Create a Contact Form in PHP with Laravel and Twilio SendGrid
Time to read: 2 minutes
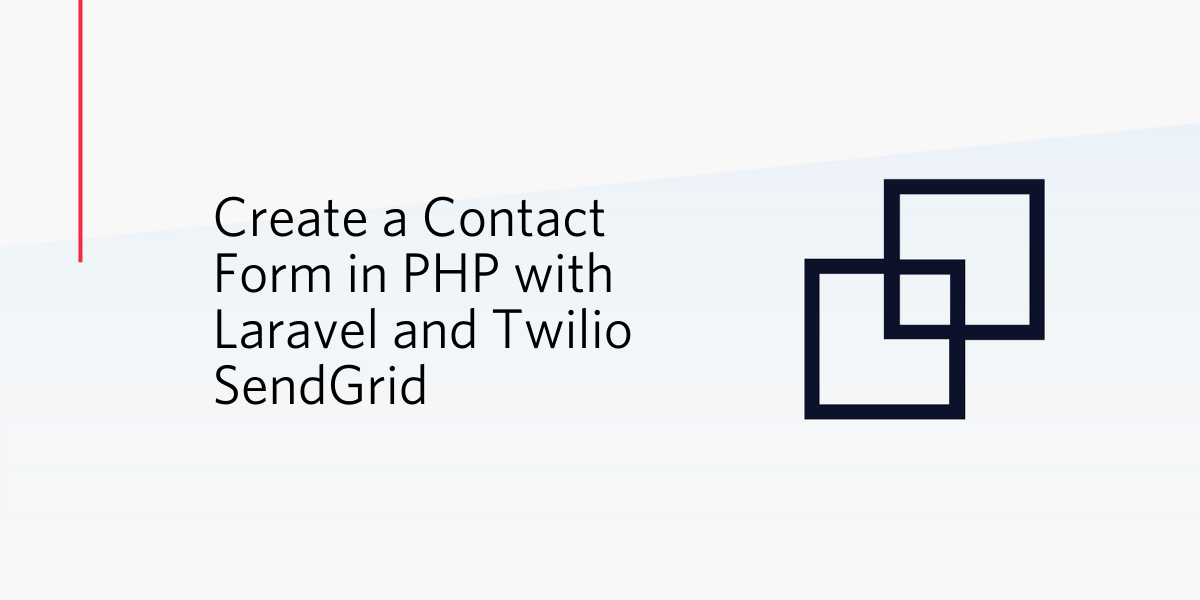
Rolling out your own email solution from scratch can prove to be challenging due to issues such as avoiding being flagged as SPAM or scaling to many users. Luckily, APIs such as Twilio SendGrid exist to fix deliverability and scale when implementing email-based solutions.
If you are interested in sending out newsletters to users, email notifications for admin related actions, or also keeping track of successful and failed email triggers keep reading. Throughout this guide, we will be focusing on dispatching emails to various end-users. To achieve this, we will be using Twilio SendGrid and Laravel to dispatch emails to various recipients. Once completed, you will have built an email form that can be incorporated into your Laravel application.
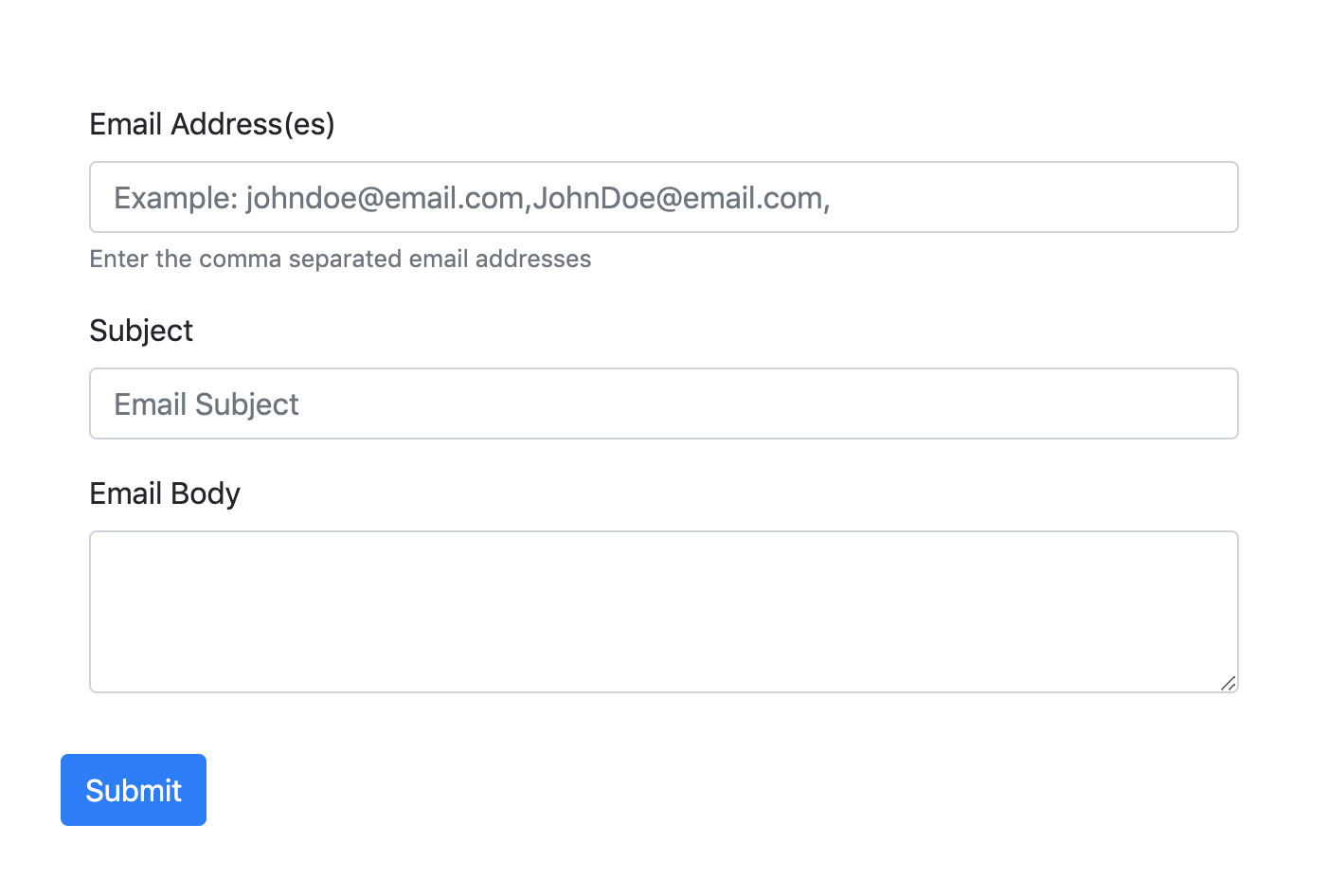
Installing dependencies and requirements
To complete this tutorial, we need to install all of the dependencies required to send emails using SendGrid and Laravel.
You should have the following modules/packages installed:
Once you have Laravel installed on your machine, you can proceed to create a new project using the Laravel CLI:
Or using the Composer create-project
command:
Next, we will need to install the SendGrid package to be able to send and manage emails. To achieve that, we will need to use Composer to install the dependency with the command below:
Once installed, make sure to set your SendGrid API credentials in your .env
file as that will be used for authenticating the email requests:
NOTE: Your SendGrid API Key can be found here.
After all of these configurations have been set up, we need to create a controller called EmailController to house the code for the email functionality. e can achieve that using this command:
In case you’re not familiar with Laravel, all controllers are found in the app/Http/Controllers
directory.
Now let's make our controller aware of the SendGrid email module by requiring it in the class:
Creating the view
We need to create a view where we can test this code to make sure it works as expected and returns the expected results. We will create a view in our resources/views/
directory called send-email.blade.php
. Add following code:
Trigger the mail function
Now that we have created the views, we need to write the function responsible for triggering and dispatching the email to the various email addresses. In the EmailController.php
file, add this method to enable the email dispatch. That should look like this:
NOTE: Don’t forget to replace the “SENDER_EMAIL” and “SENDER_NAME” values with the correct entries.
Now we need to create the routes needed to call the controller methods for email dispatch.
Creating the routes
In the web.php
file located in the routes
directory, we will add the following route to return the view and hence, trigger the sendEmail()
function when called.
Here is the complete EmailController:
Testing
To see the application in action, all you need to do is start up your development server using the command below in the terminal of your local machine.
Finally, using the web browser of your choice, visit http://localhost:8000. Fill in the form and submit your message.
Conclusion
You should now be able to use SendGrid to send emails with so much ease. Now that you have finished this tutorial, you could extend the application by adding a MySQL database to store users’ information, programmatically retry failed email triggers, and also send emails to a group of end-users.
You can always reach out to me using any of the following channels if you need assistance with anything related to this topic. I would love to see what you are building.
Ugendu Martins Ositadinma
- Email: ugendu04@gmail.com
- Twitter: https://twitter.com/ohssie_
- Github: https://github.com/ohssie
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.