How to Send an MMS with Python
Time to read: 3 minutes
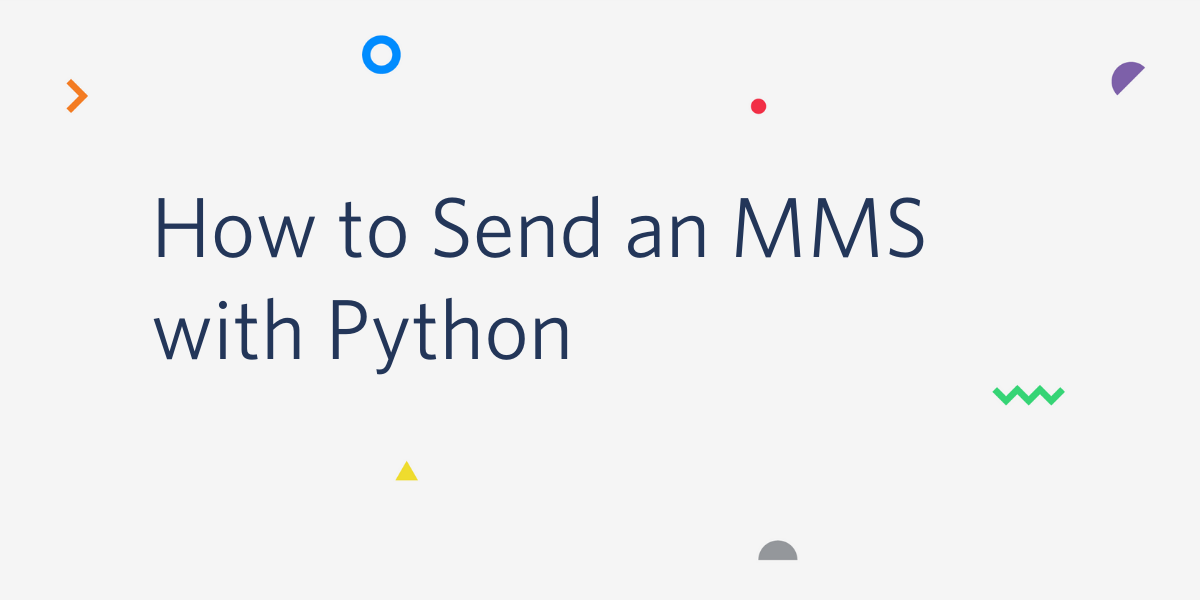
Twilio is all about powering communication and doing it conveniently and fast in any language.
With the help of Twilio and Python, you can deliver a quick media message to someone without having to pick up your mobile device.
In this article, you'll be writing a short Python program to send an MMS in an insanely fast manner. So why wait? Let's get started!
Tutorial requirements
- A Twilio account. If you are new to Twilio click here to create a free account now and receive $10 credit when you upgrade to a paid account. You can review the features and limitations of a free Twilio account.
- A smartphone with active service, to test the project.
Configuration
We’ll start off by creating a directory to store the files of our project. Inside your favorite terminal, enter:
Following Python best practices, you are now going to create a virtual environment, where you are going to install the Python dependencies needed for this project.
If you are using a Unix or Mac OS system, open a terminal and enter the following commands:
If you are following the tutorial on Windows, enter the following commands in a command prompt window:
With the virtual environment activated, you are ready to install the only Python dependency required for this project:
The twilio
package will allow us to work with the Twilio APIs for sending MMS.
Buy a Twilio phone number
If you haven't done so already, purchase a Twilio number to send the MMS.
Log in to the Twilio Console, select Phone Numbers, and then click on the red plus sign to buy a Twilio number. Note that if you are using a free account you will be using your trial credit for this purchase.
In the “Buy a Number” screen you can select your country and check MMS in the capabilities field. If you’d like to request a number from your region, you can enter your area code in the “Number” field.
Keep in mind that MMS is only supported in the US and Canada at this time so you might not see the MMS box if you are outside these countries. In that case you can purchase a number that has SMS capability instead.
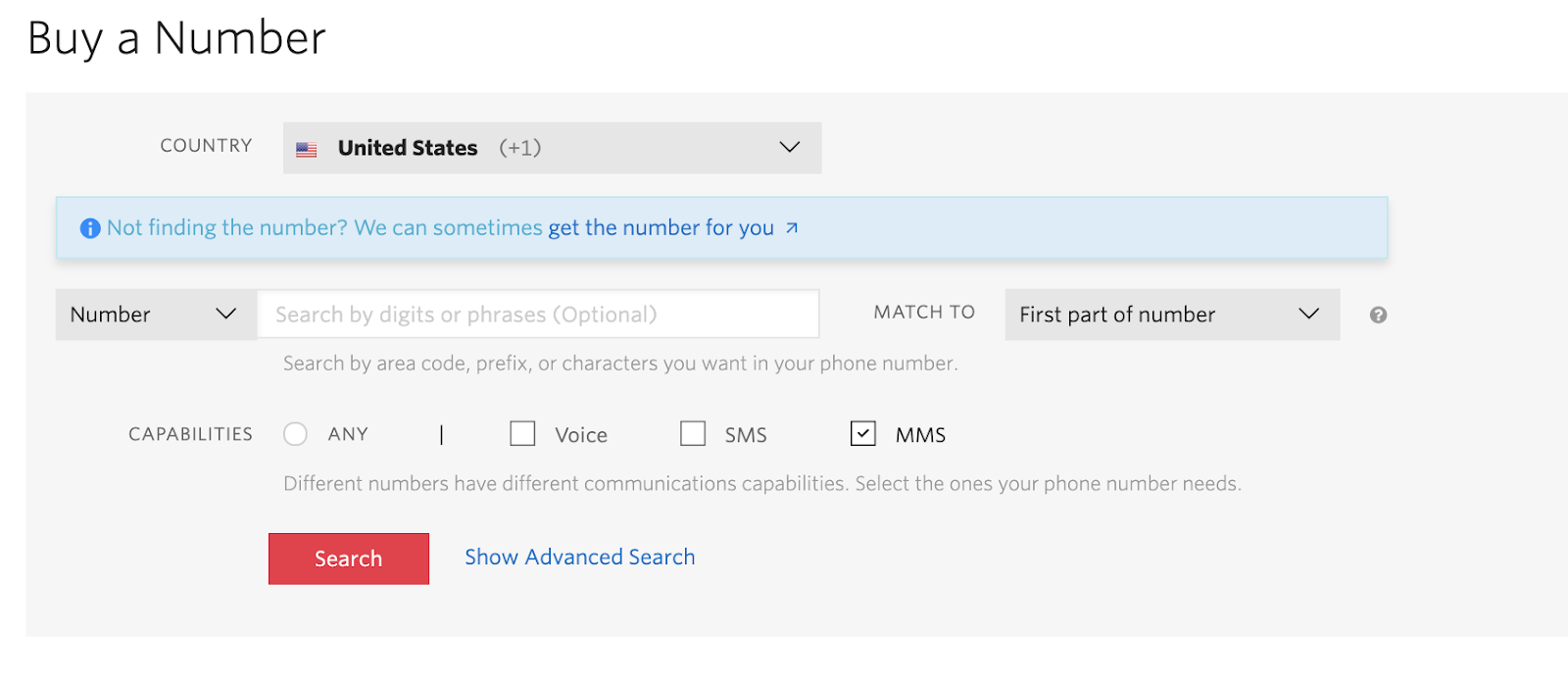
Click the Search button to see what numbers are available, and then click “Buy” for the number that you like from the results. After you confirm your purchase, click the Close button.
Send an MMS with Python
Create a file named send_mms.py in your project directory and paste the following code:
The code above needs access to your Twilio account credentials. To protect your account details we are going to set environment variables.
You can find your account credentials on the Twilio Console.
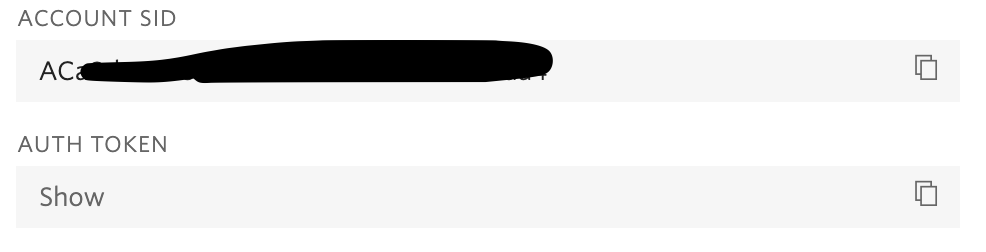
If you are using Mac OS or Linux, run the commands below to add your account credentials to your environment:
If you are following this tutorial on a Windows computer, you can assign your credentials to environment variables as seen below:
The send_mms()
function starts by initializing a Twilio client instance, using the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
variables set above.
We then create a new message
instance in order to send an MMS to your personal phone number from your Twilio phone number.
Since we want to send a media message, the media_url
argument is called so that Twilio knows how to retrieve and send the media message. The URL that you pass in this argument must be publicly accessible, so keep that in mind when choosing an image to send. The example image above displays Twilio's favorite "Draw the Owl" meme, but feel free to change the URL to point to your favorite image.
After the message is sent, the Message SID is printed on your terminal for your reference.
To complete the script, replace the <YOUR_PHONE_NUMBER>
and <YOUR_TWILIO_NUMBER>
placeholders with your actual phone numbers, using the E.164 format.
Once you are done editing the file, save it to the project directory.
Run the application with the following command:
In just a moment, you will see a ping from your personal phone with the MMS sent directly from your terminal!
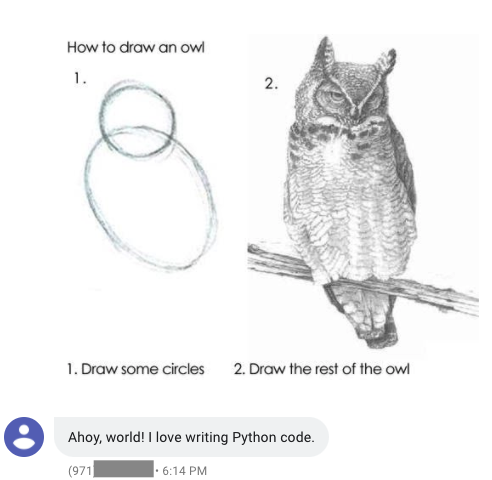
What's next for sending MMS with Python?
Congratulations on writing a short Python script and using the command line to send a quick MMS to your phone! This was so wickedly quick, can you even believe it was possible?
There's so much more you can do with Twilio and Python code! If you want some ideas, try the tutorials below:
- Build an SMS Chatbot with Python, Flask and Twilio
- Building a Bulk SMS Service with Python, Flask and Twilio Notify
I can’t wait to see what you build!
Miguel Grinberg is a Principal Software Engineer for Technical Content at Twilio. Reach out to him at mgrinberg [at] twilio [dot] com if you have a cool project you’d like to share on this blog!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.