How to Call the Twilio API from Java Programs on the Commandline
Time to read:
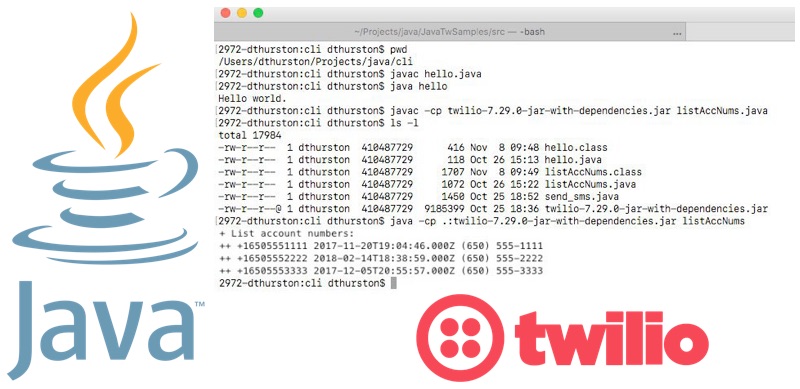
If you you need to do a quick Twilio test, or compile and run programs on a remote computer without any GUI, or run test programs on your own computer and you don’t want to use a full blown IDE development environment, this is the article for you.
Check Java Installation
Confirm that you have a Java runtime environment (JRE) on your computer for running Java class files:
If you don’t have both, please go to Oracle’s Java SE page and download the Java JDK.
The Java Standard Edition (Java SE) is what you need for developing and running Java programs from command line. Java SE has a Java Development Kit (JDK) which contains a Java Virtual Machine (JVM), the runtime environment (JRE) for running Java programs, and the compiler (javac).
Create and Run a Hello World Program
Create a working directory, for example: /projects/jtwilio
. Before trying a Twilio request program, create and run the Hello World program, hello.java
. This will confirm your environment is setup and working.
Compile the file into bytecode using the javac
command.
Run the program using the JVM. The JVM is an interpreter that reads and runs the hello.class
file.
You now have a tested, working Java environment to compile and run Java programs. Next, is to integrate the Twilio Java helper library into your compilations and run the programs using the helper library.
Create and Run a Twilio API Request Java Program
Download the Twilio helper library JAR file into your working directory. JAR stands for Java ARchive file. Essentially a JAR file is an archive zip file. Go to the repository directory and download the recent helper JAR file. At the time of writing the most recent JAR file is 7.29.0.
Create a program to list your Twilio account phone numbers: listAccNums.java
.
You can view the contents of a JAR file using the jar
command. You could rename the JAR file to have the extension, “.zip” and unzip the file to see the contents. But the jar
command is much easier.
Note, in the above listing, is the file: IncomingPhoneNumberReader.class
, which is imported into the sample program: listAccNums.java
.
Compile the program. List the class file program. Run the program.
The compile command includes the Twilio helper library JAR file. The run command includes the Twilio helper JAR file, and the current directory: “.” which his where the class file listAccNums.class
is located. “-cp” is the short form for “-classpath”.
Optionally, create a scripts for compiling and running your Twilio Java programs. Put script in your PATH for ease of execution. Following are my UNIX script examples: jc
for compiling, jr
for running.
Test the scripts by re-compiling the account phone number list program and re-running it.
With jc
and jr
, you have an easy way to compile and run Twilio Java programs.
If you are using an IDE, and you need a quick method to add the Twilio Java library, without using Gradle or Maven, you can download the library JAR file and include it in your IDE’s project. The following screen print shows the JAR file included in my NetBeans project. The same approach will work with Eclipse.
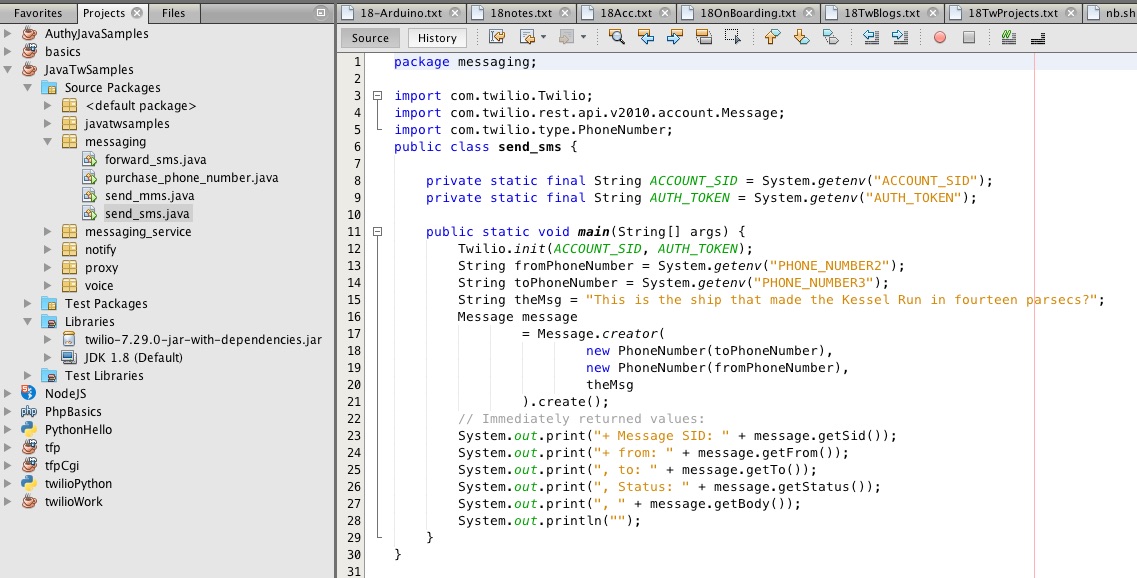
Summary
There you have it, a quick methods of running Java programs that use the Twilio Java helper library to make Twilio REST API requests, using a minimum amount of setup. This is handy for quick testing and managing programs on remote computers where command line is the only option.
To help you get started writing Twilio Java programs, take a look at these set of programs I recently created based on the Twilio documentation.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.