12 Hacks of Christmas – Day 3: Santa CLI
Time to read: 7 minutes
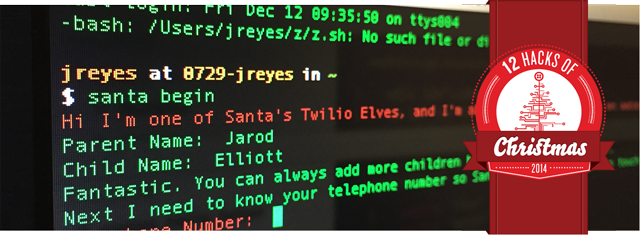
Hi folks! Today we on the devangel crew wanted to sprinkle the 12 Hacks of Christmas with some holiday cheer. The following hack started out as a Santa location hack. Then we found out that there is no such thing as a ‘Santa API’, which means first we’re going to have build that API and then maybe next year we’ll build a tool for tracking Santa in real-time via Twilio.
Instead Jarod, one of the parents on our team, put together a fun little command-line tool for sending secret messages to festive little recipients. Let us know what you think on Twitter with the hashtag #12HacksOfChristmas.
Santa’s Helper – Command Line tool using Ruby-gem, Thor and Twilio
Growing up in my house during Christmas was a delight. Every year we’d put out a tray of cookies on Christmas Eve, hang the stockings and try to sleep quietly upstairs as Santa visited our house and delivered gifts. It has always been a special night but now that I am a new parent I appreciate the magic of Santa even more.
When I began thinking about how parents like myself might want to interact with the North Pole I started by making a list of requirements and concerns:
- It needed to seem magical (simply texting a phone number might not be magical)
- It needed to be hard to find for a child (ie; not a website)
- It shouldn’t be a phone-to-phone hack (since we don’t want Mom’s phone chiming when little johnny thinks he is talking to the North Pole).
- It needs to be extendable by the community
In the end I decided the best implementation would be a ruby-gem command-line tool where hacker parents could interact discreetly with the North Pole. This would allow for magical experiences, is pretty hard for a child to stumble upon and gives us a tool that is easily extendable by the community. Luckily I had been looking for an excuse to build something using the Thor gem… and a command line interface to Santa’s helpers seemed the perfect console for creating magic :)
In this post I’ll show you how to build the SantaCLI from start to finish, but thats not my real goal. The real goal of this post is to equip you with enough information to inspire you to build an experience customized for your own family. So check out the code and start tweaking!
Demo:
Text +1 860-947-HOHO(4646) to ping the North Pole and see what Santa is up to.
The Code:
santas_twilio_helper on github
santas_twilio_helper on ruby-gems
Twilio: Ruby helper library, REST API, Twilio Number, Thor
Sign Up for Twilio
The North Pole is listening: command list
When I sat down to create my command line interface to the North Pole I wanted to make sure it was a two way channel. I wanted to make sure the elves had a way to communicate with my kids while also giving me a way of sending messages as an ambassador of Santa. I also wanted to give all of you hacker Moms and Dads a foundation that you could build on. Here’s the list of commands I came up with:
$ santa begin
: this command should register you as a parent, your children’s names and your zip code. This gives you and the elves access to certain details (variables) down the road.$ santa ping
: ping the North Pole which should send a random message status (SMS) of what Santa is up to. This could be extended to give location updates on Christmas Day, or to send MMS pics of Santa selfies.$ santa telegraph
: this allows mom or dad to send a message as one of Santa’s helpers. This should have an option for a time delay.
Great, now let me show you how I built this beauty.
Before we move on, feel free to grab the code from github and follow along: https://github.com/jarodreyes/santas-twilio-helper
Creating a Ruby gem that used Twilio
Feel free to skip this section if you just want to install my santa gem and play around with it, for the rest of us, here is a quick rundown of how I chose to create the gem.
There are a few ways to create a ruby gem, including by following the ruby-gem docs. I decided to use Bundler to bundle up the gem. The biggest benefit to using Bundler is that it can generate all of the files needed for a gem and with a few TODO
notes to help you fill it out. Additionally you get some handy rake commands for building and releasing the gem when you’re finished.
First you need to install Bundler:
Next you can generate the gem:
Next you can open the directory you just created and fill out all of the TODOs in the .gemspec
file.
Finally you need to make sure Twilio and Thor are available in your gem. This means requiring them in two places; the .gemspec
and your main application. First you should add them to the .gemspec file:
Eventually you’ll need to require them in the application.rb file but that’s getting ahead of ourselves.
In the santas_twilio_helper
gem this has all been done for you so you can just install the gem now and build on top of it.
Putting the fruit in the fruitcake: building the CLI using Thor.
Thor is a nifty little library. By including it we get a lot of built-in functionality that will make our minimal code act like a full fledged CLI. To give you an example of what I mean type this into the ‘santas_twilio_helper.rb’ file:
Now because we are using Bundler we can execute the command line tool using the bundle command:
That’s all it takes to create a CLI using Thor, a set of descriptions and methods that execute code in the console.
If you’ve installed the santas_twilio_helper
gem you can see a bit of Thor’s magic by typing santa help
:
Using Thor
The simplest way to use Thor is by creating some commands within a Thor class that are defined by their description. However, Thor also includes Actions, which are modules that interact with our local environment to give us some cool functionality.
To illustrate this example here is the begin
command that intakes some input from the console and writes it to a file.
Right away you can see some cool Thor functionality, by using the methods say and ask Thor will pause the shell session to prompt the user for some feedback, wait for a response and then store the response to a variable that our script can reference.
I mentioned earlier that Thor includes Actions which allow us to save input to disk among other things. One of these Actions is create_file() which I use in the function below, to save the user input from begin to the filesystem.
I chose to write the data to a santarc
file as opposed to writing to db. If you would like a more secure data store you should probably change this.
Next let’s take a look at the ping command since I believe this command has a ton of potential ways it could be extended to do amazing things.
This command is pretty straight-forward as it stands, but you may want to extend it based on the TODO messages in the comments. For now it reads messages from the ‘messages.json’ file and pulls a random message. Then we call sendMessage() which actually does the job of sending the SMS with Twilio.
Before we can send a message in Ruby using Twilio all we need to do is add ‘require twilio-ruby’ to the top of application.rb
This version of sendMessage() is the simplest way we can send an SMS using Twilio. To send a picture you would simply need to add a :media_url parameter that would point to a url of some media. However on github, you will find a more robust version of sendMessage() that actually appends a greeting and a sign-off from the elves.
I’ll quickly mention the ‘telegraph’ command which allows me to send a message as Santa’s Helper with a time delay (in seconds). This means I can create scenarios where I am in the kitchen cooking with the family when the ‘Santa Phone’ mysteriously delivers a custom message. Pretty sneaky ;) Try it yourself (assuming you installed the gem) with the command:
The last bit of know-how I picked up on this journey to a gem was how to build and release the gem.
Building and releasing the ruby gem.
Before we can use the handy Rake tasks we need to save your ruby-gem credentials to your .gem file. So run:
Once we have done this we can run:
Grok’in around the christmas tree
So now we have a working CLI to interact with the North Pole, but there are a few things I would challenge you all to do. First this CLI could use a location specific hack. Since neither Google or Norad(Bing) offer an api for Santa’s location this is going to require some work on your end. But if you want to collaborate on a Santa API hit me up and we’ll get started.
I would also recommend adding a Christmas day switch to the ‘ping’ command that maybe kicks off an hourly update via SMS of where Santa is located.
Hopefully learning how I built this tool has given you a plethora ideas of how to make it even more magical. I look forward to all of the pull requests on github, but until then feel free to ping me on twitter or email and we can talk about how The Santa Clause movie with Tim Allen is actually a cultural gem.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.