Create Tasks from Phone Calls using TwiML: Dequeue a Call to a Worker
In the previous step we created a Task from an incoming phone call using <Enqueue workflowSid="WW0123401234..">
. In this step we will create another call and dequeue it to an eligible Worker when one becomes available.
Back in Part 1 of the Quickstart we created a Worker named Alice that is capable of handling both English and Spanish inquiries. With your Workspace open in the TaskRouter web portal, click 'Workers' and click to edit the details of our Worker Alice. Ensure that Alice is set to a non-available Activity state such as 'Offline'. Next, edit Alice's JSON attributes and add a contact_uri
field. When Alice accepts a Task, she will receive a call on the phone number entered in the contact_uri
field. Replace the dummy 555 number below with your own phone number.
Alice's modified JSON attributes:
{"languages": ["en", "es"], "contact_uri": "+15555555555"}
Or, as displayed in the web portal:
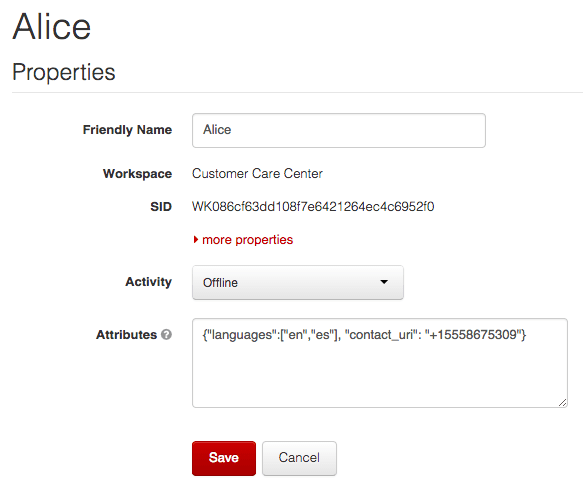
In this step, we again use <Enqueue>
to create a Task from an incoming phone call. When an eligible Worker (in this case Alice) becomes available, TaskRouter will make a request to our Assignment Callback URL. This time, we will respond with a special 'dequeue' instruction; this tells Twilio to call Alice at her 'contact_uri' and bridge to the caller.
For this part of the Quickstart, although not totally necessary it will be useful to have two phones available - one to call your Twilio number, and one to receive a call as Alice. Experienced Twilio users might consider using the Twilio Dev Phone as one of the endpoints.
Before we add the 'dequeue' assignment instruction we need to create a new Activity in our TaskRouter Workspace. One of the nice things about integrating TaskRouter with TwiML is that our Worker will automatically transition through various Activities as the call is assigned, answered and even hung up. We need an Activity for our Worker to transition to when the call ends.
With your Workspace open in the TaskRouter web portal, click 'Activities' and then 'Create Activity'. Give the new Activity a name of 'WrapUp' and a value of 'unavailable'. Once you've saved it, make a note of the Activity Sid:
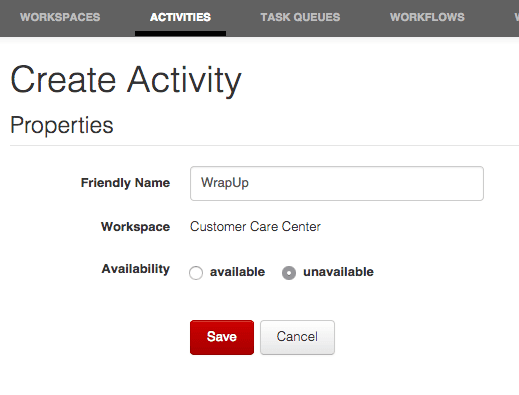
To return the 'dequeue' assignment instruction, modify run.py
assignment_callback endpoint to now issue a dequeue instruction, substituting your new WrapUp ActivitySid between the curly braces:
1# -*- coding: latin-1 -*-23from flask import Flask, request, Response4from twilio.rest import Client5from twilio.twiml.voice_response import VoiceResponse67app = Flask(__name__)89# Your Account Sid and Auth Token from twilio.com/user/account10account_sid = "{{ account_sid }}"11auth_token = "{{ auth_token }}"12workspace_sid = "{{ workspace_sid }}"13workflow_sid = "{{ workflow_sid }}"1415client = Client(account_sid, auth_token)1617@app.route("/assignment_callback", methods=['GET', 'POST'])18def assignment_callback():19"""Respond to assignment callbacks with an acceptance and 200 response"""2021ret = '{"instruction": "dequeue", "from":"+15556667777", "post_work_activity_sid":"WA0123401234..."}' # a verified phone number from your twilio account22resp = Response(response=ret, status=200, mimetype='application/json')23return resp2425@app.route("/create_task", methods=['GET', 'POST'])26def create_task():27"""Creating a Task"""28task = client.workspaces(workspace_sid) \29.tasks.create(workflow_sid=workflow_sid,30attributes='{"selected_language":"es"}')3132print(task.attributes)33resp = Response({}, status=200, mimetype='application/json')34return resp3536@app.route("/accept_reservation", methods=['GET', 'POST'])37def accept_reservation(task_sid, reservation_sid):38"""Accepting a Reservation"""39task_sid = request.args.get('task_sid')40reservation_sid = request.args.get('reservation_sid')4142reservation = client.workspaces(workspace_sid) \43.tasks(task_sid) \44.reservations(reservation_sid) \45.update(reservation_status='accepted')4647print(reservation.reservation_status)48print(reservation.worker_name)4950resp = Response({}, status=200, mimetype='application/json')51return resp5253@app.route("/incoming_call", methods=['GET', 'POST'])54def incoming_call():55"""Respond to incoming requests."""5657resp = VoiceResponse()58with resp.gather(numDigits=1, action="/enqueue_call", method="POST", timeout=5) as g:59g.say("Para Español oprime el uno.".decode("utf8"), language='es')60g.say("For English, please hold or press two.", language='en')6162return str(resp)6364@app.route("/enqueue_call", methods=['GET', 'POST'])65def enqueue_call():66digit_pressed = request.args.get('Digits')67if digit_pressed == "1":68language = "es"69else:70language = "en"7172resp = VoiceResponse()73with resp.enqueue(None, workflowSid=workflow_sid) as e:74e.task('{"selected_language":"' + language + '"}')7576return str(resp)7778if __name__ == "__main__":79app.run(debug=True)
This returns a very simple JSON object from the Assignment Callback URL:
{"instruction":"dequeue", "from": "+15556667777", "post_work_activity_sid": "WA01234012340123401234"}
The JSON instructs Twilio to dequeue the waiting call and, because we don't include an explicit "to" field in our JSON, connect it to our Worker at their contact_uri
. This is convenient default behavior provided by TaskRouter.
In the next step, we test our incoming call flow from end-to-end.