How to Check your Twilio Account Balance in Python
Time to read: 3 minutes
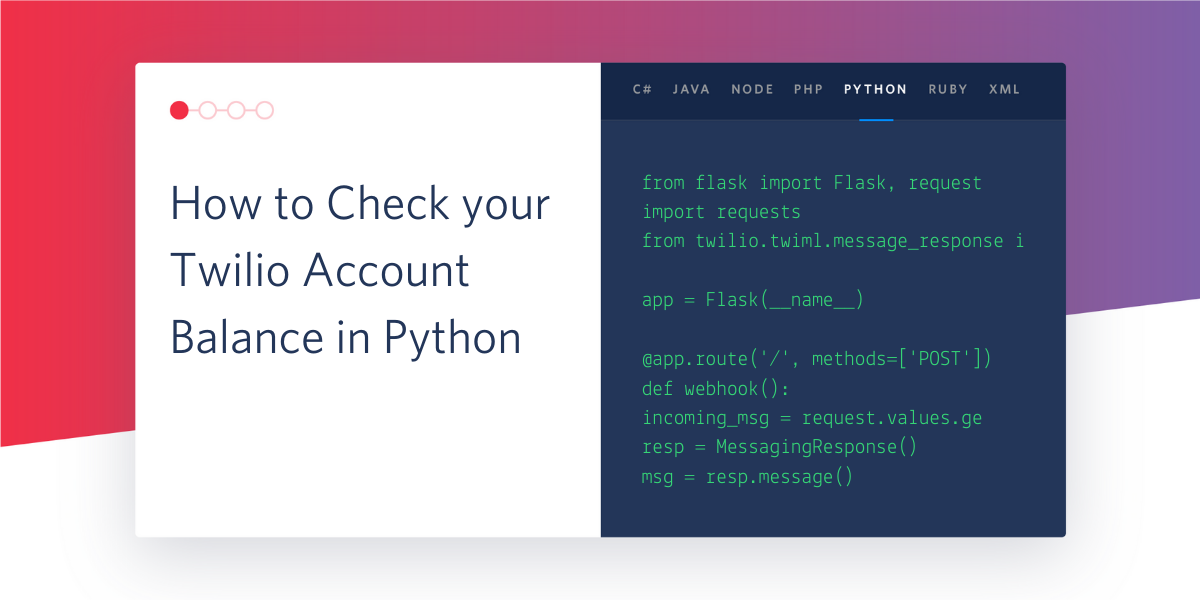
Twilio APIs make it easy to add communication features to your application, but to consume these APIs you have to keep track of your spending and ensure your account balance stays above zero.
Your balance is available in the main page when you log in to your Twilio Console, but having to actively monitor your account is tedious and inconvenient. In this tutorial you are going to learn how to retrieve your account balance using Python, which will allow you to build automated account balance monitoring within your application!
Prerequisites
To follow this tutorial you will need:
- Python 3.6 or newer. If your operating system does not provide a Python interpreter, you can go to python.org to download an installer.
- A free or paid Twilio account. If you are new to Twilio get your free account now!
Creating a Python Project
Let’s begin by creating the directory where you will store this project. Open a terminal window, find a suitable parent directory, and enter the following commands:
Following best practices, you are going to create a Python virtual environment where the Python dependencies will be installed.
If you are using a UNIX or macOS system, open a terminal and enter the following commands to complete the tasks described above:
For those of you following the tutorial on Windows, enter the following commands in a command prompt window:
The pip
command installs the Twilio Python Helper library which is used to access Twilio APIs.
Configuring the Twilio Client
If you already have an application built with the Twilio Python Helper Library, you know that the client uses your Twilio Account SID and Auth Token to authenticate, and likely have this configured. If you are building a new application as part of this tutorial, these need to be configured.
Log into your Twilio Console and you will see your Account SID and Auth Token values assigned to your account in the dashboard:
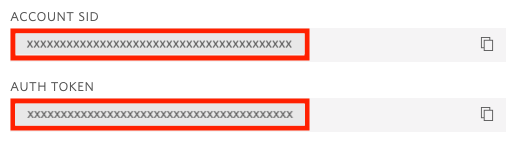
Back in your terminal window, set two environment variables with the names TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
to these two values. Use the "copy" buttons on the right to transfer the values easily and without making any mistakes. If you are using a macOS or UNIX computer, do it as follows:
If you are doing this on a Windows computer, you can set your variables as follows:
Retrieving your account balance
Okay, now you are ready to retrieve your Twilio account balance using Python! To begin, you can do this in a Python console. Start it by typing python
on the same terminal session in which you set the environment variables:
Then import and initialize the Twilio client:
Note that the Client()
class expects the TWILIO_ACCOUNT_SID
and TWILIO_AUTH_TOKEN
environment variables. If you forget to set these, the client will not initialize and instead show an error message.
The client instance can get your account balance as follows:
Note that the amount is returned as a string. It would be more useful to have it as a number, so the result can be converted right after it is retrieved:
You probably know what currency is used in your account, but if you need to retrieve that as well, you can do it as follows:
Let’s now integrate this into a simple script that can be executed directly from the command line. Open your favorite text editor and create a file called twilio_balance.py inside the twilio-balance directory. Enter the following code in this file:
Save the file, and then run it from the same terminal you used above, which has the Twilio credentials stored as environment variables:
Conclusion
And that is it! You can now incorporate this little bit of logic into a larger application to check your balance as often as you need. You can even send yourself a notification if the balance is lower than a specific amount.
I hope this improves your experience using Twilio APIs. I can’t wait to see what you build!
Miguel Grinberg is a Python Developer for Technical Content at Twilio. Reach out to him at mgrinberg [at] twilio [dot] com if you have a cool Python project you’d like to share on this blog!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.