JavaScript Platform Overview
Twilio's Programmable Video JavaScript SDK lets you add real-time voice and video to your web applications. The JavaScript SDK connects to a Twilio Video Room and allows you to control the client-side video conference experience.
Info
Intending to write back-end JavaScript with Twilio in Node.js to support your front-end client? Check out the Twilio Node.js SDK.
Your application also needs a back-end server to create Access Tokens that the JavaScript SDK can use to connect to a Video Room. Learn more about the components of a Twilio Video app.
You can include the JavaScript SDK in your application either by installing it with Node Package Manager (npm) or using the Twilio CDN.
Install the Video JavaScript SDK using npm:
npm install --save twilio-video
Then, you can start using the JavaScript SDK in your application by importing it:
const Video = require('twilio-video');
You can also copy twilio-video.min.js
from the twilio-video/dist
folder after npm installing it and include it directly in your web app using a <script>
tag.
<script src="https://my-server-path/twilio-video.min.js"></script>
Using this method, you can access the JavaScript SDK through the Twilio.Video
browser global:
const Video = Twilio.Video;
You can also include the JavaScript SDK in your application from Twilio's CDN:
<script src="https://sdk.twilio.com/js/video/releases/2.22.1/twilio-video.min.js"></script>
You can access the JavaScript SDK through the Twilio.Video
browser global:
const Video = Twilio.Video;
Info
You should make sure you are using the latest Twilio Video JavaScript SDK release. To find the CDN link for the most recent JavaScript SDK release, visit the JavaScript SDK latest release documentation.
The JavaScript Video library requires recent versions of Chrome, Safari, Firefox or Edge (Chromium).
Chrome | Firefox | Safari | Edge (Chromium) | WebView | |
---|---|---|---|---|---|
Android | ✓ | ✓ | - | - | - |
iOS | ✓ | - | ✓ | - | ✓ |
Linux | ✓ | ✓ | - | - | - |
macOS | ✓ | ✓ | ✓ | ✓ | - |
Windows | ✓ | ✓ | - | ✓ | - |
Warning
Some MediaTrackConstraints are not supported by Safari.
In your application, you can check if a user is on a supported browser using the isSupported
flag.
1// import twilio-video, if you've used npm to install the SDK2const { isSupported } = require('twilio-video');34// if you are using the SDK via a script tag or the CDN, you would5// access isSupported as follows:6// const isSupported = Twilio.Video.isSupported;78if (isSupported) {9// Set up your video application...10} else {11console.error('This browser is not supported by twilio-video.js.');12}
WebView is now supported on iOS 14.3 and onward from JS SDK version 2.21.0.
twilio-video.js now supports WKWebView and SFSafariViewController on iOS version 14.3 or later. The flag isSupported
relies partly on the User-Agent
string to determine if twilio-video.js officially supports the user's browser. If your application modifies the default value for the User-Agent
string, the new value should follow the correct format. For iOS applications, your application will need to include the camera usage description, microphone usage description, and inline media playback for the SDK to work on WKWebView.
Warning
There are some common issues such as interruptions on mobile devices from backgrounding the application or switching between applications. These can sometimes cause VideoTracks
to go black or AudioTracks
to stop.
Android WebView is not currently supported by the JS SDK and the flag isSupported
will return false
if you use the JS SDK on Android WebView.
There are many resources you can explore when starting to build your first Twilio Video applications with the JavaScript SDK. You can follow a tutorial, read documentation, or deploy a pre-built sample video application.
Below are several tutorials that show you how to build an application from the ground up using the JavaScript SDK.
- Twilio Video webinar: A recording of a Twilio Video webinar, which introduces Twilio Video and walks through building a sample video chat application with Python and the JavaScript SDK.
- Get started with Twilio Video using the JavaScript SDK (the languages and frameworks specified below are for the language of the Access Token server).
- Python and Flask
- Node.js and Express
- Ruby and Sinatra
- PHP and Symfony
- C#/.NET and Blazor WebAssembly (uses AngularJS)
- Serverless with Twilio Functions (uses Twilio Functions to host your application and token server)
Twilio's Blog has many posts about building applications with Twilio Video. You can explore many different Twilio features and see examples using a variety of languages and frameworks. To find all Video blog posts, filter posts for the "Video" tag. You can also find translated blog posts on the Twilio Blog.
Build a basic video application with the JavaScript SDK.
Twilio's Code Exchange is a repository of code samples for common Twilio use cases.
- Basic JavaScript SDK Video Chat application (this is one-click deployable, no local setup required)
- List of all Code Exchange Video applications
Quickstart applications are minimal Twilio Video applications that demonstrate the basics of working with Twilio Video.
Quick Deploy applications are more full-featured than the Quickstart applications above. They demonstrate a wide variety of Twilio Video functionality and can be used to quickly get started with a robust set of Video tools.
Try out and experiment with a basic CodeSandbox that uses the Twilio Video JavaScript SDK to display a local user's video.
View recommendations and best practices for building applications using the Programmable Video JavaScript SDK.
Twilio has tools for understanding, troubleshooting, and enhancing your Twilio Video applications throughout the development lifecycle. You can use these tools to perform pre-call quality checks, as well as to gain insight into your application's performance and participants' experiences during or after a video call.
The Twilio Video Diagnostics Application is an open-source ReactJS application that tests participants' device and software setup, connectivity with the Twilio Cloud, and network performance. It uses Twilio's RTC Diagnostics SDK and Preflight API to provide end-users feedback about their network quality and device setup and also includes recommendations for improving their video call quality.
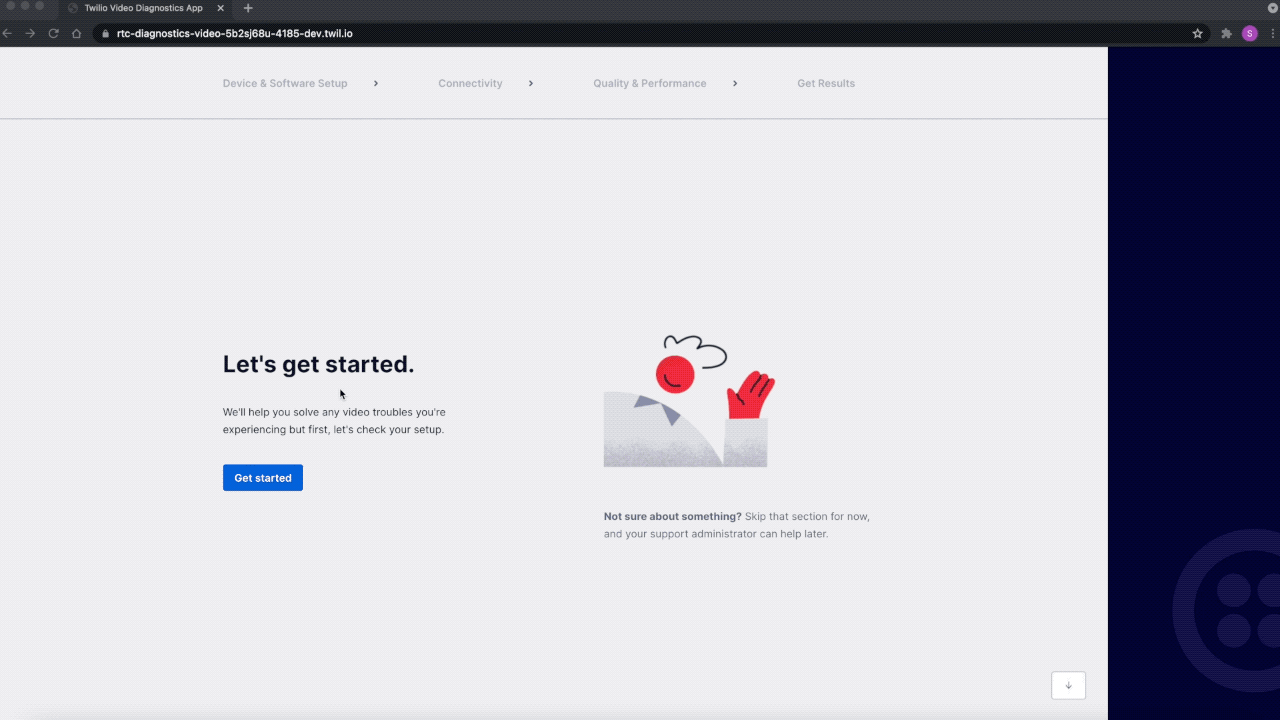
Learn more about the JavaScript Video diagnostics application.
The RTC Diagnostics SDK and Preflight API can also be used independently in your application to test your participants' device setup and network connectivity.
Twilio's RTC Diagnostics SDK for JavaScript applications provides functions to test a participant's input and output devices, including microphones, speakers, and cameras, as well as functionality to confirm that a participant meets the network bandwidth requirements required to make a voice call or conduct a video call.
This is a general WebRTC SDK and does not rely on Twilio infrastructure. You can incorporate it into your applications for pre-call testing or troubleshooting user issues during a call.
The Preflight API provides functions for testing connectivity to the Twilio Cloud. The API can identify signaling and media connectivity issues and provide a report at the end of the test.
Twilio's JavaScript Room Monitor is a browser-based tool that displays real-time information and metrics about a Twilio Video Room. It gathers and processes information from the Room object, including information about Participants' bandwidth, packet loss, and jitter, and displays the information in a modal window in the video application.
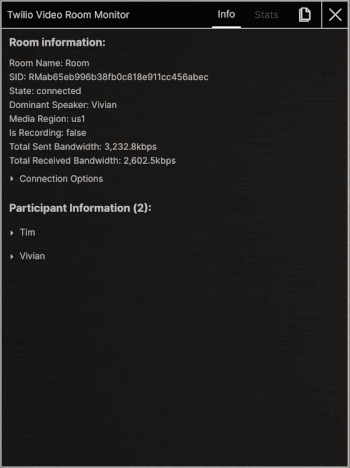
The JavaScript Room Monitor can be added to any Twilio Video JavaScript application to help during all stages of development and/or for debugging in-progress calls.
The JavaScript Logger allows you to capture logs generated by the Twilio Video JS SDK in real-time so that you can monitor your frontend applications and see how they behave in production.
Twilio Video Processors is a collection of tools for applying transformations and filters, such as blurred backgrounds and virtual backgrounds, to Twilio VideoTracks
. See a live demo with blurred backgrounds and virtual backgrounds using the Video Processors tools.
The Twilio Video JavaScript SDK has autogenerated documentation for all classes and methods in the SDK.