Programmable Messaging Quickstart - Ruby
Info
Ahoy there! All messaging transmitted using Twilio's messaging channels is treated as Application-to-Person (A2P) messaging and subject to Twilio's Messaging Policy. For detailed information on policy rules to ensure you remain compliant while using Twilio's services, see our Acceptable Use Policy.
With just a few lines of code, your Ruby application can send and receive text messages with Twilio Programmable Messaging.
This Ruby Quickstart will teach you how to do this using our Programmable Messaging REST API, the Twilio Ruby helper library, and Ruby's Sinatra framework to ease development. If you prefer using Rails, check out this blog post.
In this Quickstart, you will learn how to:
- Sign up for Twilio and get your first SMS-enabled Twilio phone number
- Set up your development environment to send and receive messages
- Send your first SMS
- Receive inbound text messages
- Reply to incoming messages with an SMS
Prefer to get started by watching a video? Check out our Ruby SMS Quickstart video on YouTube.
Info
If you already have a Twilio account and an SMS-enabled Twilio phone number, you're all set here! Feel free to jump to the next step.
Warning
If you are sending SMS to the U.S. or Canada, be aware of updated restrictions on the use of Toll-Free numbers for messaging, including TF numbers obtained through Free Trial.
You can sign up for a free Twilio trial account here.
- When you sign up, you'll be asked to verify your personal phone number. This helps Twilio verify your identity and also allows you to send test messages to your phone from your Twilio account while in trial mode.
- Once you verify your number, you'll be asked a series of questions to customize your experience.
- Once you finish the onboarding flow, you'll arrive at your project dashboard in the Twilio Console. This is where you'll be able to access your Account SID, authentication token, find a Twilio phone number, and more.
If you don't currently own a Twilio phone number with SMS functionality, you'll need to purchase one. After navigating to the Buy a Number page, check the SMS box and click Search.
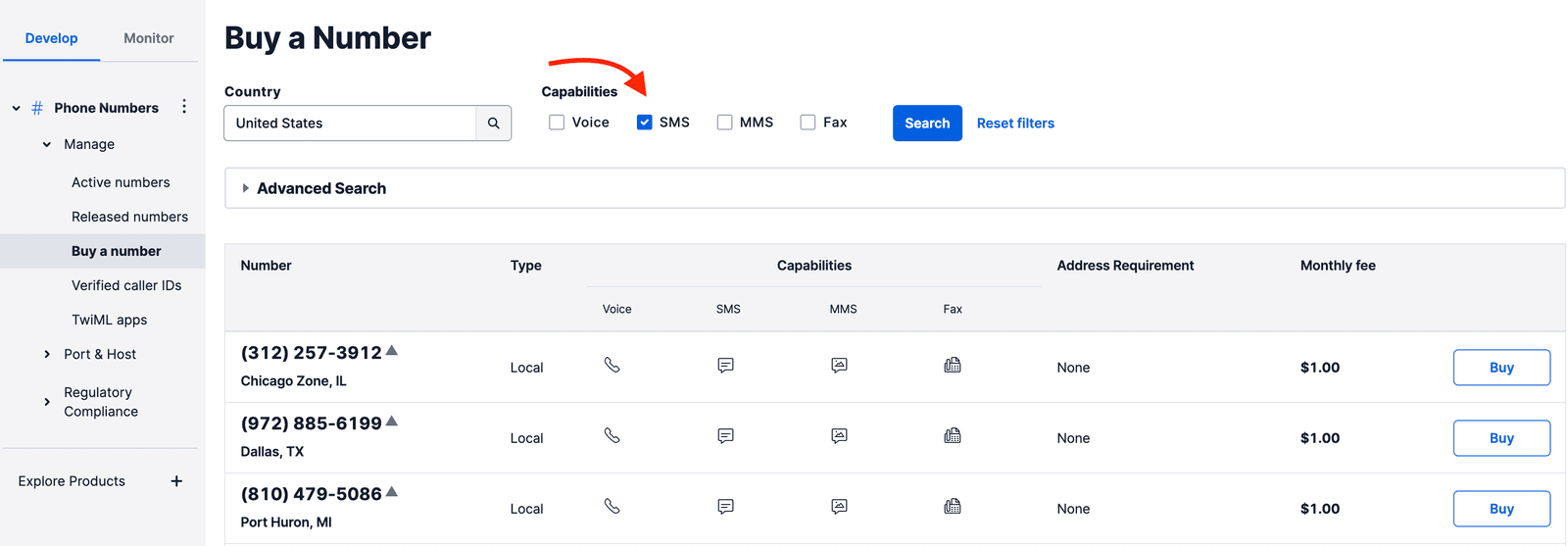
You'll then see a list of available phone numbers and their capabilities. Find a number that suits your fancy and click Buy to add it to your account.
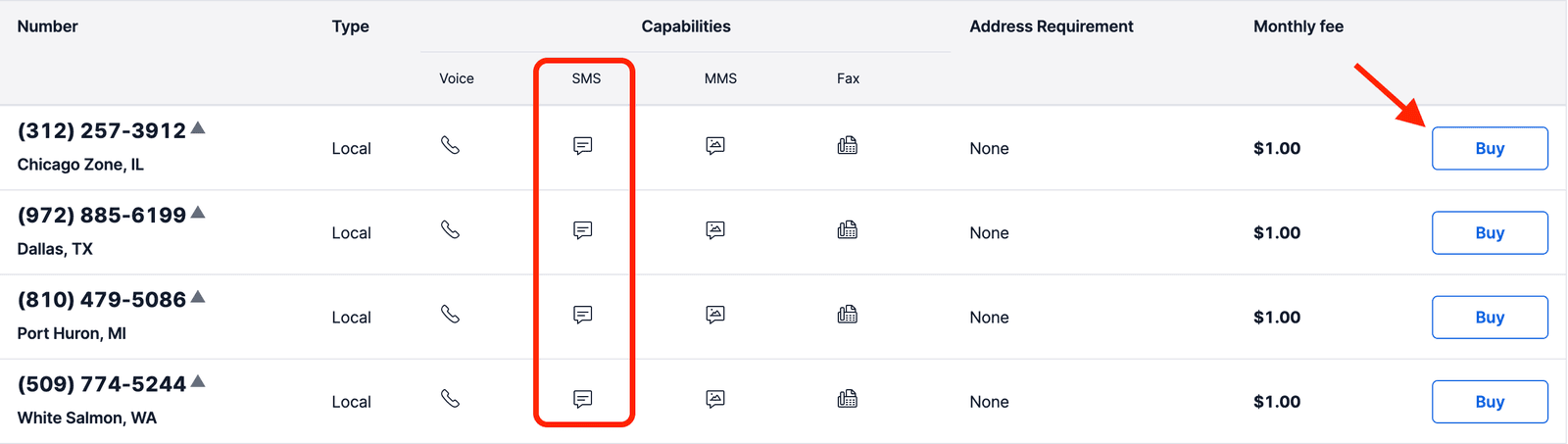
We'll need to use the Twilio CLI (command line interface) for a few tasks, so let's install that next.
The suggested way to install twilio-cli
on macOS is to use Homebrew. If you don't already have it installed, visit the Homebrew site for installation instructions and then return here.
Once you have installed Homebrew, run the following command to install twilio-cli
:
brew tap twilio/brew && brew install twilio
Info
For other installation methods, see the Twilio CLI Quickstart.
Run twilio login
to get the Twilio CLI connected to your account. Visit the Twilio Console, and under Account Info, you'll find your unique Account SID and Auth Token to provide to the CLI.
Next, we need to install Ruby and the Twilio Ruby Helper Library.
Info
If you've gone through one of our other Ruby Quickstarts already and have Ruby and the Twilio Ruby helper library installed, you can skip this step and get straight to sending your first text message.
To send your first SMS, you'll need to have Ruby and the Twilio Ruby helper library installed.
If you're using a Mac or Linux machine, you probably already have Ruby installed. You can check this by opening up a terminal and running the following command:
$ ruby --version
You should see something like:
ruby 2.7.2
Windows users can use RubyInstaller to install Ruby.
Twilio's Ruby SDK is tested against and supports Ruby versions from 2.4 through 3.0. (Got an older version of Ruby? You can use rbenv, RVM or Homebrew to upgrade to the minimum supported version.)
The easiest way to install twilio-ruby is from RubyGems.
gem install twilio-ruby
Manual Installation
Or, you can clone the source code for twilio-ruby
, and install the library from there.
"Permission Denied"
If the command line gives you a long error message that says Permission Denied in the middle of it, try running the above commands with sudo:
sudo gem install twilio-ruby
Now that we have Ruby and twilio-ruby
installed, we can send an outbound text message from the Twilio phone number we just purchased with a single API request. Create and open a new file called send_sms.rb
and type or paste in this code sample.
This code creates a new instance of the Message resource and sends an HTTP POST to the Messages resource URI.
1# Download the twilio-ruby library from twilio.com/docs/libraries/ruby2require 'twilio-ruby'34# To set up environmental variables, see http://twil.io/secure5account_sid = ENV['TWILIO_ACCOUNT_SID']6auth_token = 'yyyyyyyyyyyyyyyyyyyyyyyyy'7client = Twilio::REST::Client.new(account_sid, auth_token)89from = '+15551234567' # Your Twilio number10to = '+15555555555' # Your mobile phone number1112client.messages.create(13from: from,14to: to,15body: "Hey friend!"16)
You'll need to edit this file a little more before your message will send.
- Go to the Twilio Console and find your unique Account SID and Auth Token.
- Open
send_sms.rb
and replace the values foraccount_sid
andauth_token
with your Account SID and Auth Token.
Danger
It's okay to hardcode your credentials when getting started, but you should use environment variables to keep them secret before deploying to production. Check out how to set environment variables for more information.
Remember that SMS-enabled phone number you bought just a few minutes ago? Go ahead and replace the existing from
number with that one, making sure to use E.164 formatting:
[+][country code][phone number including area code]
Replace the to
phone number with your mobile phone number. This can be any phone number that can receive text messages, but it's a good idea to test with your own phone to see the magic happen! As above, you should use E.164 formatting for this value.Save your changes and run this script from your terminal:
ruby send_sms.rb
That's it! In a few moments, you should receive an SMS from your Twilio number on your phone.
Info
Are your customers in the U.S. or Canada? You can also send them MMS messages by adding just one line of code. Check out this guide to sending MMS to see how it's done.
Warning
If you are on a Twilio trial account, your outgoing SMS messages are limited to phone numbers that you have verified with Twilio. Phone numbers can be verified via your Twilio Console's Verified Caller IDs.
In order to receive and reply to incoming SMS messages, we'll need to create a very lightweight web application that can accept incoming requests. We'll use Sinatra for this Quickstart, but if you prefer to use Rails, you can find instructions in this blog post.
First, you need a Gemfile with the following content on it. Create a file named Gemfile
and paste the following into it:
1# Gemfile2source 'https://rubygems.org'34gem 'sinatra'5gem 'twilio-ruby'
Ruby projects use Bundler to manage dependencies, so the command to pull Sinatra and the Twilio SDK into our development environment is bundle install
.
1bundle install2…3Use `bundle show [gemname]` to see where a bundled gem is installed.
We can test that our development environment is configured correctly by creating a Sinatra application. Copy the following code and drop it in a new file called quickstart.rb
:
1require 'sinatra'23get '/' do4"Hello World!"5end
We can then try running our new Sinatra application with the command ruby quickstart.rb
. You can then open http://localhost:4567 in your browser and you should see a "Hello World!" message.
When someone sends an SMS to your Twilio phone number, Twilio makes an HTTP request to your server asking for instructions on what to do next. Once you receive the request, you can tell Twilio to reply with an SMS, kick off a phone call, store details about the SMS in your database, or trigger something else entirely - it's all up to you!
For this quickstart, we'll have our Sinatra app reply to incoming SMS messages with a thank you to the sender. Open up quickstart.rb
again and update the code to look like this code sample:
1# Download the twilio-ruby library from twilio.com/docs/libraries/ruby2require 'twilio-ruby'3require 'sinatra'45post '/sms-quickstart' do6twiml = Twilio::TwiML::MessagingResponse.new do |r|7r.message(body: 'Ahoy! Thanks so much for your message.')8end910twiml.to_s11end
Output
1<?xml version="1.0" encoding="UTF-8"?>2<Response>3<Message>4Ahoy! Thanks so much for your message.5</Message>6</Response>
Save the file and restart your app with:
1ruby quickstart.rb2
Now Twilio will be able to find your application - but first, we need to tell Twilio where to look. We will use ngrok to do this.
We'll use ngrok to set up a tunnel from the public internet to your localhost. This will let us use a public URL as the webhook for your application.
First, download and configure ngrok.
Next, run this command to have ngrok set up a tunnel to your localhost:
ngrok http 4567
This will start an ngrok tunnel. Copy down the Forwarding URL that ends with ngrok.io
.
Then, you need to configure your Twilio phone number to call your webhook URL whenever a new message comes in:
- Go to Phone Numbers > Active Numbers in the Twilio Console.
- Select the SMS-enabled Twilio number you want to use.
- For the A MESSAGE COMES IN webhook, enter the ngrok URL you copied down earlier. Append
/sms-quickstart
to the end of the URL.

Now that everything is glued together, it's time to test.
Send a text message from your mobile phone to your Twilio phone number. You'll see a couple of things happen very quickly:
- Your Sinatra dev server will note a new connection
- Twilio will forward your response back as an SMS!
Now that you know the basics of sending and receiving SMS text messages with Ruby and Sinatra, you might want to check out these resources.
- Dive into the API Reference documentation for Twilio SMS
- Learn how to create an SMS conversation in Ruby
- Track the Message Status of Outbound Messages
- Send an SMS during a phone call
Let's build something amazing.