How to Use SMS for Customer Alerts & Notifications
Time to read: 2 minutes
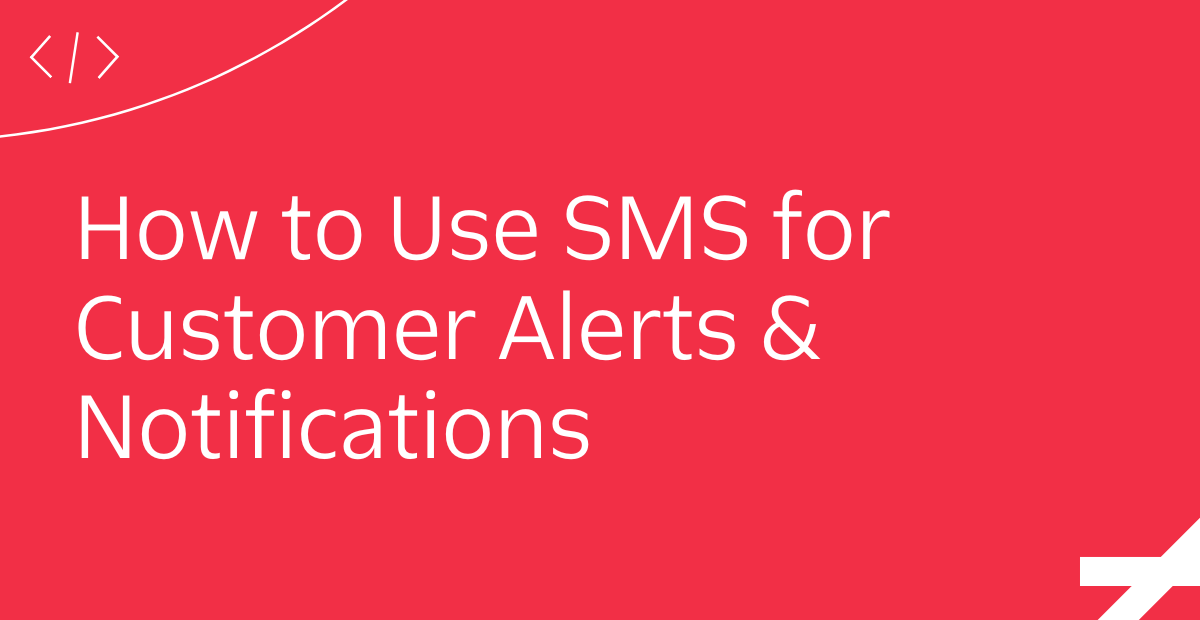
SMS is a guaranteed method for reaching your customers quickly. After all, close to 6.8 billion people own a smartphone, and they tend to check their devices an average of 96x per day. That means even if your customer doesn't feel the vibration or notification sound from your text message (which isn't likely), they’ll probably open their phone at least once in the next 10 minutes anyway.
When time is of the essence, count on SMS.
But remember, regardless of the timing or circumstances, you need to get opt-in approval before messaging your customers. You might do this during the account setup, onboarding stage, or further down the customer lifecycle—but you need to do it.
Here are a few common use cases for using SMS for customer alerts and notifications.
7 ways to use SMS for customer alerts
1. Emergency notifications
Send mass SMS when there's an emergency or public crisis. This might be for weather, natural disaster, violence, or suspicious activity. You also want to send an emergency text to your customers if a cyberattack compromised their account or personal data.
2. Financial alerts
Use SMS to alert customers of potential fraud or unusual account activity. A text message will give them instant notice rather than finding out a week later, which allows them to act quickly. If it's a legitimate financial transaction, they can immediately approve the expense and move on with their day—but if it is fraud, they can deny the transaction and take steps to secure their account.
3. Appointment reminders
Send a last-minute text to remind your customers about an upcoming appointment. If it's a virtual appointment, you might send this text 10–15 minutes before the appointment is about to start. But if it's an in-person appointment, you should send the appointment reminder text 12–24 hours in advance.
4. Billing reminders
Remind customers about large upcoming payments or overdue bills with SMS. This can be a powerful channel to improve timely payments and help customers avoid late fees.
5. Limited-time promotions
Use SMS to let your customers know about limited-time marketing promotions. Don't abuse this channel, though—your customers might unsubscribe from your SMS messaging if it's not a very good discount or compelling deal. Instead, save your SMS marketing for big-time markdowns you know your customers don't want to miss.
Ensure you send the right SMS marketing messages by allowing your recipients to tailor their preferences. Allow them to choose what deals or items they receive via text message.
6. Expiring credits
Let your customers know about expiring store credits to empower them to use them before they lose them. A few days’ notice before their credits expire provides them with enough time to find something worthy.
7. Delivery updates
Allow your customers to opt in for delivery updates to receive SMS messages about steps in the order fulfillment process. You can send text messages letting them know when the order was received, processed, shipped, and delivered. These updates keep your customers in the know about their orders and off the phone with customer service agents.
Start sending SMS customer alerts with Twilio
Ready to send text messages at scale? Get started with MessagingX, Twilio's trusted platform for cross-channel messaging.
MessagingX lets you send MMS, SMS, and WhatsApp messages from a single API with reach to 180+ countries. Sign up for a free account (no credit card required) to try it for yourself.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.