Hacking Halloween: Using Arduino and Twilio To Build An Interactive Haunted House
Time to read: 5 minutes
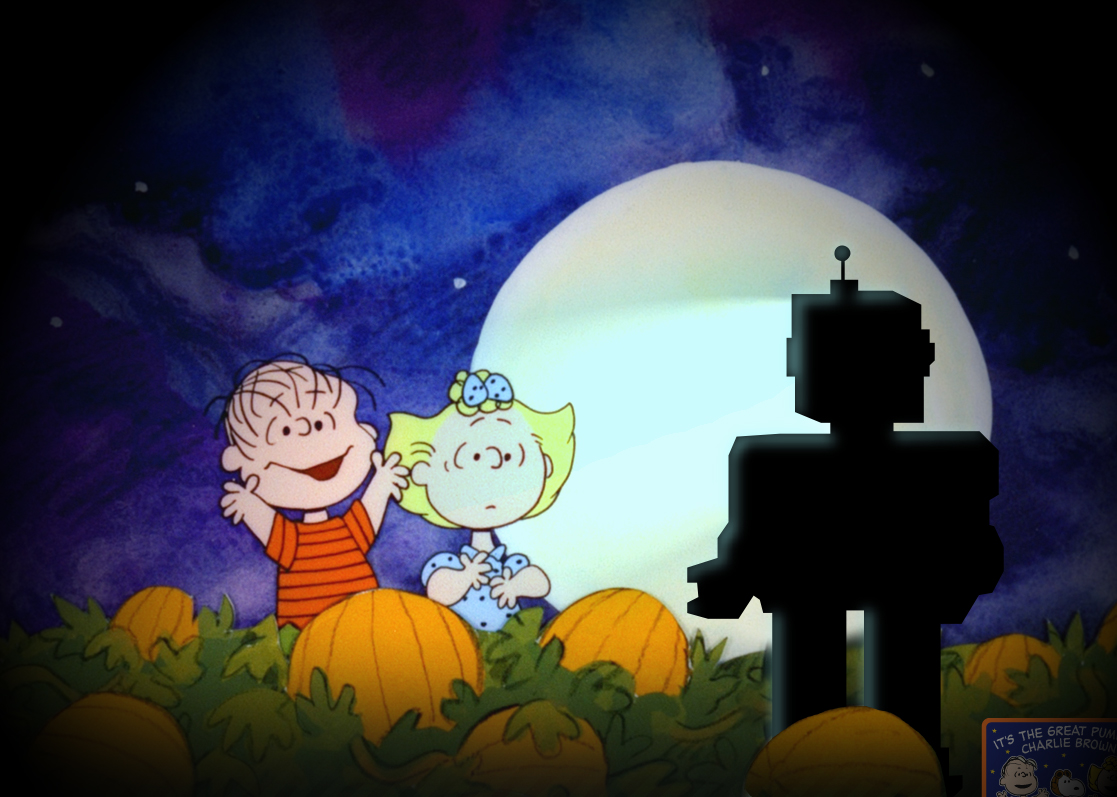
Talk about this post on twitter: #hauntedHack
When I was a young trick-or-treater I never missed the house on the hill that gave full-size candy bars; that was the Halloween destination on our street. But these days setting up the ideal trick-or-treating destination requires thinking outside the Snickers bar. I want kids to remember our house. I want parents to tell other parents about it. But more importantly I want a “surprise factor” when people discover our house. So this year I amped up the “trick” and put together an interactive haunted house using Twilio, Arduino and some cheap electronic components.
Haunted Hack: Pumpkins That Change Color When You Text Them
My initial goal was simple. When people text a color to my app I wanted the pumpkins in our lawn to change colors.
Here’s a video of the final product.
First my wife texts “red” to the Twilio number, which turns the pumpkins red. Then she texts the word “chaos”, which unleashes a world of terror. Can’t wait to see how the trick-or-treaters react when their parents are texting chaos as they walk up! Let’s walk through how I built these interactive pumpkins using Arduino and Twilio.
Here’s what you’ll need to make this happen:
- Arduino Uno
- Arduino Ethernet Shield
- 2 x 12V DC power supply
- M/M Jumper Wires
- 3 1k resistors
- 3 PNP Mosfets
- LED Strip Lights
- Foam Pumpkins
Extras:
- Fog Machine
- Speakers
- Bats
- Other spooky business
This circuit is based off of this make guide tutorial and is relatively simple. The only trick here is that we are using MOSFETs (a type of transistor) in order to amplify the voltage going to the LEDs. Because the LEDs require more than the 5V the arduino puts out, we have to use MOSFETs to amplify the power. The way it works is the Arduino sends a digital signal (PWM) to the MOSFET through the (G) gate pin. The signal switches the transistor passing the higher current at 12 V, gathered through the (S) source, out through the (D) drain to the LED strip. This is a smart way of amplifying a small PWM signal to a device that requires a higher source of power.
Safety Warning!!
Remember whenever working with power to be extremely careful. Always build circuits with the power unplugged. The last thing we want is for you to fry your Arduino or yourself, leave the shocking to Dr. Frankenstein.
Once you’ve got the circuit looking like this, we should be ready to go on to Step 2.
The Arduino Software
Now let’s get the Arduino piece working. Before we look at the code let’s make sure you are all setup.
First you need to:
- Download the Arduino IDE
- Connect the Arduino to your computer
- Download then Install the ArduinoPusherLibrary
- Sign up for Pusher
- Locate your Pusher API key
Now that you’re all set to go, let’s set up a sketch to make the Arduino talk to Pusher and trigger some fun LED colors.
Once you have the Arduino app (IDE) open, create a new file and paste the following code into it.
Let’s walkthrough this sketch together. First we are including the two libraries we are going to need for this project; the Ethernet and Pusher libraries. Then all we are doing is setting up our three PWM pins to prepare them to manipulate our three LED strip leads. In this case we are using pins 5, 6 and 9. Pin 5 controls the RED signal, 6 is GREEN and 9 is BLUE.
Now that we have prepped the PINS to do their jobs, we can create complex colors by sending RGB values through each pin. Notice in the setup() function that we are writing different values to each pin. Pin 5 (RED) is sending 255, pin 6 (GREEN) is sending 102, and pin 9 (BLUE) is sending 0. This of course matches the RGB values for Orange.
Next we need to connect to our Pusher channel via the ethernet connection and setup up our handlers for the different events will trigger the pumpkins to change colors.
Paste this next snippet within the setup() function where I’ve indicated in the code.
Once our Pusher client has connected to the internet, it authorizes with our app key and subscribes to the channel that we will eventually be sending our events through. Then our client listens for certain event names, in this case colors, and binds those events to event handlers.
Sweet, we’re almost there. Now all we have to do is write the loop and the callback functions that will change the color. Here is the next piece of code.
This last piece is straightforward. Inside the loop we tell the Pusher client to constantly monitor the event channel.
Then we create the callback functions for the Pusher event listeners which change the color of the LED strip lights by pulsing new values through the RGB leads. This could be simplified by writing a helper function that takes an RGB array as an argument and loops through the pins. Since we only have a few colors this is fine for now.
Finally we can upload our sketch to the Arduino. You can find the full sketch here for reference.
Next we are going to write a simple Sinatra app and connect all the pieces to create some jacked-up Jack O Lanterns!
Step 3: The Sinatra App
The server piece of this haunted hack is a very basic Sinatra app that we will host on heroku. The first thing to do is set up basic configuration with two new files in your project directory; the Gemfile and the Procfile:
We’re just going to start with the whole Ruby file since it’s pretty simple. Now you can go ahead and create haunted-hack.rb and add the following code:
Let’s walk through this file. At the top we are setting up our Pusher configuration variables and including necessary gems. We will set up the environment variables later when we push to Heroku.
Next we set up our ‘/trick/’ route, which receives incoming SMS messages and triggers a Pusher event that the Arduino is already listening for. Once the event has been fired, we send a text back to the user using the Twiml.Message verb.
Lastly we throw up our root route which renders a simple index.erb file. Here is the index file.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.