Send SMS and MMS Messages with a Particle Photon
Time to read: 4 minutes
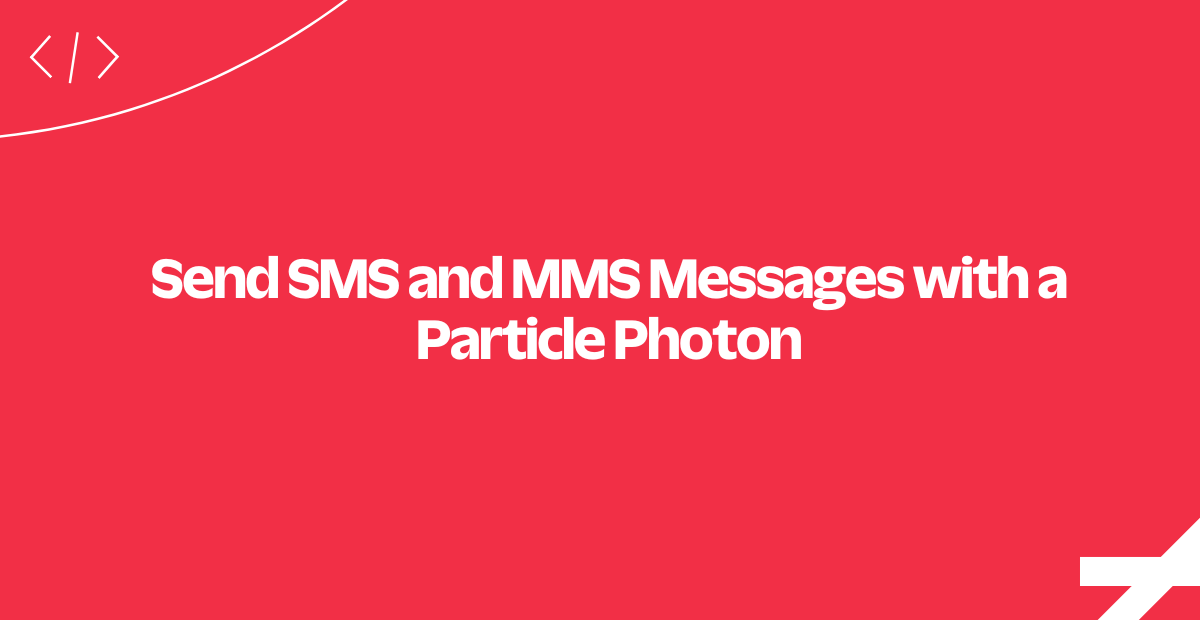
If you've got WiFi available, the Particle Photon is an amazing little device for building your Internet of Things... Thing. Measuring a mere 1.44" x .8", it still packs a powerful 120 MHz ARM Cortex M3 from STMicroelectronics onboard, as well as a WiFi device from Cypress Semiconductor. That sums to quick build cycles and a powerful IoT product base for not-a-lot of money.
Today we're going to show you how to get your Particle Photon sending MMSes and SMSes with Twilio. All you'll need is your Photon, a friendly WiFi network, and about 20 minutes of time.
Let's do it!
Sign Into or Sign Up For a Twilio Account
Create a new account with Twilio (click here for a free Twilio trial) or sign into your Twilio account.
Find or Purchase an SMS and/or MMS Capable Number
For this to work, you need to use an SMS (or SMS and MMS) enabled number.
- Enter the Twilio Console
- Select the Phone Numbers ('#' / hash tag) section on the left side
- Navigate to your current number inventory
Under 'Capabilities,' you will see the phone number capabilities. You'll need numbers with SMS or both SMS and MMS enabled:
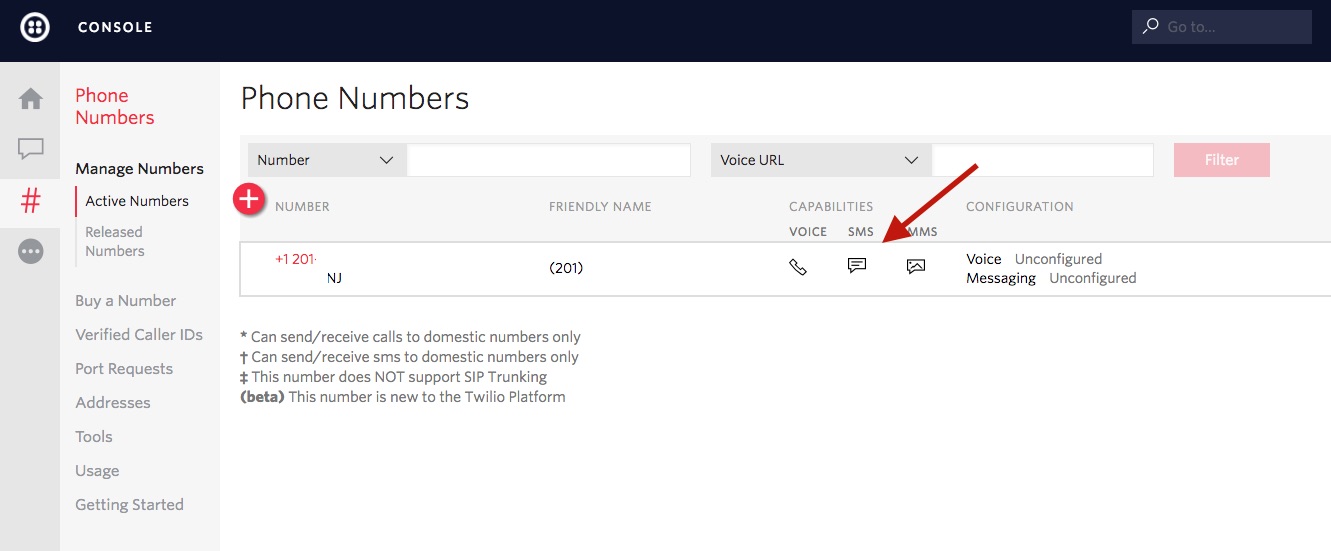
If you don't currently have a number with SMS or MMS capabilities, you can purchase one. After navigating to the 'Buy a Number' link, click the SMS and/or MMS checkbox:
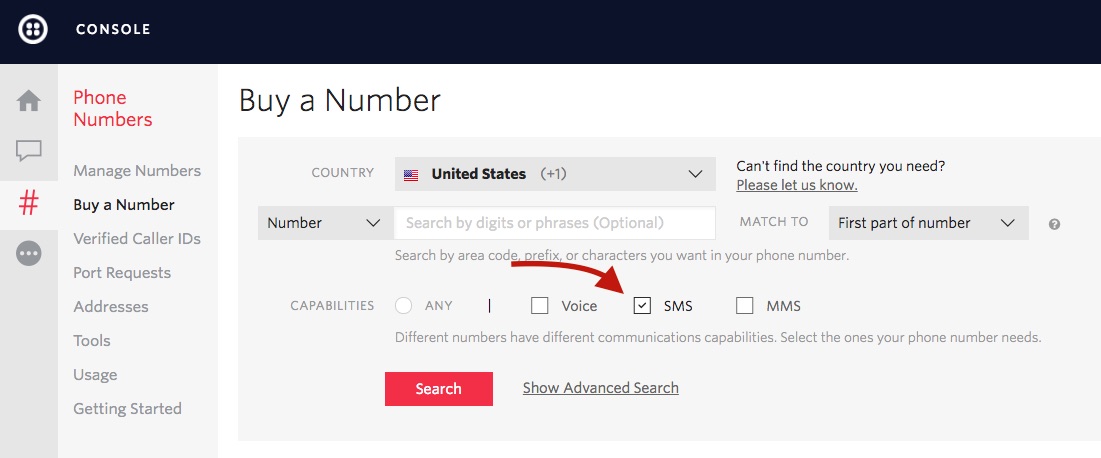
Save the number that you use in a browser tab, so it is readily available.
Retrieve Your Twilio Account SID and API Secret
In order to call the Twilio API from the Photon, you will need to authenticate. You need to have your Twilio Account SID and a Twilio Auth Token handy.
You can find these both in the Twilio Console:
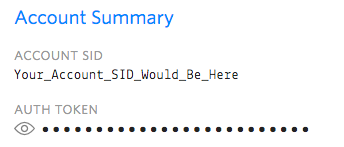
As before, keep these handy.
Prepare Your Particle Photon for Flashing
To flash your Particle Photon, you'll first need to ensure it's connected to your WiFi network.
If you haven't yet, please follow Particle's Getting Started guide to get your Photon set up.
Example 1: A Simple Example of Sending SMSes with a Particle Photon
Particle uses Webhooks (just like Twilio!) to trigger external APIs when events occur. When you publish an event to Particle's cloud, the webhook you create is then used to trigger whatever external event you'd like. That might mean your own server or business logic, but for today we're going to link our Particle Webhooks to Twilio.
Log into the Particle Web IDE with your account.
Copy Our Shared Particle Photon App
There are two ways to get the sample code for this application:
- Copy it directly from Particle Web IDE Shared Apps service
- Clone it from our Github Repo
That short snippet is all you need to send an SMS with the Photon.
Particle.publish("twilio_sms", body, PRIVATE);
This line of code is how we push an event to the cloud. We haven't yet added it (that's next!), but "twilio_sms
" is the event which will spawn a call to Twilio, while body
is a variable that gets passed along.
Flash the code to your Photon with the 'Flash' (Lightning) icon. You'll see the LEDs work, but you won't get a text yet.
Set Up a Particle Webhook
Now's the time - have your Twilio credentials and an SMS-capable phone number handy. We're going to go through some very simple steps to get our Particle Webhook working.
- Navigate to the the Particle Console's Webhook Integration screen
- Click the '+' 'Plus' button icon to create a new integration
- Select 'Webhook'
- Event Name:
twilio_sms
- URL:
https://api.twilio.com/2010-04-01/Accounts/{{ACXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX}}/Messages
(replacing "ACXXX....
" with your Twilio Account SID) - Request Type:
POST
- Device:
Any
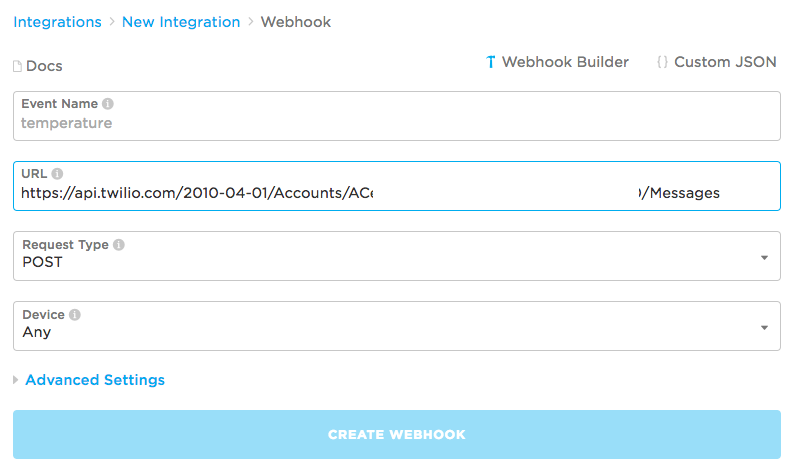
Next, we'll change some of the advanced options so we can give Twilio what it needs to send a text.
Change the Advanced Particle Webhook Options
Make the following changes after expanding the 'Advanced Options' section.
- Send Custom Data: Form
- Under Data, set the following three:
To - [[YOUR CELL PHONE NUMBER/NUMBER THAT CAN RECEIVE SMSES]]
From - [[YOUR TWILIO NUMBER FROM ABOVE]]
Body -{{PARTICLE_EVENT_VALUE}}
- In HTTP BASIC AUTH:
Username - [[Your Account SID]]
Password - [[Your API Secret Key]]
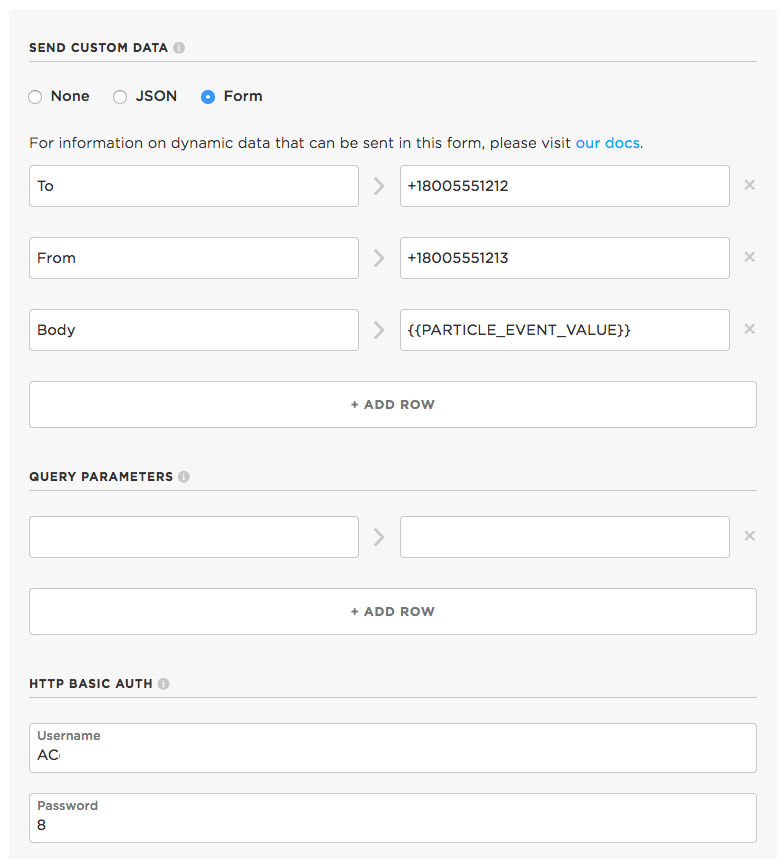
After saving, feel free to send a 'Test' message with the 'Test' button. Or, you know, just wait for the next step - you're all glued together and good to go!
Power Cycle the Photon
Hit the reset button on the Photon or power cycle it.
It should send you a text message (fingers crossed!). Nice!
Example 2: Adding MMS Support to a Particle Photon
The first example, by design, is very simple. In this example, we'll dial up the complexity by adding optional media messaging through MMSes to the app.
With properly formed JSON, Particle also allows you to pass multiple variables along. That's the mechanism we're going to use to build out MMS capability.
Copy Our Shared Photon MMS App
As before, there are two options to retrieve our code. You can:
Again, we've boiled down the code to the minimum. In your production application, you'll want to check on things like message size and text encoding, as well as add your business logic. You'll also need to consider special characters like double quotes.
Set Up Another Webhook
Name a new Webhook twilio_mms,
but otherwise, go through the same steps as the above 'twilio_sms' Webhook.
Also, you need to change 'Send Custom Data' for 'Form':
- To - [[YOUR CELL PHONE NUMBER/NUMBER THAT CAN RECEIVE AN MMS]]
- From - [[YOUR TWILIO NUMBER FROM ABOVE]]
- Body -
{{body}}
- MediaUrl -
{{image}}
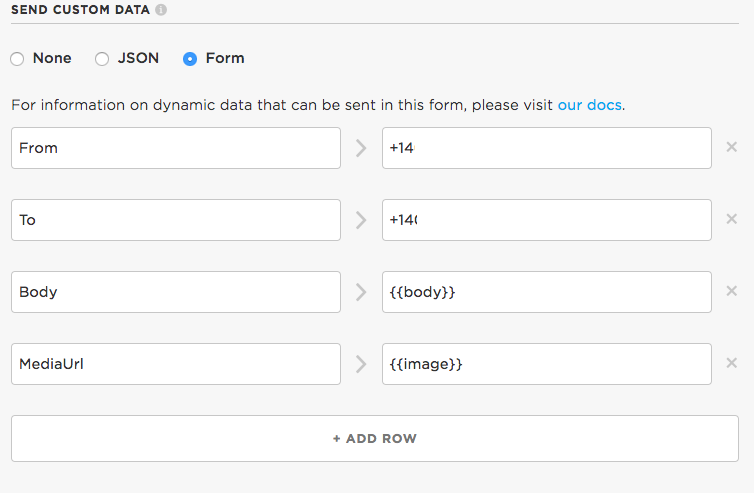
We now have 2 custom variables, body
and image
. In C++, we manually craft JSON and add the image path and message body.
Reset or Power Cycle the Photon
After a reset or power cycle (assuming you flashed it...) you should see a message and a nice picture of some wildlife.
Can you draw the owl that appears?
Texts From Moving Light Particles (or Waves?)
20 minutes after starting this tutorial, you've now got snippets which let you send SMSes or MMSes from a Particle Photon. Whether you're automating your house, monitoring the weather, or making the Next Big Thing™... we know you're going to build something awesome. Hopefully, this base proves useful!
We can't wait to see what you build, and please keep us posted on Twitter!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.