3 Ways to create an API in Python
Time to read:
3 Ways to create an API in Python
Creating an API (Application Programming Interface) in Python can be a straightforward process, thanks to various frameworks and libraries available. In this post, we'll explore three popular methods to set up a web API in Python: Flask, FastAPI, and Django Rest Framework.
Prerequisites
- Python 3.9 or higher
- IDE or Code Editor of your choice, I recommend Visual Studio Code
- Postman or Postman Visual Studio Code extension (optional)
Flask
Flask is a lightweight framework that can be used to develop web applications and APIs. To create an API using Flask, start by creating a new directory for your application.
Now, create a virtual environment using the following command:
This will create a new directory .venv in our project folder for our virtual environment.
Install Flask with the following command.
Now, to create our API, create a new file main.py and add the following:
To run our application, open a new window in your terminal and run the command to execute the script:
You'll have a basic API that returns a greeting message when accessed at http://localhost:5000/api/greet .
You can test this either in your browser, or by sending a GET request in Postman to the http://localhost:5000/api/greet endpoint. You will see a JSON response:
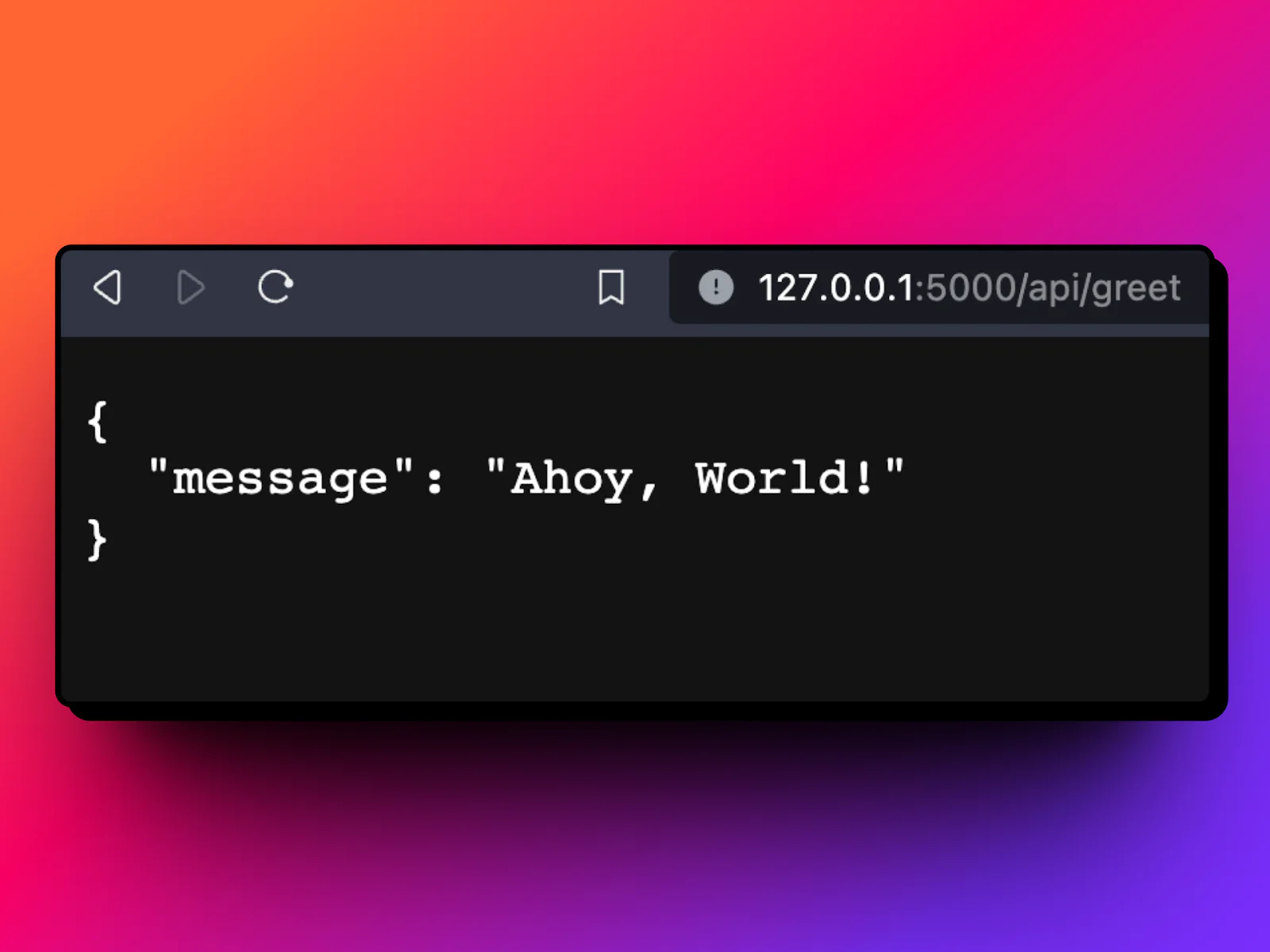
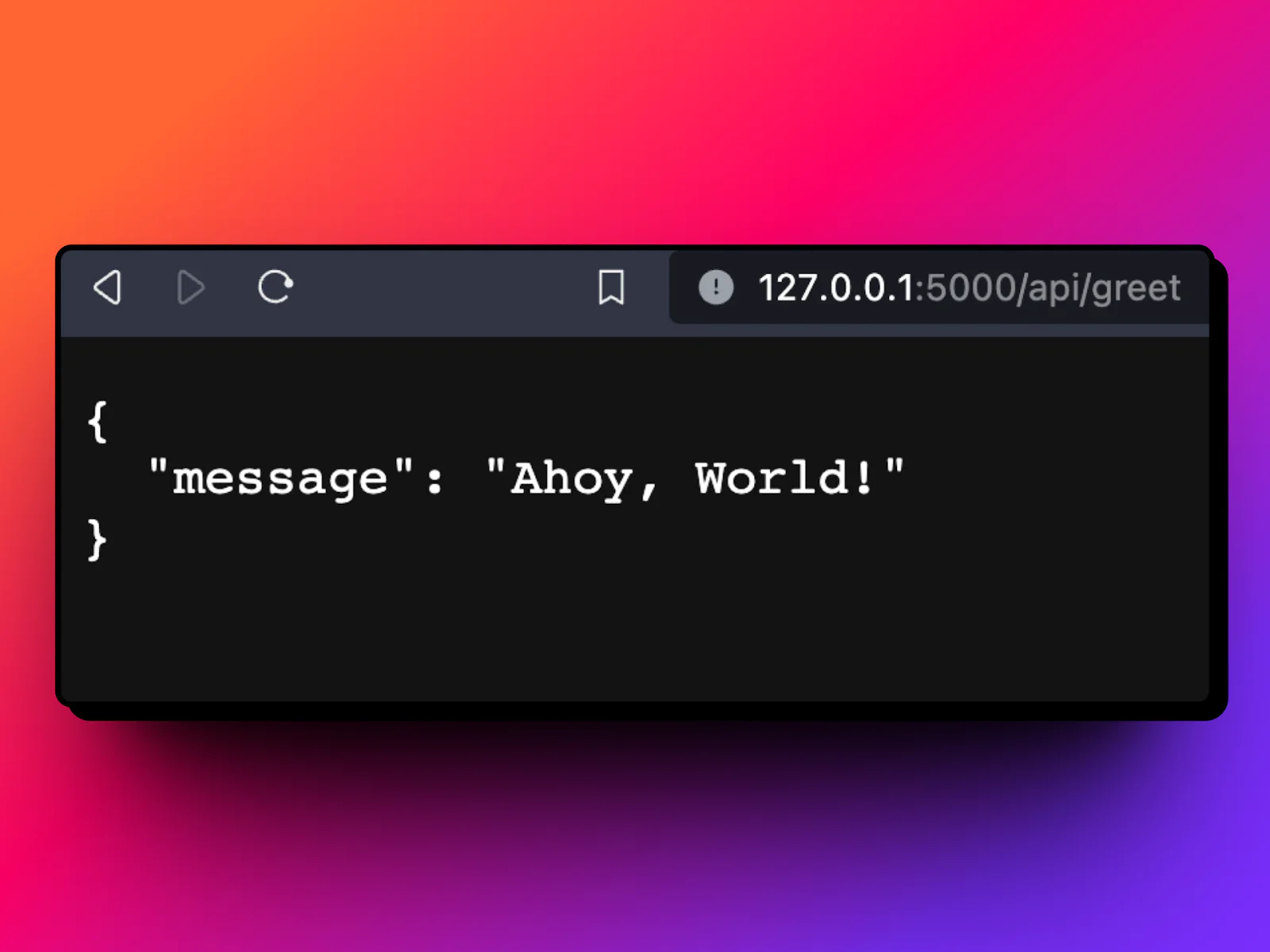
Now, create a virtual environment using the following command:
Let’s code a simple API, create a new file main.py and add the following code:
To run the FastAPI app, execute the script, by running the command in your terminal:
You now have a basic API that returns a greeting message when accessed at http://localhost:8000/api/greet
Again you can either use Postman, your browser or even test it in the terminal by using curl to test your API by sending a GET request.
Here is a curl example:
You should get a JSON response from the API:
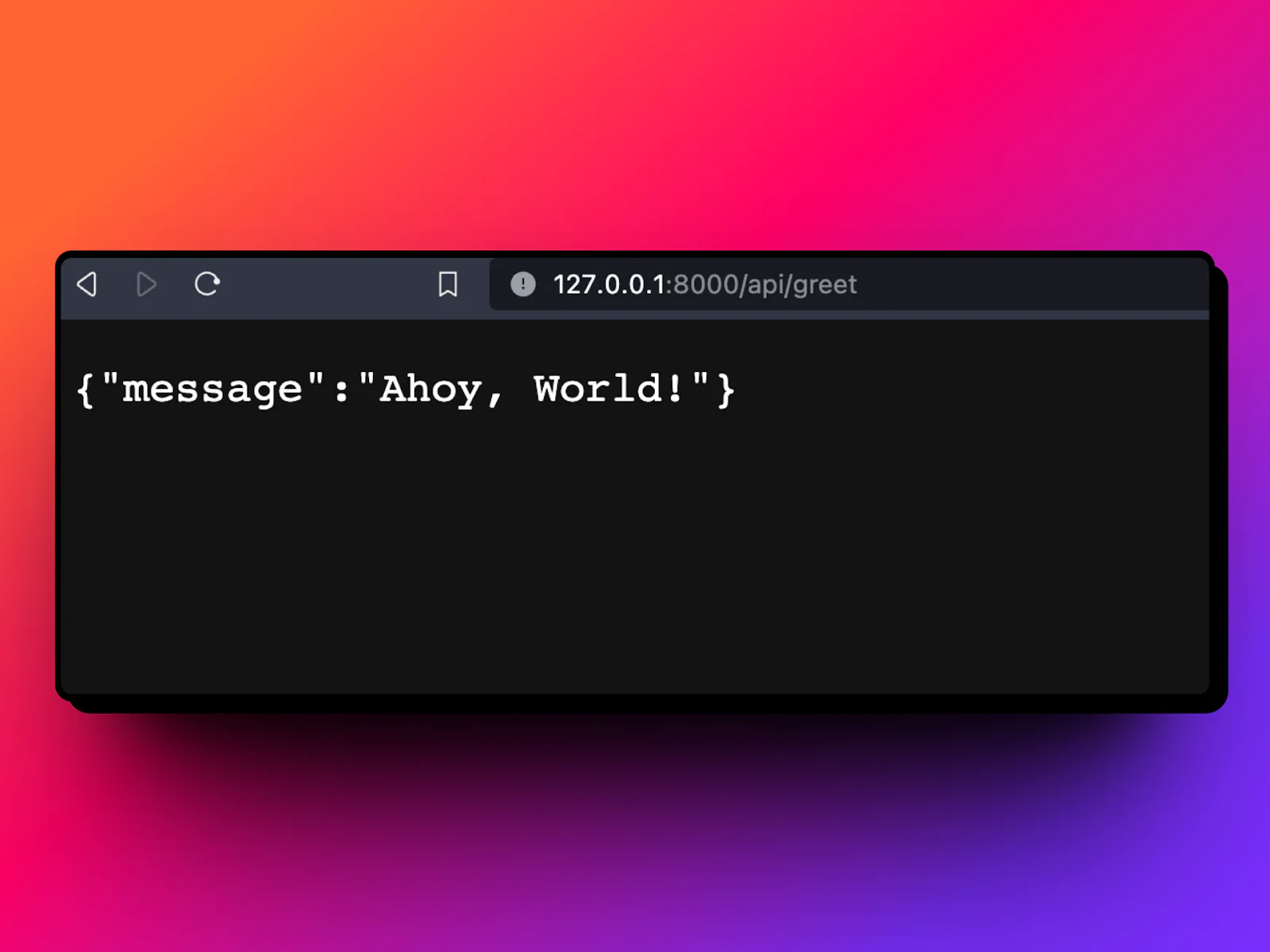
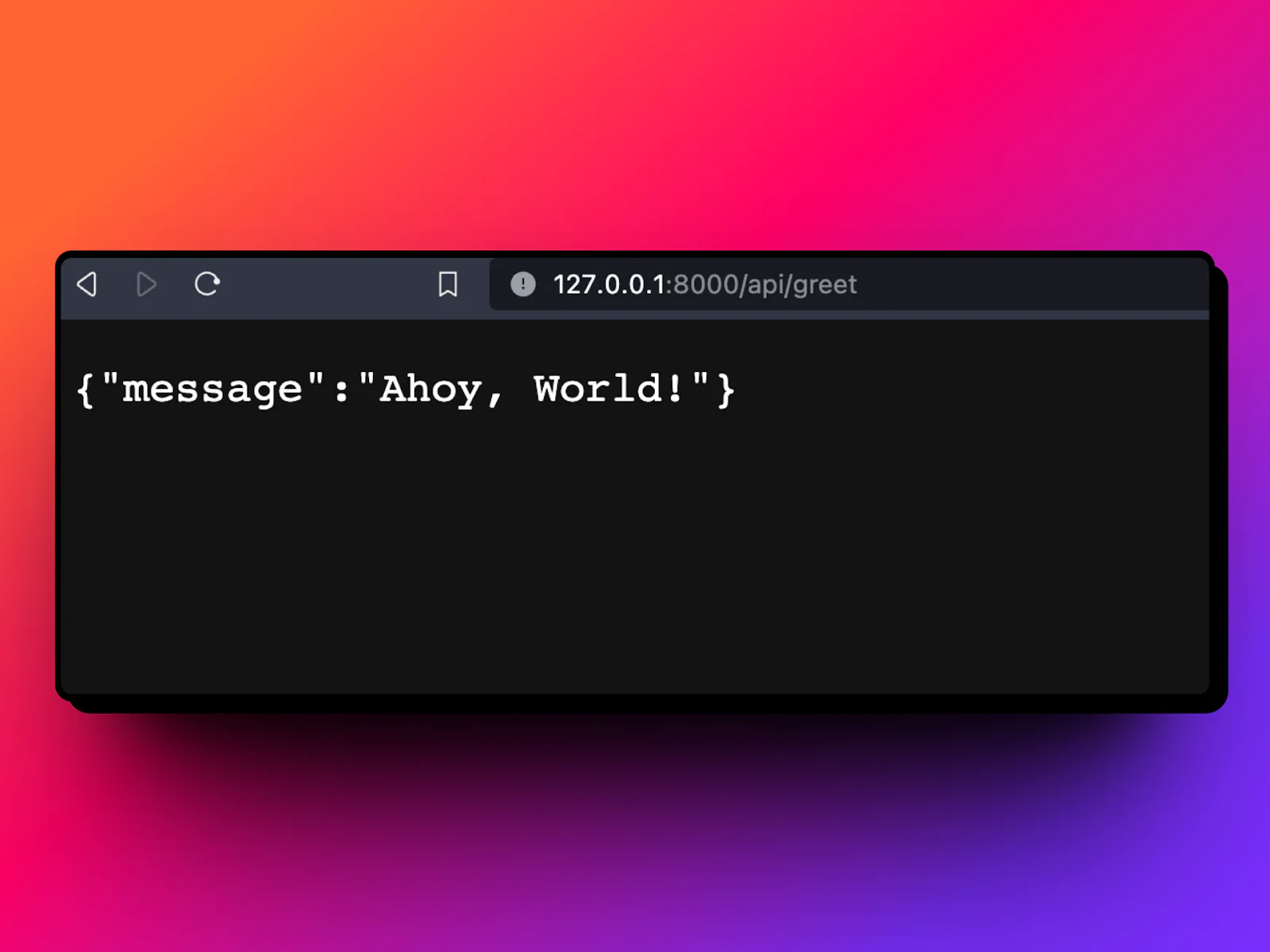
Also, you get Interactive API documentation automatically!
Head over to http://localhost:8000/docs in your browser to access them.
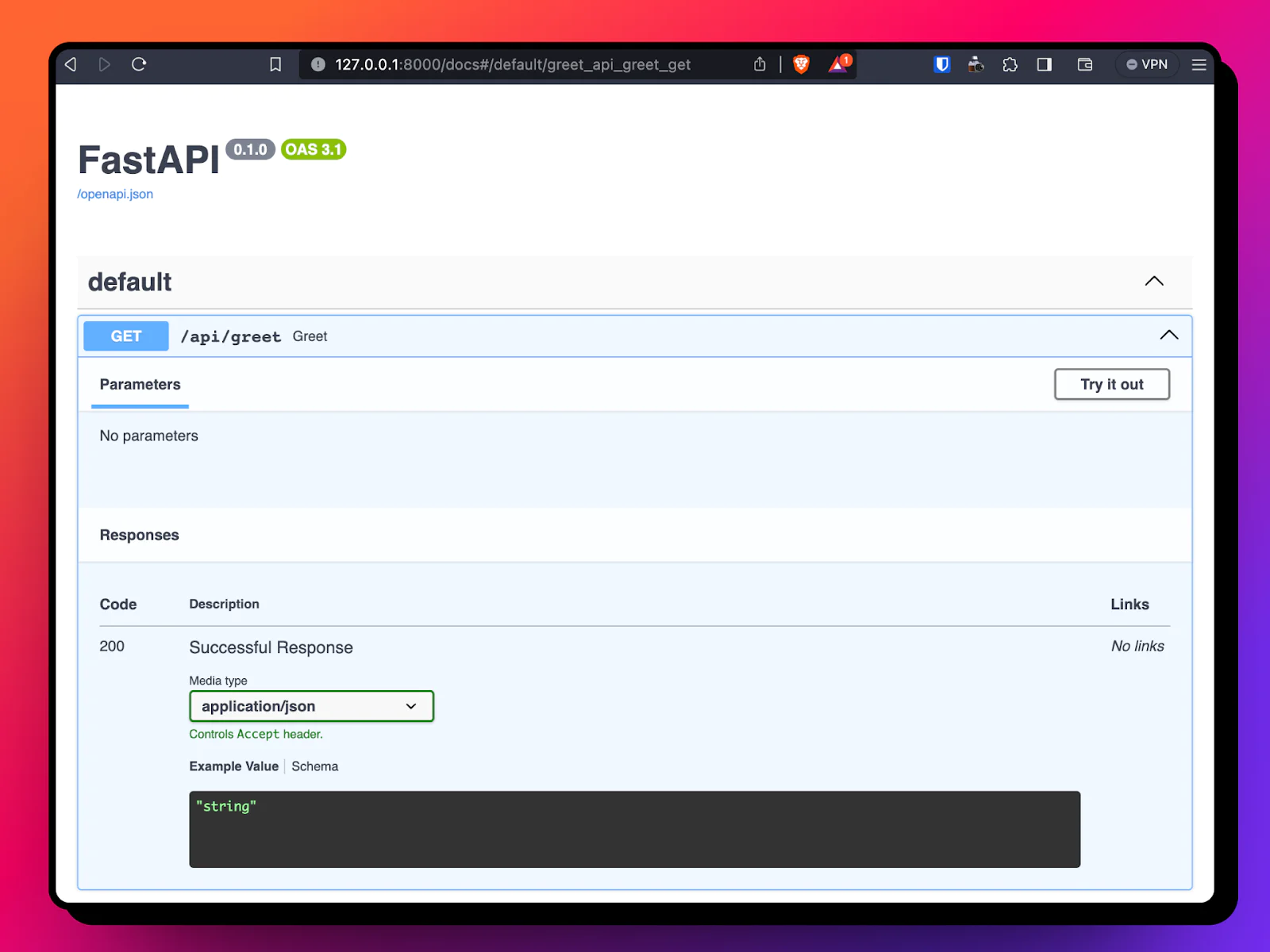
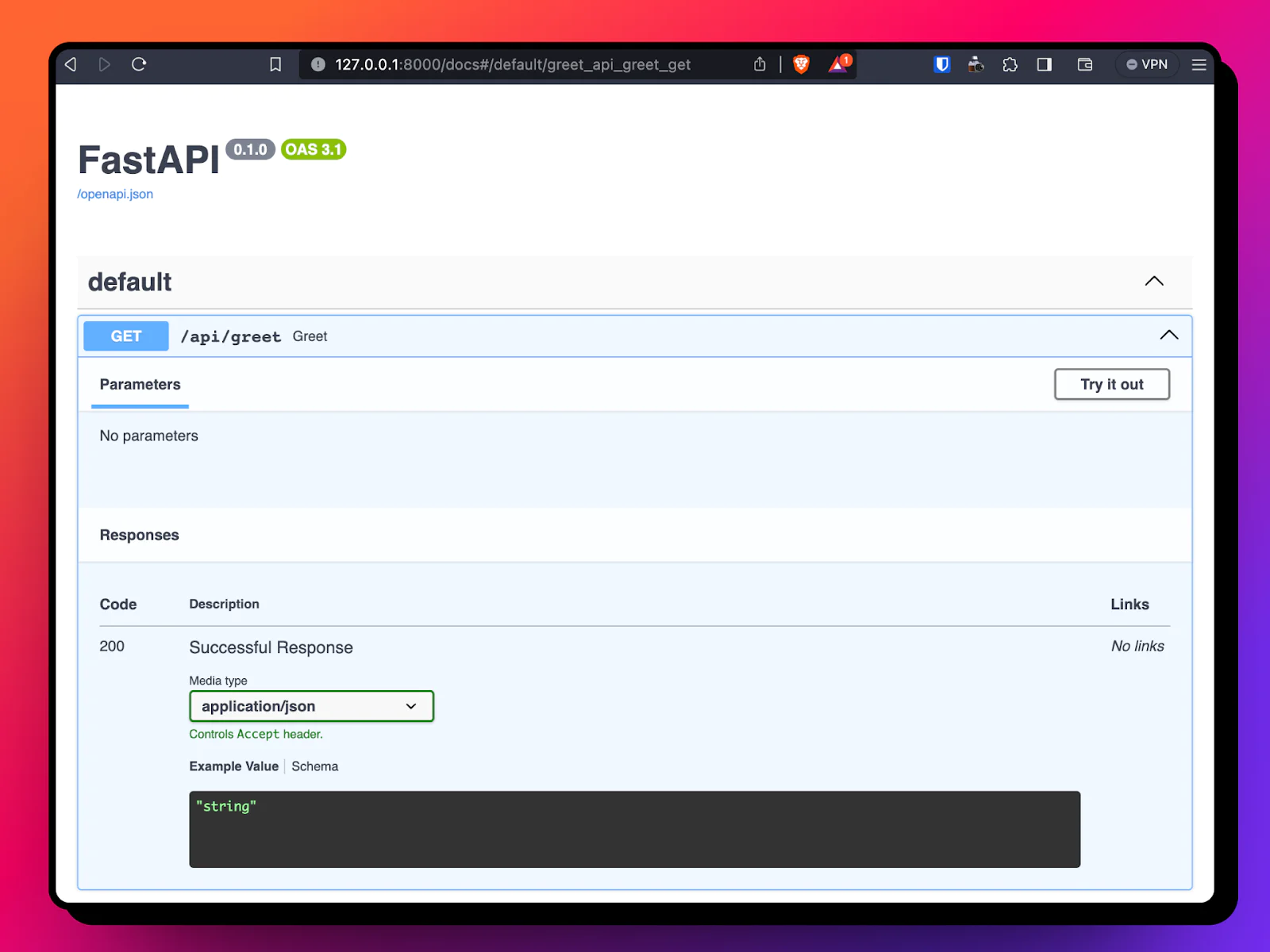
Django Rest Framework (DRF)
Django Rest Framework is a powerful and flexible toolkit for building Web APIs and is built on top of the Django framework.
Just like the previous two examples, start by creating a new directory for your application.
Now, create a virtual environment using the following command:
This will create a new directory .venv in our project folder.
Install Django and DRF.
Start a new Django project(django-api) and create a new app(api).
Now let’s write some code.
First, set up the model in models.py in the api directory
Next, create a file named serializers.py inside the api folder and add:
Set up the API view in views.py.
Now to set up the URL routing, create a file named urls.py inside the api folder and add:
Include your app's URLs into the main project. In django-api-example/urls.py, you will need to include the app's URLs:
Update Django settings, add rest_framework and api to the INSTALLED_APPS list in django-api-example/settings.py.
Now let’s run our API. Run migrations and start the server.
In your terminal, run the following command, make sure you are in the django-api-example directory.
Start the server.
Now, if you navigate to http://localhost:8000/api/greet/ in your web browser, you should see:
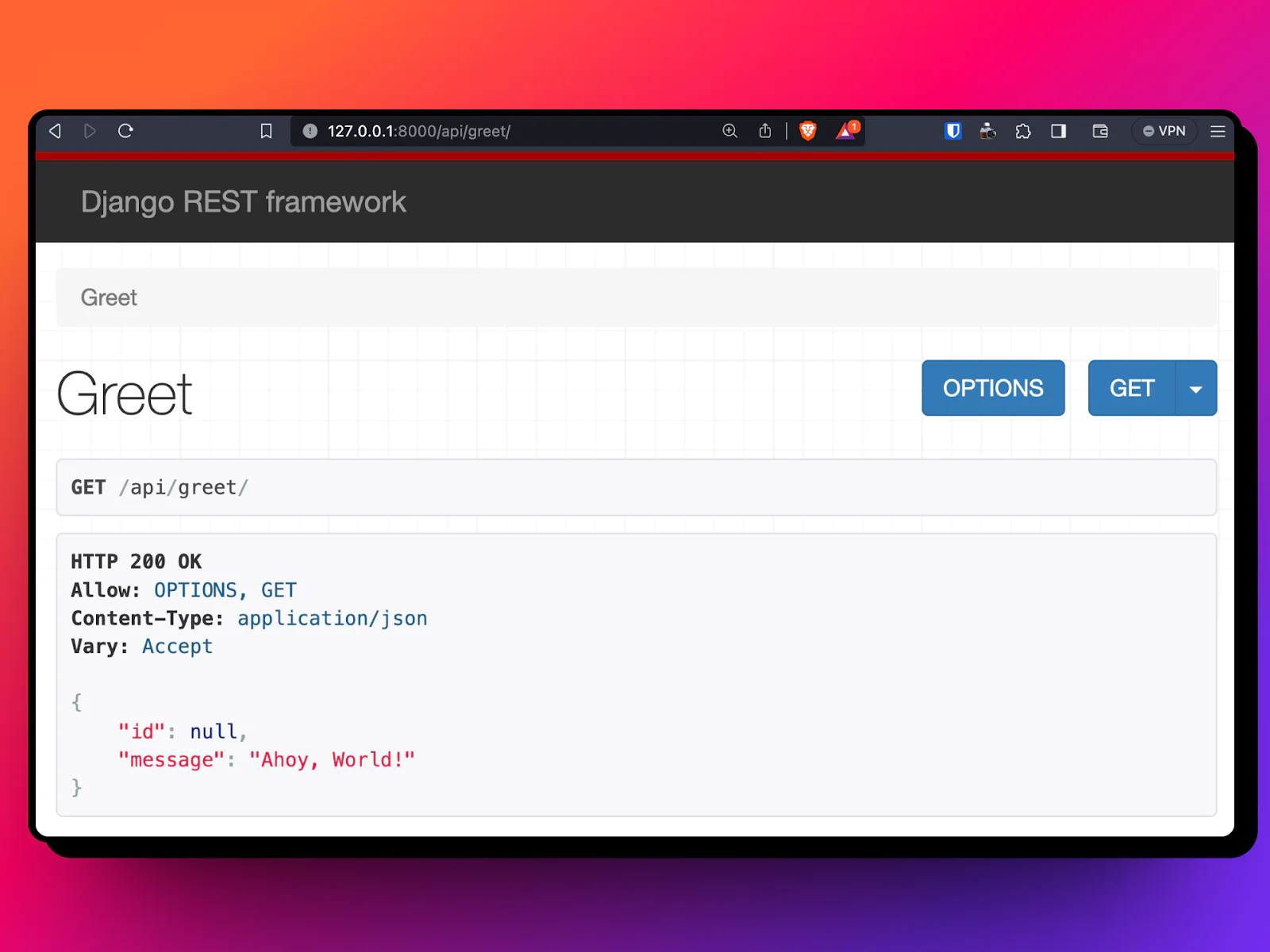
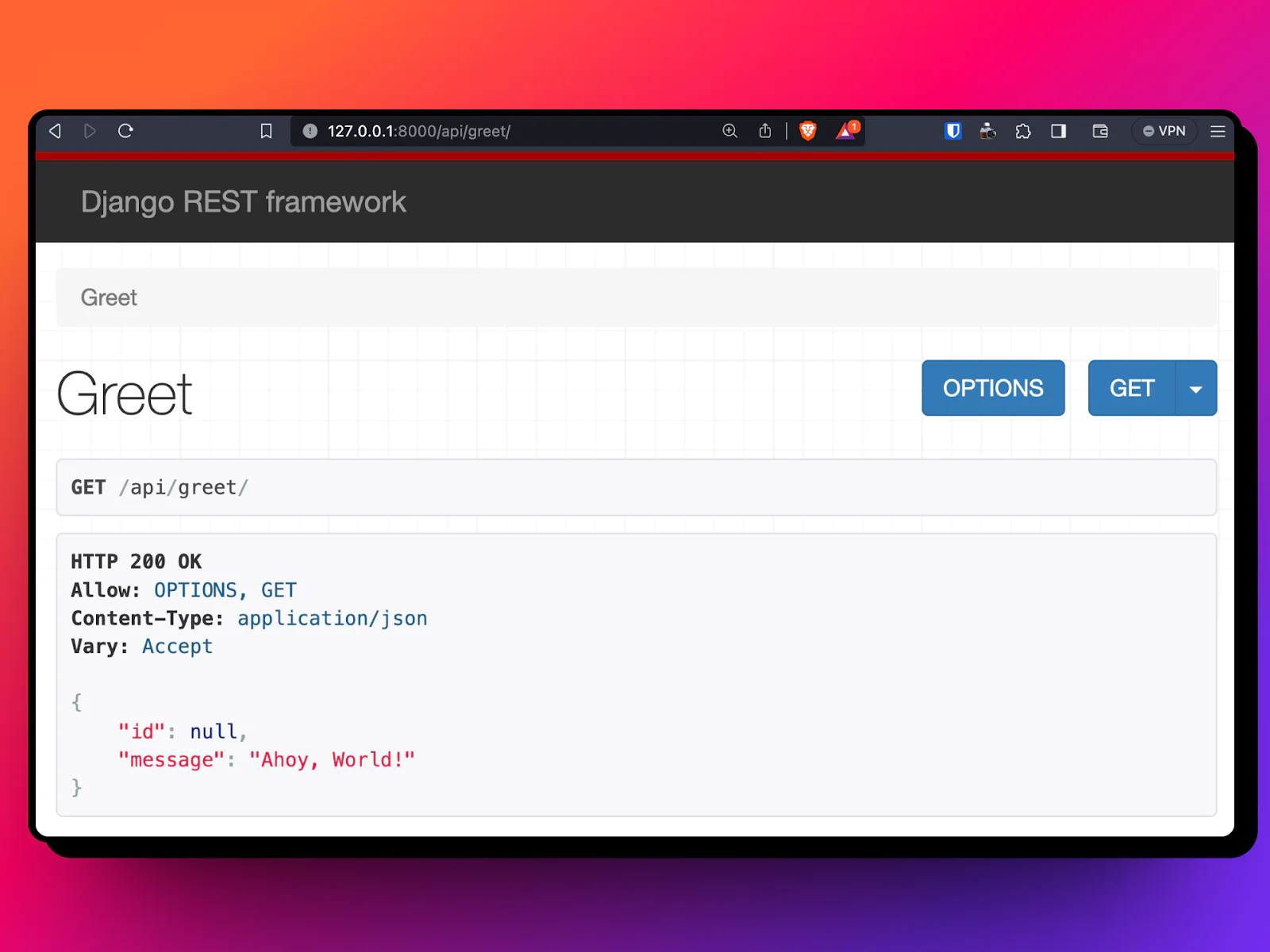
You can also add the JSON format parameter to the endpoint and use curl or Postman to try it out. Here is an example of a GET request with format parameter in the Postman Visual Studio Code extension.
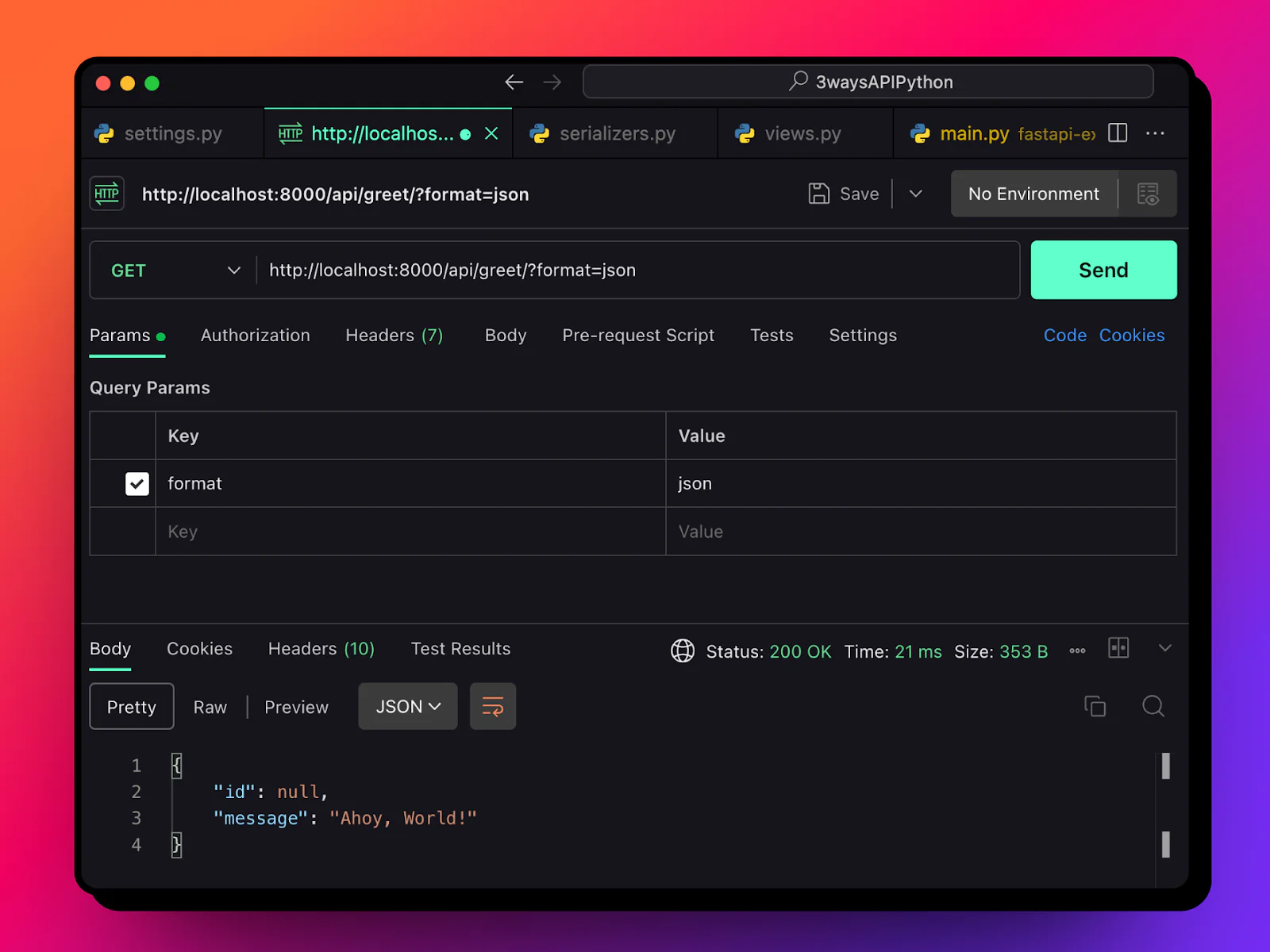
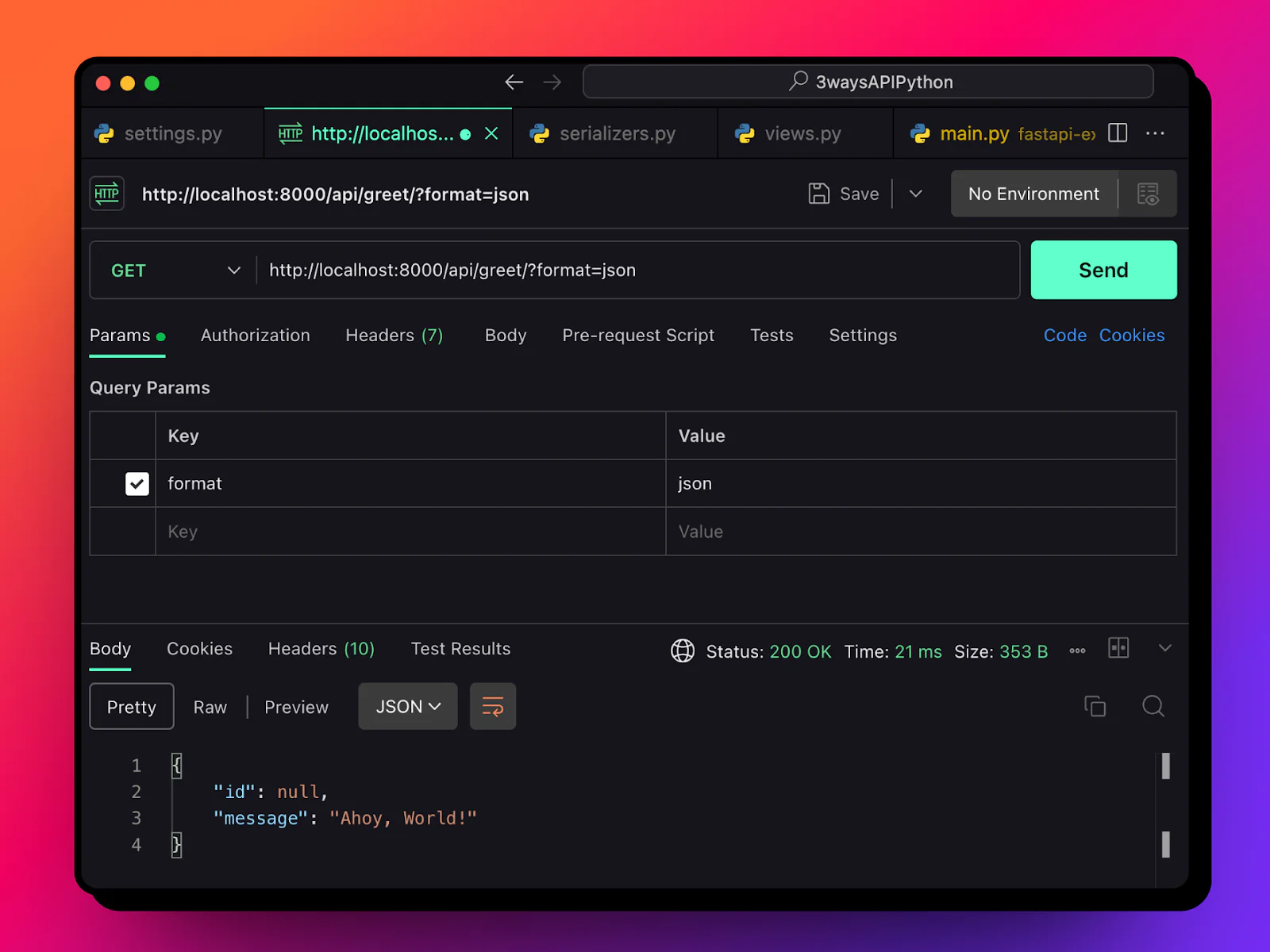
Conclusion
Whether you're looking for the simplicity of Flask, the speed of FastAPI, or the robustness of Django Rest Framework, Python offers a range of options to quickly and efficiently develop APIs. Your choice depends on the project requirements, your familiarity with the frameworks, and the features you need, let’s say you just started coding and looking to build your first API, try Flask, but if you want automatic API documentation with ease of use and speed - go for FastAPI. Whatever you choose, happy coding!
Rishab Kumar is a Developer Evangelist at Twilio and a cloud enthusiast. Get in touch with Rishab on Twitter @rishabk7 and follow his journey in tech on cloud, DevOps, and DevRel adventures at youtube.com/@rishabincloud .
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.