How to convert audio to different formats using C# and FFmpeg
Time to read: 3 minutes
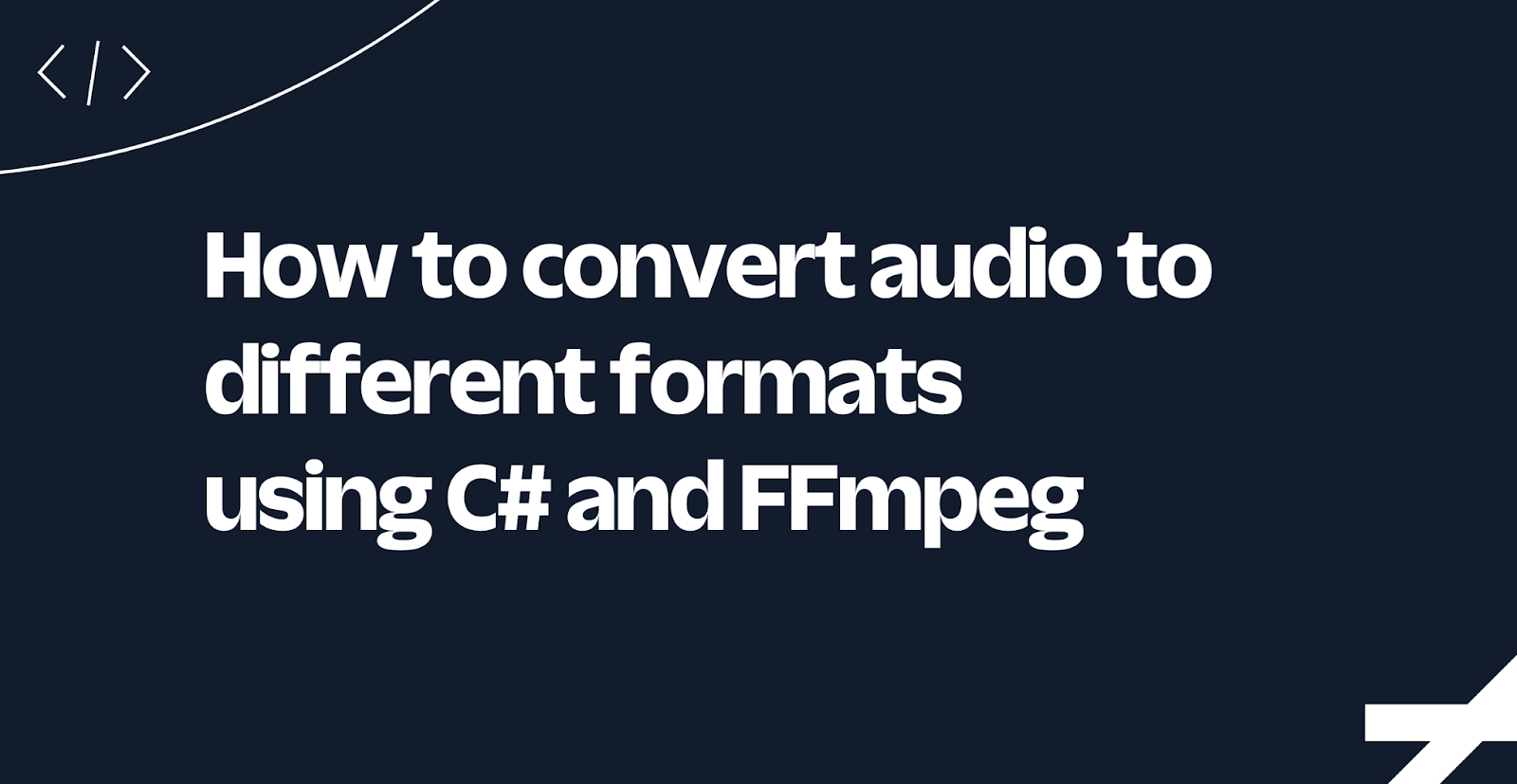
Working with audio and video can be a very daunting task if it's not your area of expertise. Luckily, there are some great tools that help you achieve difficult tasks with little media manipulation experience. In this tutorial, you'll learn how to use one of these tools, FFmpeg, to transcode audio from one format to another from a C# .NET application.
Prerequisites
Here’s what you will need to follow along:
- .NET 7 SDK (earlier and newer versions may work too)
- A code editor or IDE (I recommend JetBrains Rider, Visual Studio, or VS Code with the C# plugin)
You can find the source code for this tutorial on GitHub. Use it if you run into any issues, or submit an issue if you run into problems.
Set up FFmpeg
FFmpeg is a command-line interface (CLI) tool capable of decoding, encoding, transcoding, muxing, demuxing, streaming, filtering, and playing a large variety of media. FFmpeg is open source and supported across platforms.
To use it, you'll first need to download and install the binary. You can find some options to download FFmpeg for your operating system on the FFmpeg download page, but I'd recommend you check if FFmpeg is available in your preferred package manager and install it that way.
If you use a package manager, it's likely already installed in your system path. Verify your FFmpeg installation by running the following command:
You should see output that looks like this:
If you don't see output similar to this, locate the folder where the ffmpeg
binary is stored, and add the path to that folder to your PATH
variable.
While FFmpeg is capable of accomplishing many different tasks, however this tutorial will focus solely on changing audio from one format to another. For example, run the following command to download classic.mp3 and convert it to a wav-file:
The -i
options told ffmpeg
where to find the input file, which was then followed by the output file; ./classic.wav on your disk. FFmpeg can detect the input file's format by analyzing it, and knows the format to transcode to because of the output file's .wav file extension. Enjoy the song, it's one of my favorites! 🕺
Convert audio files to different formats from .NET
Let's see how you can achieve the same task from .NET.
First, create a C# command-line project using the .NET CLI:
While you could interact with FFmpeg using the Process APIs or libraries like CliWrap, there's already a very helpful library that wraps the ffmpeg
CLI for you: FFMpegCore.
Add the FFMpegCore NuGet package to your project using this command:
Now open the project in your preferred .NET IDE and update the Program.cs file with the following C#:
This code will do the same as the ffmpeg
command you executed before, but the library makes it a lot more readable and self-explanatory.
Run the project using the .NET CLI:
After running the project, the classic.wav file should appear in your project directory.
Awesome work!
Convert audio streams to different formats
If you don't want to pull the media directly from a URL or disk, you can also use FFmpeg's pipes to pass in data and receive data back without network or file I/O.
Let's recreate the same functionality using the FromPipeInput
and OutputToPipe
methods instead of the FromUrlInput
and OutputToFile
methods, by updating Program.cs as follows.
The code above opens a network stream, audioInputStream
, to stream the audio from the internet, and creates a file stream, audioOutputStream
, to write the audio to. As you aren't passing an output file name directly to FFmpeg, FFmpeg cannot deduce the desired format, so you need to tell FFmpeg to use the wav
format using options.ForceFormat("wav")
.
Run the project again:
The result should be the same. So what's the big deal? Well, if the default FromX
and OutputToX
methods don't fit your needs, using Stream
's gives you the ultimate flexibility to provide input to FFmpeg and where to write the output to.
Next steps
You've just scratched the surfaces of what FFmpeg is capable of. There are a ton of extra arguments and capabilities to manipulate media files.
When building Twilio applications, FFmpeg can be a very useful tool too, for example, here's a tutorial that transcribes audio files sent through WhatsApp using the OpenAI Whisper API, but the Whisper API doesn't support the .ogg format that WhatsApp uses, so FFmpeg is used to convert the audio to a wav-file before the audio is passed to the Whisper API.
We can't wait to see what you build. Let us know!
Niels Swimberghe is a Belgian American software engineer and technical content creator at Twilio, and a Microsoft MVP in Developer Technologies. Get in touch with Niels on Twitter @RealSwimburger and follow Niels’ personal blog on .NET, Azure, and web development at swimburger.net.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.