Receive and Reply to Incoming Messages - PHP
In this guide, we'll walk through how to use Programmable Messaging to respond to incoming messages in a PHP web application.
When someone sends a text message to a Twilio number, Twilio can call a webhook you create in PHP from which you can send a reply back using TwiML. This guide will help you master those basics in no time.
Info
Twilio can send your web application an HTTP request when certain events happen, such as an incoming text message to one of your Twilio phone numbers. These requests are called webhooks, or status callbacks. For more, check out our guide to Getting Started with Twilio Webhooks. Find other webhook pages, such as a security guide and an FAQ in the Webhooks section of the docs.
The code snippets in this guide are written using PHP version 7.1, assume a local web server exists, and make use of the Twilio PHP SDK.
Let's get started!
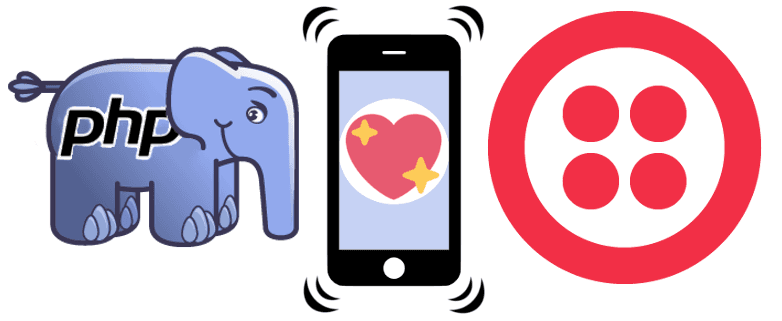
Later we will configure a Twilio phone number to send an HTTP request to a URL that we specify every time someone sends that number a message. Before we set that up, let's create the PHP code that will handle receiving and replying to those messages.
We'll create a new PHP file replyToMessage.php
to hold our code. If you haven't already installed the Twilio helper library, you'll want to do that now.
1<?php2// Get the PHP helper library from https://twilio.com/docs/libraries/php34require_once 'vendor/autoload.php'; // Loads the library5use Twilio\TwiML\MessagingResponse;67$response = new MessagingResponse();8$response->message("The Robots are coming! Head for the hills!");9print $response;
Here we're using the helper library to generate and send TwiML back to the Twilio API which will reply to incoming messages using those instructions.
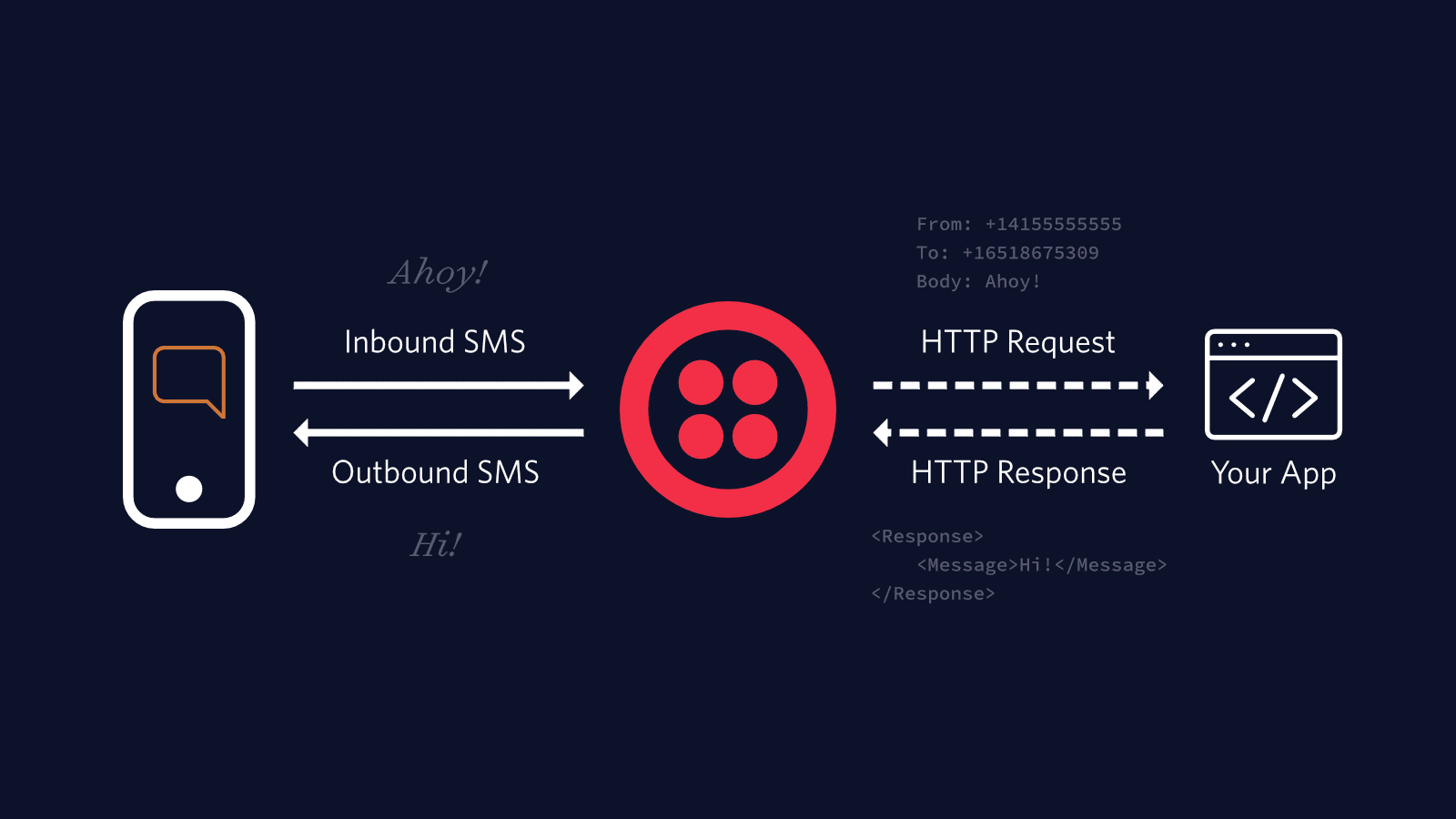
Now we need a public URL where the Twilio API can send incoming requests.
For a real project we'll want to deploy our code on a web or cloud hosting provider (of which there are many), but for learning or testing purposes, it's reasonable to use a tool such as ngrok to create a temporary public URL for our local web server.
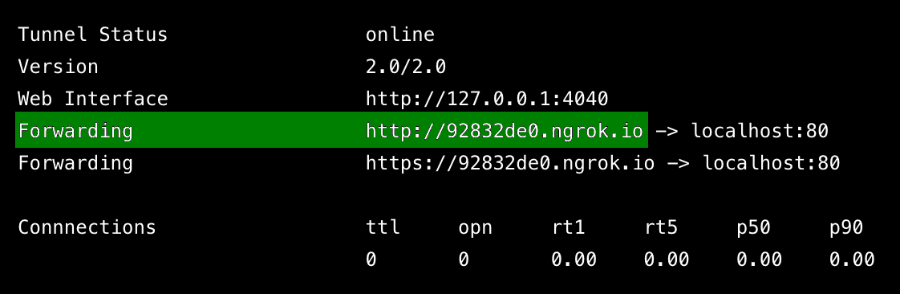
Now that we have a URL for our code, we want to add that to the configuration of a Twilio number we own:
- Log into Twilio.com and go to the Console's Numbers page
- Click on the phone number we want to use for this project
- Find the Messaging section and the "A MESSAGE COMES IN" option
- Select "Webhook" and paste in the URL we want to use, being sure to include the relevant file name:
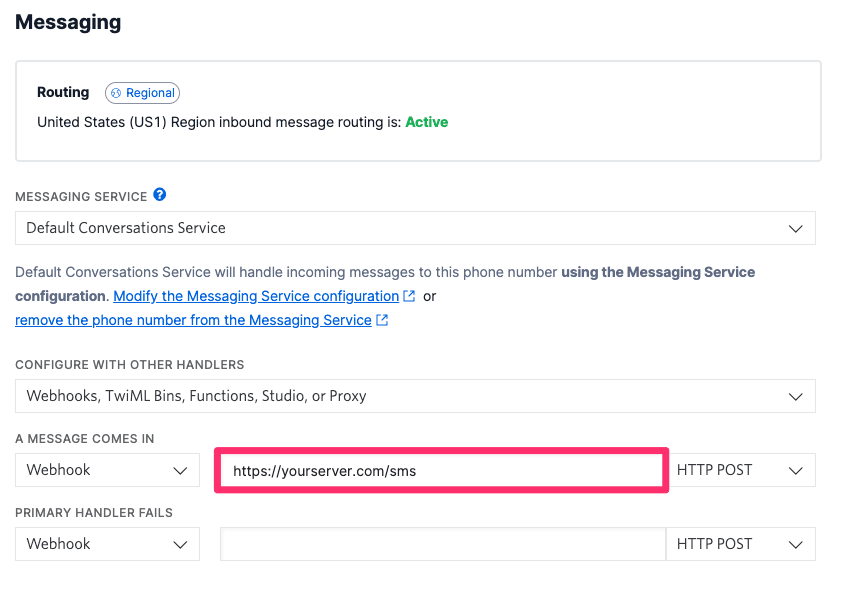
If we wanted our webhook to receive another type of request, like GET
or PUT
, we could specify that using the drop down to the right of our URL.
You'll notice in the console that there is also a spot to provide a Webhook URL for when the "PRIMARY HANDLER FAILS." Twilio will call this URL if your primary handler returns an error or does not return a response within 15 seconds. Refer to our Availability and Reliability guide for more details on the fallback URL.
Protect your webhooks
Twilio supports HTTP Basic and Digest Authentication. Authentication allows you to password protect your TwiML URLs on your web server so that only you and Twilio can access them.
Learn more about HTTP authentication and validating incoming requests here.
We can send a message with embedded media (e.g. an image) by using the media
method to add an image URL to our message. We can send multiple images by adding more media
calls with additional image URLs.
After updating the code, you may need to restart your local server. When you text the Twilio number you just set up with your webhook, you should get a reply back with the added image! Check out the API Reference for more info.
Warning
MMS messages can only be sent and received by numbers which have MMS capability. You can check the capabilities of numbers in the account portal or query the Available Phone Numbers resource to search for Twilio numbers that are MMS enabled.
1<?php2// Get the PHP helper library from https://twilio.com/docs/libraries/php34require_once 'vendor/autoload.php'; // Loads the library5use Twilio\TwiML\MessagingResponse;67$response = new MessagingResponse;8$message = $response->message("");9$message->body("The Robots are coming! Head for the hills!");10$message->media("https://farm8.staticflickr.com/7090/6941316406_80b4d6d50e_z_d.jpg");11print $response;
We've sent text and image replies, but what if we want to choose our reply based on the message we received? No problem!
The content of the incoming message will be in the Body
parameter of the incoming API request. Let's take a look at how we might use that to customize our response.
1<?php2// Get the PHP helper library from https://twilio.com/docs/libraries/php34require_once 'vendor/autoload.php'; // Loads the library5use Twilio\TwiML\MessagingResponse;67$response = new MessagingResponse;8$body = $_REQUEST['Body'];9$default = "I just wanna tell you how I'm feeling - Gotta make you understand";10$options = [11"give you up",12"let you down",13"run around and desert you",14"make you cry",15"say goodbye",16"tell a lie, and hurt you"17];1819if (strtolower($body) == 'never gonna') {20$response->message($options[array_rand($options)]);21} else {22$response->message($default);23}24print $response;
Here we've created a default message and an array of options for replying to the specific incoming message "never gonna." If any messages come in other than "never gonna" then we send out our default message. When the incoming message is "never gonna" we'll pick one of our array of options at random. Once we've updated our code, we can try sending our Twilio number an SMS that says "never gonna" or anything else and should get the corresponding response.
If you would like to receive incoming messages but not send an outgoing reply message, you can return an empty TwiML response. Twilio still expects to receive TwiML in response to its request to your server, but if the TwiML does not contain any directions, Twilio will accept the empty TwiML without taking any actions.
Need more information about the phone number that sent the message? Need to analyze the message itself for sentiment or other data? Add-ons are available in the Twilio Marketplace to accomplish these tasks and more.
To learn how to enable Add-ons for your incoming SMS messages, refer to our Add-ons tutorial. To learn how to enable Add-ons for your incoming SMS messages, see the How to Use Twilio Marketplace Add-on Listings guide.
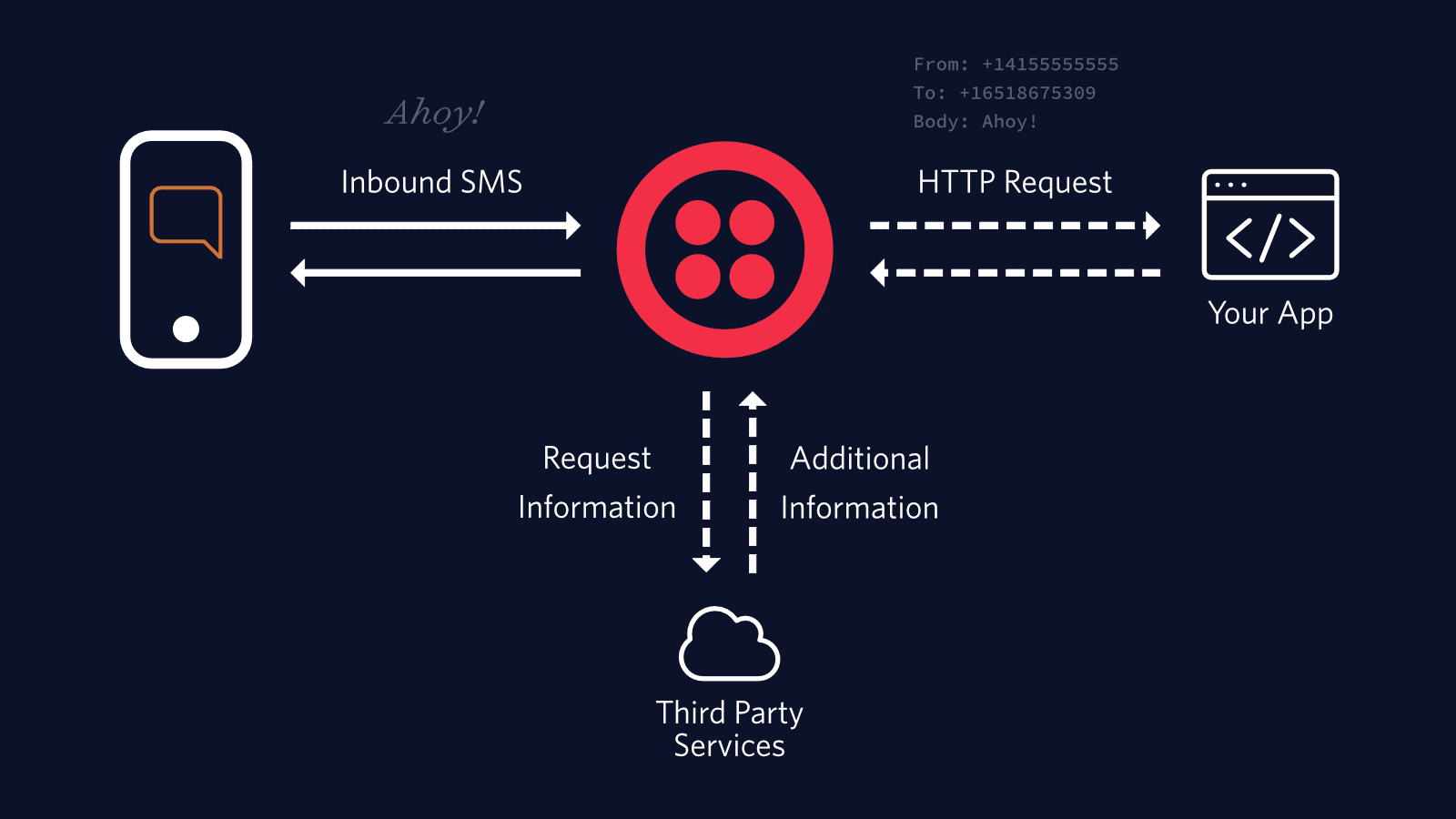
Ready to dig deeper into handling incoming messages? Check out our guide on how to Create an SMS Conversation and our Automated Survey tutorial.