Create an Artisan Command to View Twilio Calls
Time to read: 4 minutes
Laravel's Artisan Console provides a wealth of helpful commands to assist with all manner of tasks when building and managing Laravel applications. It can clear the application and configuration cache, create all manner of classes, generate the application's key, manage database migrations, and so much more.
In this tutorial, you're going to learn how to create a custom Artisan command to list the voice calls you've made on your Twilio account and print the call information in tabular form.
Prerequisites
To follow along with this tutorial, you will need the following:
- PHP 7.4 or higher.
- A free or paid Twilio account. If you are new to Twilio click here to create a free account.
- Composer installed globally.
Create a new Laravel project
The first thing to do, if you don't already have an existing Laravel application, is to generate a new one using Composer. To do so, run the following commands to create one and change into the new project's directory.
Add the required dependencies
While Laravel comes with a wealth of dependencies, you will need to add two more. These are:
- The Twilio PHP Helper Library, to simplify interacting with Twilio's APIs
- Money for PHP, to simplify formatting currency information rendered in the console
Run the following command in the current directory to install them.
Retrieve your Twilio credentials
The next thing to do is to make your Twilio credentials (your Twilio Account SID and Auth Token) available to the application. You're going to make use of Laravel's existing environment variable functionality to make life easier.
Start off by pasting the code below at the bottom of the .env file, in the project's top-level directory.
These add an environment variable for each of the required values.
Next, from the Twilio Console's Dashboard, copy your Account SID and Auth Token and paste them in place of the respective placeholder values (<TWILIO_ACCOUNT_SID>
and <TWILIO_AUTH_TOKEN>
) in the .env file.
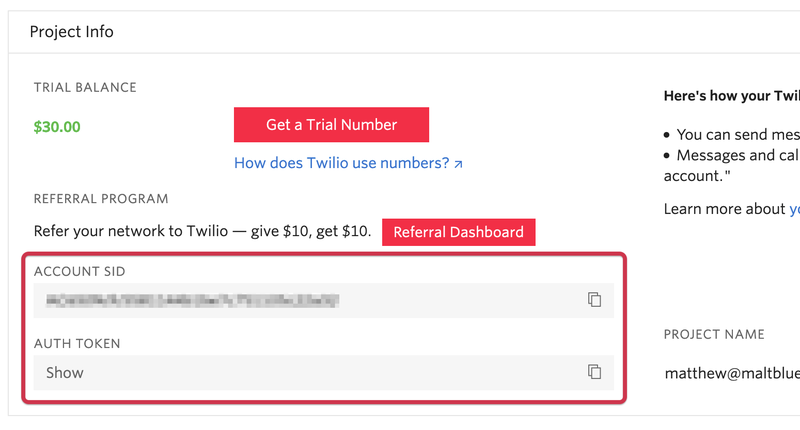
Generate the new Artisan command
The next thing to do is to generate the stub of the Artisan command, using Artisan, by running the command below.
Then, in the new Command
class (app/Console/Commands/TwilioListCalls.php) replace the code with the following.
The class starts by defining two date constants. These are used depending on whether the user chooses to view the call information in short date format or not, using an option in the command signature.
It then defines the command signature and description. The signature is split into two parts: the command's name, twilio:list-calls
, and the sole supported option --short-date
.
The handle method
After that, it defines the handle()
method, where the core business logic is defined. The method starts by instantiating a Twilio Client
object, from which all interaction with the Twilio APIs are made, passing to its constructor the two Twilio environment variables defined earlier.
Then, it retrieves all calls on the user's account by calling the call
property's read()
method. If the method throws a \Twilio\Exceptions\EnvironmentException
, an error message is printed to the terminal and the command returns a non-zero status code to indicate that the command completed unsuccessfully.
If successful, it does one of two things.
- No calls were returned? It prints a message to the terminal saying that none were available.
- Calls were returned? some of the returned call properties(shown in the table below) are then stored in an array (
$callDetails
).
Property | Description |
---|---|
sid | The call's unique id. |
to | The phone number, SIP address, Client identifier or SIM SID that received this call |
status | The call's status. The value can be one of queued, ringing, in-progress, canceled, completed, failed, busy, or no-answer. |
startTime & endTime | The time that the call started and ended in RFC 2822 format. |
price & priceUnit | The amount that the call cost the user. |
For easier readability, the created date, and start and end times are formatted using the applicable date format constant, and the call price information is formatted in the formatCallPrice()
method, which you'll see shortly.
The collated call information is then printed in tabular format using the handy table method. The information is preceded by a header, telling the user that call information is available and a footer showing the total number of calls. Following that, 0 is returned to indicate that the command completed successfully.
The formatCallPrice() method
The class finishes off by defining the formatCallPrice()
method. This method converts the call's price information (a string) into a float representation, then converts that to a value in cents.
Following that, it uses a combination of three classes, Money
, NumberFormatter
, and IntlMoneyFormatter
to render the amount as an international currency string, e.g., $0.33
, which is easier to read than the underlying value, e.g., -0.330000
.
At this point, the new command is ready to use. You don't have to register it as that's automatically done for you by Laravel.
Test the new Artisan command
So, it's time to test that it works. To do that, run the following command.
You should see output similar to the following in your terminal.
Now, run the following command to view the same output, but with shorter dates.
You should see output similar to the following in your terminal.
That's how to create an Artisan command to view Twilio voice calls
You might have been expecting to write more code to create a custom Laravel command. However, with the official Twilio PHP Helper Library and Artisan itself, there was very little code required.
Over the next few weeks, I'll be showing how to create a number of helpful custom Artisan commands for interacting with your Twilio account, so stay tuned.
Till then, happy hacking! I can’t wait to see what you build with the Twilio APIs.
Matthew Setter is a PHP and Go editor in the Twilio Voices team, and a PHP and Go developer. He’s also the author of Mezzio Essentials and Deploy With Docker Compose. You can find him at msetter@twilio.com. He's also on LinkedIn and GitHub.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.