Build an English to Shakespearean Translator using SMS and PHP
Time to read: 3 minutes
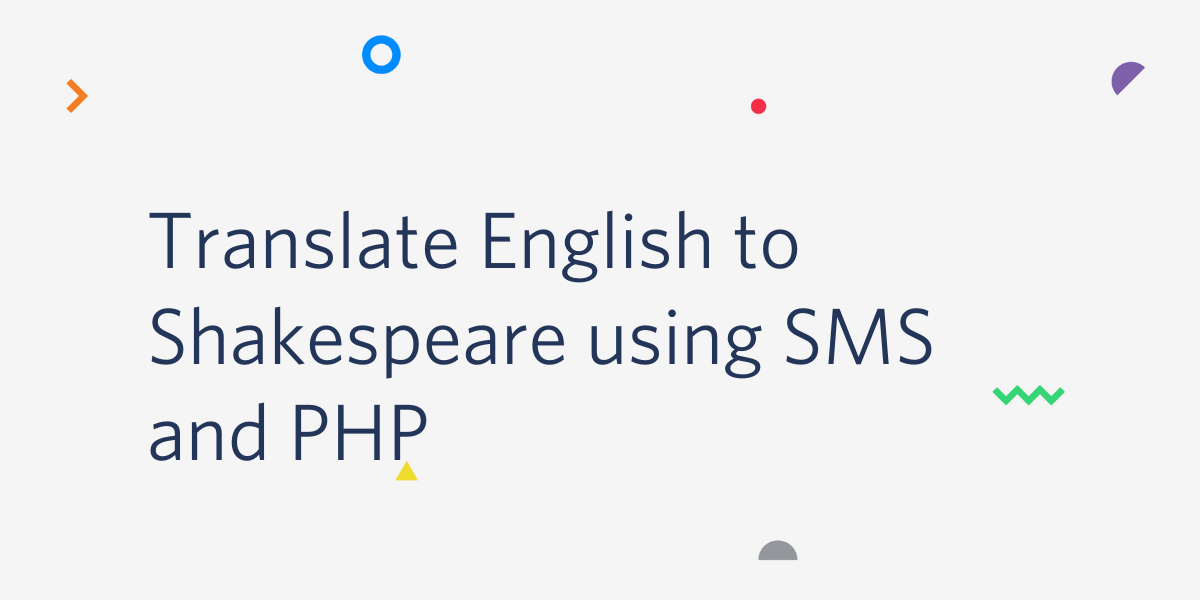
Technical Requirements
To complete this tutorial, you will need the following dependencies globally installed on your computer:
- PHP 7 development environment
- Global installation of Composer
- Twilio Account
- FunTranslations Shakespeare API
- Global installation of ngrok
Set Up Our Development Environment
To kick start our project we will need to create a project directory for it. You may use Shakespeare
as this is what I will be using too. In the folder create the following files:
- .env
- webhook.php
- functions.php
Next, we need to set up our .env
file.
NOTE: The .env
file is a hidden file we create on our servers to store secret and private keys. It is not accessible by the browser, so we can store our API keys or credentials in it and access it from anywhere within our app on the server-side.
To allow the support of .env
configs in our app, we need to install a package using Composer. Run this command in your terminal in the project directory:
We also need to install one more package from Twilio. There are many libraries that enable us to access the Twilio API more efficiently than traditional cURL requests. For this project, we need to install the Twilio PHP SDK. Run the following command in your terminal in the project directory.
Setup Twilio Credentials & Phone Number
If you have not already done so, take a few minutes and head to your Twilio account to create a new number. We need to get the following information from the Twilio console to allow us to send and receive SMS from our project:
- Twilio Account SID
- Twilio Auth Token
- A Phone Number
Once we have these items, we can add them to our .env
file.
Create a Function to Translate our English SMS into Shakespeare
To translate our English language SMS into Shakespeare, we are going to leverage the FunTranslations API service by creating a function for it.
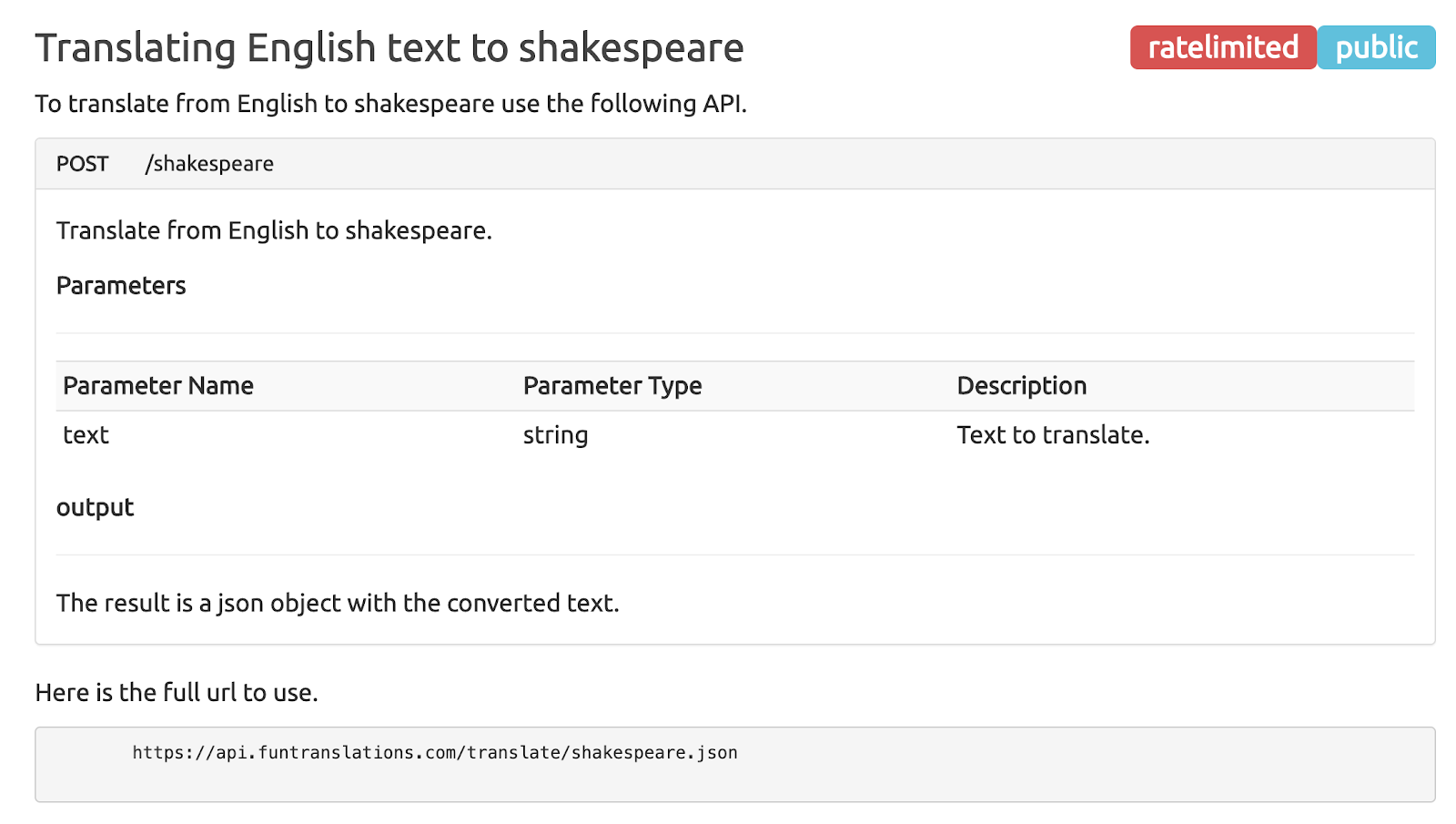
Update you .env
file with FunTranslation’s API URL like so:
The functions.php
file we created earlier will contain the function that will take the user’s SMS, process it, and send it to FunTranslation’s API. Add the following code to the functions.php
file.
Create a Twilio Webhook
To receive SMS translation requests from users, we will need to create a Twilio webhook. Twilio has a webhook feature that sends a response to an endpoint whenever your phone number receives a message.
We will need to create our own webhook to receive translation requests from users. In the webhook.php
file, copy and paste the following code into it.
We will now use Ngrok in order to make this webhook accessible through a public URL from our localhost.
From the terminal, run the command:
On a new terminal window, run the command:
Your terminal window should look like this:
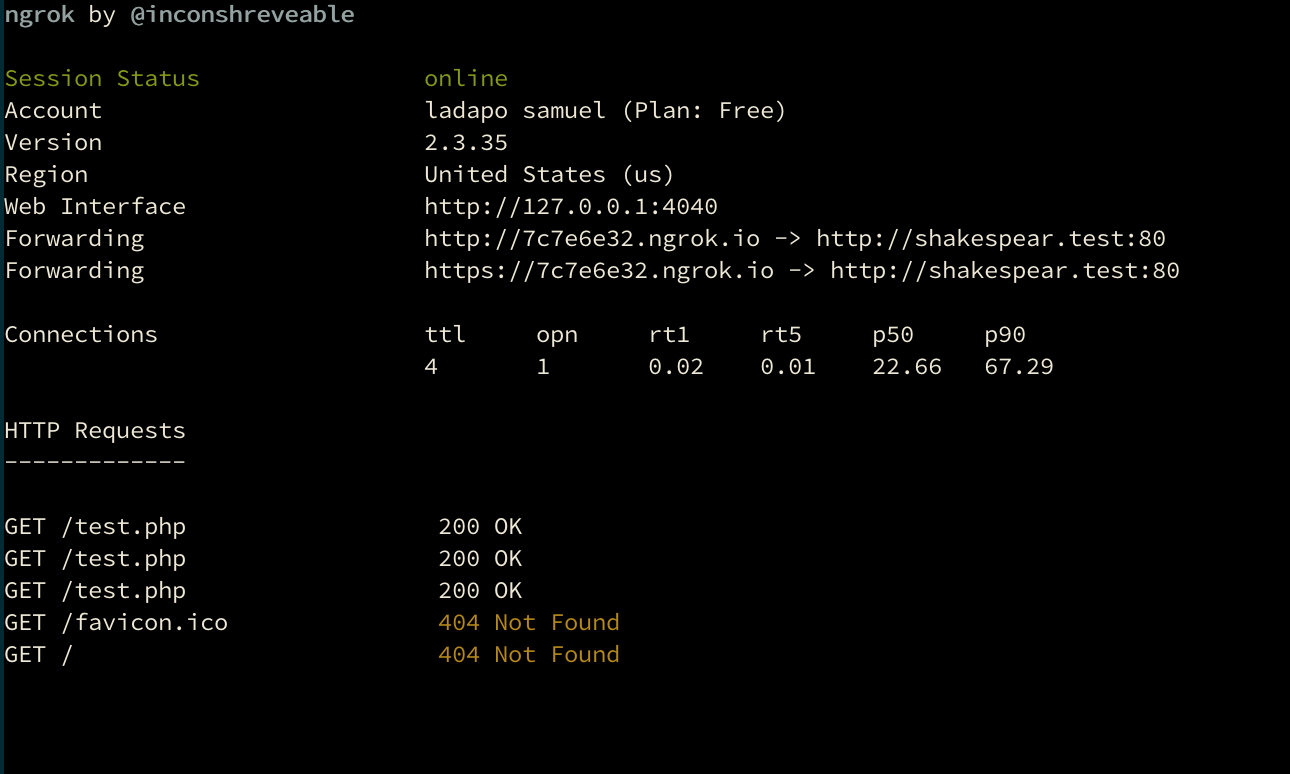
In our Twilio project, we will provide the webhook URL above, followed by the path to our webhook file. For example, mine is:
The final step is hooking our Twilio number to the webhook we created. Navigate to the active numbers on your dashboard and click on the number you would like to use. In the messaging section, there is an input box labeled “A MESSAGE COMES IN”. Replace the URL with our webhook URL and click “Save”!
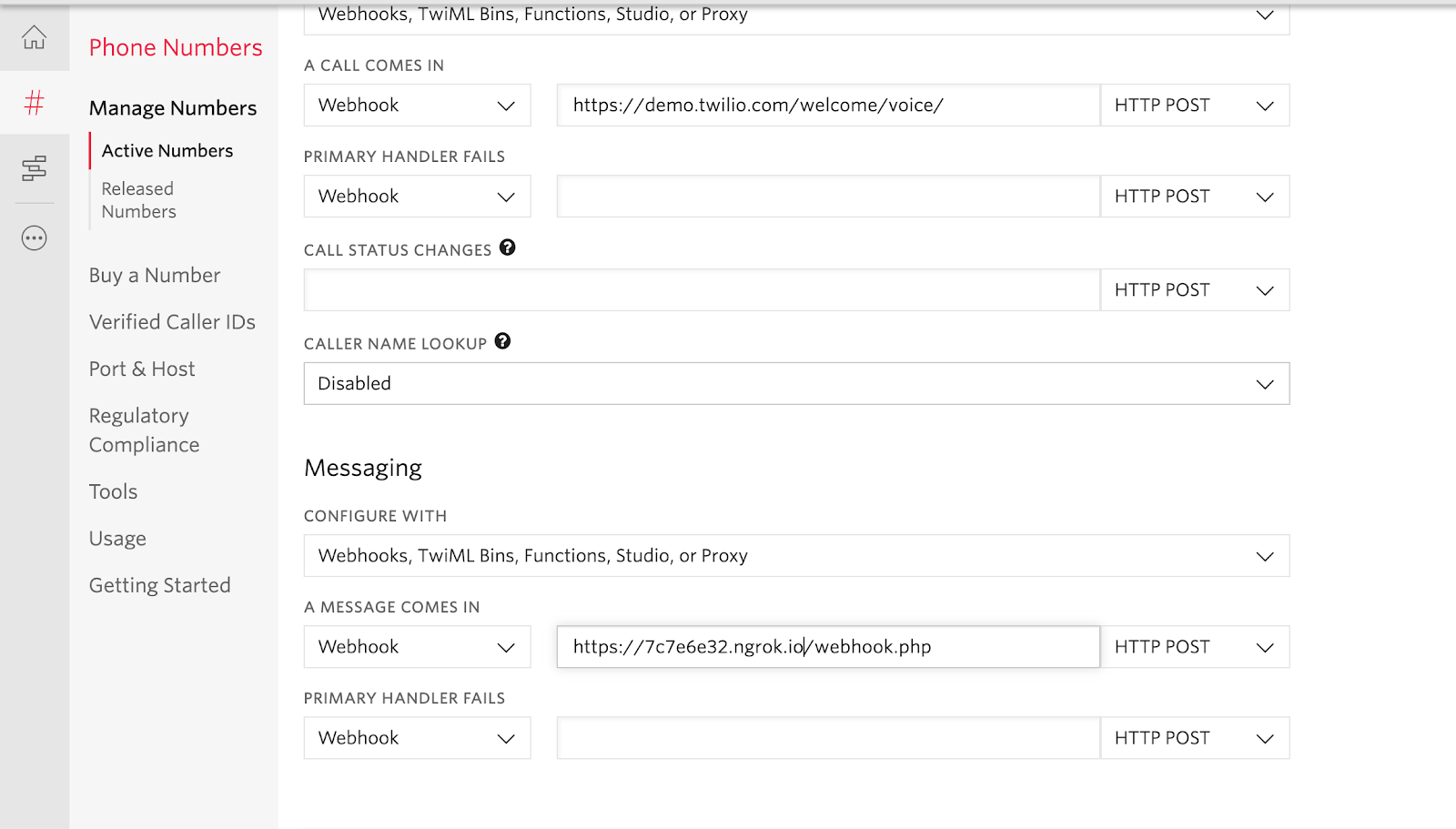
Testing Our App
Send I want to drink water
for example to your Twilio number to get its translation to Shakespeare.
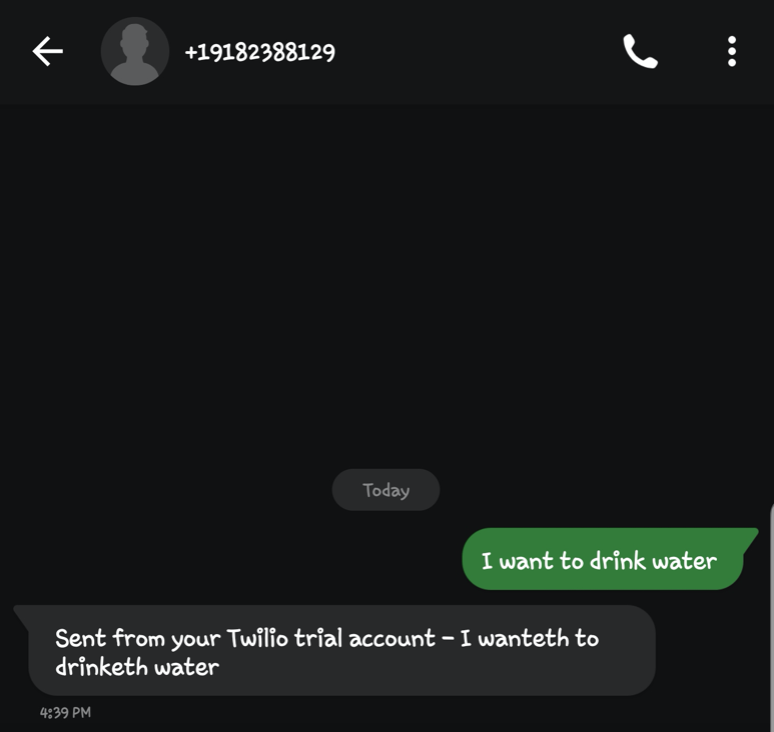
Conclusion
Good job! Now we can translate the English language to Shakespeare using SMS. You can implement other fun translations like translating to minions language.
Bonus
The Github Repo is available for a quick start: https://github.com/ladaposamuel/english-shakespeare
Additional Reading
If you would like to learn more about any of the technologies mentioned within this tutorial, check out the links below:
Ladapo Samuel
Email: ladaposamuel@gmail.com
Twitter: @samuel2dotun
Github: ladaposamuel
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.