PHP-Laravel Quickstart for Twilio Authy Two-factor Authentication
Warning
As of November 2022, Twilio no longer provides support for Authy SMS/Voice-only customers. Customers who were also using Authy TOTP or Push prior to March 1, 2023 are still supported. The Authy API is now closed to new customers and will be fully deprecated in the future.
For new development, we encourage you to use the Verify v2 API.
Existing customers will not be impacted at this time until Authy API has reached End of Life. For more information about migration, see Migrating from Authy to Verify for SMS.
Adding two-factor authentication to your application is the easiest way to increase security and trust in your product without unnecessarily burdening your users. This quickstart guides you through building a PHP, Laravel and AngularJS application that restricts access to a URL. Four Authy two-factor authentication channels are demoed: SMS, Voice, Soft Tokens and Push Notifications.
Ready to protect your toy app's users from nefarious balaclava wearing hackers? Dive in!
Create a new Twilio account (you can sign up for a free Twilio trial), or sign into an existing Twilio account.
Once logged in, visit the Authy Console. Click on the red 'Create New Application' (or big red plus ('+') if you already created one) to create a new Authy application then name it something memorable.
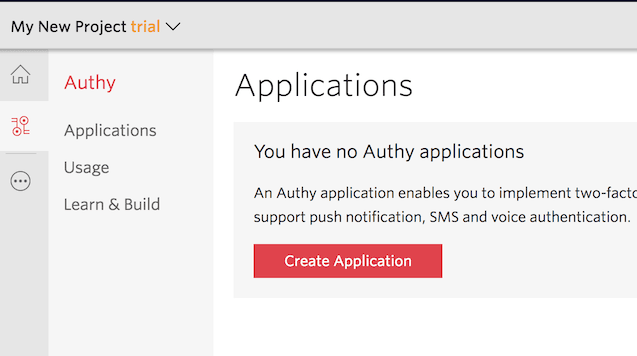
You'll automatically be transported to the Settings page next. Click the eyeball icon to reveal your Production API Key.
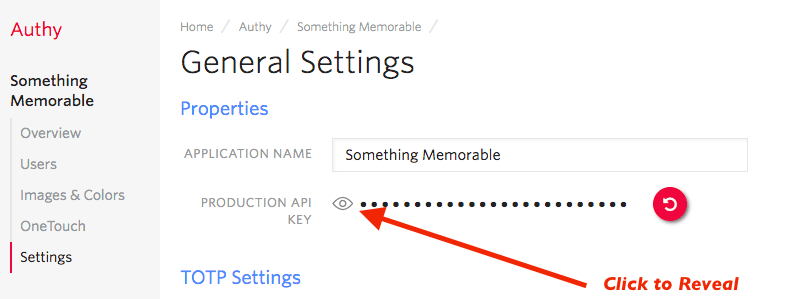
Copy your Production API Key to a safe place, you will use it during application setup.
This Authy two-factor authentication quickstart demos two channels which require an installed Authy Client to test: Soft Tokens and Push Notifications. While SMS and Voice channels will work without the client, to try out all four authentication channels download and install Authy Client for Desktop or Mobile:
To complete the quickstart today we'll use PHP 7.0+, Composer, MySQL, and the Twilio PHP Helper Library. Let's walk through installation below. Skip ahead if you have already installed one.
When doing PHP web development, we strongly suggest using Composer for package management. This quickstart relies on Composer to install the Twilio PHP Helper library. You can find manual installation instructions on the PHP Helper Library page.
You'll need a database for storing Authy IDs when a user registers in this quickstart. For this demo, we built our user database on top of MySQL 5.x.
If you haven't yet installed MySQL, here are instructions for your platform:
When installed, start it. If you're using the default MySQL credentials (as below), create schema account_security
with an admin user homestead
and password secret
.
Clone our PHP repository locally, then enter the directory.
1git clone git@github.com:TwilioDevEd/account-security-quickstart-php.git2cd account-security-quickstart-php3composer install
cp .env.example .env
Next, copy your Authy API Key from the Authy Dashboard and set the API_KEY
variable in your .env
file.
Enter the API Key from the Account Security console and optionally change the port.
1# Get your API key here: https://www.twilio.com/console/authy/getting-started2API_KEY=your-authy-api-key34APP_DEBUG=true5APP_ENV=development6APP_KEY=7APP_LOG=errorlog89# By default, if you'd like to match this install MySQL locally.10# host: localhost:330611# DB user: homestead12# DB pass: secret13# DB name: account_security1415DB_DATABASE=account_security16DB_HOST=localhost17DB_PASSWORD=secret18DB_PORT=330619DB_USERNAME=homestead20MYSQL_DATABASE=account_security21MYSQL_PASSWORD=secret22MYSQL_PORT=330623MYSQL_ROOT_PASSWORD=secret24MYSQL_USER=homestead25SECRET=your-account-secret26
Once you have added your API Key, you are ready to run! Launch the app with:
1php artisan key:generate2php artisan migrate3php artisan serve --port 8081
You should get a message your new app is running!
With your phone (optionally with the Authy client installed) nearby, open a new browser tab and go to http://localhost:8081/register
Enter your information and invent a password, then hit 'Register'. Your information is passed to Twilio (you will be able to see your user immediately in the console), and the application is returned a user_id
.
Go to http://localhost:8081/login
and login. You'll be presented with a happy screen:
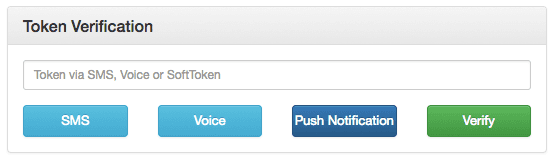
If your phone has the Authy Client installed, you can immediately enter a Soft Token from the client to Verify. Additionally, you can try a Push Notification by pushing the labeled button.
If you do not have the Authy Client installed, the SMS and Voice channels will also work in providing a token. To try different channels, you can logout to start the process again.
1<?php23namespace App\Http\Controllers\Auth;45use \Exception;6use App\Http\Controllers\Controller;7use App\Library\Services\OneTouch;8use App\User;9use Authy\AuthyApi;10use Illuminate\Http\Request;11use Illuminate\Support\Facades\Validator;1213class AccountSecurityController extends Controller14{15/*16|--------------------------------------------------------------------------17| Account Security Controller18|--------------------------------------------------------------------------19|20| Uses Authy to verify a users phone via voice, sms or notifications.21|22*/2324/**25* Create a new controller instance.26*27* @return void28*/29public function __construct()30{31$this->middleware('auth');32}3334/**35* send verification code via SMS.36*37* @param Illuminate\Support\Facades\Request $request;38* @return Illuminate\Support\Facades\Response;39*/40protected function sendVerificationCodeSMS(41Request $request,42AuthyApi $authyApi43) {44$user_id = session('user_id');45$user = User::find($user_id);46$authyID = $user['authyID'];47$authyApi->requestSms($authyID, ['force' => 'true']);4849return response()->json(['message' => 'Verification Code sent via SMS succesfully.']);50}515253/**54* send verification code via Voice Call.55*56* @param Illuminate\Support\Facades\Request $request;57* @return Illuminate\Support\Facades\Response;58*/59protected function sendVerificationCodeVoice(60Request $request,61AuthyApi $authyApi62) {63$user_id = session('user_id');64$user = User::find($user_id);65$authyID = $user['authyID'];66$authyApi->phoneCall($authyID, ['force' => 'true']);6768return response()->json(['message' => 'Verification Code sent via Voice Call succesfully.']);69}7071/**72* verify token.73*74* @param Illuminate\Support\Facades\Request $request;75* @return Illuminate\Support\Facades\Response;76*/77protected function verifyToken(78Request $request,79AuthyApi $authyApi80) {81$data = $request->all();82$token = $data['token'];83$user_id = session('user_id');84$user = User::find($user_id);85$authyID = $user['authyID'];86$authyApi->verifyToken($authyID, $token);8788return response()->json(['message' => 'Token verified successfully.']);89}9091/**92* Create One Touch Approval Request.93*94* @param Illuminate\Support\Facades\Request $request;95* @return Illuminate\Support\Facades\Response;96*/97protected function createOneTouch(98Request $request,99OneTouch $oneTouch100) {101$data = $request->all();102$user_id = session('user_id');103$user = User::find($user_id);104$authyID = $user['authyID'];105$username =$user['username'];106107$data = [108'message' => 'Twilio Account Security Quickstart wants authentication approval.',109'hidden' => 'this is a hidden value',110'visible' => [111'username' => $username,112'AuthyID' => $authyID,113'Location' => 'San Francisco, CA',114'Reason' => 'Demo by Account Security'115],116'seconds_to_expire' => 120117];118119$onetouch_uuid = $oneTouch->createApprovalRequest($data);120session(['onetouch_uuid' => $onetouch_uuid]);121122return response()->json(['message' => 'Token verified successfully.']);123}124125/**126* Get OneTouch Status.127*128* @param Illuminate\Support\Facades\Request $request;129* @return Illuminate\Support\Facades\Response;130*/131protected function checkOneTouchStatus(132Request $request,133OneTouch $oneTouch134) {135$response = $oneTouch->oneTouchStatus(session('onetouch_uuid'));136137session(['authy' => $body['approval_request']['status']]);138139return response()->json($response, 200);140}141}142
And there you go, Authy two-factor authentication is on and your PHP/Laravel app is protected!
Now that you are keeping the hackers out of this demo app using Authy two-factor authentication, you can find all of the detailed descriptions for options and API calls in our Two-factor Authentication API Reference. If you're also building a registration flow, also check out our Phone Verification product and the Verify Quickstart which uses this codebase.
For additional guides and tutorials on account security and other products, in PHP and in our other languages, take a look at the Docs.