Send SMS and MMS Messages in Java
In this tutorial, we'll show you how to send SMS and MMS messages from Java using Twilio's Programmable Messaging. The code snippets in this guide target Java SDK 8 or higher, and make use of the Twilio Java Helper Library.
Warning
While you can send text-only SMS messages almost anywhere on the planet, sending media is currently only available in the US and Canada. Learn more in this support article.
Info
If you have a Twilio account and Twilio phone number with SMS (and MMS if possible) capabilities, you're all set! Feel free to jump straight to the code.
Warning
If you are sending SMS to the U.S. or Canada, be aware of updated restrictions on the use of Toll-Free numbers for messaging, including TF numbers obtained through Free Trial.
Before you can send messages, you'll need to sign up for a Twilio account and purchase a Twilio phone number.
If you're brand new to Twilio, you can sign up for a free trial account to get started. Once you've signed up, head over to your Console and grab your Account SID and your Auth Token. You will need those values for the code samples below.
Sending messages requires a Twilio phone number with SMS capabilities. If you don't currently own a Twilio phone number with SMS capabilities, you'll need to buy one. After navigating to the Buy a Number page, check the 'SMS' box and click 'Search':
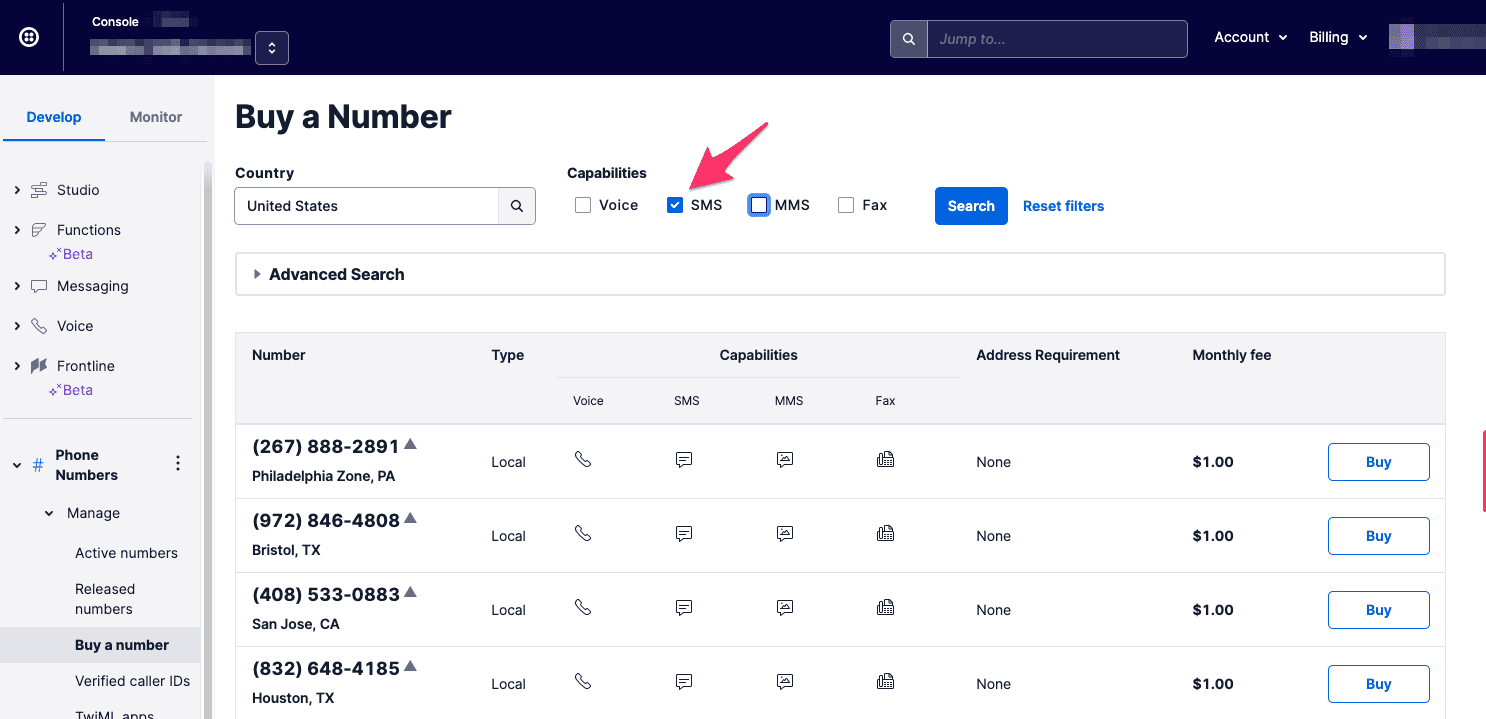
If you live in the US or Canada and also wish to send MMS messages, you can select the 'MMS' box. When viewing the search results, you can see the capability icons in the list of available numbers:
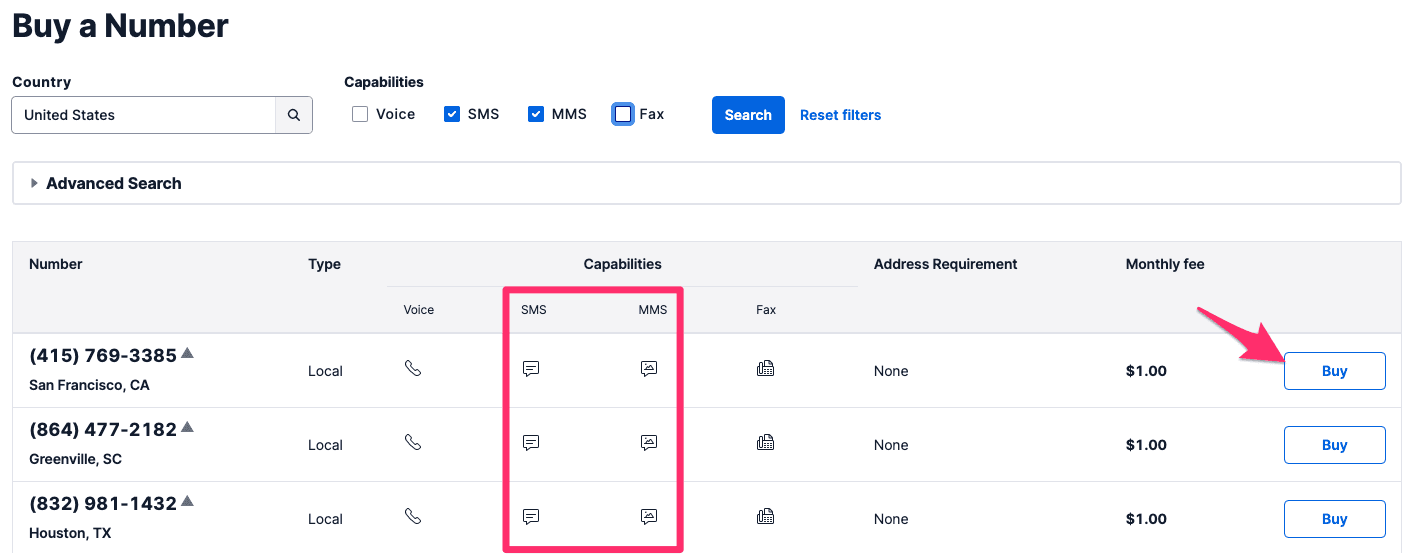
Find a number you like and click "Buy" to add it to your account.
Warning
If you're using a trial account, you will need to verify your personal phone number via the console so that you can test sending SMSes to yourself.
Learn more about how to work with your free trial account.
Sending an outgoing SMS message requires sending an HTTP POST
to the Messages resource URI. Using the helper library, you can create a new instance of the Message resource and specify the To, From, and Body parameters for your message.
The first phone number in the example below is the To parameter (your Twilio number), and the second phone number is the From parameter (your mobile number).
1// Install the Java helper library from twilio.com/docs/java/install23import com.twilio.type.PhoneNumber;4import com.twilio.Twilio;5import com.twilio.rest.api.v2010.account.Message;67public class Example {8// Find your Account SID and Auth Token at twilio.com/console9// and set the environment variables. See http://twil.io/secure10public static final String ACCOUNT_SID = System.getenv("TWILIO_ACCOUNT_SID");11public static final String AUTH_TOKEN = System.getenv("TWILIO_AUTH_TOKEN");1213public static void main(String[] args) {14Twilio.init(ACCOUNT_SID, AUTH_TOKEN);15Message message = Message16.creator(new com.twilio.type.PhoneNumber("+15558675310"),17new com.twilio.type.PhoneNumber("+15017122661"),18"This is the ship that made the Kessel Run in fourteen parsecs?")19.create();2021System.out.println(message.getBody());22}23}
Response
1{2"account_sid": "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",3"api_version": "2010-04-01",4"body": "This is the ship that made the Kessel Run in fourteen parsecs?",5"date_created": "Thu, 30 Jul 2015 20:12:31 +0000",6"date_sent": "Thu, 30 Jul 2015 20:12:33 +0000",7"date_updated": "Thu, 30 Jul 2015 20:12:33 +0000",8"direction": "outbound-api",9"error_code": null,10"error_message": null,11"from": "+15017122661",12"messaging_service_sid": "MGaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",13"num_media": "0",14"num_segments": "1",15"price": null,16"price_unit": null,17"sid": "SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",18"status": "queued",19"subresource_uris": {20"media": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Messages/SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Media.json"21},22"tags": null,23"to": "+15558675310",24"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Messages/SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json"25}
If you want to send a message to several recipients, you could create an array of recipients and iterate through each phone number in that array.
You can send as many messages as you like, as fast as you like and Twilio will queue them up for delivery at your prescribed rate limit. See our guide on how to Send Bulk SMS Messages for more tips.
You may wish to send outgoing MMS using Twilio. To send an MMS, you also make an HTTP POST
request to the Messages resource but this time specify a parameter for the URL of media, such as an image.
We'll add a line to our code from the above example that sets the MediaUrl for the message.
Warning
While you can send text-only SMS messages almost anywhere on the planet, sending media is currently only available in the US and Canada.
1// Install the Java helper library from twilio.com/docs/java/install23import com.twilio.type.PhoneNumber;4import java.net.URI;5import java.util.Arrays;6import com.twilio.Twilio;7import com.twilio.rest.api.v2010.account.Message;89public class Example {10// Find your Account SID and Auth Token at twilio.com/console11// and set the environment variables. See http://twil.io/secure12public static final String ACCOUNT_SID = System.getenv("TWILIO_ACCOUNT_SID");13public static final String AUTH_TOKEN = System.getenv("TWILIO_AUTH_TOKEN");1415public static void main(String[] args) {16Twilio.init(ACCOUNT_SID, AUTH_TOKEN);17Message message = Message18.creator(new com.twilio.type.PhoneNumber("+15558675310"),19new com.twilio.type.PhoneNumber("+15017122661"),20"This is the ship that made the Kessel Run in fourteen parsecs?")21.setMediaUrl(Arrays.asList(22URI.create("https://c1.staticflickr.com/3/2899/14341091933_1e92e62d12_b.jpg")))23.create();2425System.out.println(message.getBody());26}27}
Response
1{2"account_sid": "ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",3"api_version": "2010-04-01",4"body": "This is the ship that made the Kessel Run in fourteen parsecs?",5"date_created": "Thu, 24 Aug 2023 05:01:45 +0000",6"date_sent": "Thu, 24 Aug 2023 05:01:45 +0000",7"date_updated": "Thu, 24 Aug 2023 05:01:45 +0000",8"direction": "outbound-api",9"error_code": null,10"error_message": null,11"from": "+15017122661",12"num_media": "0",13"num_segments": "1",14"price": null,15"price_unit": null,16"messaging_service_sid": "MGaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",17"sid": "SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",18"status": "queued",19"subresource_uris": {20"media": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Messages/SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Media.json"21},22"tags": {23"campaign_name": "Spring Sale 2022",24"message_type": "cart_abandoned"25},26"to": "+15558675310",27"uri": "/2010-04-01/Accounts/ACaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa/Messages/SMaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa.json"28}
The setMediaUrl()
method call in this code tells Twilio where to go to get the media we want to include. This must be a publicly accessible URL - Twilio will not be able to reach any URLs that are hidden or that require authentication.
Just as when you send an SMS, Twilio will send data about the message in its response to your request. The JSON response will contain the unique SID and URI for your media resource:
"subresource_uris": {"media": "/2010-04 01/Accounts/ACxxxxxxxx/Messages/SMxxxxxxxxxxxxx/Media.json"}
When the Twilio REST API creates your new Message resource, it will save the image found at the specified media URL as a Media resource. Once created, you can access this resource at any time via the API.
You can print this value from your Java code to see where the image is stored. Add the following line to the end of your Example.java
file to see your newly provisioned Media URI:
System.out.println(message.getSubresourceUris().get("media"));
Want to build more messaging functionality into your Java Application?
Check out these in-depth resources to take your programmatic messaging a step further:
- Monitor the status of your message to confirm its delivery.
- Learn how to reply to an SMS sent to your Twilio phone number.
- Learn how to manage message state to turn individual messages into a true SMS conversation.
- Dig into the details with our API reference documentation for Messages.
- Sending high-volume messages? Check out our messaging services.
Also, try our SMS developer quickstart, and see how to receive and reply to messages in Java.