Making Phone Calls with Twilio & Blazor
Time to read: 5 minutes
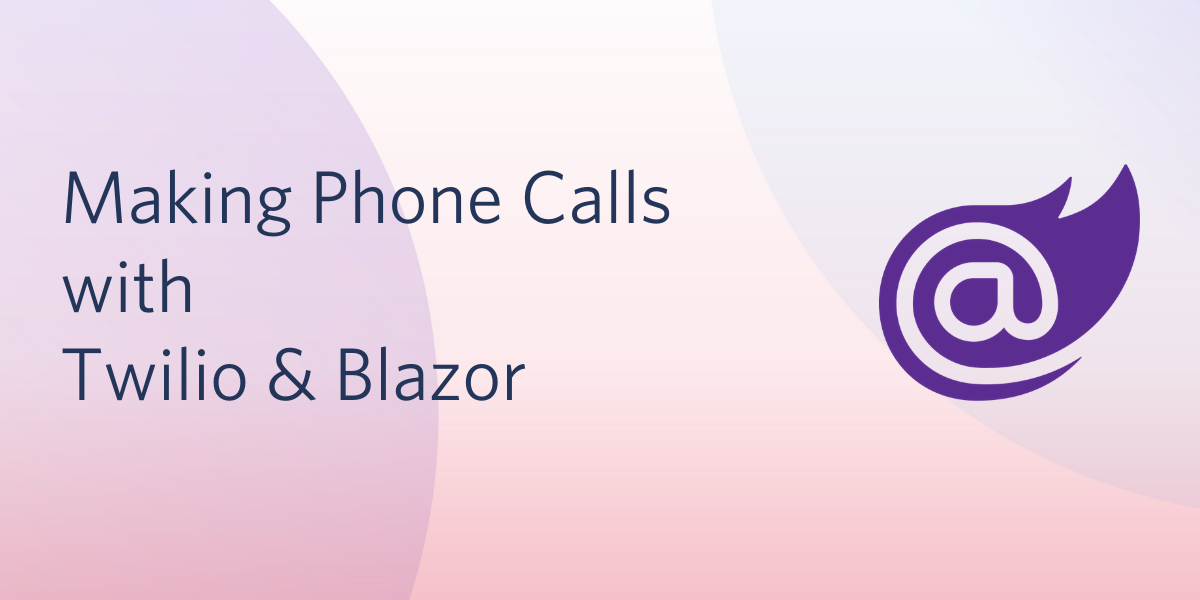
It's an exciting time to be a .NET developer as .NET Core 3.0 has reached general availability. With this release comes Blazor, Microsoft's take on building web applications using WebAssembly and the technologies you already know and love such as HTML, CSS, and C#. I published a prior blog post when Blazor was still in active development. It's time to join the release party and share our excitement about Blazor with Twilio and C#.
The first thing you should do when a new product gets announced is tell your friends, right - but what if we phoned them with a special note sharing our excitement? Let's build a web application that does exactly that - it uses Twilio to phone a friend, and is powered by Blazor.
Installing Our Developer Tools
Now in order to follow along - you'll need a few things first. You'll want to grab
- Visual Studio Community 2019 (we used version 16.3.5 and included the ASP.NET and Web Development workload)
- Ngrok Extensions (after installing Visual Studio)
- A Twilio Account
Once we've set up our Twilio account, let's head over to the Twilio console and purchase a phone number to use in our app. Click the add button and enter an area code of your choosing to purchase a number from the results.
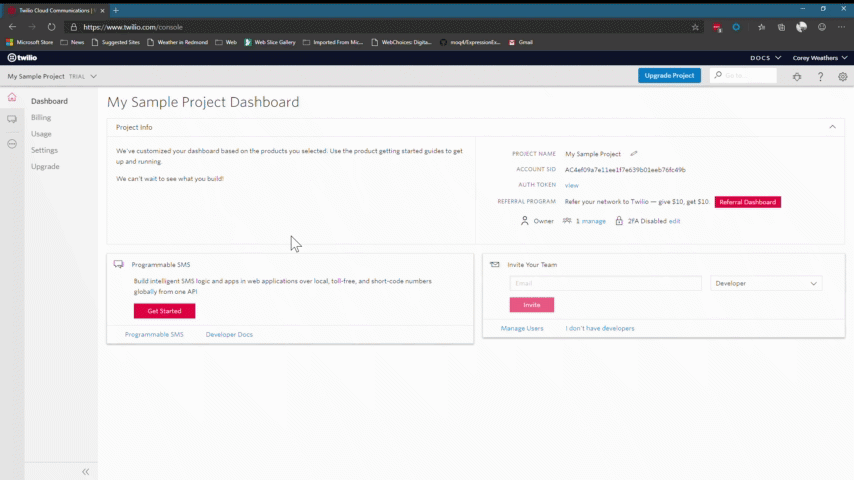
Now that we have a phone number, let's jump into some code.
Setting Up Our Developer Environment
Start up Visual Studio and then click Create a New Project. In the project dialog, we'll type Blazor
in the search box and select Blazor App
. Next, we'll give the project a name. I'm calling mine Dial-A-Friend
but you can call and place yours in a location of your choosing before hitting the Create
button.
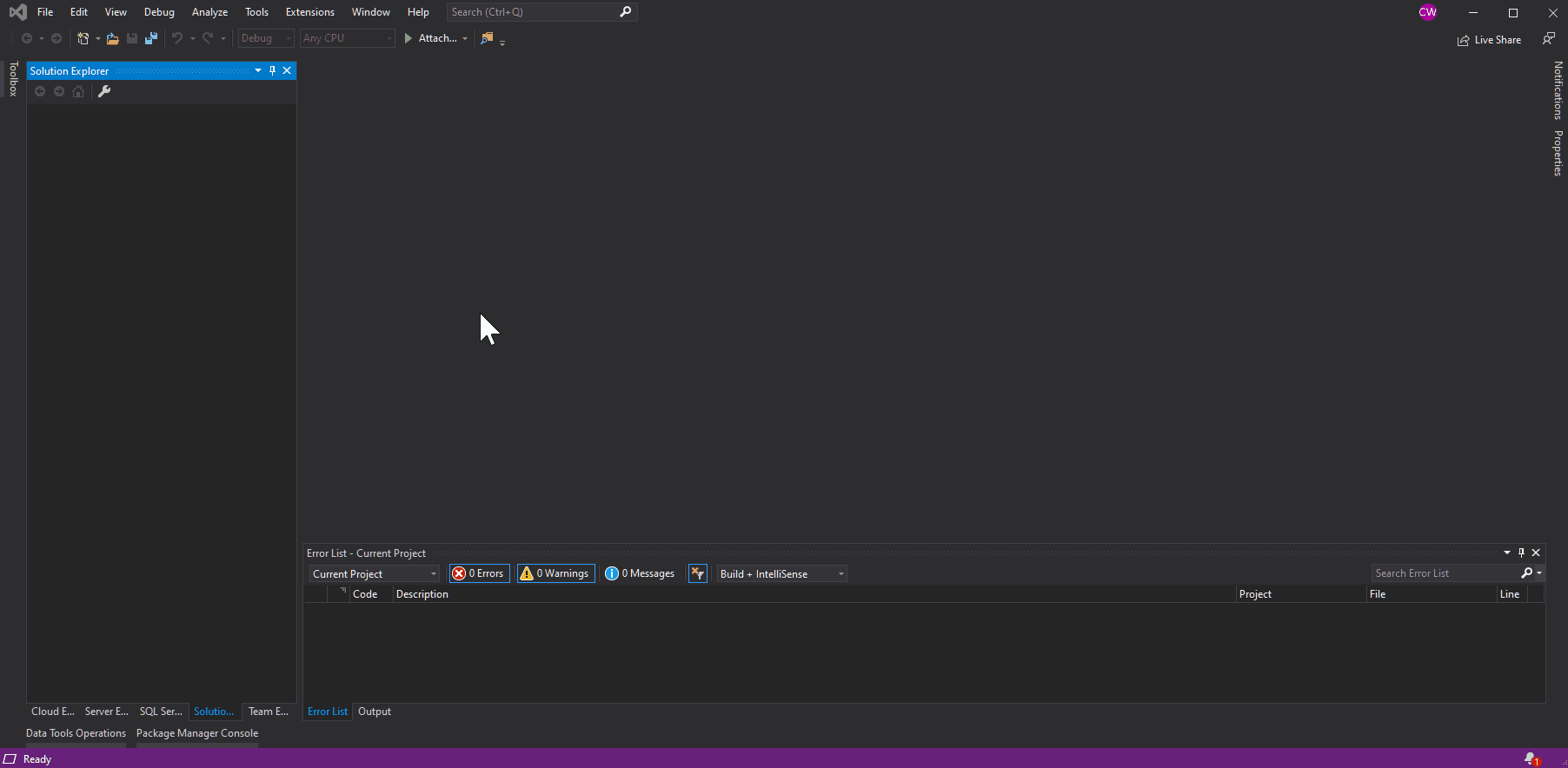
One more thing - we need to add an API project to our solution. Once our project is created, right click on the solution name → Add → New Project. This time, we'll type C# api
in the box and select the ASP.NET Core Web Application result. Name it api
before clicking the Create button, and then select API from the list of applications. Now that our Blazor & API projects are created, let's write some code.
Building a Twilio Voice API
First, let's add code to our API project that gets us connected to Twilio. We'll do this by creating a controller and adding two methods - the first retrieves a client token and the second returns our voice TwiML to Twilio when a call is placed.
To get started, right click on the api project in the Solution Explorer→ Manage Nuget Packages and search for Twilio
. Once we find that, hit the Install button. Now let's right click on Controllers → Add → Class and name it TwilioBackEndController.cs
. Here we're going to paste in the below code:
Next we should replace the AccountSID, AuthToken, and PhoneNumber with the ones in the Twilio console. We'll update the AppSid in a little bit. With our API code in place, next up is building our user interface.
Creating a Blazor Application
We'll start by creating a page in our Blazor project. In the Solution Explorer, right click on Pages → Add → New Item. In the dialog window, select Razor Component
and change the name to Dial.razor
before pressing Enter. Then we'll replace the code in that file with the following:
This code does a few things. It sets up a /dial route for us to navigate to, creates a text box and buttons to dial phone numbers, and wires up our buttons to C# code on events. Notice that our C# code doesn't quite exist yet. So let's copy this code to the bottom of Dial.razor:
Now we'll add our new page to the navigation menu provided in the project we're using. In the Solution Explorer, open the Shared folder → NavMenu.razor file and copy the highlighted lines right underneath the Home entry:
With this change we'll see Call a Friend which routes to our new page right in the navigation menu.
Making Phone Calls with the Twilio Client SDK
Its time to add the Twilio client SDK into our application in order to start and end phone calls. In _Host.cshtml (which can be found in the Pages folder), copy the highlighted lines below the <script src="_framework/blazor.server.js"></script>
line:
Notice that we're adding a reference to scripts/site.js
here. We need to add some Javscript to interact with Twilio's client. Under wwwroot, create a folder called scripts, and add a new item in there called site.js.
We're going to copy the below code to site.js:
Starting an Ngrok Tunnel in Visual Studio
If we ran this right now, it would fail - we've got just a few more tasks to take care of. First we need to get our Ngrok URL to allow Twilio to call into our API. In Visual Studio, head to Tools → Start Ngrok Tunnel. This will install the ngrok tool to your computer and run it for your projects.
You may see two URLs like in the photo below:
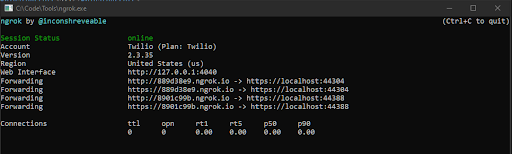
To determine which ngrok URL connects to our API, right-click on the api project in the Solution Explorer → Properties. Click on the Debug menu option and look for the App URL, and Enable SSL settings, as in the photo below:
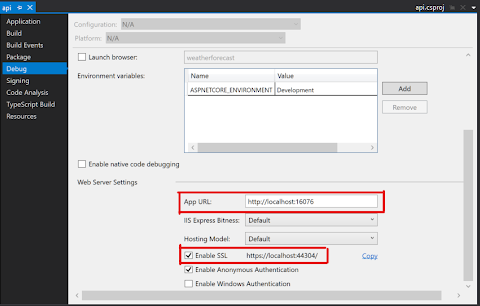
One of those URLs will have an ngrok URL we can use to connect to our API. Time to configure our TwiML App.
Creating a TwiML App in Twilio's Console
The Twilio client uses a TwiML app when we attempt to place a voice call from our Blazor application. To create a TwiML app, let's head to the Twilio console, enter a friendly name for our app, and set a URL for the Voice configuration. We will use our ngrok URL https://<REPLACE_WITH_YOUR_NGROK_URL>/api/twiliobackend
, replacing the first part of the URL with your own ngrok hostname.
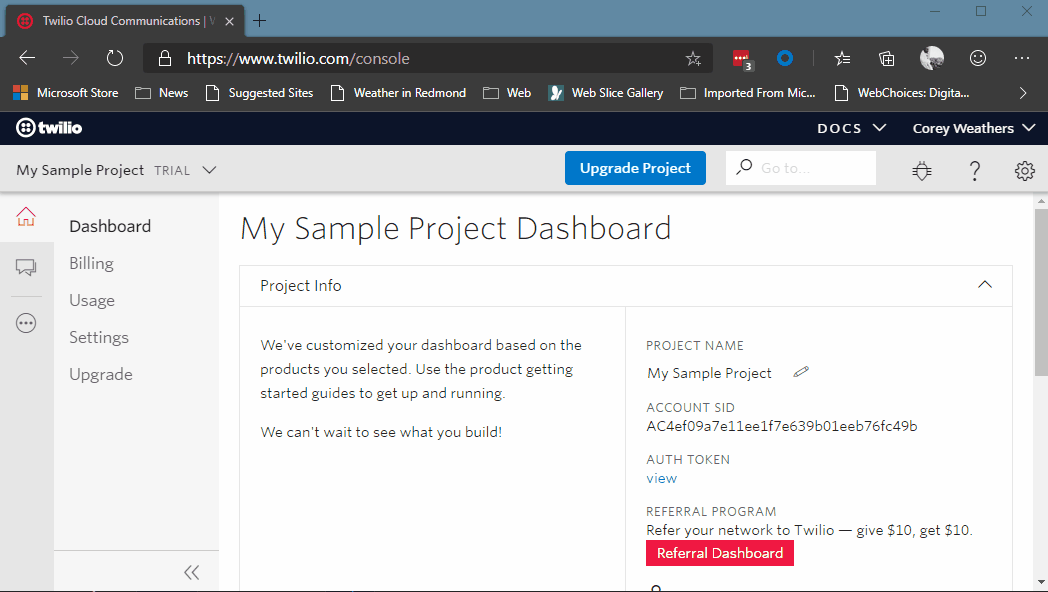
Next we'll copy the App Sid listed in the console and copy it into the TwilioBackEndController.cs in our API project, replacing the <REPLACE_APP_SID_HERE>
value. Finally we'll copy that same ngrok URL into our Dial.razor file, replacing the <REPLACE_WITH_NGROK_URL>
.
Call Your Friends (aka Run our Application)
Are you ready to make a phone call? In Visual Studio, right click on the solution name in the Solution Explorer → Set Startup Projects. Select Start on both of the projects listed, click the Ok button, and hit F5 on your keyboard to start this thing up. Enter a number, call a friend, and enjoy your conversation 😉.
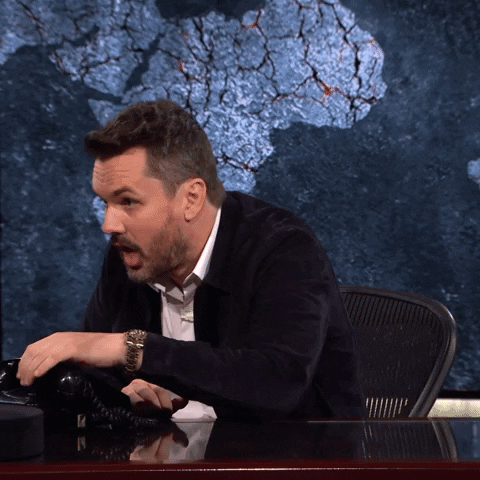
What's Next
Great! You've called someone using Twilio, Blazor, and your web browser. How cool is that! What if you could add some cool features like a dialpad, or setup your call to play a message before connecting. These are just a few of the ideas you can use to develop this further. Here's the Github repo that contains the completed project you've just built to try more things out.
In the meantime, drop me a note at corey@twilio.com or @coreylweathers on Twitter if you have questions, run into trouble, or want to chat more cool ideas. That's all for now. I can't wait to see what you build!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.