Control Worker Activities using Worker.js: Add an Agent UI to our Project
Let's get started on our agent UI. Assuming you've followed the conventions so far in this tutorial, the UI we create will be accessible using your web browser at:
http://localhost:8080/agent.php?WorkerSid=WKXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
(substitute your Alice's WorkerSid)
We pass the WorkerSid in the URL to avoid implementing complex user management in our demo. In reality, you are likely to store a user's WorkerSid in your database alongside other User attributes.
First, create a file called agent.php
and add the following code. Remember to substitute your own account and Workspace credentials between the curly braces:
1<?php2// Get the Twilio-PHP library from twilio.com/docs/libraries/php,3// following the instructions to install it with Composer.4require_once "vendor/autoload.php";56// Your Account SID from www.twilio.com/console7// To set up environmental variables, see http://twil.io/secure8$accountSid = getenv('TWILIO_ACCOUNT_SID');9// Your Auth Token from www.twilio.com/console10$authToken = getenv('TWILIO_AUTH_TOKEN');11$workspaceSid = 'WSXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX';1213$workerSid = $_REQUEST['WorkerSid'];1415$workerCapability = new Twilio\Jwt\TaskRouter\WorkerCapability(16$accountSid, $authToken, $workspaceSid, $workerSid);17$workerCapability->allowActivityUpdates();18$workerToken = $workerCapability->generateToken();1920?>21<!DOCTYPE html>22<html>23<head>24<title>Customer Care - Voice Agent Screen</title>25<link rel="stylesheet" href="//media.twiliocdn.com/taskrouter/quickstart/agent.css"/>26<script src="//media.twiliocdn.com/taskrouter/js/v1.13/taskrouter.min.js"></script>27<script src="agent.js"></script>28</head>29<body>30<div class="content">31<section class="agent-activity offline">32<p class="activity">Offline</p>33<button class="change-activity" data-next-activity="Idle">Go Available</button>34</section>35<section class="agent-activity idle">36<p class="activity"><span>Available</span></p>37<button class="change-activity" data-next-activity="Offline">Go Offline</button>38</section>39<section class="agent-activity reserved">40<p class="activity">Reserved</p>41</section>42<section class="agent-activity busy">43<p class="activity">Busy</p>44</section>45<section class="agent-activity wrapup">46<p class="activity">Wrap-Up</p>47<button class="change-activity" data-next-activity="Idle">Go Available</button>48<button class="change-activity" data-next-activity="Offline">Go Offline</button>49</section>50<section class="log">51<textarea id="log" readonly="true"></textarea>52</section>53</div>54<script>55window.workerToken = "<?= $workerToken ?>";56</script>57</body>58</html>
You'll notice that we included three external files:
- taskrouter.min.js is the primary TaskRouter.js JavaScript file that communicates with TaskRouter's infrastructure on our behalf. You can use this URL to include TaskRouter.js in your production application, but first check the reference documentation to ensure that you include the latest version number.
- agent.css is a simple CSS file created for the purpose of this Quickstart. It saves us having to type out some simple pre-defined styles.
- agent.js contains our custom JavaScript logic for this application. We need to create that next, but first...
Download and extract Twilio's PHP helper library into the same directory that you are working in. This is required as our code references twilio-php/Services/Twilio.php
. For more advanced PHP users, the composer package manager will also work fine. See the twilio-php documentation.
Create the agent.js file in the same directory as your agent.php and add the following code:
1/* Subscribe to a subset of the available Worker.js events */23function registerTaskRouterCallbacks() {4worker.on('ready', function(worker) {5agentActivityChanged(worker.activityName);6logger("Successfully registered as: " + worker.friendlyName)7logger("Current activity is: " + worker.activityName);8});910worker.on('activity.update', function(worker) {11agentActivityChanged(worker.activityName);12logger("Worker activity changed to: " + worker.activityName);13});1415worker.on("reservation.created", function(reservation) {16logger("-----");17logger("You have been reserved to handle a call!");18logger("Call from: " + reservation.task.attributes.from);19logger("Selected language: " + reservation.task.attributes.selected_language);20logger("-----");21});2223worker.on("reservation.accepted", function(reservation) {24logger("Reservation " + reservation.sid + " accepted!");25});2627worker.on("reservation.rejected", function(reservation) {28logger("Reservation " + reservation.sid + " rejected!");29});3031worker.on("reservation.timeout", function(reservation) {32logger("Reservation " + reservation.sid + " timed out!");33});3435worker.on("reservation.canceled", function(reservation) {36logger("Reservation " + reservation.sid + " canceled!");37});38}3940/* Hook up the agent Activity buttons to Worker.js */4142function bindAgentActivityButtons() {43// Fetch the full list of available Activities from TaskRouter. Store each44// ActivitySid against the matching Friendly Name45var activitySids = {};46worker.activities.fetch(function(error, activityList) {47var activities = activityList.data;48var i = activities.length;49while (i--) {50activitySids[activities[i].friendlyName] = activities[i].sid;51}52});5354/* For each button of class 'change-activity' in our Agent UI, look up the55ActivitySid corresponding to the Friendly Name in the button's next-activity56data attribute. Use Worker.js to transition the agent to that ActivitySid57when the button is clicked.*/58var elements = document.getElementsByClassName('change-activity');59var i = elements.length;60while (i--) {61elements[i].onclick = function() {62var nextActivity = this.dataset.nextActivity;63var nextActivitySid = activitySids[nextActivity];64worker.update({"ActivitySid":nextActivitySid});65}66}67}6869/* Update the UI to reflect a change in Activity */7071function agentActivityChanged(activity) {72hideAgentActivities();73showAgentActivity(activity);74}7576function hideAgentActivities() {77var elements = document.getElementsByClassName('agent-activity');78var i = elements.length;79while (i--) {80elements[i].style.display = 'none';81}82}8384function showAgentActivity(activity) {85activity = activity.toLowerCase();86var elements = document.getElementsByClassName(('agent-activity ' + activity));87elements.item(0).style.display = 'block';88}8990/* Other stuff */9192function logger(message) {93var log = document.getElementById('log');94log.value += "\n> " + message;95log.scrollTop = log.scrollHeight;96}9798window.onload = function() {99// Initialize TaskRouter.js on page load using window.workerToken -100// a Twilio Capability token that was set in a <script> in agent.php101logger("Initializing...");102window.worker = new Twilio.TaskRouter.Worker(workerToken);103registerTaskRouterCallbacks();104bindAgentActivityButtons();105};
And that's it! Open http://localhost:8080/agent.php?WorkerSid=WK012340123401234
in your browser and you should see the screen below. If you make the same phone call as we made in Part 3, you should see Alice's Activity transition on screen as she is reserved and assigned to handle the Task.
If you see "Initializing..." and no progress, make sure that you have included the correct WorkerSid in the "WorkerSid" request parameter of the URL.
For more details, refer to the TaskRouter.js Worker JavaScript SDK documentation.
- This simple PoC has been tested in the latest version of popular browsers, including IE 11. *
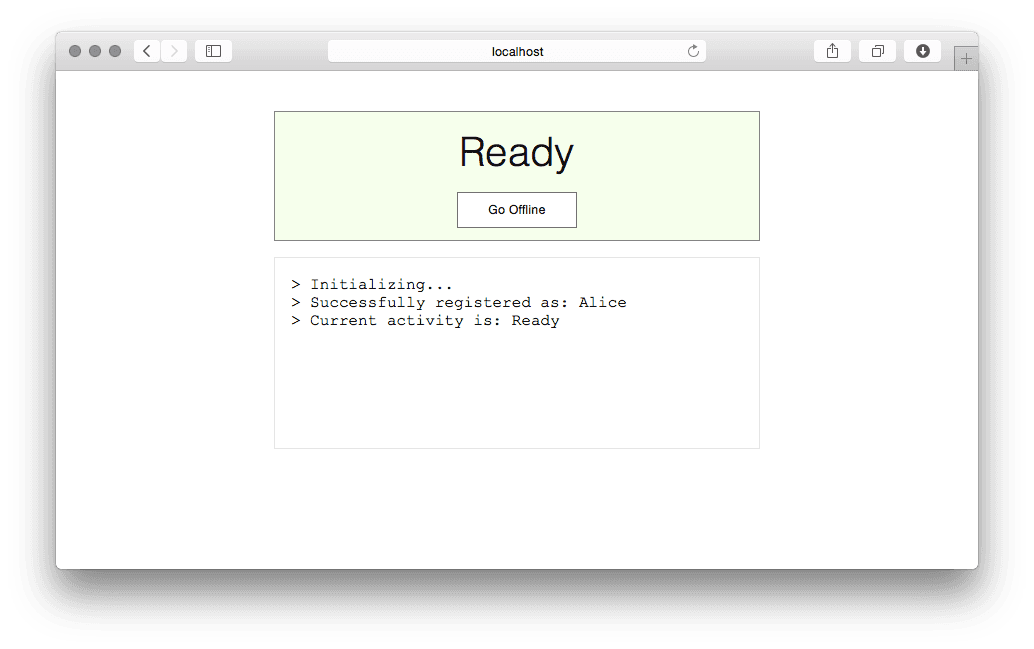