Introducing The Twilio Module For Node.js
Time to read:
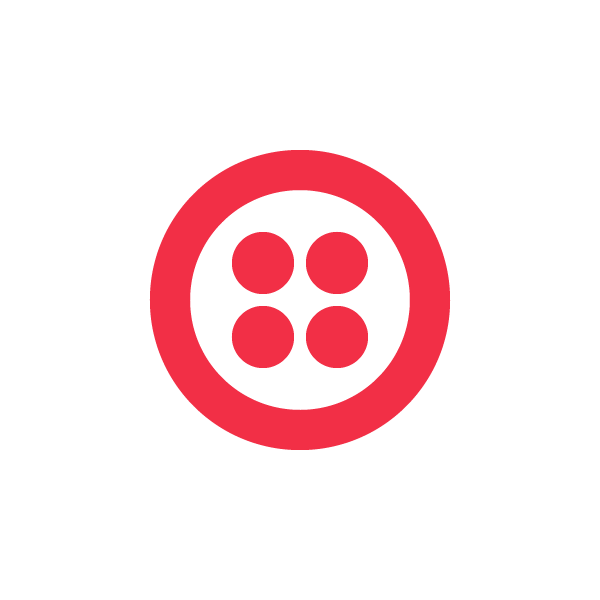
This is the first in a series of tutorial blog posts covering the use of Twilio’s new helper library for node.js. In this tutorial, we will cover the setup and basic features of the module to get you started fast. The next post in this series will help you learn how to turn your browser into a soft phone with Twilio Client and node.
The official node.js helper library documentation is located here.
Making Phones Ring With JavaScript
These days you can write just about any kind of software in JavaScript. Whether it’s a web front end, a 3D game, a native mobile app, or back end services on a server, today you can do it all in JavaScript. On the server, node.js is leading the charge, providing a super fast runtime built on the V8 JavaScript engine, a lean but powerful standard library, and a thriving community of module developers packing npm with useful software.
To support this growing user community, Twilio recently released a full-featured, officially supported helper library for node.js. To help introduce the module, I will do a series of blog posts in the coming weeks to show how you can implement awesome communications apps using JavaScript on the server. This week, we will cover the installation and key functional areas of the Twilio module at a high level to get you started.
Getting Started
First things first – if you have not yet installed node.js on your system, you can download an installer for your platform from nodejs.org. The installer should place both the “node” and “npm” executable commands on your system path. To confirm this is the case, open up a command prompt or terminal window and type “node -v” and “npm -v”.
As of this writing, the latest stable versions of node and npm are 0.10.1 and 1.2.15, respectively. As you use node, you may want to install multiple versions – if so, I would recommend taking a look at nvm. But for now, using the standard installer should suffice.
Now, let’s create a simple node program which uses the Twilio API. From the terminal, create a new directory for this demo exercise. Inside this new directory, create a file called “ahoy.js”, where we will write code to send a text message using the REST API. Next, install the Twilio module using npm, in the same directory as “ahoy.js”. Note that depending on your system configuration, you may need to install the module using “sudo”:
[sudo] npm install twilio
node.js has a simple module loading system which looks for modules in a “node_modules” directory in the path where the node program is run. After installing the Twilio module from npm using the method above, you will notice a “node_modules” directory in your demo folder, and that the twilio module is inside it.
Now let’s start writing some code. The Twilio module provides three main functional areas:
- A client for making authenticated requests against the REST API
- A utility for generating TwiML
- A utility for generating capability tokens for use with Twilio Client VoIP SDK
Writing a VoIP application with node.js will be the focus of next week’s post, so we won’t be exploring the third bullet just yet. But let’s take a look at the first two bits of functionality, starting with the REST API.
Using the REST API
Our first example will use the REST client to send a text message. Open “ahoy.js” with your favorite text editor and paste in the code below, replacing “TWILIO_ACCOUNT_SID” and “TWILIO_AUTH_TOKEN” with the appropriate values from your account dashboard. You will also need a Twilio phone number to send messages from – you can buy one here if you don’t already have one to use for testing. Replace “TWILIO_NUMBER” with this phone number. For testing, you’ll probably just want to send the message to your own mobile phone – replace “+16512223344” with your own mobile number:
To run this code, return to the terminal and run:
node ahoy.js
After a second or so, you should see information about the text message you just sent printed out to the console.
Since the REST API is the most commonly used feature of the module, we’ve tried to tighten up the API as much as possible for common functionality. In this example, we initialize a REST client with a single line of code, and use the “sendSms” function, which is a shorter alias for “sms.messages.create”:
In a production environment, you will not want to have your account SID and auth token stored directly in your source code, where they might be checked into version control by mistake. One solution is to configure your account SID and auth token as system environment variables, and access them from there. The node.js module automatically looks for this configuration in “process.env.TWILIO_ACCOUNT_SID” and “process.env.TWILIO_AUTH_TOKEN”, so if those variables are set, the following would be sufficient to initialize a REST client:
Generating TwiML to handle inbound calls and SMS
As experienced Twilio hackers know, when you receive an inbound call or text message to your Twilio number, Twilio makes an HTTP request to a URL controlled by the developer. This URL must return a special set of XML instructions called TwiML, which tells Twilio how to handle the call or SMS.
While you are free to generate TwiML using a template language or other utility, the Twilio node.js module provides a utility library which makes it very easy to create and output one of these documents. Let’s create a simple application which responds to any HTTP request with a simple set of TwiML instructions. In the terminal, create a new file called “inbound.js” and paste in the following example code:
Run this code in the terminal using the command “node inbound.js” – this will cause an HTTP server process to be created. After the server is started, visit http://localhost:1337/ in your browser and you should see an XML document that looks like this:
When you are finished testing, kill the HTTP server process in the terminal by pressing Control-C.
Nesting TwiML
Several TwiML verbs allow for nested nouns and verbs. One example is the tag, which collects input from a voice user via DTMF tones (the numeric buttons on a standard phone, plus * and #). To create a nested TwiML document, you will pass a function as an argument to a parent tag, as in the following example. Replace the contents of “twiml.js” with the following code, which will prompt the user for input:
Restart the HTTP server by running “node inbound.js”. When you visit http://localhost:1337/ you should now see an XML document that looks like the following:
Conclusion
With the Twilio module for node.js, you can develop a wide range of communications applications using the top server-side JavaScript platform. Today, we covered how to set up the module, use the REST API, and generate TwiML. Next week, we’ll explore VoIP in the browser with Twilio Client – be sure to stop back then!
If you have any questions or comments, please let us know (help at twilio.com). Or better still, fork the repo or submit an issue to let us know how we can make the module even better.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.