Send Task-Related SMS Notifications in Asana Using Twilio SMS, Automate.io and Laravel
Time to read: 5 minutes
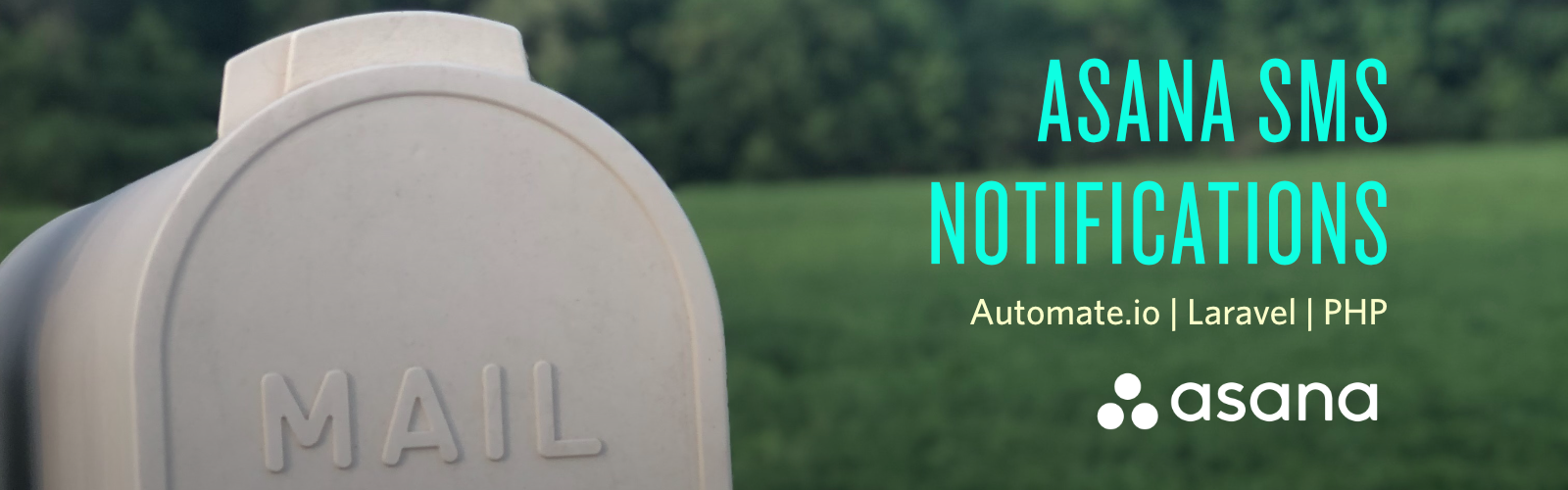
Introduction
Currently, the only way to get Asana project notifications is either by email or from within their app. With limited push notification support, your team members are likely to get overrun by email alerts sent and miss important project updates. Any developer or project manager knows that missed notifications can result in missed deadlines which turns into broken confidence of clients. Therefore, it is important to figure out alternative ways of sending out important notifications.
In this tutorial, we are going to discuss how to send SMS notifications to project members when an Asana task is created, completed or comment on.
Requirements
- Twilio account
- Asana account
- Automate.io account
- Composer globally installed
- Ngrok
- PHP development environment with Laravel
Set Up Laravel Project
If you don't have one already installed, you’ll need to install a fresh Laravel application. A guide on how to set up Laravel can be found in the official Laravel documentation.
We also need to install the Twilio SDK for PHP in our project. In your terminal, navigate to the project directory and run the following command:
Create an Automate.io Asana Connect App
To grant automate.io secure access to our Asana account, we need to first create an Asana Connect app. Follow the steps below to create an app:
- Login into your Automate.io account and click on APPS menu. A new page is loaded.
- Click Add (+) button.
- Select Asana from the list. Opens a pop-up window.
- Enter a unique name.
- Click Authorize to create the app.
- Click Save.
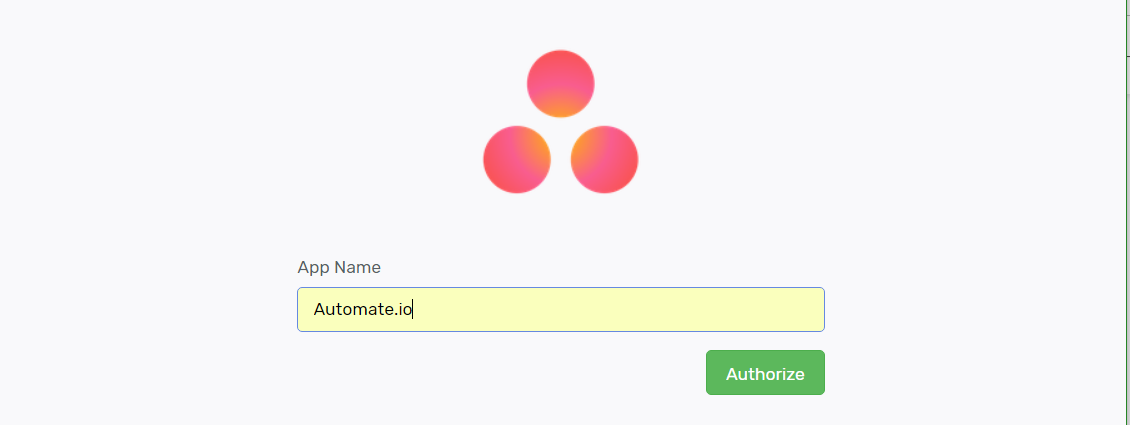
Create a Temporary Public URL
To test locally, we need DNS software like ngrok that assigns a local port a publicly accessible URL over the Internet. Once ngrok is set up, run the command below to expose our local web server running on port 8000 to the Internet.
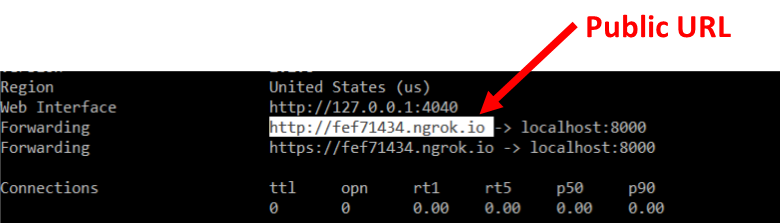
Create Bots in Automate.io
The next step is to create bots in Automate.io that will be triggered by task-related events in Asana.
Follow the steps below to create the bots:
Create a Task Bot to Listen To Asana Events
First, we’ll set up the listener for the bot:
- Navigate to the BOTS menu and click on the “Create a Bot” button.
- Click the Select Trigger App dropdown and select the Asana app we created earlier.
- From the secondary dropdown, select “New Task” as the trigger event.
- Then, select the project you want to use from the dropdown.
Now we’ll define the action from the trigger:
- In the select panel, click “Select Action app”.
- Select “Webhooks” from the dropdown followed by “Post data”
- Enter the Webhook URL (your ngrok URL) followed by
/api/notifications
and the content type as JSON. - Now drag and drop the Task Assignee Name and Task Id output fields from the first panel into the Data field.
- Create the JSON data as shown in the picture below.
- Click Save and then activate the Bot.
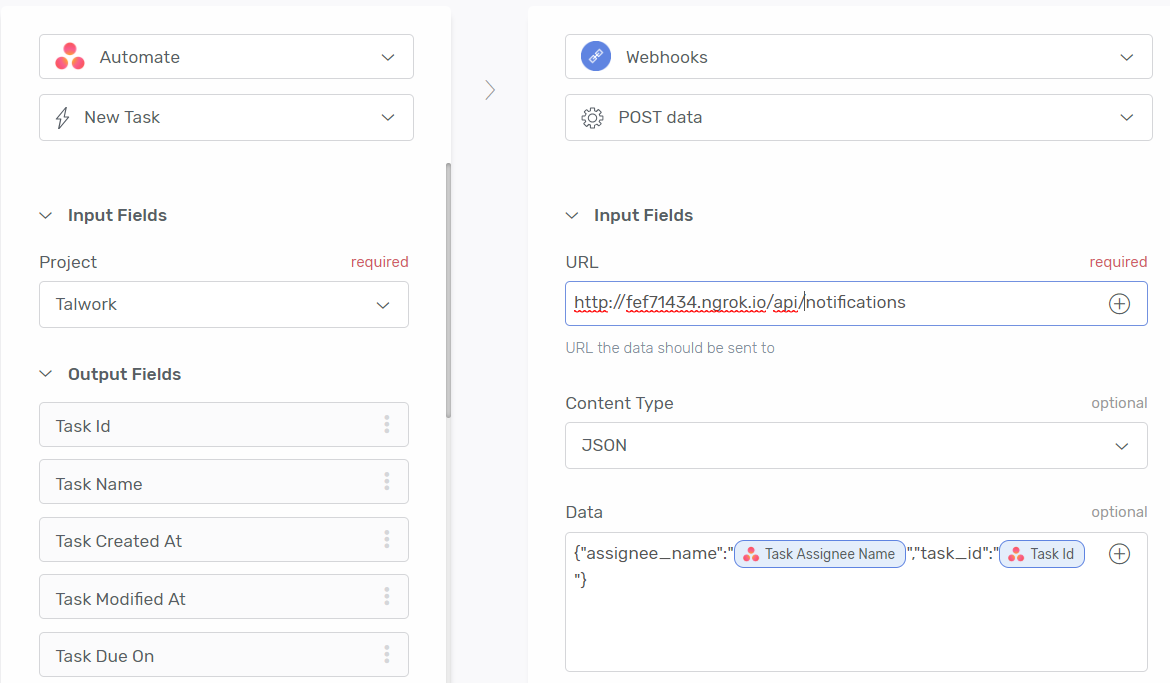
Create New Comment On Task Bot
This next bot will be responsible for sending an SMS when someone comments on a task:
- Create a new bot and select the Select Trigger App dropdown. Choose the Asana app.
- Select “New Comment On Task” as the trigger event.
- Now select the project you want to use from the dropdown.
We’ll need to capture some information about the task so let’s add another trigger:
- In the second panel, click Select Action app.
- Select the Asana app.
- Select Find Task as the trigger event.
- Select Workspace followed by Project.
- Select Task Id from the variable list in the Task Id Field.
Now we’ll define the action from the triggers:
- In the third panel, click Select Action app.
- Select “Webhooks” from the dropdown followed by “Post data”.
- Enter the Webhook URL (your ngrok URL) followed by
/api/notifications
and the content type as JSON. - Drag and drop Comment Created By Name, Comment Text and Task Name output fields and drop them in the Data field.
- Create the JSON data as shown in the picture below.
- Click Save and then activate the Bot.
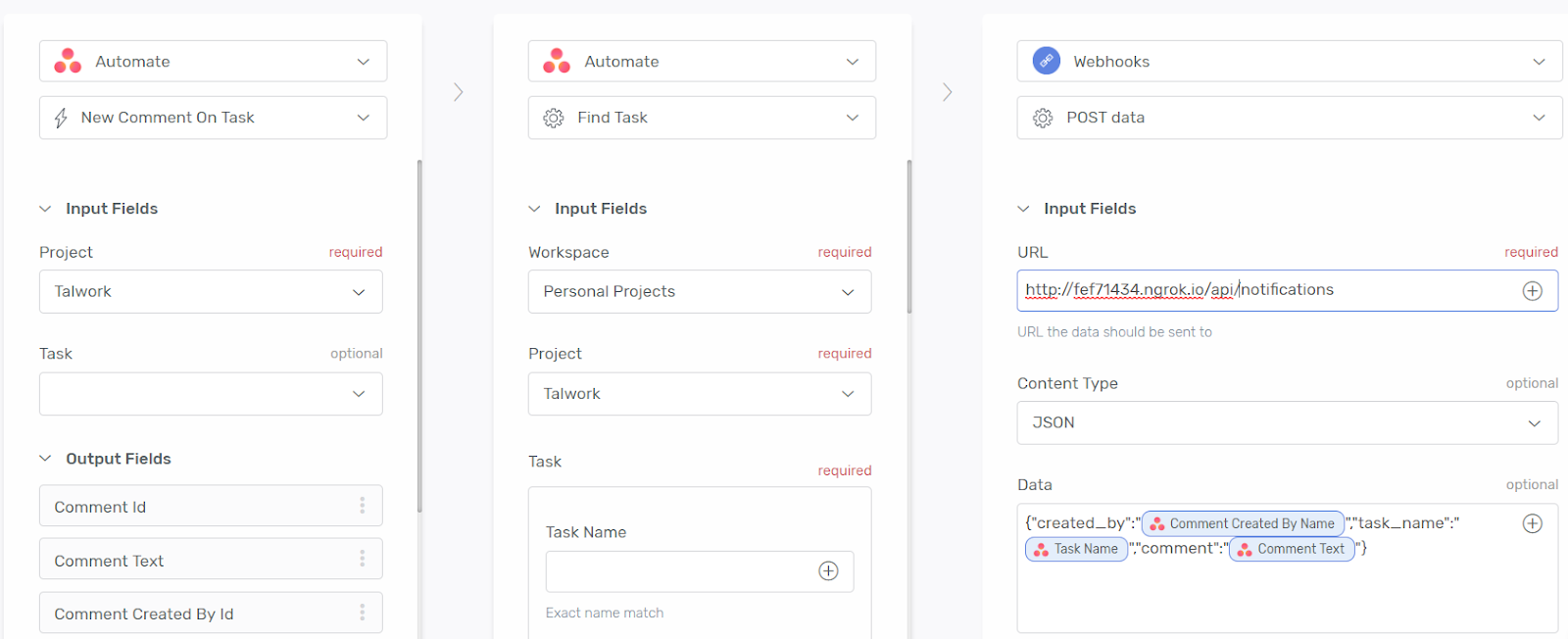
Create Task Completed Bot
The last bot we’ll create will notify us via SMS when a task is marked as completed:
- Create a new bot and select the Select Trigger App dropdown. Choose the Asana app.
- Select “Task Completed” as the trigger event.
- Now select the project you want to use from the dropdown.
Now we’ll define the action from the trigger:
- In the second panel, click Select Action app.
- Select “Webhooks” from the dropdown followed by “Post data”.
- Enter the Webhook URL (your ngrok URL) followed by
/api/notifications
and the content type as JSON. - Drag and drop the Task Completed, Task Completed At and Task Name output fields from the first panel into the Data field.
- Create the JSON data as shown in the picture below.
- Click Save and then activate the Bot.
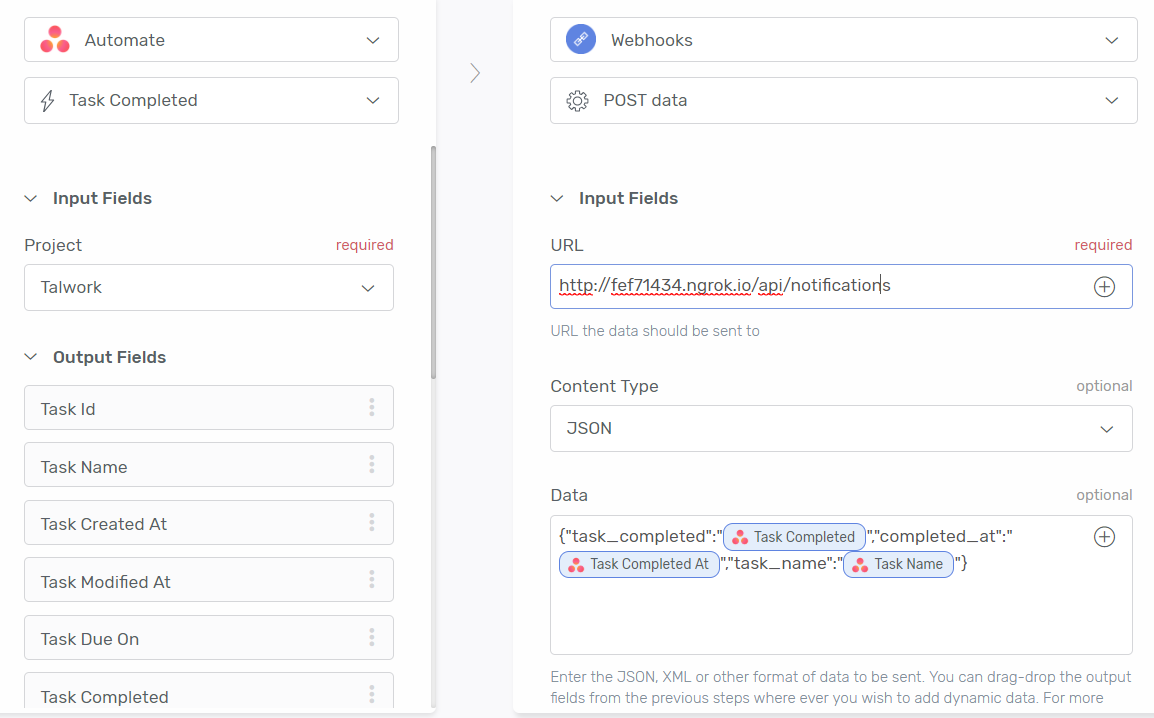
Purchase an SMS Enabled Twilio Phone Number
- Login into your Twilio account and go to the console numbers page.
- Click the plus button to purchase a phone number if you don't have one already.
You'll use this phone number as the "From" phone number when sending notifications to project members.
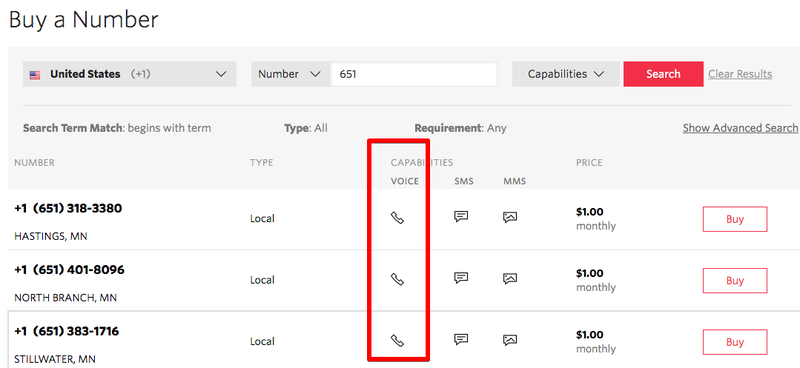
Remember to also retrieve your account credentials; specifically the Account SID and Auth Token.
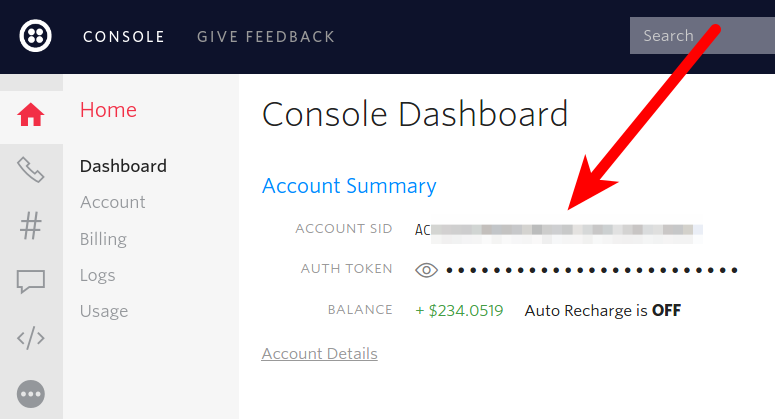
Update the Environmental File with Account SID, Token and Phone Number
Open the .env file and add the following code:
These variables should be assigned the credentials retrieved from your Twilio account in the step above.
Create a Webhook to Handle Messages from Automate.io
Finally, let's create the webhook we need to send notifications.
Run the following command in the project directory to create AsanaController
:
Open the file app/Http/Controllers/API/AsanaController.php
and add the following code:
Create The Endpoint
Inside routes/api.php
, add this line of code:
Test the App
Let's test our application. Add your phone number to the $numbers
array and
login into your Asana account and create a task.
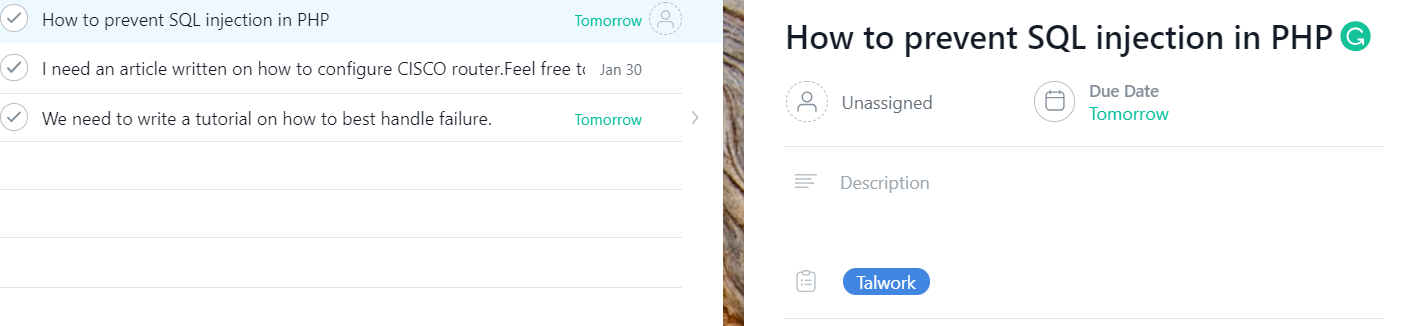
You should immediately get a notification through SMS.
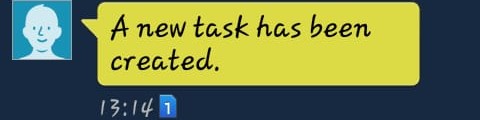
Next, comment on the task we just created above.
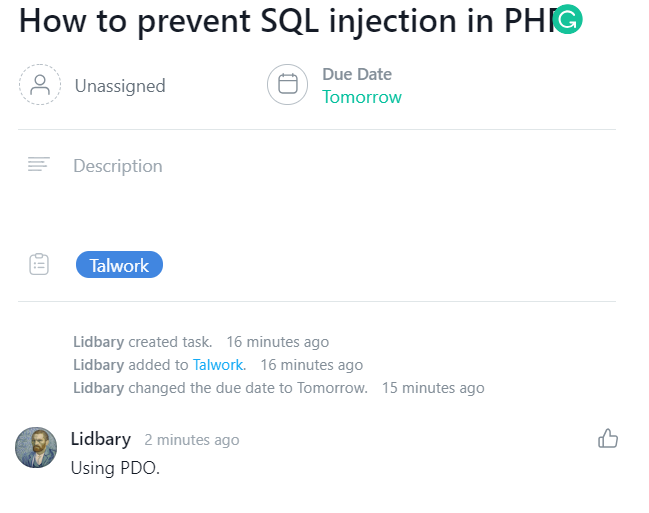
You should get an SMS similar to the one shown below.
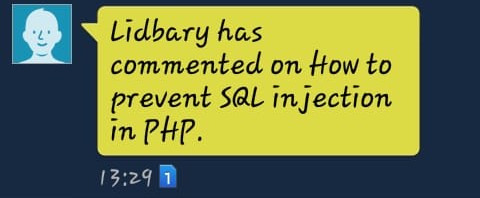
Lastly, mark the task as completed in Asana to get the task completed notification.
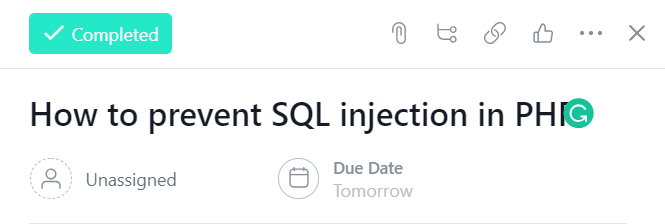
You should get an SMS notification on this too.
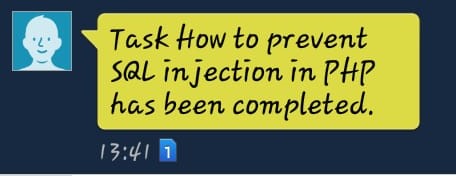
Conclusion
Asana API has a lot more features. Task-related APIs and webhooks covered in this tutorial are just a part of it. To get more details on Asana API, check out their documentation.
The complete code for the tutorial can be found on Github.
You can reach me at info@talwork.net or on Twitter at @mikelidbary.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.