4 Ways to Parse a JSON API with Ruby
Time to read: 4 minutes
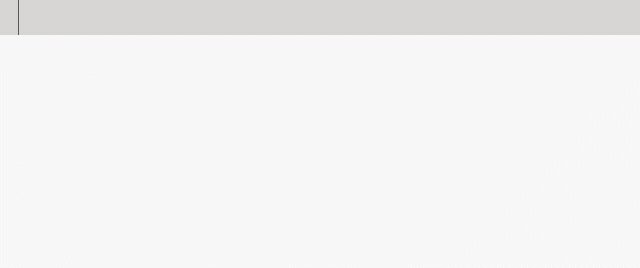
The Ruby Toolbox lists no less than 25 HTTP clients. Let’s look at how to retrieve and parse JSON results from a RESTful API using the four most popular Ruby HTTP libraries.
The four code snippets below will:
- Define a URL to be parsed. We’ll use the Spotify API because it allows requests without authentication.
- Make an HTTP GET request to that URL.
- Parse the JSON result.
Each snippet is a different path to the same destination; if you pp the result from any of them, you’ll see a hash with Spotify search results:
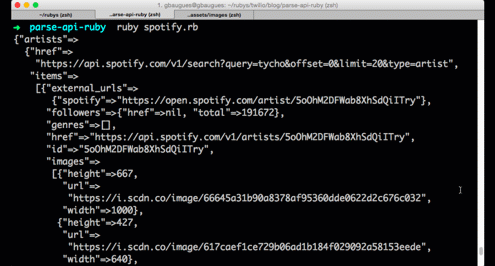
Once you have tried a few, head over to our documentation site and try some Ruby Quickstarts. But for now, onward!
net/http
net/http is built into the Ruby standard library. There’s no gem to install and no dependencies to cause headaches down the road. If you value stability over speed of development, this is the right choice for you (for example, the twilio-ruby gem is built on net/http).
Unsurprisingly, using Net/http can require more work than using the gems built on top of it. For example, you have to create a URI object before making the HTTP request.
HTTParty
net/http feels cumbersome and spartan at times. HTTParty was built on top of net/http in order to “Make HTTP fun again.” It adds a lot of convenience methods and can be used for all manners of HTTP requests.
It also works quite nicely with RESTful APIs. Check out how calling parsed_response on a response parses the JSON without explicitly using the JSON library:
HTTParty also offers a command line interface — useful during development when trying to understand the structure of HTTP responses.
rest-client
rest-client is “a simple HTTP and REST client for Ruby, inspired by the Sinatra’s microframework style of specifying actions: get, put, post, delete.” Like HTTParty, it’s also built upon net/http. Unlike HTTParty, you’ll still need the JSON library to parse the response.
Faraday
Faraday is for developers who crave control. It has middleware to control all aspects of the request/response cycle. While rest-client and HTTParty lock you into net/http, Faraday lets you choose from seven HTTP Clients. For instance, you can use EventMachine for asynchronous request. (For the others, check out the github repo).
That customization means that our Faraday code snippet is more involved. Before we make our HTTP request and parse our results, we have to:
- Choose an HTTP adapter. The default is net/http.
- Identify the response type. In this case we’ll use JSON, but you could also use XML or CSV.
You’ll notice that we pass our query as optional parameters on the get method instead of concatanating them into the URL string. And because we’ve already told Faraday that the response is going to be JSON, we can call response.body and get back a parsed hash.
Wrapping Up
The Ruby ecosystem offers a ton of options for interacting with JSON APIs. While these approaches are similar for the simplest of GET requests, the differences become more apparent as your HTTP requests grow in complexity. Play around and see which one best fits your needs.
Did we miss your favorite library? Let us know in the comments.
Try our Ruby Quickstarts, or our other Twilio Guides and Tutorials.
Many thanks to Phil Nash and Matt Makai for their contributions to this post. And thanks to updog and rjungemann for their feedback on /r/ruby.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.