How to Build a Command Line Application (CLI) to View Your Twilio Account Usage
Time to read: 5 minutes
Building APIs and web-based applications are the mainstays of what we as web developers do. However, command line applications are also an essential skill to master. They help us do what we do more effectively and easily, such as by allowing us to build small tools and utilities to help us with routine, repetitive tasks.
In this tutorial, I'm going to show you how to use Symfony's Console Component to build a command line application that can retrieve usage records from a Twilio account.
Prerequisites
To follow along with this tutorial, you will need the following:
- Composer installed globally.
- PHP, 7.4 or higher, with the Internationalization extension.
- A Twilio account (free or paid). If you are new to Twilio click here to create a free account.
Create the project directory structure
The first thing to do is to create the core project directory structure (to aid in project readability) and then change into the top-level directory. Do that by running the following commands.
Add the required dependencies
The next thing to do is to add the required dependencies. Only three are required. These are:
- The Symfony Console Component: This provides all of the core classes for creating a custom Symfony command.
- The Twilio PHP Helper Library: This simplifies interacting with Twilio's APIs.
- PHP Dotenv: This simplifies storing and retrieving environment variables.
To install them, run the command below in the project directory.
Retrieve your Twilio credentials
The next thing to do is to make your Twilio credentials (your Twilio Account SID and Auth Token) available to the application. First, create a new file named .env in the top-level directory of the project.
Then, in that file paste the code below, which adds environment variables and a placeholder for them.
Next, from the Twilio Console's Dashboard, copy your Account SID and Auth Token and, in .env, replace the respective placeholder values with them (<TWILIO_ACCOUNT_SID>
and <TWILIO_AUTH_TOKEN>
).
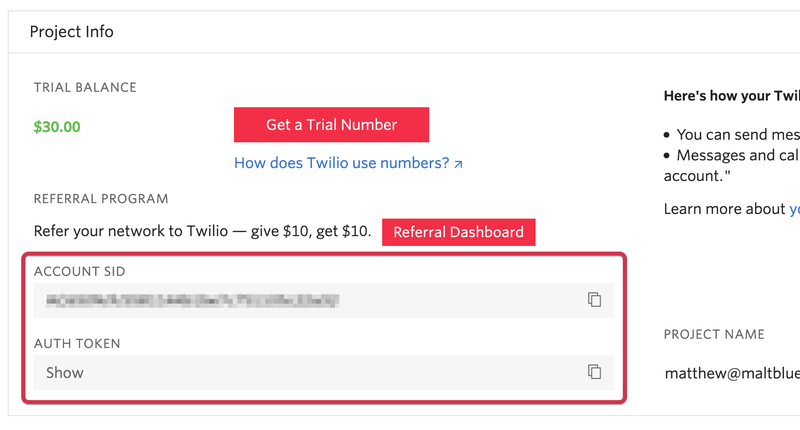
Create a class to retrieve the usage records
Next, you need to create TwilioUsage
, the first of three classes, by creating a new file named TwilioUsage.php in src. Then, in that file paste the code below.
The class starts by defining a constant and two properties. The constant holds the default number of records to retrieve. The first property is a Twilio Client
, which provides access to all of Twilio's APIs. The second property maps the internal usage categories to more human-readable versions. While not strictly necessary, I try to avoid exposing too much internal structure of an API if I can.
The __invoke()
magic method contains the class' core logic. It takes four parameters:
Parameter | Description |
---|---|
$maxResults | The maximum number of results to return. |
$startDate | This is the date that records must be created on or after. |
$endDate | This is the date that records must be created on or before. |
$category | A usage category. |
The start and end dates are converted to DateTime objects and, if the category provided is in the list of allowed categories, its internal mapping is retrieved.
$startDate
, $endDate
, and $category
are then stored in an options array ($options
) and passed, along with $maxResults
, to $client
's usage->records->read()
method. This method reads all of the usage records from the user's account.
Create the custom Symfony command
Now, you need to create the custom Symfony command. In src/Command create a new file named TwilioUsageCommand.php. Then, in that file, paste the code below.
The class starts off by defining the command's core settings, including the command's name ($defaultName
), description ($defaultDescription
), and a help message ($helpMessage
). Then, it initialises a TwilioUsage
and a NumberFormatter
object in the class constructor.
You've already seen TwilioUsage
. NumberFormatter
, part of PHP's Internationalization extension, formats usage pricing information in a more human-readable way, which you'll see shortly.
The configure()
method adds four options to the command, listed in the following table.
Option Name | |
---|---|
Long | Short |
--limit-records | -l |
--start-date | -s |
--end-date | -e |
--category | -c |
Next, comes the execute()
method. The method starts by retrieving the value for each of the four options or provides a default if the user hasn't provided them. Then, it writes a header to the terminal output so it's clear what the output is about.
After that, it retrieves the user's usage information with the getUsageData()
method, covered next, and renders it in tabular form. I know some people aren't a fan of tables, regardless of where they're used. But I believe — when used appropriately — they make information easier to scan, allowing it to be used more efficiently.
Finally, Command::SUCCESS
(0
) is returned, showing that the command completed successfully.
The getUsageData()
method invokes the TwilioUsage
object to retrieve the user's usage information, and then formats some of the call information while storing it in an array. The intent is to filter out the usage properties that won't be displayed and make the currency information easier to read.
Create the command container
There's one final class to create, the one that allows the Symfony custom command to be used. In the project's top-level directory, create a new file named application.php. Then, in that file, paste the code below.
This class is a container for a collection of commands. In this case, only one command is defined. It connects the command with the user input and allows it to write output to the terminal.
There's not a lot going on, but it's still worth stepping through. It uses PHP Dotenv to put the environment variables defined in .env into PHP's $_ENV and $_SERVER superglobals.
Then, it initialises a new TwilioUsage
object, an Application
object, and adds an instance of the custom command, TwilioUsageCommand
, to the application. After that, the Application
object's run()
method is called to run the application, so that the command can be called.
Add a PSR-4 Namespace
The last thing to do is to add a custom PSR-4 Autoloader so that the two classes that you have created can be autoloaded when required. To do that, update composer.json to match the configuration below.
Finally, add update Composer's autoloader, by running the command below.
Test the command
Now that the code's ready, it's time to test it. To do that, run the following command in the terminal:
You should see output similar to the following.
Now, try out the options to see how the output changes. This time, use the --limit-records and --category options, as in the following example:
This time, you should see fewer records, similar to the output below.
Finally, experiment with the --start-date
and --end-date
options. You can use any date that PHP's DateTime::format() function supports. Here's an example:
That's how to build a command line application to view your Twilio account usage
Symfony's Console Component makes it trivial to create console commands in PHP — so much so that it's the de facto package for creating console commands, used by Laravel, laminas-cli and so many other frameworks.
I hope that this tutorial has shown you two key things:
- How quick they can be to create
- How they help you interact with Twilio's APIs from the command line just as readily as you do within your web-based applications.
Check out the Usage Records documentation to see what else you can do with the API, and have fun playing with the code.
I can't wait to see what you build!
Matthew Setter is a PHP Editor in the Twilio Voices team and (naturally) a PHP developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. When he’s not writing PHP code, he’s editing great PHP articles here at Twilio. You can find him at msetter[at]twilio.com, on LinkedIn, and on GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.