Receive and Reply to Incoming Messages - Node.js
In this guide, we'll show you how to use Programmable Messaging to respond to incoming messages in your Node.js web application. When someone sends a text message to your Twilio number, Twilio can call a webhook you create in Node.js from which you can send a reply back using TwiML. All this talk of webhooks and TwiML got you feeling anxious? Fear not. This guide will help you master the basics in no time.
Info
Twilio can send your web application an HTTP request when certain events happen, such as an incoming text message to one of your Twilio phone numbers. These requests are called webhooks, or status callbacks. For more, check out our guide to Getting Started with Twilio Webhooks. Find other webhook pages, such as a security guide and an FAQ in the Webhooks section of the docs.
The code snippets in this guide are written using modern JavaScript language features in Node.js version 14 or higher, and make use of the following modules:
Let's get started!
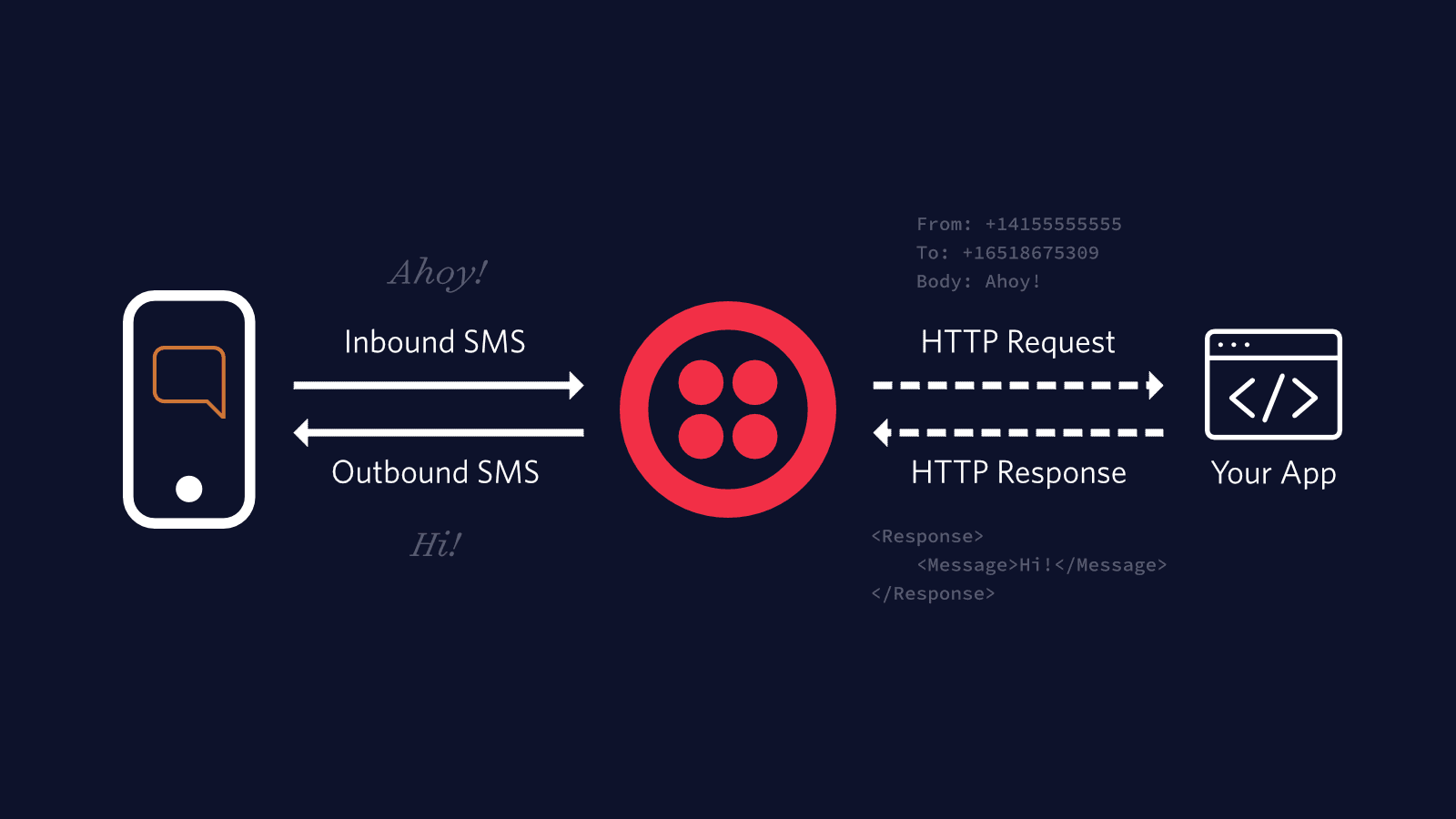
Webhooks are user-defined HTTP callbacks. They are usually triggered by some event, such as receiving an SMS message or an incoming phone call. When that event occurs, Twilio makes an HTTP request (usually a POST
or a GET
) to the URL configured for the webhook.
To handle a webhook, you only need to build a small web application that can accept the HTTP requests. Almost all server-side programming languages offer some framework for you to do this. Examples across languages include ASP.NET MVC for C#, Servlets and Spark for Java, Express for Node.js, Django and Flask for Python, and Rails and Sinatra for Ruby. PHP has its own web app framework built in, although frameworks like Laravel, Symfony and Yii are also popular.
Whichever framework and language you choose, webhooks function the same for every Twilio application. They will make an HTTP request to a URI that you provide to Twilio. Your application performs whatever logic you feel necessary - read/write from a database, integrate with another API or perform some computation - then replies to Twilio with a TwiML response with the instructions you want Twilio to perform.
TwiML is the Twilio Markup Language, which is just to say that it's an XML document with special tags defined by Twilio to help you build your SMS and voice applications. TwiML is easier shown than explained. Here's some TwiML you might use to respond to an incoming phone call:
1<?xml version="1.0" encoding="UTF-8"?>2<Response>3<Say>Thanks for calling!</Say>4</Response>
And here's some TwiML you might use to respond to an incoming SMS message:
1<?xml version="1.0" encoding="UTF-8"?>2<Response>3<Message>We got your message, thank you!</Message>4</Response>
Every TwiML document will have the root <Response> element and within that can contain one or more verbs. Verbs are actions you'd like Twilio to take, such as <Say> a greeting to a caller, or send an SMS <Message> in reply to an incoming message. For a full reference on everything you can do with TwiML, refer to our TwiML API Reference.
When someone sends a text message to your Twilio number, you can send a TwiML reply back using a configured webhook. Here's how to generate TwiML using the helper library.
1const express = require('express');2const { MessagingResponse } = require('twilio').twiml;34const app = express();56app.post('/sms', (req, res) => {7const twiml = new MessagingResponse();89twiml.message('The Robots are coming! Head for the hills!');1011res.type('text/xml').send(twiml.toString());12});1314app.listen(3000, () => {15console.log('Express server listening on port 3000');16});
When you use the helper library, you don't have to worry about generating the raw XML yourself. Of course, if you prefer to do that, then we won't stop you.
You have the code, now you need a URL you can give to Twilio. Twilio can only access public servers on the Internet. That means you need to take your web application and publish it to a web or cloud hosting provider (of which there are many), you can host it on your own server, or you can use a service such as ngrok to expose your local development machine to the internet. We generally only recommend the latter for development and testing purposes and not for production deployments.
Now that you have a URL for your web application's TwiML reply generating routine, you can configure your Twilio phone number to call your webhook URL whenever a new media message comes in for you.
- Log into Twilio.com and go to the Console's Numbers page
- Click on the phone number you'd like to modify
- Find the Messaging section and the "A MESSAGE COMES IN" option
- Select "Webhook" and paste in the URL you want to use:
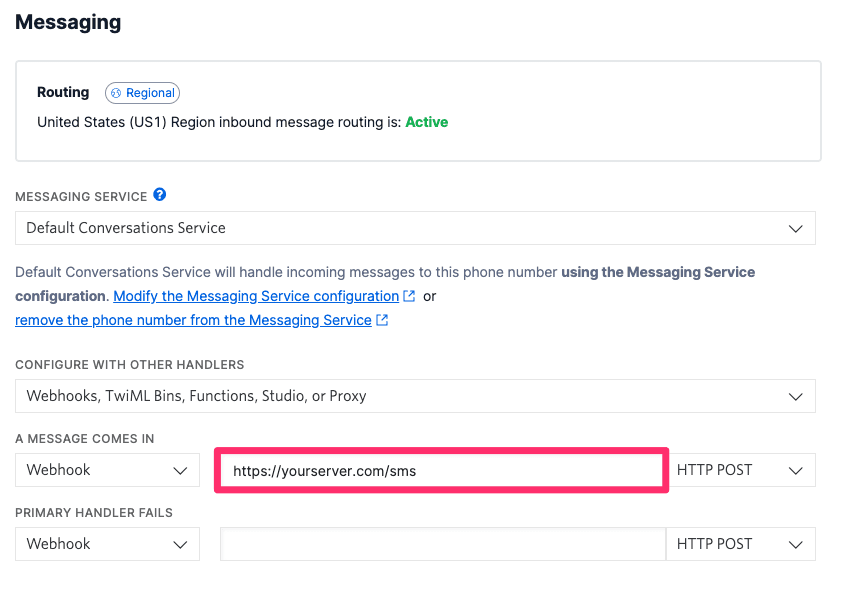
Make sure you choose HTTP POST
or HTTP GET
to correspond to what your web application is expecting. Usually the default of POST
will be fine.
You'll notice in the console that there is also a spot to provide a Webhook URL for when the "PRIMARY HANDLER FAILS." Twilio will call this URL in the event that your primary handler returns an error or does not return a response within 15 seconds. Refer to our Availability and Reliability guide for more details on the fallback URL.
Protect your webhooks
Twilio supports HTTP Basic and Digest Authentication. Authentication allows you to password protect your TwiML URLs on your web server so that only you and Twilio can access them.
Learn more about HTTP authentication here, and check out our full guide to securing your Express application by validating incoming Twilio requests.
To send a message containing media (e.g. an image), add an image URL to the text body of your message. If necessary, restart your server, then text your Twilio number again. You should receive a text message that includes an image. You can even send multiple images by adding more Media elements to your response. Check out the API Reference for more details.
Info
MMS messages can only be sent and received by numbers having MMS capability. You can check the capabilities of numbers in the account portal or query the Available Phone Numbers resource to search for Twilio numbers that are MMS enabled.
1const express = require('express');2const { MessagingResponse } = require('twilio').twiml;34const app = express();56app.post('/sms', (req, res) => {7const twiml = new MessagingResponse();89const message = twiml.message();10message.body('The Robots are coming! Head for the hills!');11message.media(12'https://farm8.staticflickr.com/7090/6941316406_80b4d6d50e_z_d.jpg'13);1415res.type('text/xml').send(twiml.toString());16});1718app.listen(3000, () => {19console.log('Express server listening on port 3000');20});
Let's take a look at how we might respond to an incoming media message with a different message depending on the incoming Body parameter from the incoming Twilio Request.
1const express = require('express');2const bodyParser = require('body-parser');3const { MessagingResponse } = require('twilio').twiml;45const app = express();67app.use(bodyParser.urlencoded({ extended: false }));89app.post('/', (req, res) => {10const twiml = new MessagingResponse();1112if (req.body.Body == 'hello') {13twiml.message('Hi!');14} else if (req.body.Body == 'bye') {15twiml.message('Goodbye');16} else {17twiml.message(18'No Body param match, Twilio sends this in the request to your server.'19);20}2122res.type('text/xml').send(twiml.toString());23});2425app.listen(3000, () => {26console.log('Express server listening on port 3000');27});
Now, try sending your Twilio number a text that says "hi" or "bye", and you should get the corresponding response.
If you would like to receive incoming messages but not send an outgoing reply message, you can return an empty TwiML response. Twilio still expects to receive TwiML in response to its request to your server, but if the TwiML does not contain any directions, Twilio will accept the empty TwiML without taking any actions.
1const express = require('express');2const { MessagingResponse } = require('twilio').twiml;34const app = express();56app.post('/sms', (req, res) => {7const twiml = new MessagingResponse();89res.type('text/xml').send(twiml.toString());10});1112app.listen(3000, () => {13console.log('Express server listening on port 3000');14});
Need more information about the phone number that sent the message? Need to analyze the message itself for sentiment or other data? Add-ons are available in the Twilio Marketplace to accomplish these tasks and more.
To learn how to enable Add-ons for your incoming SMS messages, refer to our Add-ons tutorial. To learn how to enable Add-ons for your incoming SMS messages, see the How to Use Twilio Marketplace Add-on Listings guide.
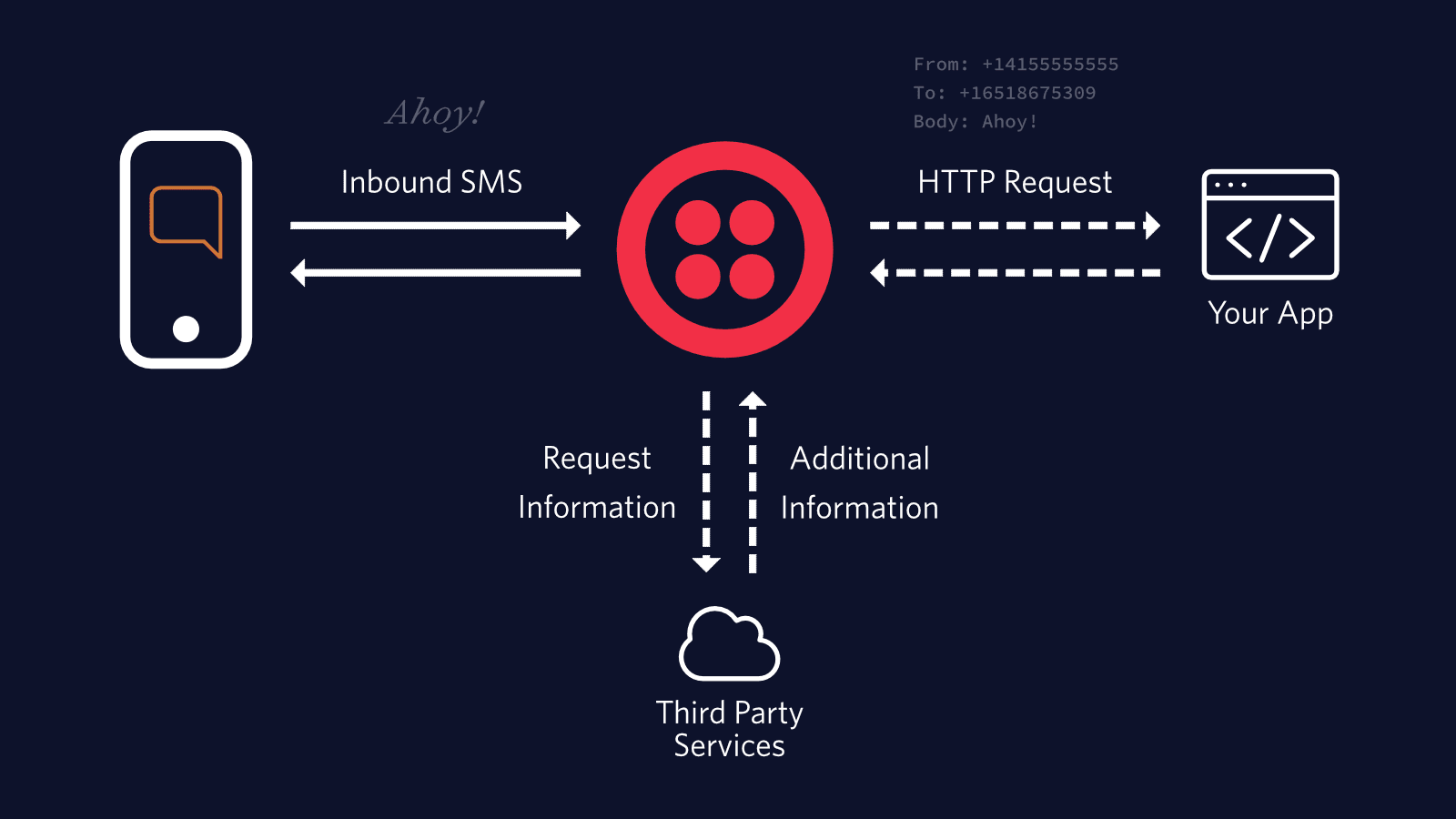
When you're ready to dig deeper into handling incoming messages, check out our guide on how to Create an SMS Conversation and our Automated Survey tutorial.