Making Your Inbox Intelligent with Context.IO and Twilio
Time to read: 5 minutes
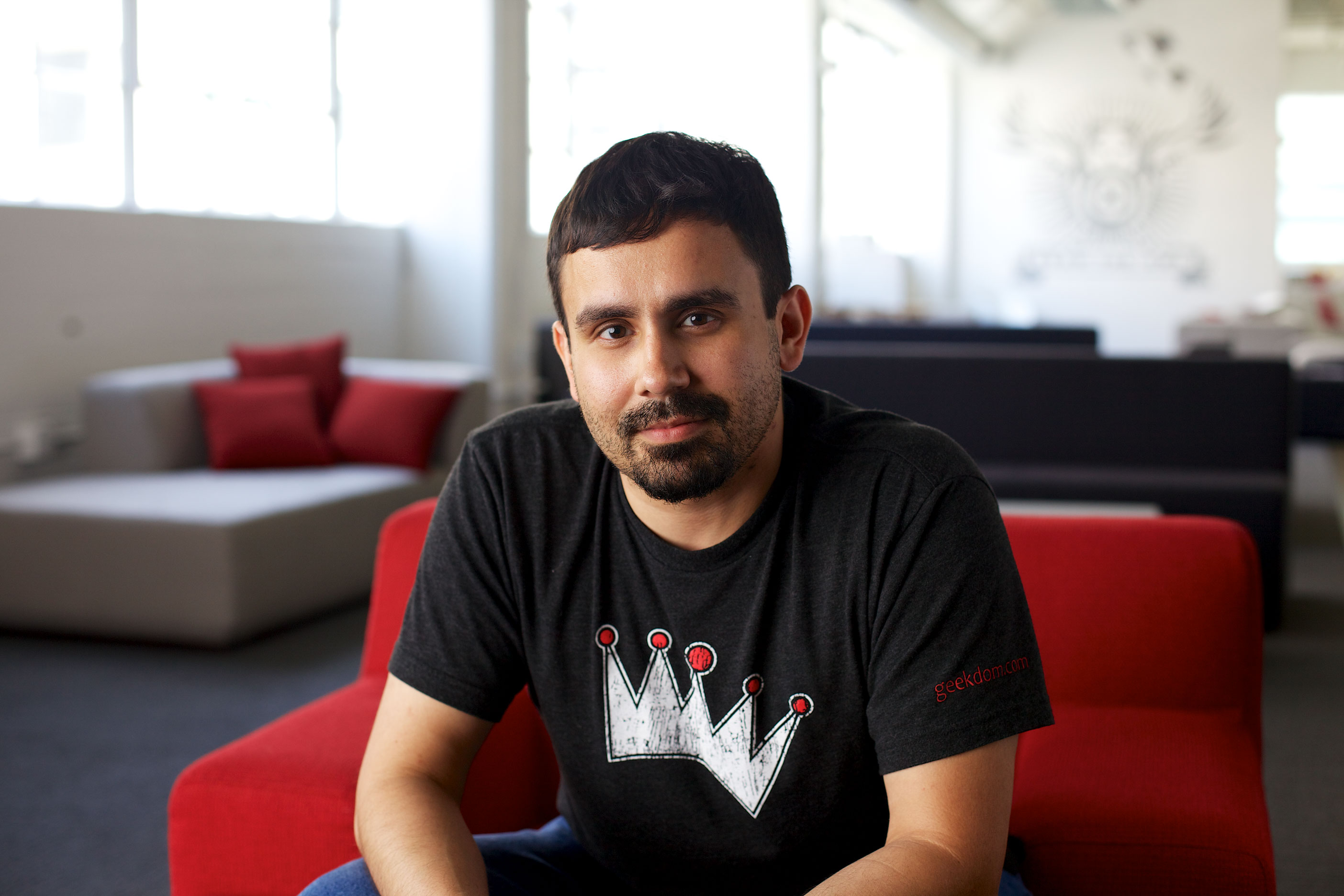
Email is broken. We get too much of it. We get irrelevant stuff. Reply-all is the default and CC is the norm. Even worse, smart phones constantly interrupt our day with every single message with almost no intelligence involved. How do we sort through the email and make sure we get the important information in a timely manner in a way we can use? Recently I discovered Context.io API and saw a light at the end of the tunnel.
In brief, Context.io is an API for your email inbox. After creating an account and synchronizing it to your account, you can retrieve any email, sort through them by date, sending, content, or a variety of other things. More importantly, you can create webhooks to trigger events as things occur within your inbox. Just like Twilio, they abstract the complicated details away under a much simpler interface. More importantly, by combining Twilio and Context.io, you can have an intelligent inbox that enables you to filter out the important details and ignore the rest.
Configuring Your Webhook
Let’s start simple by creating a text notification whenever we get a new email. Yes, any smart phone will show a notification for a new message, but let’s walk before we run.
All of the following examples make heavy use of Context.io’s webhooks. To create your first webhook, create an account with Context.io, visit their web console, and connect at least one email account. By default, you only need a callback_url and a failure_notif_url. We’ll discuss the rest of the options later.
These webhooks work exactly like the Twilio Voice and SMS Urls. When a new email comes in, Context.io will perform a HTTP POST including a payload with information about the message. The full payload is detailed in their documentation but the primary fields of interest are the account_id and message_id. We can retrieve both with a simple PHP snippet:
SMS Notifications
For our first revision, we’ll use the Twilio PHP Helper library to generate the TwiML as seen here:
As seen above, this file receives in incoming payload and parses out the name of the sender and the subject line. We concatenate these together and generate a text message from our Twilio phone number to our cell phone. Now when I send a message to myself, I get a text message like this within a few moments:
Combining Email with SMS
Now that our proof of concept is complete, we’ll make the messages more useful. While Context.io’s webhooks include the message_id and all of the email participants in the payload, it does not include the body of the message itself. To get this, we’ll have to make a second API call:
This request uses the message_id from the payload to request the entire body of the message. We can get the body in either html or plain text, but in this case we’ll get the plain text. It will be the easiest way to embed in a text message.
While the message body includes file attachments, we’re not going to use them in these examples. One area for improvement is to use a short code to send those attached images via our MMS-based Picture Messaging.
Once you combine the body retrieval with the previous script, you come up with this:
Now when I send myself a test message, I get this text message within a few minutes:
Keith Casey just emailed:
This is my test subject line
This is the body of my message.
—
Keith Casey
Sr Developer Evangelist – Austin, TX
Find me at an upcoming event here:
http://bit.ly/trackDKC
You’ll notice that this includes the signature from the email itself. One area for improvement would be to parse the message and remove the signature. This will be challenging at best unless you figure out how to cheat. We’ll cover that in our last segment.
Email By Phone
As long as we are including the body of the message in a text message, we can just as easily convert this to placing a phone call. In this case, we’ll use TwiML to generate a series of Say verbs to read each portion of the message. The first script looks much like the SMS script from earlier:
On the other side, to generate the TwiML, we do much the same as how we created the SMS. First we get the message_id, retrieve both the message header and body information, and then put it all together with the Say:
While this example uses phrases to separate each of the segments, a better approach may be to use the different Twilio voices. It would give a clear distinction with minimal effort.
Manage You Inbox Via Phone
Of course now that we can receive our messages via voice or text, the next logical step is to allow you to manipulate them and a good parallel is voice mail. In our case, we’ll implement a way to delete the message with the default action being to keep the message.
In this case, we’ll replace our original Say structure with Gather to collect the input of a single key:
And finally, we take that input and make one final call to the Context.io API to actually delete the message:
Advanced Webhooks & Callback Filters
Our final step goes back to the beginning with our webhooks. At present, the above scripts will generate a text message or voice call for each and every message received. To put it simply, that’s not scalable on one’s sanity. To make this a little more selective and therefore powerful, we’ll go back to the Context.io webhooks console and dive into the optional parameters.
By using the optional parameters, we can add functionality and even simplify what we’ve already written. For example, by setting “include_body” to 1, we can get the body in the original payload and completely eliminate our second API request. By using the filter_* parameters we can limit the webhook calls to messages to or from certain addresses, only those that have files, or even those that have files with specific names. At this point, we’re really only limited by the complexity of the rules we want to build.
This is also where our cheat from above comes into play. While detecting and removing everyone’s email signature is challenging at best, if you’re only forwarding messages from certain people, you can limit your parsing to address those specific cases.
While the above scripts take into account a number of basic scenarios, these scripts could easily be expanded to support moving messages between folders, archiving emails, or even using Twilio’s transcription to draft responses. Once you ponder text and voice as another user interface, a number of things become possible. If you take these scripts further, I’d love to hear about what you build and how.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.