Call Monitoring with Node.js, Express and Twilio Call Progress Events
Time to read: 5 minutes
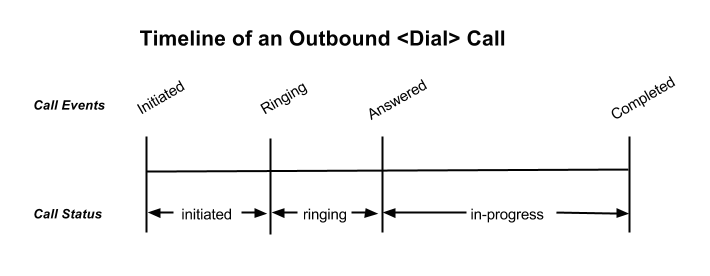
Call progress events are a really useful tool if you ever need to track the state of phone calls an app you built makes with the Twilio Voice API. Let’s say you are building a dashboard to manage the huge influx of calls that your heavy metal themed, over the phone radio app makes to listeners. By providing a status callback to your web app you can track the state of a voice call from when it is initiated to when it is completed by receiving requests in real-time whenever a call’s state changes.
In this post we will be going through the basics of how to set up a web app in Node.js using the Express framework to receive HTTP requests from Twilio whenever the status of a phone call changes.
Getting started
In order for everything to run correctly, you are going to need to have have Node.js and npm installed. At the time of writing this blog post, I have the brand new Node v4.0 and npm v2.14.2 installed. Node 4.0 allows us to use some newer ES6 features directly without having to transpile your code with something like Babel. You will also need to create a Twilio account and purchase a phone number with Voice capabilities:
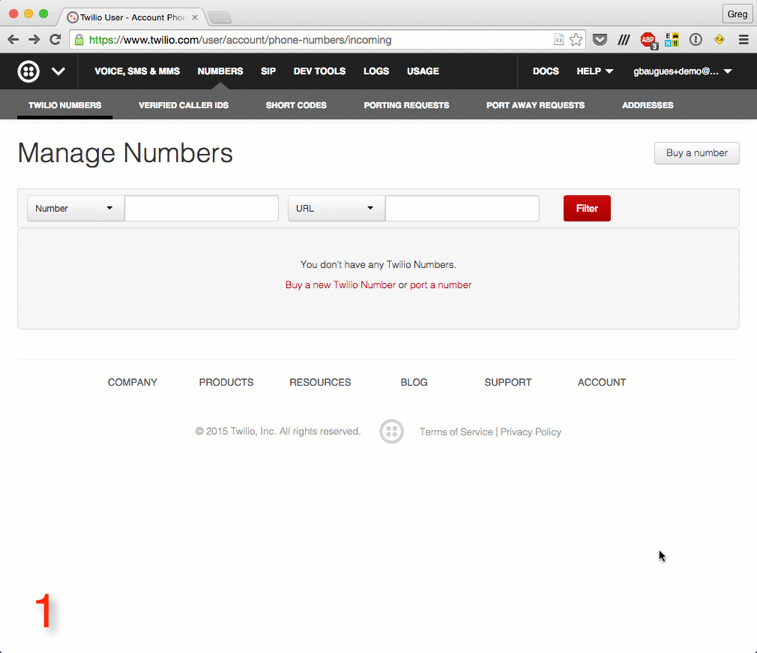
Now let’s initialize an app. Navigate to the directory you want to start this project in and run:
This will generate a package.json
file that will contain all of the information about our project. You can totally breeze through the prompts if you are not interested in customizing this information. With that taken care of we can get to installing the dependencies that we’ll need for our Node app.
Let’s grab the Twilio (3.x) Node module:
When we send out phone calls we will need to provide a URL for Twilio to send HTTP requests to when progress events occur. We will build a server side application with the Express web framework for Node to do this:
In order to parse the requests that we receive from Twilio we will need to install the BodyParser middleware for Express:
Receiving call progress events with Express
Now we are ready to jump into some code. In order to receive updates from Twilio on the status of our phone calls we need to set up an Express server for handling these requests.
When the state of one of our phone calls is updated, we will receive a request similar to this one with these POST parameters:
Let’s get started with a barebones Express app to handle this request and log the results to the console. Fire up your favorite text editor, open a file called index.js
and try the following code:
Before we can test to see if we can log the state of a phone call we first need to have a phone call to log. So let’s add another route to our server that will generate some TwiML to tell our phone call how to proceed. Let’s just have it say “Call progress events are rad” in a robot voice. Open up your index.js
file again and add another route, this time using the Twilio Node library:
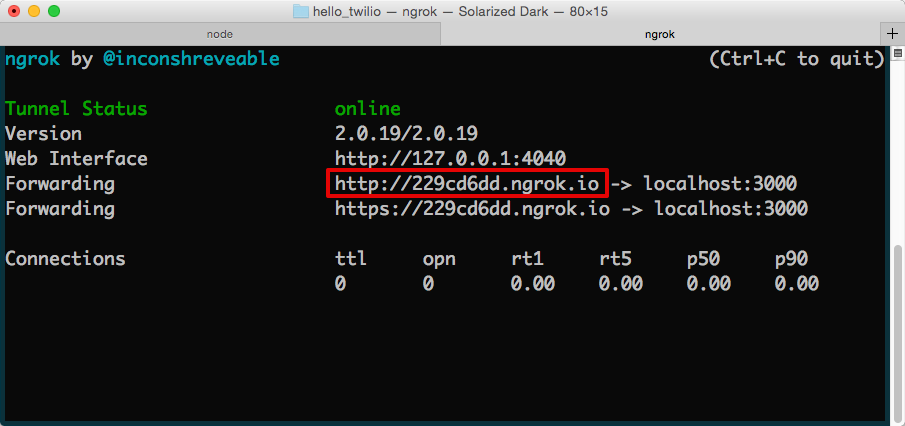
We’re almost ready to see call progress events in action, but first we need to set a status callback URL in the settings for your Twilio number. Head to your account dashboard and locate the settings for the phone number you want to use.
Using your new ngrok URL to access your Express server, make sure you set your voice request url to point to your “/voice” route and your status callback to send requests to your “/events” route. Now Twilio will make an HTTP request to “/voice” whenever you receive a phone call, and will send a request to “/events” whenever a voice call is completed.
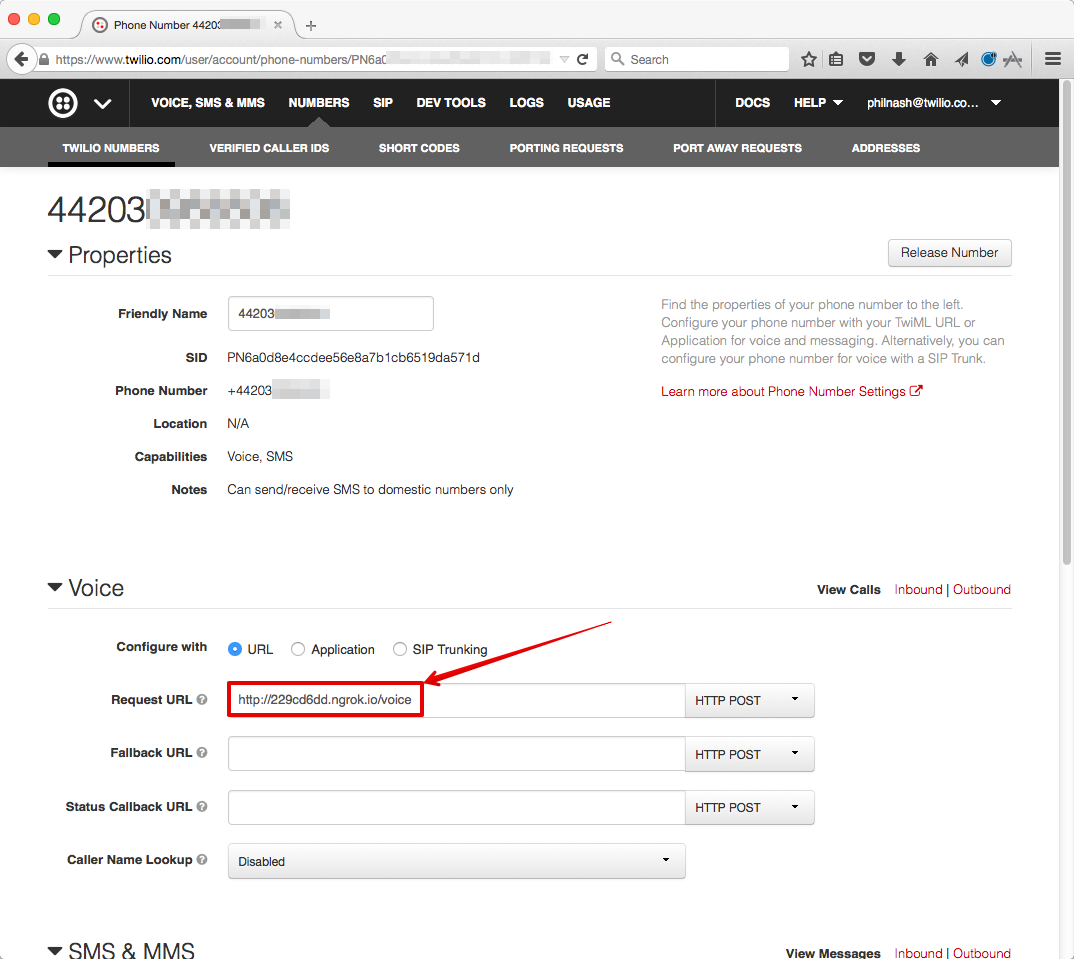
Now run your server:
And now we can make a phone call to our new Twilio number and see our console print the data we receive when the call is completed:
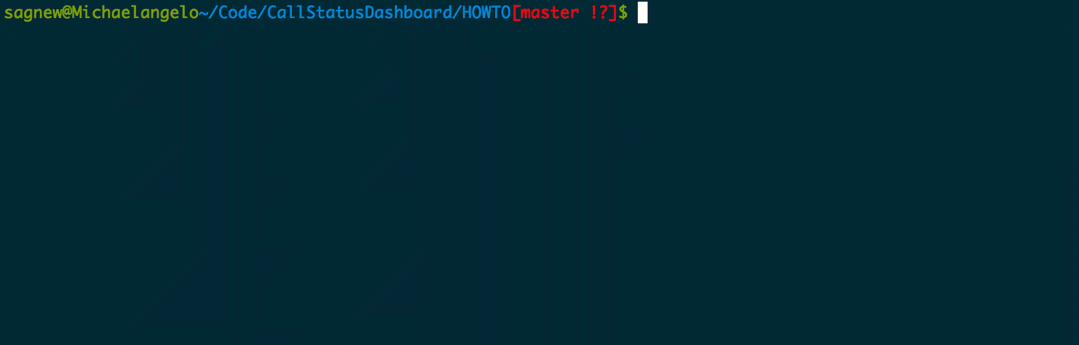
Making outgoing calls
That was awesome but this feature has existed for a while. What’s cool about the new call progress events is that we can now specify which events we want to listen for whenever we make a voice call with the REST API. Instead of just knowing when a call is completed, we can tell when it is ringing or when someone answers the phone.
Let’s demonstrate this by throwing a quick Node script together to make outgoing calls using the Twilio REST API that will use our Express app to monitor their status. Open up a new file called makeCall.js
and try this code out:
This is all the code we need to make phone calls, but we still need to authenticate with Twilio. Head over to your Twilio account dashboard and grab your Account SID and Auth Token. Store them in environment variables like so:
No need to add these to your code, because upon initialization of the REST client object, the Twilio Node module checks to see if these environment variables exist. Don’t forget to replace the values in that code before you run it. Replace the url
arguments with the same ones you used on your Twilio dashboard. Head to your terminal again and run:
Now with your index.js
server running again, try opening another terminal window and running our code to send a phone call to yourself:
Watch as your server receives the status of the call as it changes:
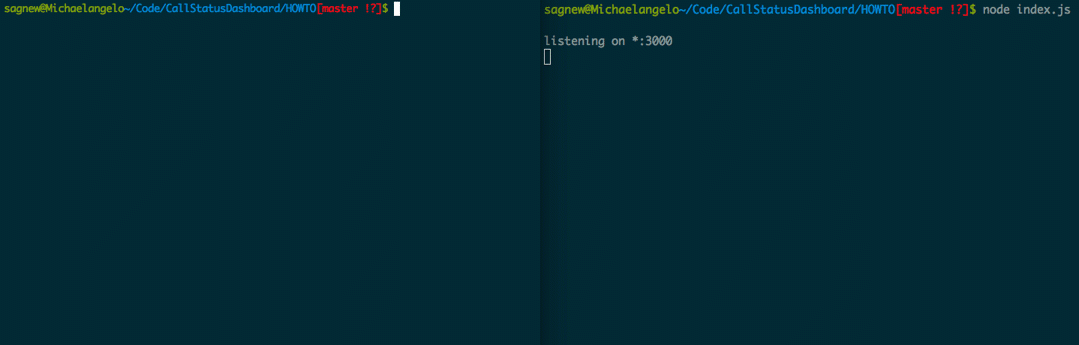
Moving forward
You’re now equipped to begin tracking the status of calls that you make with the Twilio REST API. Once you receive the request containing the call status, you can do whatever you want with that information. With some modifications, the code that we wrote in this post could act as a back end to a dashboard that monitors voice calls in real time. Some next steps could be:
- Build a dashboard with ReactJS to display the state of your phone calls.
- Use the information in a call progress event to modify the state of that call
I’d love to see what you build with this. Feel free to reach out if you have any questions or if you want to show off how you used call progress events in your Twilio app.
- Email: sagnew@twilio.com
- Twitter: @Sagnewshreds
- Github: Sagnew
- Twitch (streaming live code): Sagnewshreds
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.