2010.Account;3import com.twilio.rest.api.v2010.account.NewKey;4import com.twilio.http.TwilioRestClient;5import com.twilio.http.ValidationClient;6import java.security.PrivateKey;78public class NewSubAccount {9private static final String ACCOUNT_SID = CredStore.getEnv("TWILIO_ACCOUNT_SID");10private static final String API_KEY = CredStore.getEnv("TWILIO_MAIN_KEY");11private static final String API_SECRET = CredStore.getEnv("TWILIO_MAIN_SECRET");12private static final String PUBLIC_KEY_SID = CredStore.getEnv("TWILIO_PUBLIC_KEY_SID");13private static final PrivateKey PRIVATE_KEY = CredStore.getPrivateKey();14private static final String PUBLIC_KEY = CredStore.getPublicKey();1516public static void main(String[] args) {1718//Create client with Main Account Credentials19TwilioRestClient client = new TwilioRestClient.Builder(API_KEY, API_SECRET)20.accountSid(ACCOUNT_SID)21.httpClient(new ValidationClient(ACCOUNT_SID, PUBLIC_KEY_SID, API_KEY, PRIVATE_KEY))22.build();2324//Create new Subaccount25Account myAccount = Account.creator().setFriendlyName("PKCV Account").create(client);26String myAccountSid = myAccount.getSid();2728//Seed API Key29NewKey myKey = NewKey.creator(myAccountSid).setFriendlyName("PKCV Key").create(client);3031//Seed Public Key32PublicKey myPubKey = PublicKey.creator(PUBLIC_KEY)33.setAccountSid(myAccountSid)34.setFriendlyName("Seed PK")35.create(client);3637//Create a client for new Subaccount38TwilioRestClient newClient = new TwilioRestClient.Builder(myKey.getSid(), myKey.getSecret())39.accountSid(myAccountSid)40.httpClient(new ValidationClient(myAccountSid, myPubKey.getSid(), myKey.getSid(), PRIVATE_KEY))41.build();4243//Make API call with new account and list public key sid(s) assigned to account44Iterable pks = PublicKey.reader().read(newClient);45for (PublicKey pk : pks) {46System.out.println("key: " + pk.getSid() + " - friendlyName: " + pk.getFriendlyName());47}4849//Clean up50Account.updater(myAccountSid).setStatus(Account.Status.CLOSED).update(client);51}52}
The Console also supports creating API Keys and adding Public Keys for new Subaccounts.