Add a WhatsApp Channel to your Power Virtual Agents Bot with Twilio
Time to read: 5 minutes
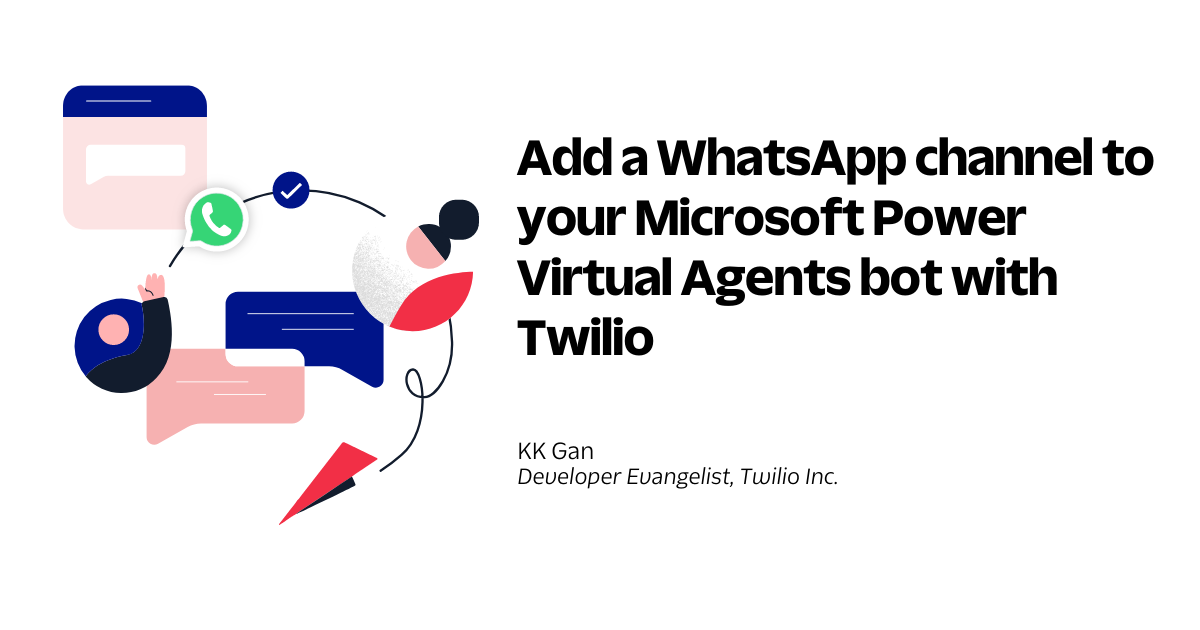
With Microsoft Power Virtual Agents, you can create a bot without writing code. However, if you would like to add the bot to Azure Bot Service channels, you will need to create a Relay Bot that acts as a bridge, and this task requires extensive programming knowledge.
This article demonstrates how to create a Relay Bot in C# to connect a bot built with Power Virtual Agents to Twilio Messaging for SMS or WhatsApp. This exercise assumes that you already have a Power Virtual Agents bot created, and would like to bridge the bot with a WhatsApp channel.
There are four sections in this article:
- Collect required parameters from Power Virtual Agent
- Create a Relay Bot with ASP.NET Core Web API
- Run and test the Relay Bot
- Configure Twilio Whatsapp Sandbox with Relay Bot
Prerequisites
To complete this tutorial, you’ll need an account with Twilio, a Power Virtual Agents subscription, a Power Virtual Agents bot created, and Ngrok installed and authenticated. If you have not done so already:
- Sign up for a free Twilio account
- Sign up for a Power Virtual Agents trial
- Create a Power Virtual Agents bot (i.e. please refer to Quickstart - Create and deploy a chatbot on how to create a Power Virtual Agents bot, if you have not done so already)
- Download, install and authenticate ngrok
Collect required parameters from Power Virtual Agents
Log in to your Power Virtual Agents dashboard.
Select the Power Virtual Agents bot you would like to add a WhatsApp channel to.
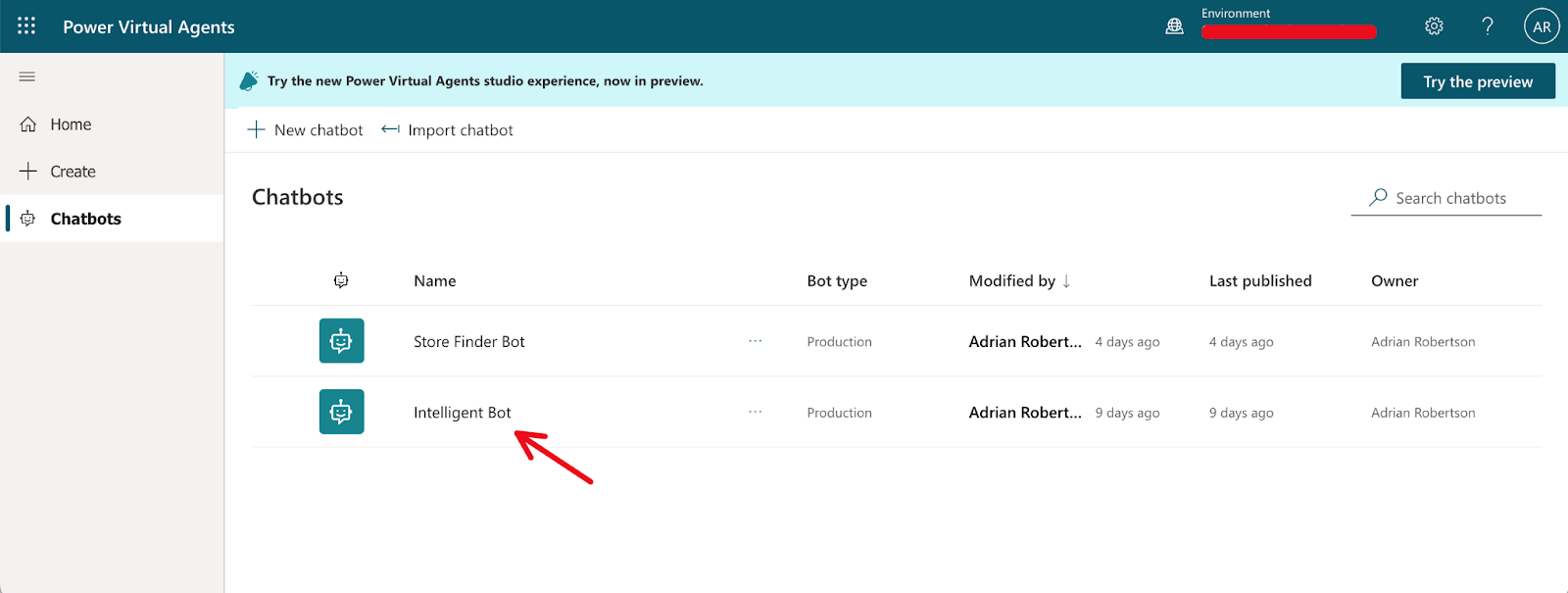
Select Details from the Settings menu of the selected bot. Then, copy the Tenant ID and Bot app ID from the bot details page as highlighted in the screenshot below. Save the values for later use.
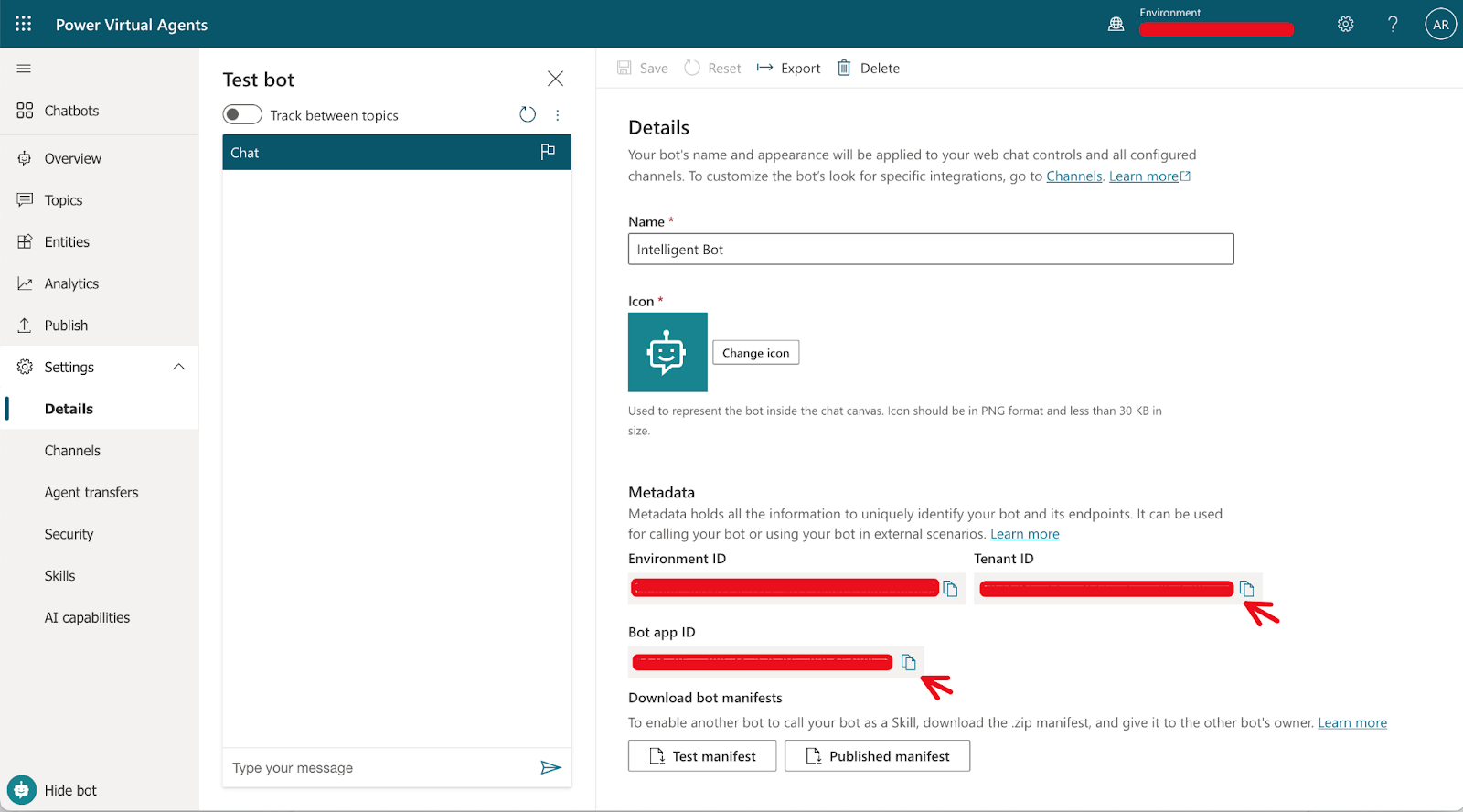
Go to the Channels section of the bot's settings and select Twilio, as shown below.
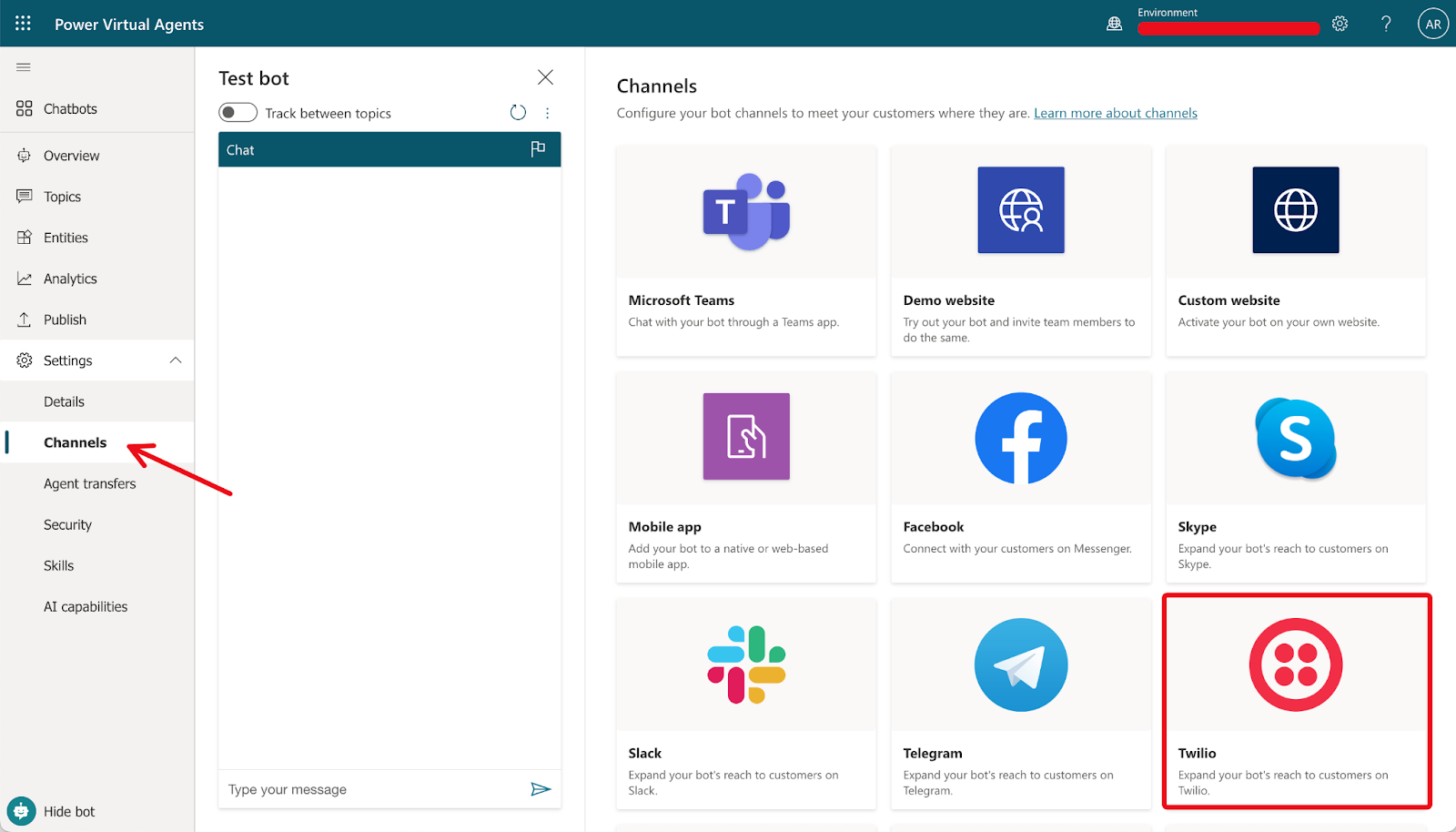
Copy and save the Token Endpoint value shown for the Twilio channel for later use.
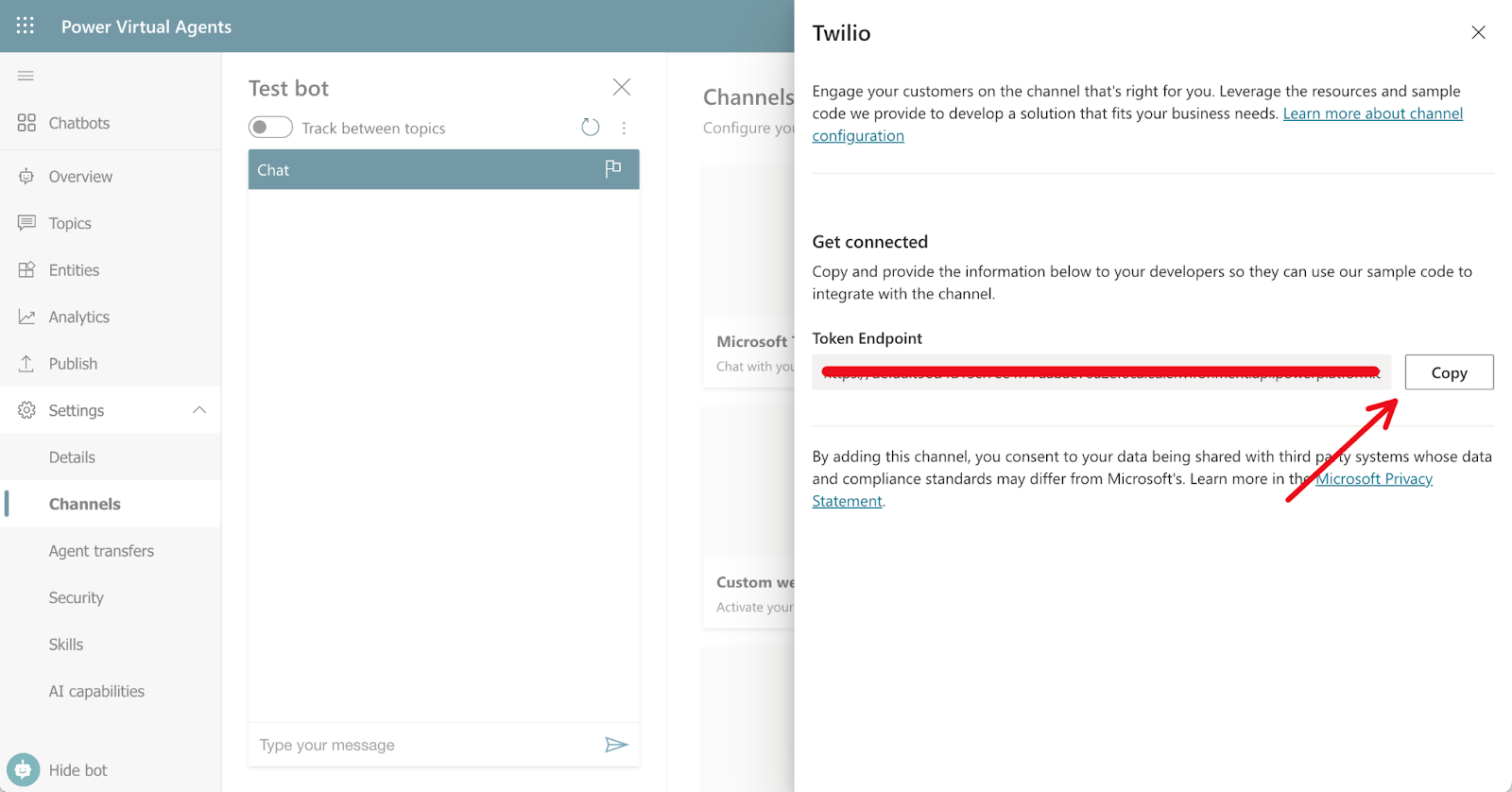
Create a Relay Bot with ASP.NET Core Web API
This section will guide you through creating a Relay Bot with ASP.NET Core in C#. The following prerequisites are needed:
The project and code that we are going to create in the following steps can be found in the BotConnectorAPI GitHub repository.
If you do not want to create the project from scratch, you can clone the repository, set the required bot parameters that you collected from the previous section in the project's appsettings.json file, and run the project directly.
If you choose to clone the project, you may skip this section and jump straight to the next section to Run and test a Relay Bot project.
If you prefer to create the project from scratch, the following instructions will guide you step by step on how to do so.
It is important to ensure that you have the right version of .NET. Verify the .NET SDK and version with the dotnet –list-sdks
and dotnet –version
commands. The sample output from these commands is shown below.
Use the command dotnet new webapi -o myBotConnector
to create a new .NET Core Web API project.
Once completed, change into the project folder and open the folder with Visual Studio Code.
Visual Studio Code will open the project with the folder where the code .
command was executed. The screenshot below shows how the project folder structure will look.
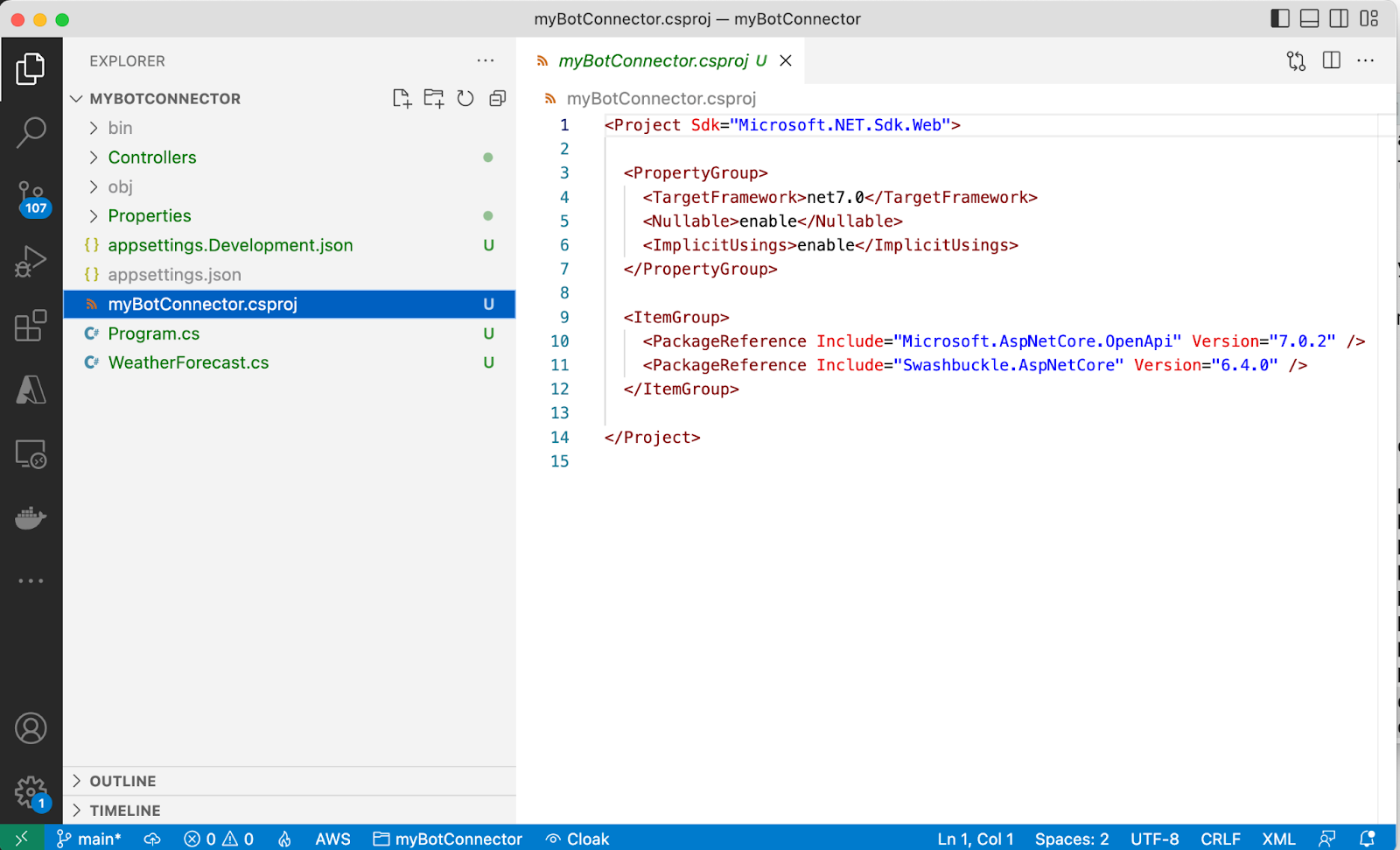
Open the myBotConnector.csproj file , and you will notice that two packages have been installed by default:
We now need to install the Microsoft.Rest.ClientRuntime
and Microsoft.Bot.Connector.DirectLine
packages manually. Run the dotnet
commands below from your terminal to install these packages:
You can verify that the packages were added to our project file as shown below.
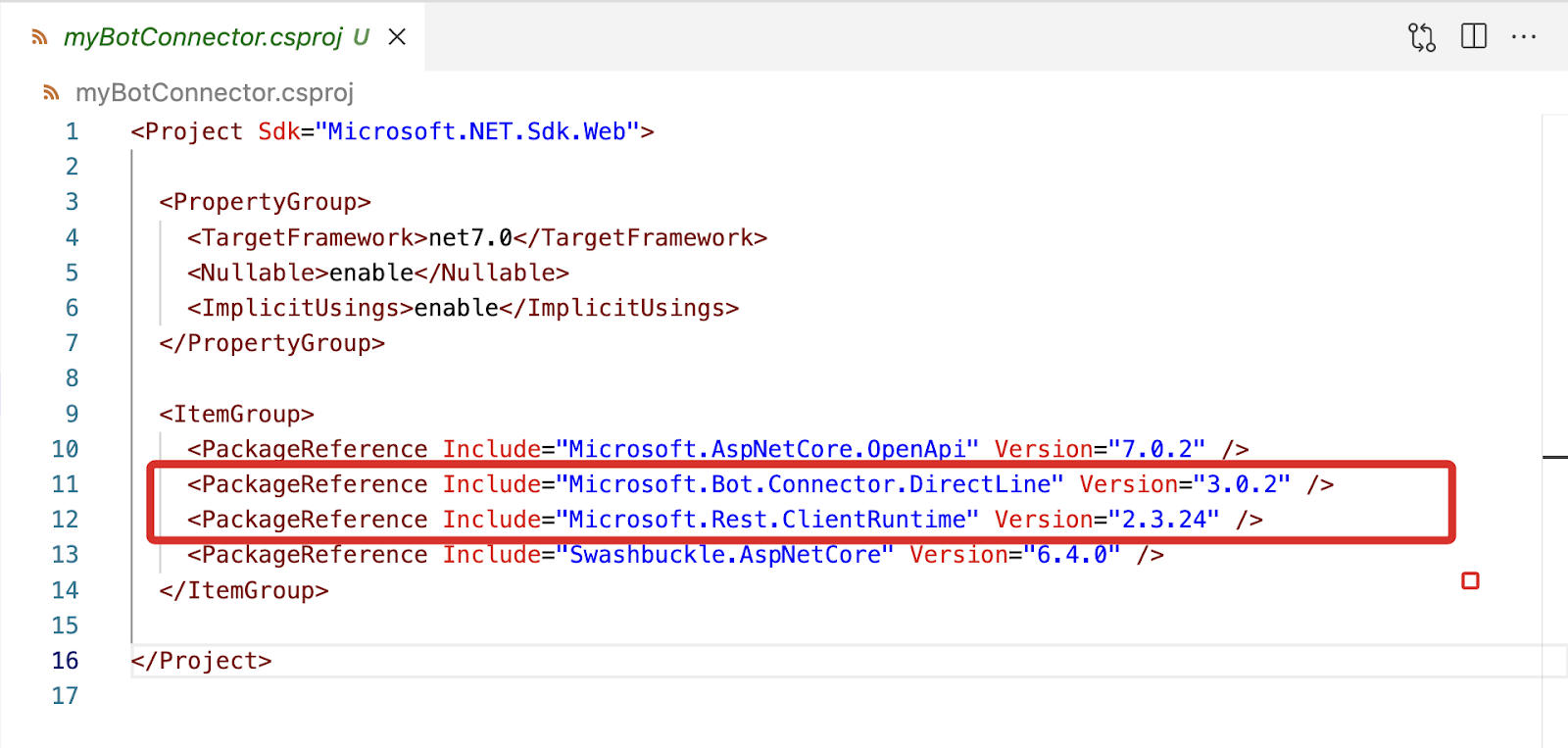
The dotnet new webapi -o myBotConnector
command created our project with default WeatherForecast.cs and Controllers\WeatherForecastController.cs files.
I would recommend we delete the unwanted WeatherForecast.cs file, clean up the unwanted code inside the WeatherForecastController.cs and rename the WeatherForecastController.cs to myBotConnector.cs as shown below.
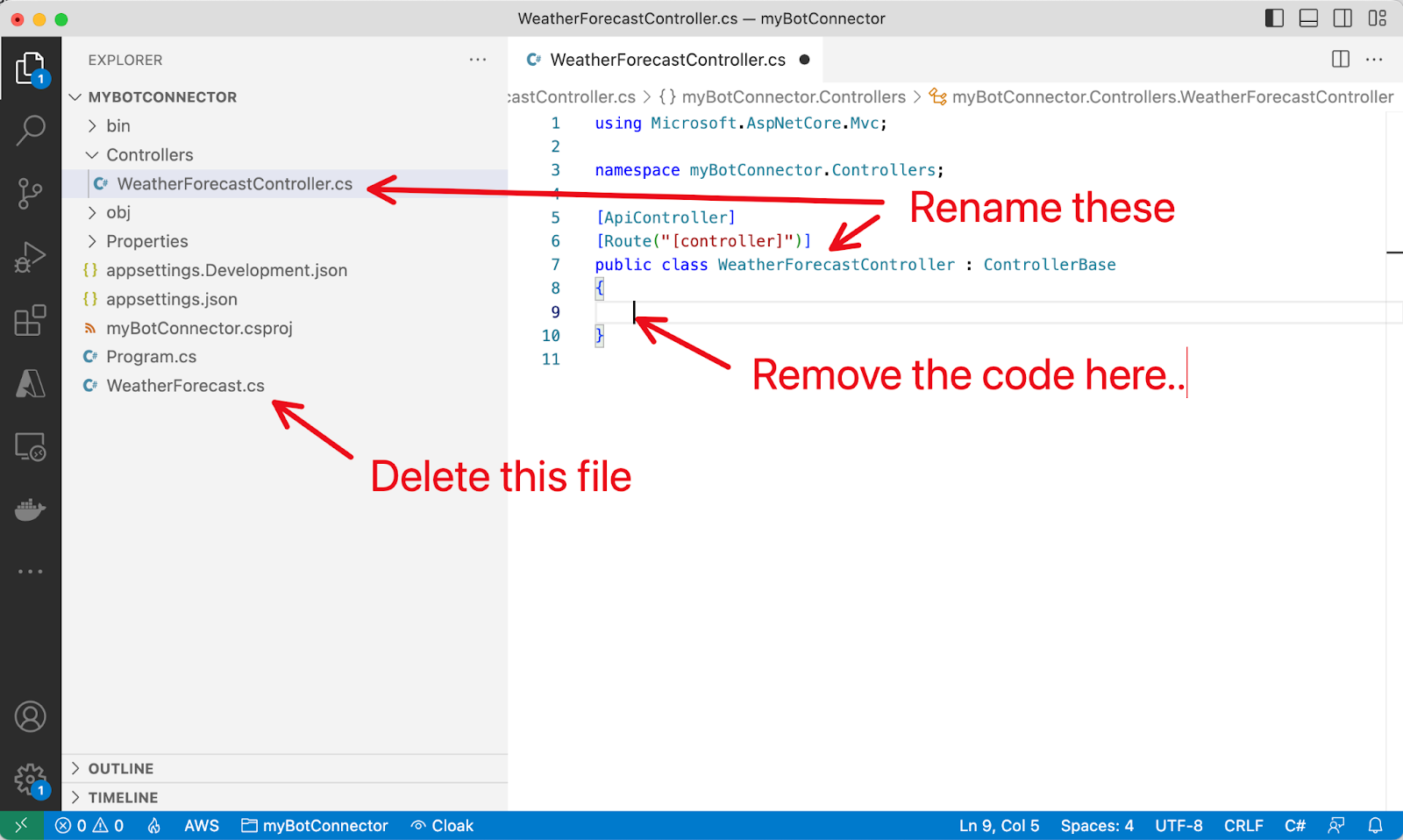
Your project folder should look like the below screenshot.
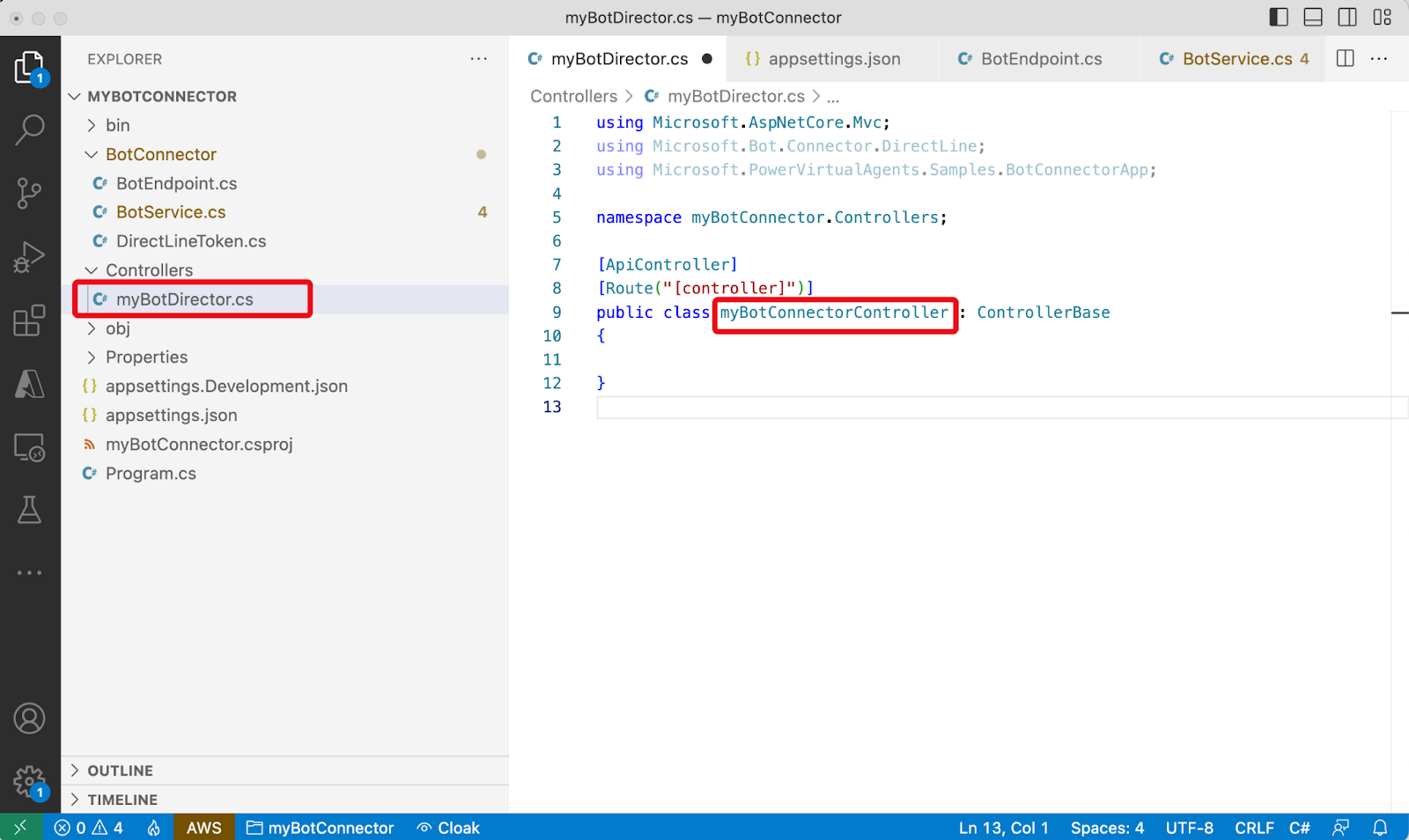
Run the project with the dotnet watch run
command. The documentation page should open, stating that “No operations defined in spec!”, as shown below.
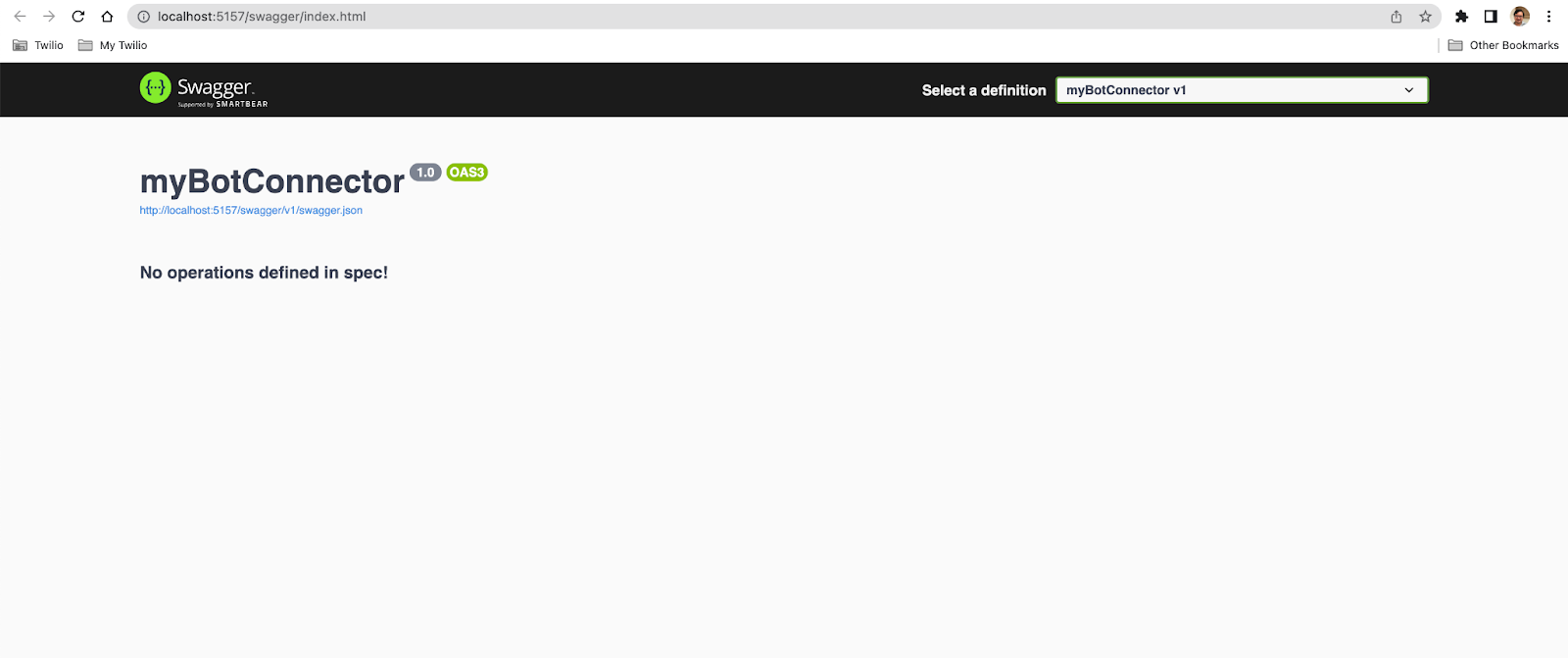
Back on Visual Studio Code, click the Explorer pane and select “New Folder” to create a new folder. Call the folder BotConnector.
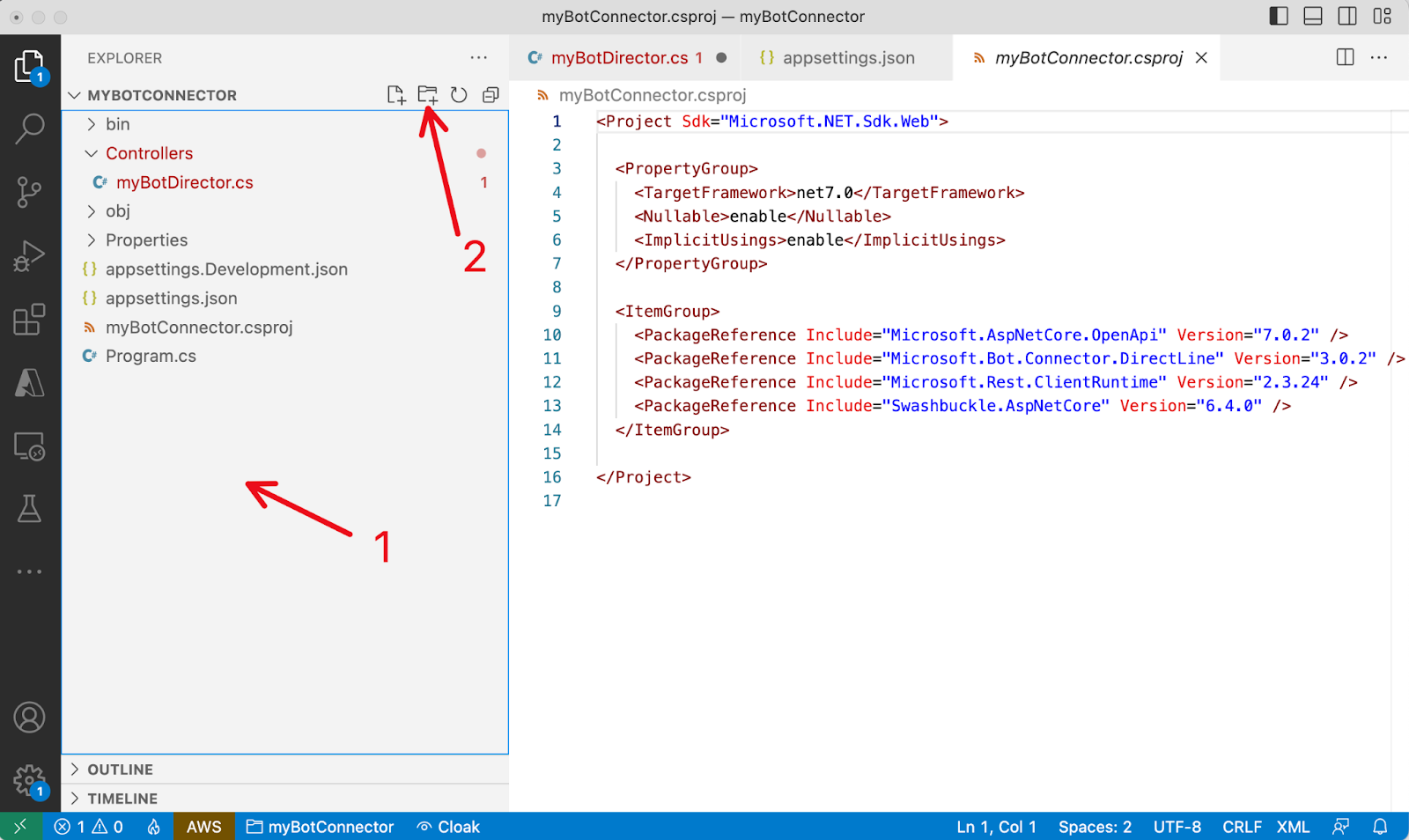
Add the following three files for the classes under the new BotConnector folder:
1. BotEndpoint.cs
2. BotService.cs
3. DirectLineToken.cs
The project folder should now look like the screenshot below.
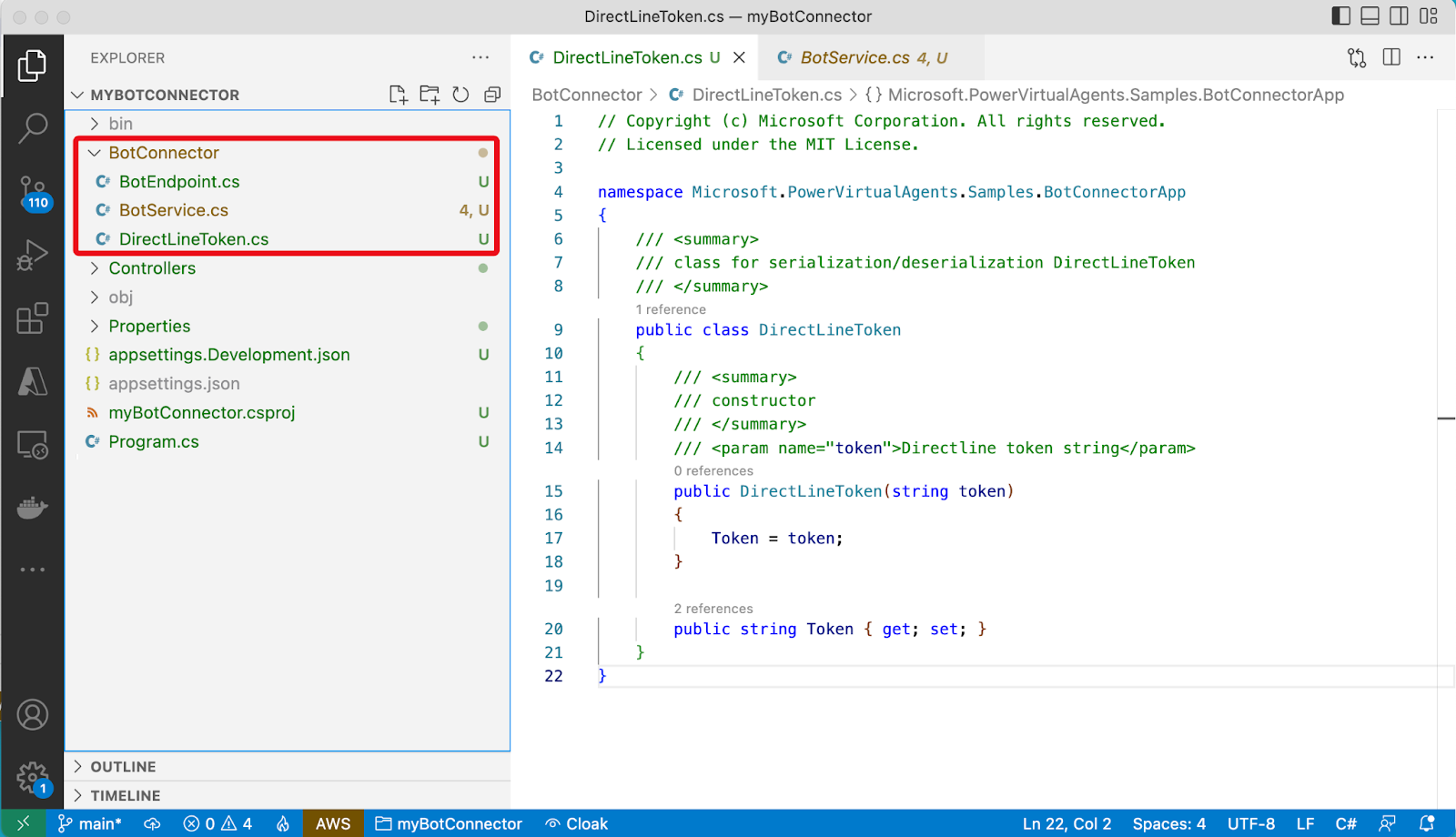
Replace the content of myBotConnector.cs with the below code.
Update the appsettings.json file with the required application settings as shown below.
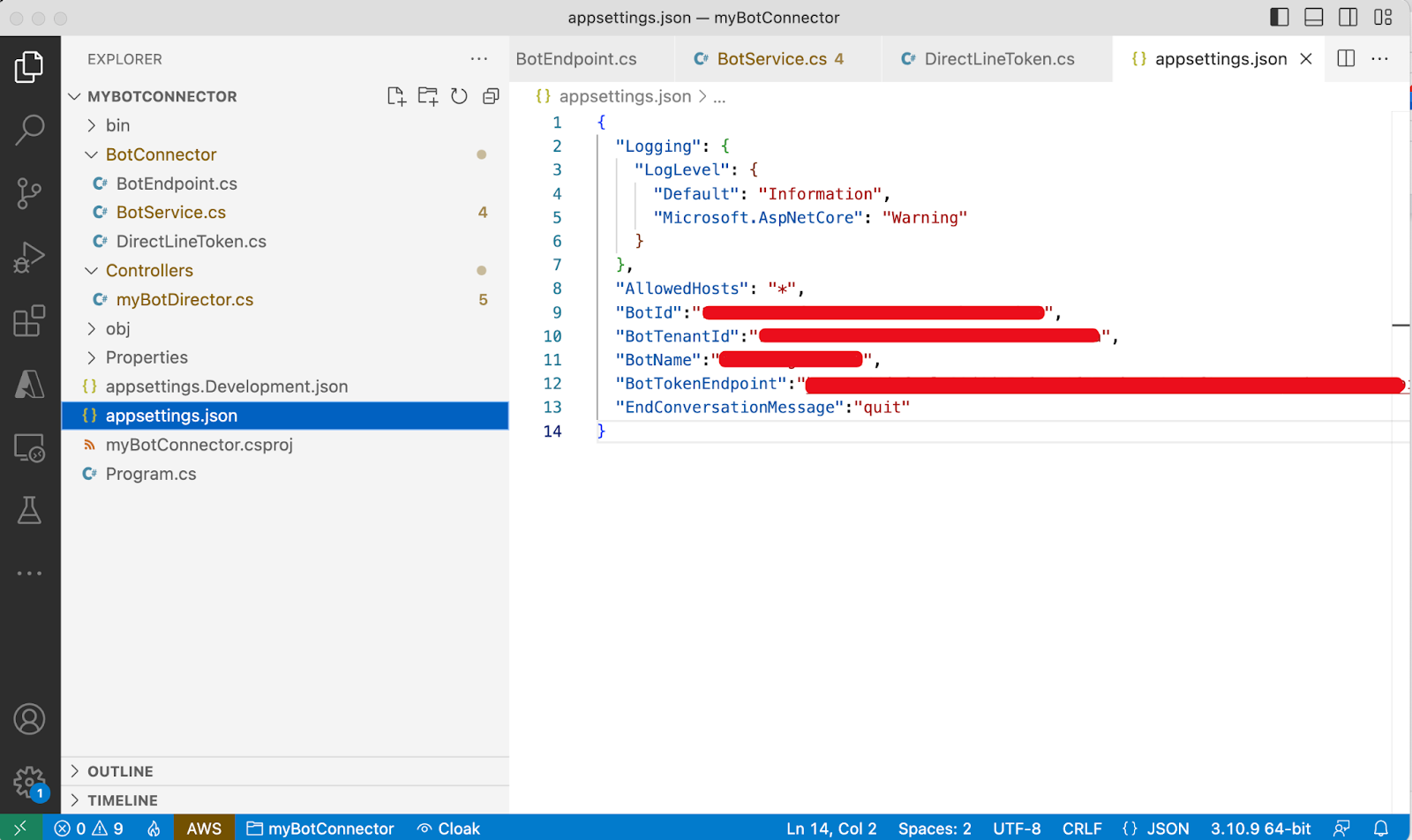
The BotConnector
is now ready to relay messages between a front end client (WhatsApp in our case) and the Power Virtual Agents bot.
Run and test the Relay Bot
Run the project with dotnet watch run
from the project folder. The project documentation page should now look as follows.
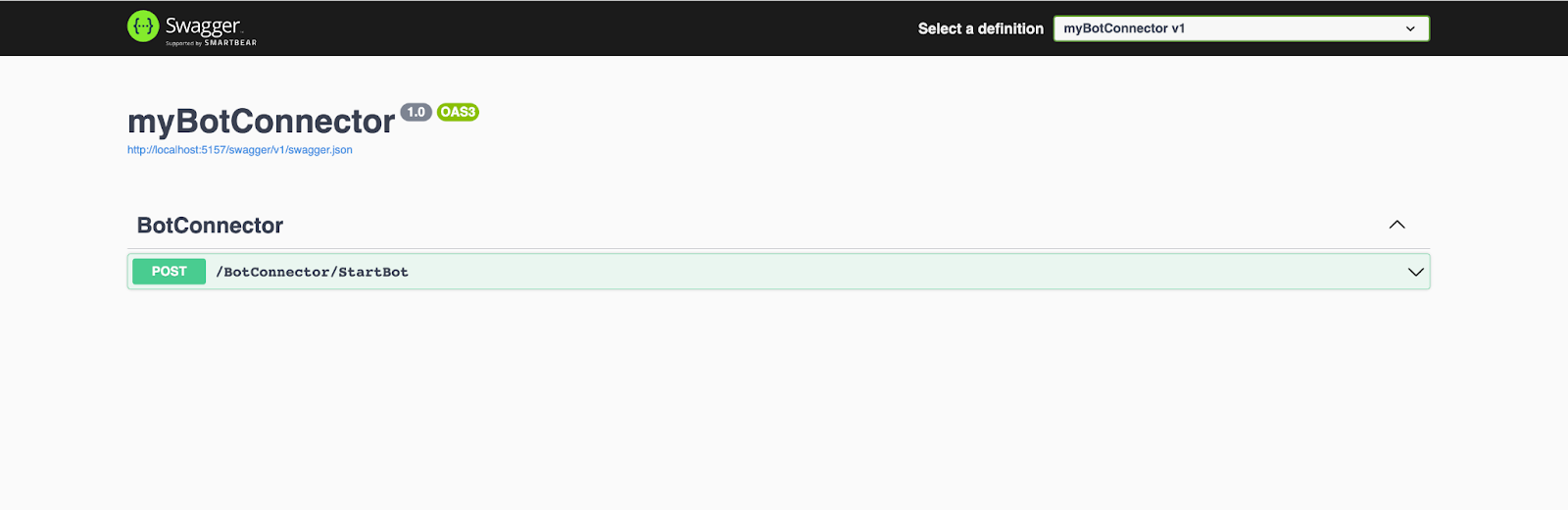
In this page, click on the only endpoint and proceed to test it by supplying the “From” and “Body” fields with any values as shown in the below screenshot.
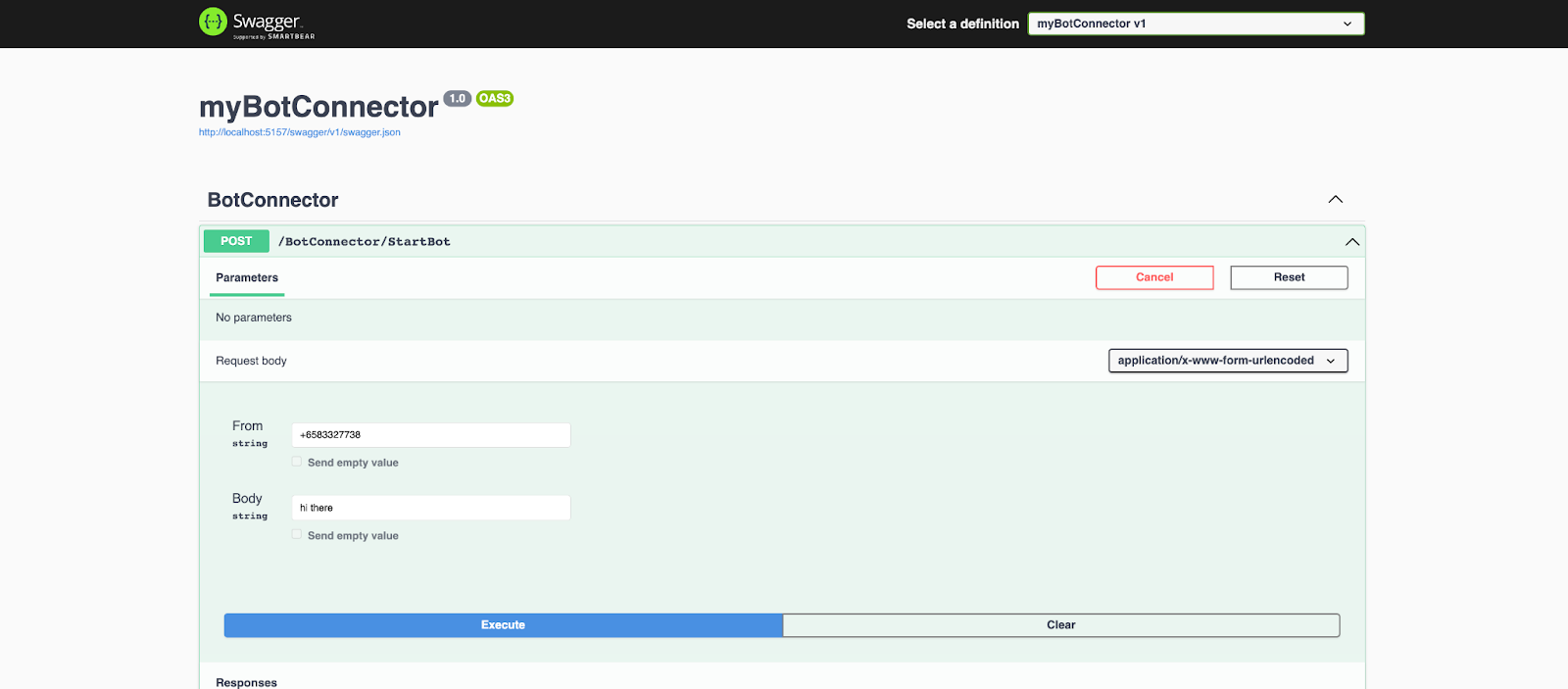
Hit the Execute button, and you should see the response from the API, as shown below.
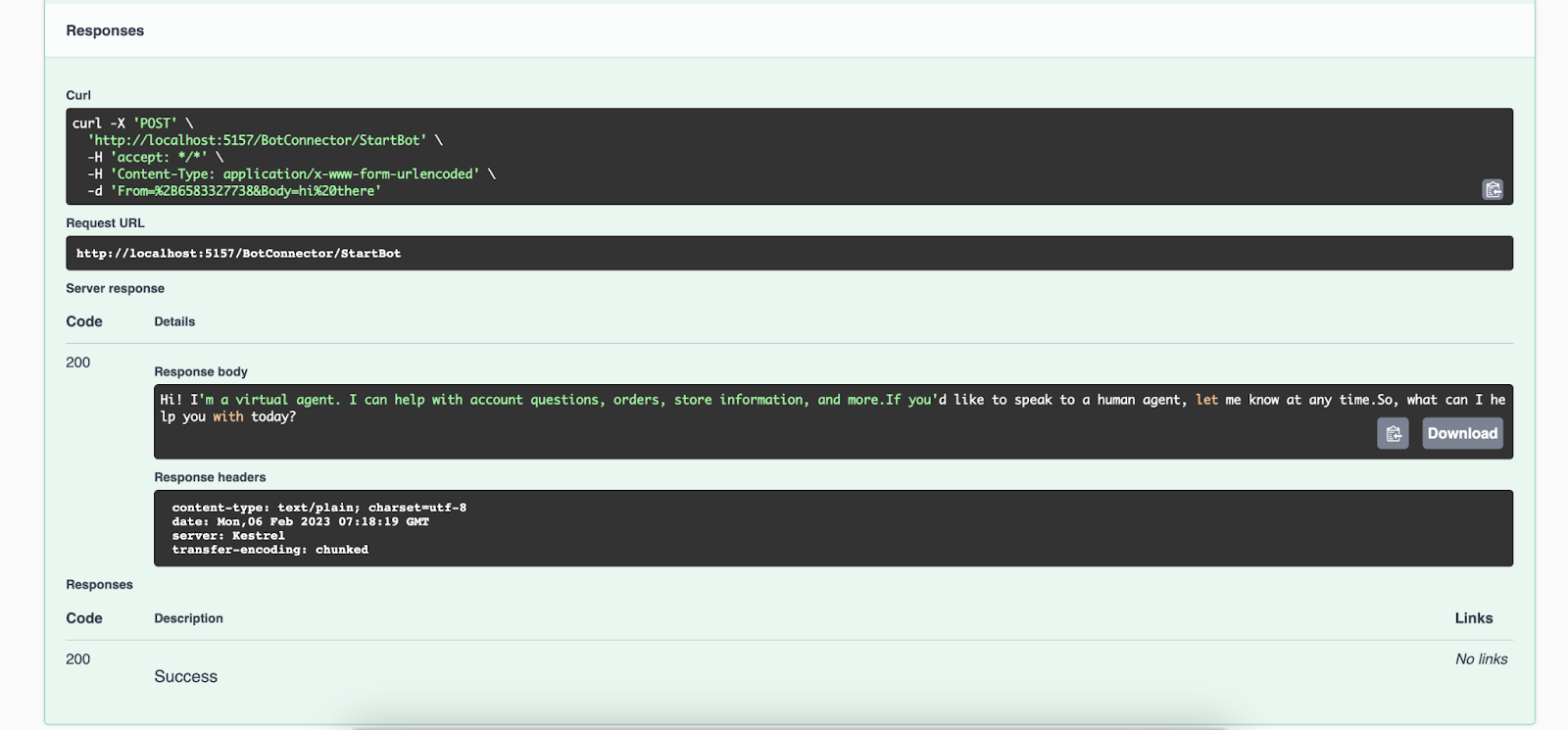
The Relay Bot is now ready for the Twilio messaging configuration. Take note of the endpoint path from the Relay Bot documentation page, highlighted in the screenshot below.
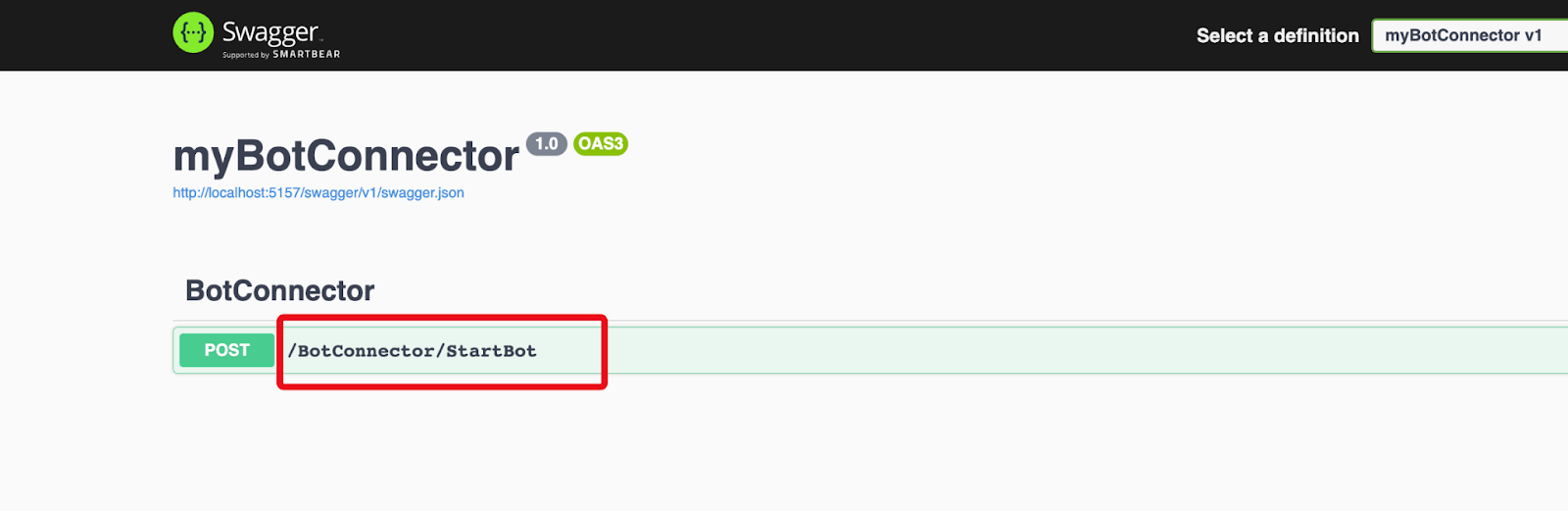
Configure the Twilio WhatsApp Sandbox with Relay Bot
Since our project is now running on localhost, we will use ngrok to set up a tunnel to expose it to the internet. To do so, start ngrok in a separate terminal session with the http port of the project, for example ngrok http 5157
.
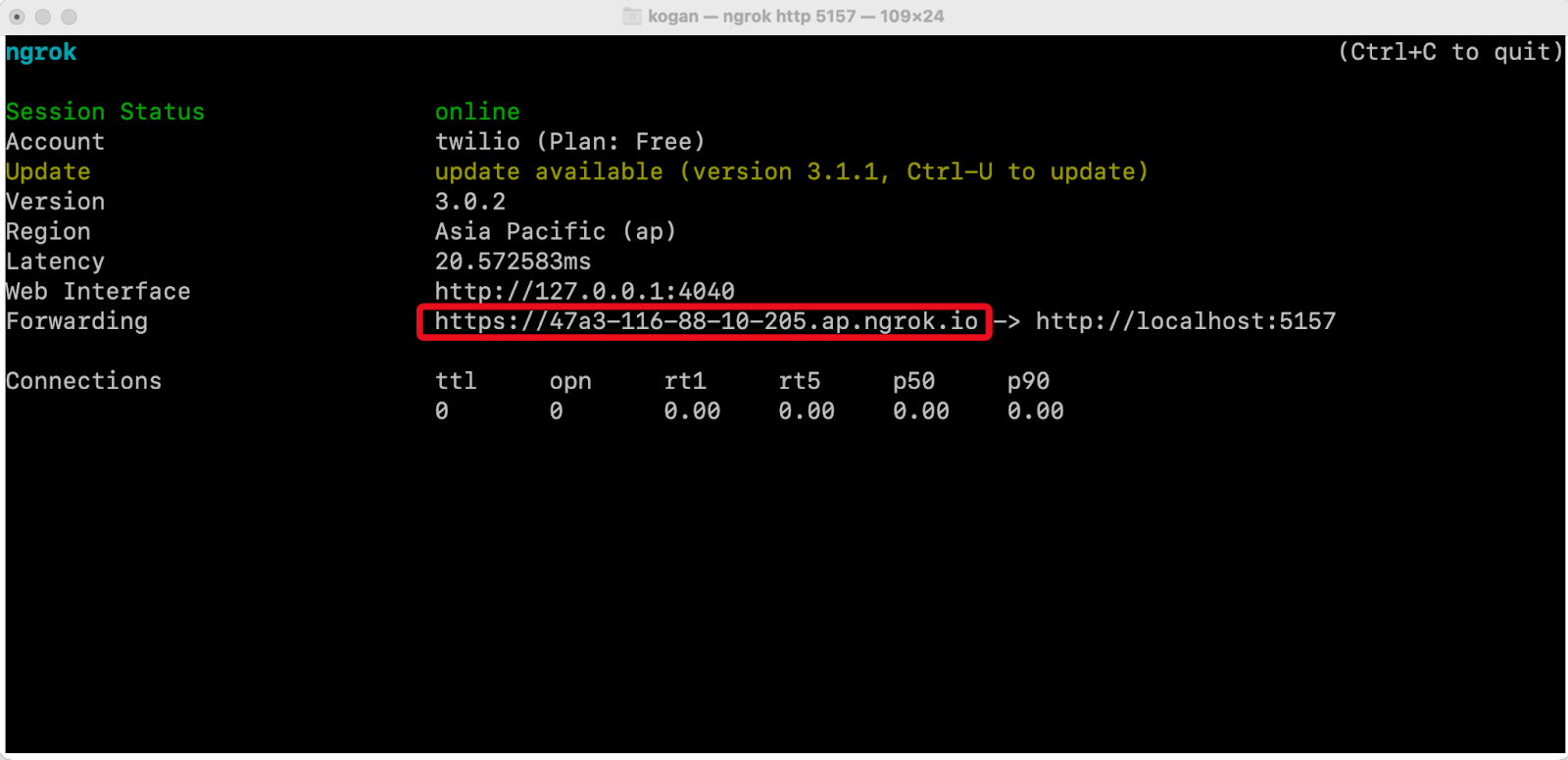
Open the Twilio console and navigate to the Messaging - Settings - WhatsApp Sandbox Settings. There, enter the full URL for the Relay Bot in the “When a message comes in” field. The URL is composed with the ngrok forwarding URL with the Relay Bot’s endpoint added at the end. An example URL should look like https://47a3-116-88-10-205.ap.ngrok.io/BotConnector/StartBot.
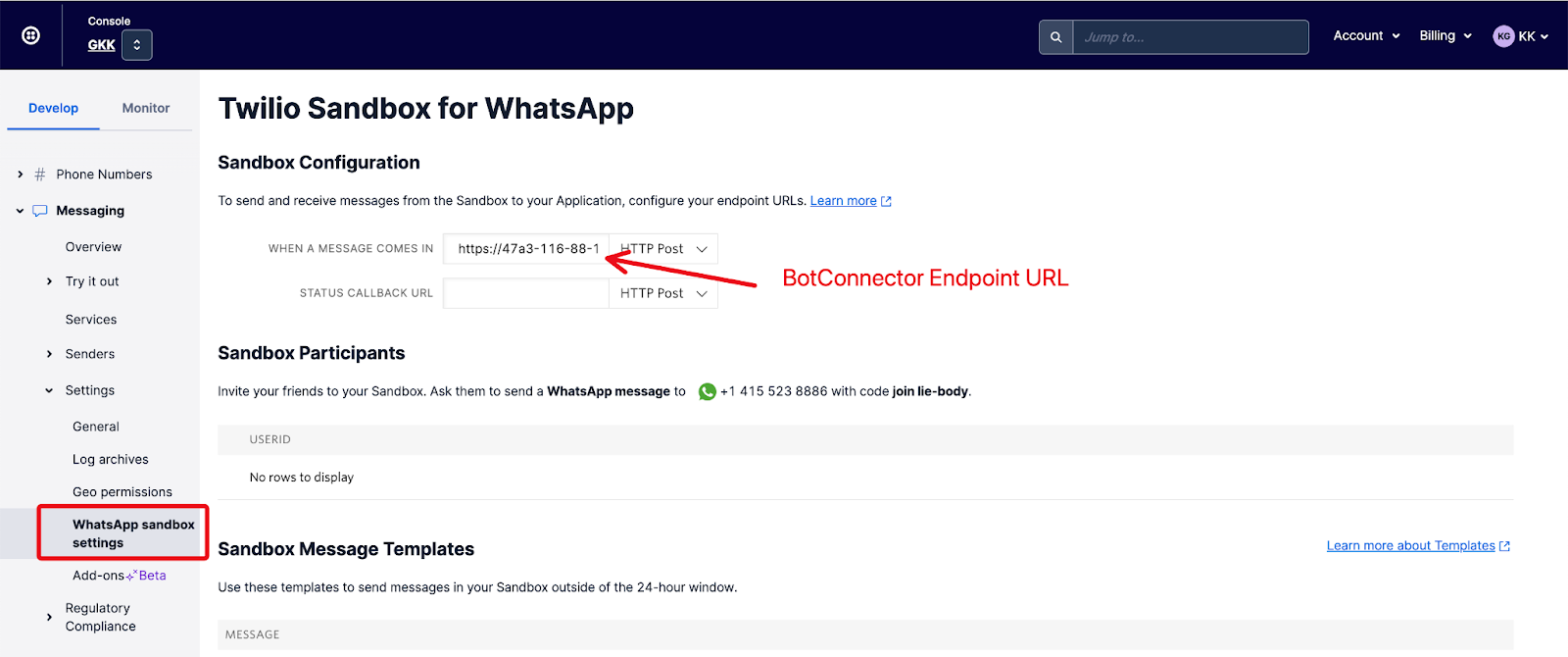
Save the WhatsApp Sandbox Settings. You can now chat with the Power Virtual Agents bot by initiating a WhatsApp message to your Twilio Sandbox for WhatsApp at the number shown in the Sandbox Participants section of the Twilio Sandbox for WhatsApp settings page. The below screenshot shows a sample interaction with the Power Virtual Agents bot over WhatsApp.
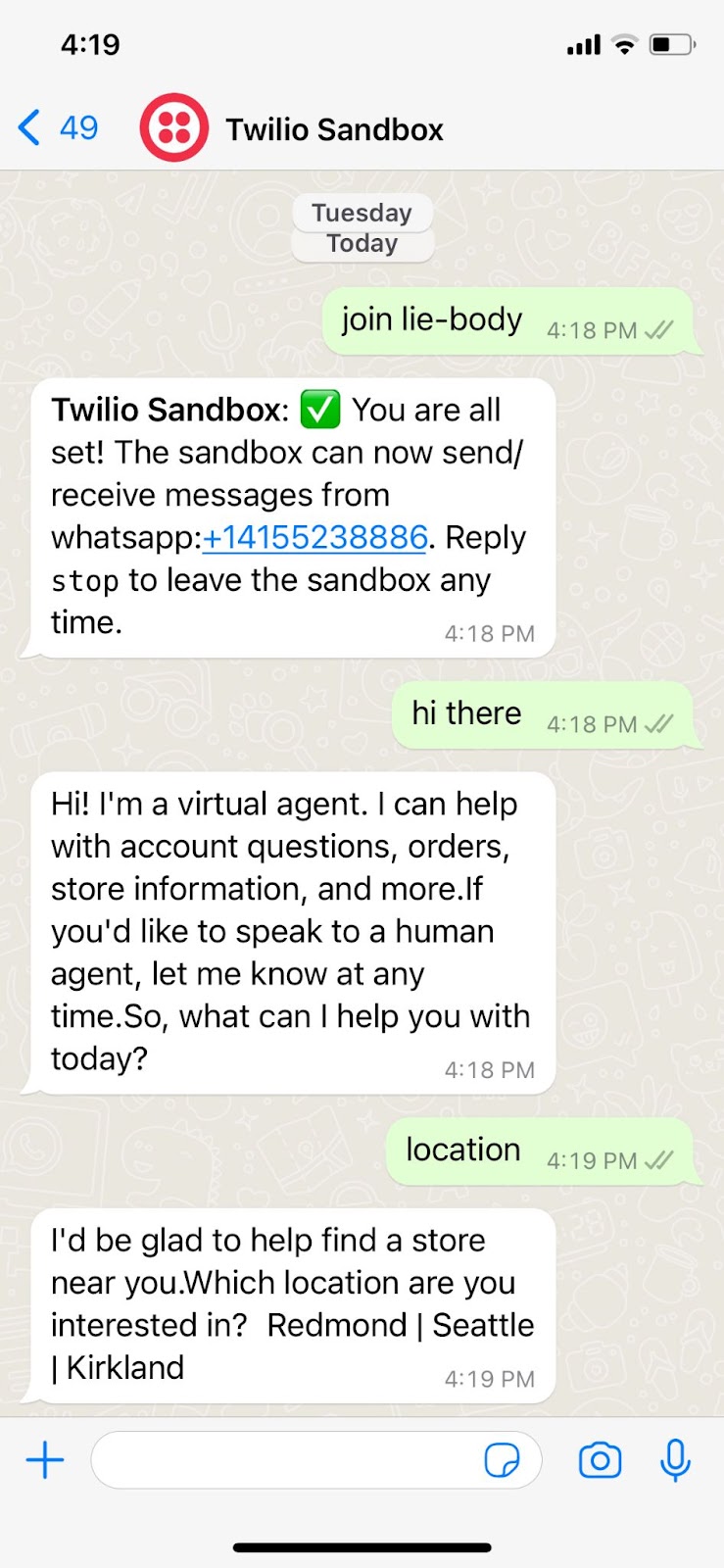
Congratulations! You've now created a Relay Bot, connecting a Power Virtual Agents bot and WhatsApp with Twilio. You can interact with the bot by texting to your WhatsApp enabled Twilio Phone Number. You may explore further on Formatting, location, and other features in WhatsApp messaging to further enhance your Power Virtual Agents bot in responding with advanced messaging features.
KK Gan is a Developer Evangelist at Twilio. He's been involved in developing and implementing business process automation, communication and collaboration solutions for different clients in the past. He loves and enjoys sharing and learning with the developer communities. He can be reached at linkedin.com/in/kkgan or kogan[at]twilio.com.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.