Twilio and Parse Collaborate on New and Improved Cloud Code Module
Time to read: 5 minutes
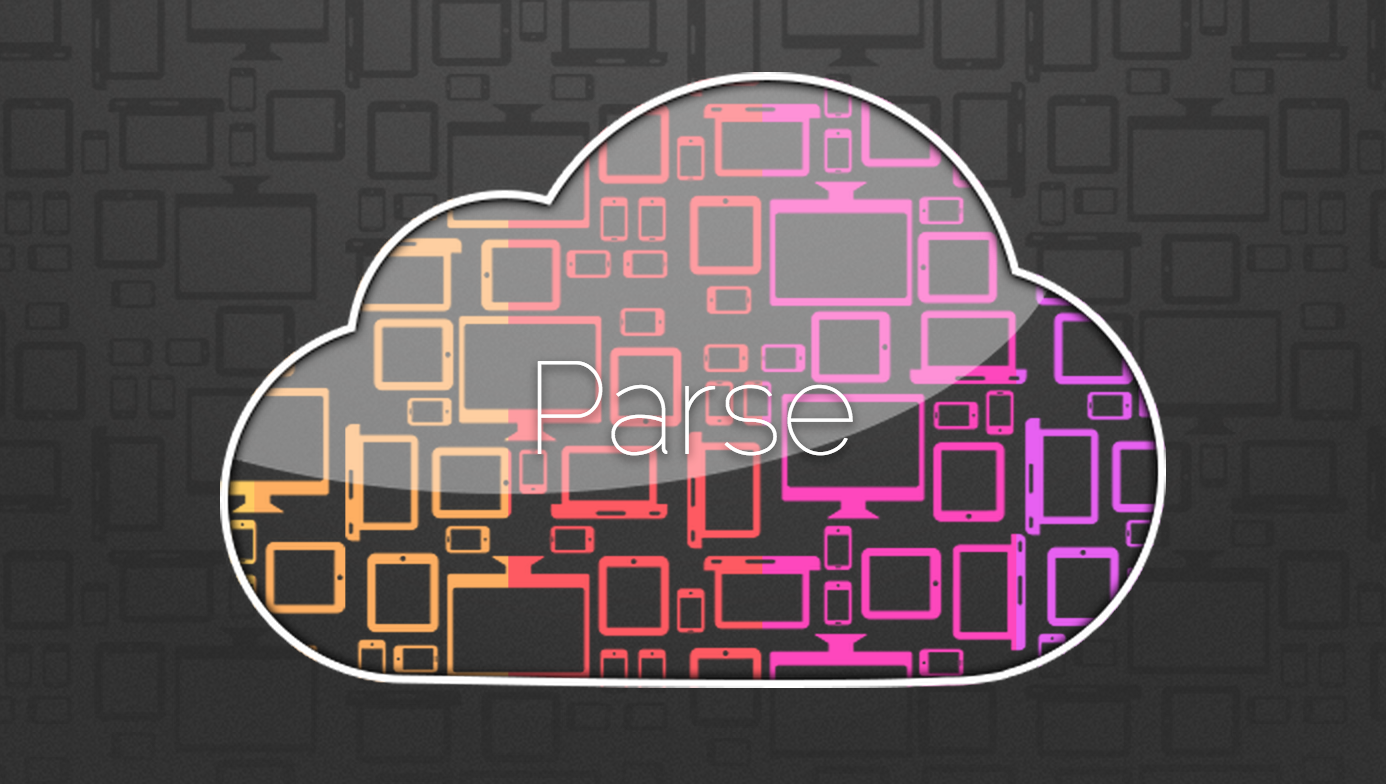
Hi there! Are you new to Twilio? Sign up for an account – it’s free to get started, and will let you follow along with the code example to follow.
Twilio and Parse are on similar paths when it comes to empowering developers. We both provide high-level application building blocks that allow developers to focus on what makes their app unique. To better serve both of our developer communities, we’ve worked together to create a new, full-fledged Twilio helper library for Parse Cloud Code.
The first version of the Twilio Cloud Code module let developers send SMS text messages. The latest version, available today in Parse’s Cloud Code environment, brings all the power of the Twilio platform to Parse, including TwiML, VoIP, and the entire REST API. It is a port of our official node.js helper library, but is also 100% backward compatible with the older version of the Cloud Code module. Combined with Parse’s new support for dynamic web applications, there’s no communications app you can’t build with Twilio and Parse Cloud Code.
To help you get started, we’ll provide a quick tutorial using the Parse JavaScript SDK along with Cloud Code. The same techniques will work with Android, iOS, Xamarin, Unity, and all other platforms supported by Parse.
Getting Started
To start building a Cloud Code app with Twilio, you’ll first need to create a new Parse app or use an existing one. Once your app is created, go to the settings tab. Under the “web hosting” section, specify a unique subdomain for your app:
This will give your Parse Cloud Code app a public home on the internets. If you entered “chunky-danger-monkey” in this field, your web app will eventually be available on “https://chunky-danger-monkey.parseapp.com”.
Next, you will need to install the CLI tools for Parse Cloud Code. This will allow you to generate a Parse Cloud Code project to deploy. In a terminal window, enter the following command, inserting your app name in place of “chunky-danger-monkey”:
parse new chunky-danger-monkey
First, you will be prompted to log in with your Parse account credentials. Then, you will need to choose your Parse app from a list of all your apps. Once you have completed these steps, there will be a “chunky-danger-monkey” directory with your new Cloud Code app inside it.
Responding to Inbound Calls with Cloud Code
Let’s start by using Cloud Code to respond to inbound calls to a Twilio number. Enter your Parse app’s directory, and open the file “cloud/main.js”. This is the entry point for your Parse Cloud Code application, and the place where we’ll start doing some fun Twilio stuff!
Let’s create a simple web application that serves TwiML. Replace the contents of main.js with the following:
To deploy your cloud code, go to your app’s main directory a terminal window – the “chunky-danger-monkey” directory we created earlier with the “parse new” command. Enter the following command:
Which should deploy your code to Parse’s cloud, and start your Express web application. In your web browser, visit “https://[your-app-name].parseapp.com/hello” – you should see an XML document containing the TwiML instructions we just created.
Now, let’s hook that up to a Twilio number! Navigate to your Twilio numbers in the browser, and choose or buy one to work with. In your number configuration, replace the default Voice URL with your Parse app’s TwiML URL. Don’t forget to switch the request type to a GET!
Now, give your Twilio number a call. You should hear the message we configured in our TwiML!
Making Outbound Calls From Cloud Code
So we can now respond to inbound calls using Parse, but how do we initiate outbound calls? Making calls, sending text messages, and accessing other data with the REST API requires that we use the REST Client provided in the Twilio helper library for Parse Cloud code. Full docs for the RestClient object can be found in our node.js docs.
To demonstrate this functionality, let’s use the remote procedure call (RPC) functionality baked into Cloud Code. Back in “cloud/main.js” in your app directory, add the following code under the call to “app.listen()” at the very bottom of the file. Make sure to insert your Twilio Account SID and auth token as shown. You will also need to change the “URL” parameter to your Parse app’s URL, and the “from” phone number to be a Twilio number you control:
This will define a cloud function, create a Twilio REST API client using your account credentials, and respond to the client with the outcome of the REST API call.
Now, we need to create a client to actually consume this service. In your cloud code app directory, open “public/index.html” and replace it with the following HTML and JavaScript code, replacing your Parse App ID and JavaScript Key as shown:
This will create a simple UI that will allow you to enter a phone number. When the button is clicked, your Parse Cloud Code function on the server will be executed, and the phone number you entered will receive a call!
Wrapping Up
With the new Twilio Cloud Module for Parse, you can build full-featured communications applications in the cloud. Call centers, SMS notification systems, VoIP clients – all of these applications are now possible with no server configuration or management at all.
The Twilio Cloud module is a port of the Twilio node.js module, so the most complete API reference can be found here. Parse also provides some basic documentation on the module on their web site. Their documentation on getting started with Cloud Code can be found here.
With the Twilio helper for cloud code, writing native mobile apps with VoIP functionality just got a whole lot easier as well. Check back on the dev blog soon for more on how to use Parse and Twilio in iOS and Android apps.
Happy cloud coding!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.