Getting Started with Scala and Twilio
Time to read:
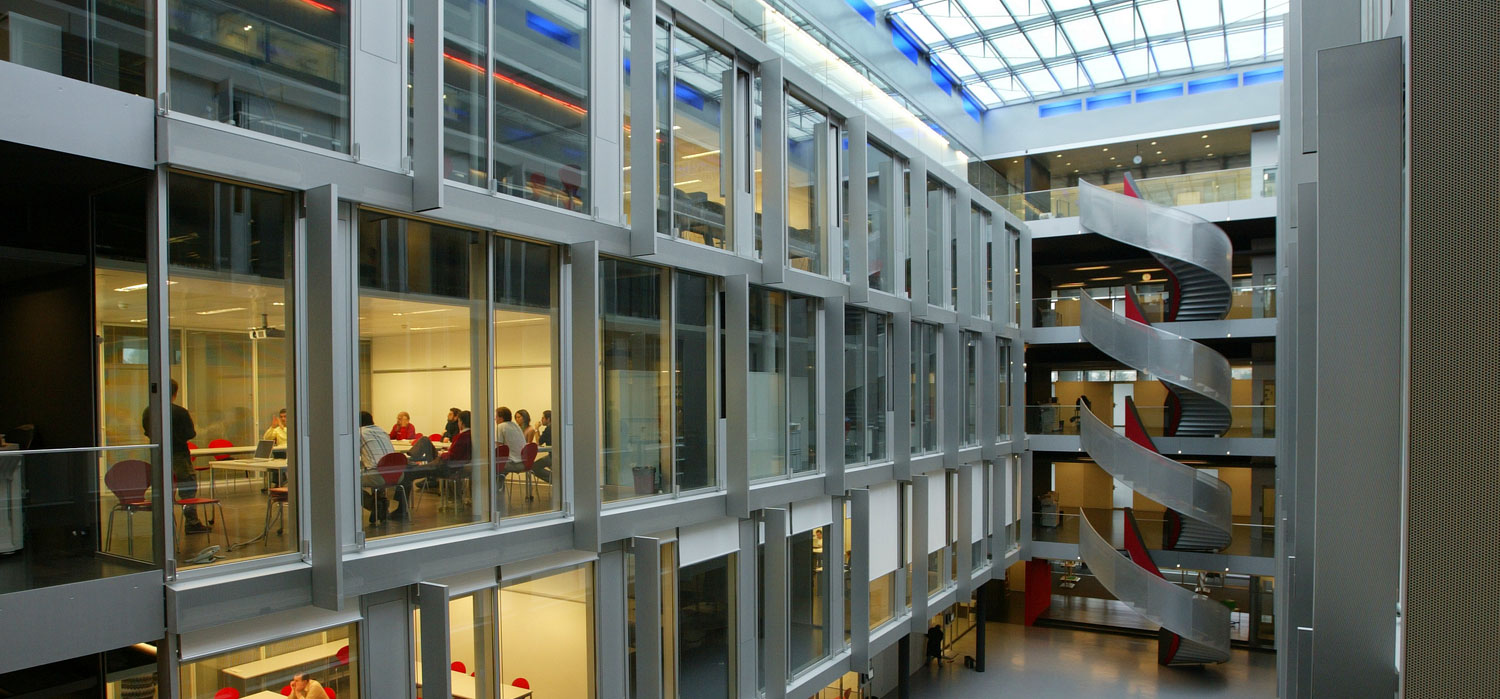
It’s functional, it’s object-oriented, it’s everything you never knew you wanted and more! In this post we’ll introduce Scala and give you the tools to build a Scala application that can send text messages using Twilio from the command line.
Scala is a JVM language that is notable for its combination of Object Oriented and Functional programming styles. Over the last fifteen years it’s gained popularity with use cases ranging from web programming to data engineering to distributed systems. Companies like Twitter, Stripe, and Twilio have all built large systems on top of the language.
Hello World
Let’s dive in. Here’s what you’ll need:
- Java JDK 8
- SBT, I’m using version 0.13.6
- Text editor (I highly recommend using an IDE like IntelliJ, the community edition is free and fully supports Scala)
- A Twilio account (you can sign up for a free account if you don’t have one)
- A Twilio phone number that can send and receive SMS messages
Check that you have Java and SBT installed:
With SBT installed, you can now access the Scala REPL with sbt console
, try that now. Here’s a good cheat sheet for working in the REPL. Some things you’ll notice right away is that every value in Scala has a return type, and that those types can be inferred:
I recommend the Tour of Scala for a great introduction to the Scala syntax.
SBT is useful for more than just the console; it’s the tool that will manage your dependencies, execute your project, and run your tests. A neat feature was added last year that also makes it easy to build new projects from templates. The directory structure isn’t strict, but there are some best practices for organizing your files and project templates will set that up for you.
You can read more about SBT’s templates here. I created a template for our project so head over to your terminal and type the following. When it asks for the name of the project, you can name it whatever you like, I named mine hello
.
After you cd
into the directory, you can now run the project:
This will take a while to download all of the dependencies the first time you run it. Once the dependencies are downloaded, you’ll be prompted for your name:
Pretty neat, you’ve just set up your first Scala project! We’ll use this base for the rest of our application.
Sending Text Messages with Scala
Now that you have a Hello World project in Scala you can extend that to send text messages. For the messaging, we’ll use the Twilio API. Twilio doesn’t have a specific Scala SDK but we don’t need one — the Java SDK works swimmingly.
To add a dependency, you’ll need to edit your build.sbt
file, which is in the top level of your project. You’ll see a section for libraryDependencies
that already contains the config
library. Add another line for the Twilio library, which I found in the SBT tab on Maven.
Before you can use the Twilio library, you’ll need set up the SDK with your credentials. Sign up for a Twilio account if you don’t already have one and grab your Account SID and Auth Token from twilio.com/console. Save your account credentials as environment variables and overwrite the placeholder configuration in src/main/resources/application.conf
with the following code.
As you’re adding code, you can run sbt ~compile
. The ~
tells SBT to incrementally compile your project every time you save a file and will help catch errors in your program early and often.
In the src/main/scala/Main.scala
file, we can replace our greeting example with our Twilio code. First we’ll need to grab some necessary imports for the Twilio library. These can be placed at the top of your file with the existing imports.
Now you can initialize the Twilio client with our Account SID and Auth Token. Since you already have our config defined, you can fetch your credentials.
As with Java, Scala is strongly typed. A method’s type signature provides documentation for the parameters and I often reference source code for this information. As we see below, the MessageCreator class requires two PhoneNumbers
and one String
.
Our from
number is one of our stored config variables, so we can grab that and wrap it in the PhoneNumber
data type that Twilio provides. Let’s hard-code our to
number and body
for now:
Now that we have the necessary inputs, we can use those to construct and send our message.
At this point, we can try running our program from the command line with sbt run
– try that now and you should see a message come through.
Accepting User Input
Let’s make a few improvements to our design so we don’t have to rely on those hard-coded values. To accept user input in Scala, we’ll use the readLine method from the standard library’s IO package. The method returns a String
and we can save that to a variable for something like:
Try running the program again and texting yourself and your friends different messages.
Adding Resiliency
Let’s improve our message sender by handling errors better.. Because we’re using the Java SDK, most of the methods will throw Exceptions when they encounter an error. For example, here’s what happens if we try to send a text to an invalid number:
There are a lot of ways we can handle this error in Scala and we’ll be using Scala’s Try and pattern matching to catch this one. For our program, runtime exceptions would happen in Message.creator(to, from, body).create()
so that’s what we want to focus on error handling.
First we’ll import some utilities.
Wrap your message creation in a Try
; this type will either return a successfully computed value (Success
) or result in an exception (Failure
).
Now when you try to send a message to an invalid number you’ll receive a more friendly error message that you can customize.
Neat!
Next Steps
Congratulations! You’ve successfully built a Scala application that can send custom text messages. There are many ways you can extend this project, like easily adding emojis!
There are many other reasons to continue exploring what’s possible with Scala. You might be interested in using Scala to take advantage of the JVM ecosystem with less verbose code. Perhaps you’re looking to process terabytes of data using Apache Spark, a popular library written in Scala and with Scala APIs. Whatever your need may be, the Scala community has grown to support the breadth of applications developers are building.
You can find a completed version of this application over on my github: https://github.com/robinske/hello-twilio-scala. Hopefully you’re inspired to learn a bit more about Scala and if you’re looking for additional resources, I can recommend the following:
- Coursera Scala courses – learn about functional programming, concurrency, big data and more in these courses developed by the Scala Center.
- Scala exercises – challenge yourself with introductory puzzles in the browser for Scala and popular Scala libraries.
- Scala eBooks – read more in these free eBooks from Underscore.io, a Scala consultancy.
For more information about the language and its features, including a more in depth look at why (and why not) to use Scala, I talked about this at the GOTO Chicago conference last year. If you have any questions or comments about Scala, I always love hearing from you! Have fun getting started and I can’t wait to see what you build.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.