Create an Incoming Webhook to Forward SMS to Slack with Laravel
Time to read: 4 minutes
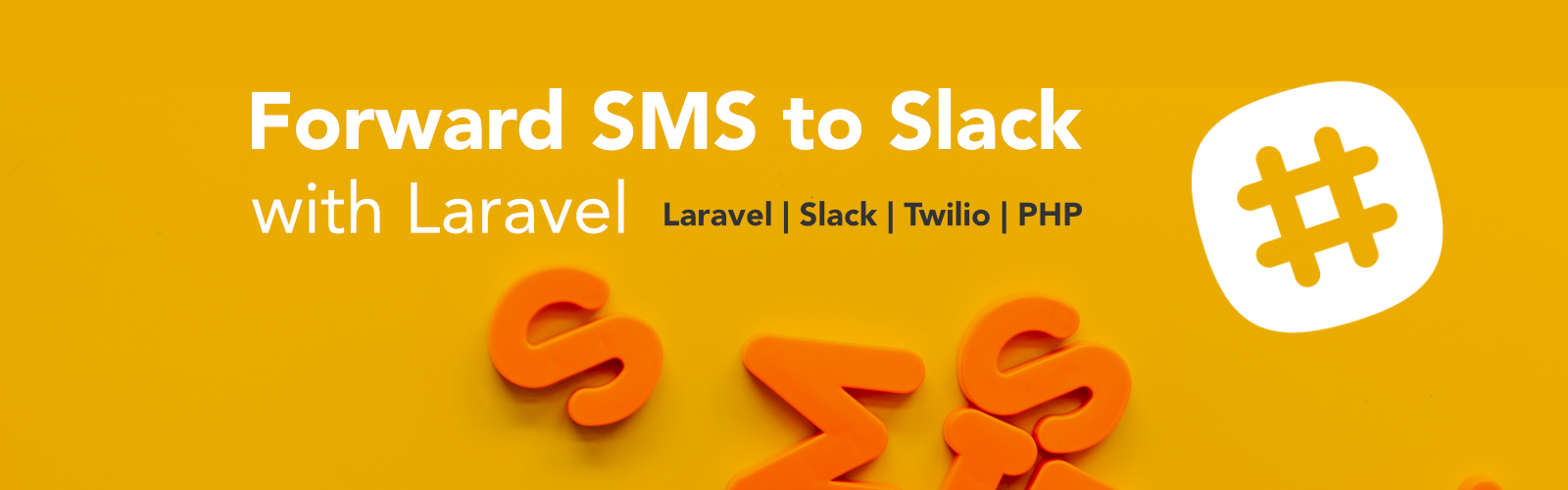
Introduction
As a business entity, you will always get SMS from customers seeking support, giving you feedback, filing complaints or even sending compliments. Because notifications can become overwhelming, it helps to consolidate all the SMS in one central place for easier reference.
In this tutorial, we will automatically forward SMS sent to a Twilio number to a slack channel using a webhook implemented in Laravel.
Requirements
- PHP environment
- Twilio account
- Slack account
- Ngrok
- Composer globally installed
Set Up a New Laravel Project
If you don't have one already set up, we’ll need to install a fresh Laravel application. A guide on how to set up Laravel can be found in the official Laravel documentation.
We also need to install the Twilio SDK for PHP in our project. In your terminal, navigate to the project directory and run the following command:
Getting started with Slack Incoming Webhooks
Incoming webhooks enable you to share information from external sources with your slack workspace.
Create a Slack App
Log in to your Slack account and navigate to the create Slack app page. Enter the name of choice, choose a workspace and click Create App.
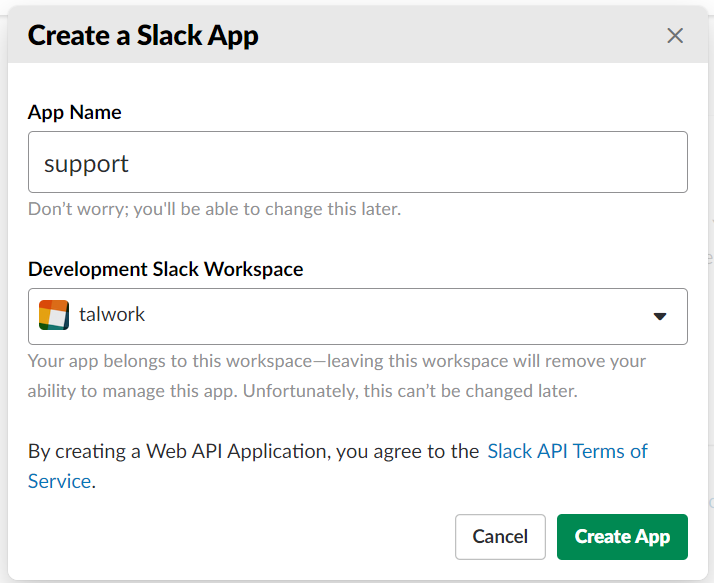
Enable the Slack Incoming Webhook
After creating the Slack app, you will be redirected to the settings page for the new app. On the page, select “Incoming Webhooks” and click “Activate Incoming Webhooks” to toggle and turn on the feature.
Create an Incoming Slack Webhook
After you enable the incoming webhook, the page should refresh and extra options will appear. Click “Add New Webhook to Workspace” button. Select a channel that the app will post to, and then click Authorize to authorize your app.
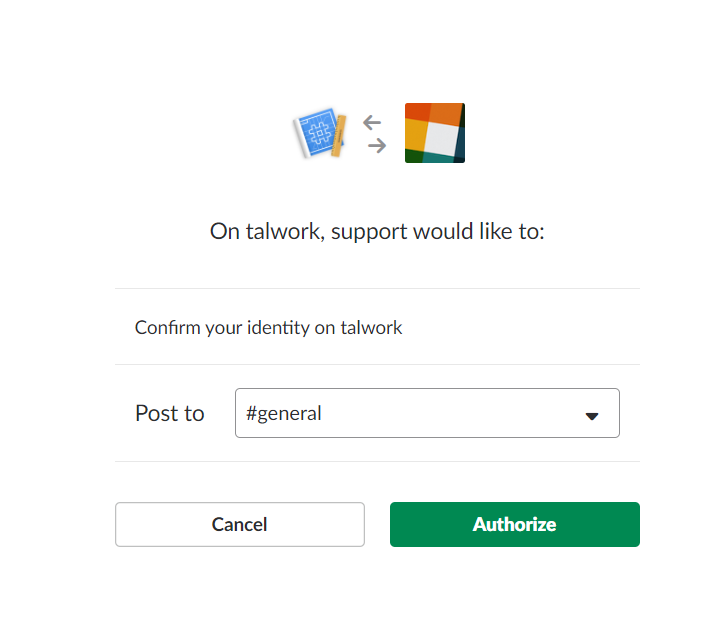
You’ll be redirected back to the app settings page which should now have a new entry under the “Webhook URLs for Your Workspace” with a webhook URL. The webhook URL should look something similar to this:
You now have a new incoming webhook. Let's see how we can use it to post a message to our channel using the Laravel application we set up earlier.
Use Your Incoming Webhook to Post a Message
Require Guzzle dependency
We will use Guzzle to post the message from the application to the webhook. To install Guzzle, navigate to the project directory and run the following command:
Update Environment File with Webhook
Open the .env file and add the following code:
The URL used here should be the one we generated earlier in the app settings page.
The Webhook Model
The next step is to create a model we’ll use to prepare the message that will be sent to our slack webhook. By default, models are found in the app folder. Run the following command to create Webhook model:
Open the file app/Webhook.php and add the following code:
The Webhook Controller
To handle the user inputs and update the model accordingly, we need a controller. By default, controllers are found in app/Http/Controllers
. Run the following command in the project directory to create WebhookController:
Open the file app/Http/Controllers/WebhookController.php and add the following code:
Create The Endpoint
The final step at this stage is to create a route; an endpoint we’ll hit to send the message to slack. Inside routes/web.php, add this line of code:
This is a get method and we can access it through the URL http://localhost:8000/webhook
You should be able to see this message in your Slack channel when you hit the endpoint.

We have established that our Slack incoming webhook is working. The next step is to integrate the Twilio SMS webhook.
Create the Incoming Twilio Message Webhook
We need an endpoint that will handle the SMS forwarded from the Twilio phone number and send the SMS to the incoming webhook we have created in Slack.
Create Temporary Public URL
To test locally, we need DNS software like ngrok that assigns a local port a publicly accessible URL over the Internet. Once ngrok is set up, run the command below to expose our local web server running on port 8000 to the Internet.
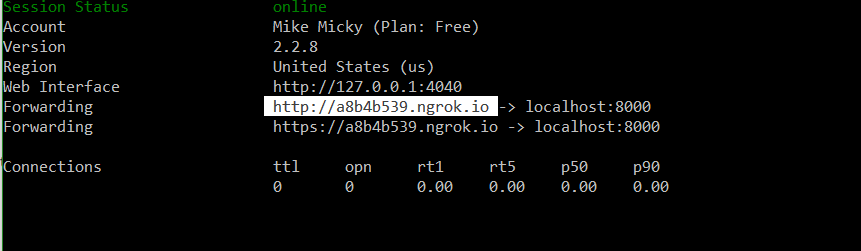
Add Twilio Webhook Code
In the Controller, app/Http/Controllers/WebhookController.php add the following code:
Add The Twilio Endpoint
Update the routes file routes/web.php and add this line of code:
Disable CSRF verification
To be able to receive posted data from external source, we need to exclude our webhook from CSRFverification. Inside app/Http/Middleware/VerifyCsrfToken.php, add the following code:
Configure a Phone Number to Reply to Incoming Messages with a webhook
- Login into your Twilio account and go to the console numbers page.
- Click the plus button to purchase a phone number if you don't already have one.
- Click the phone you want to use. You will be redirected to a new page.
- Inside of the message section “A MESSAGE COMES IN”, select option, choose “WEBHOOK” and paste the URL to the webhook we created earlier.
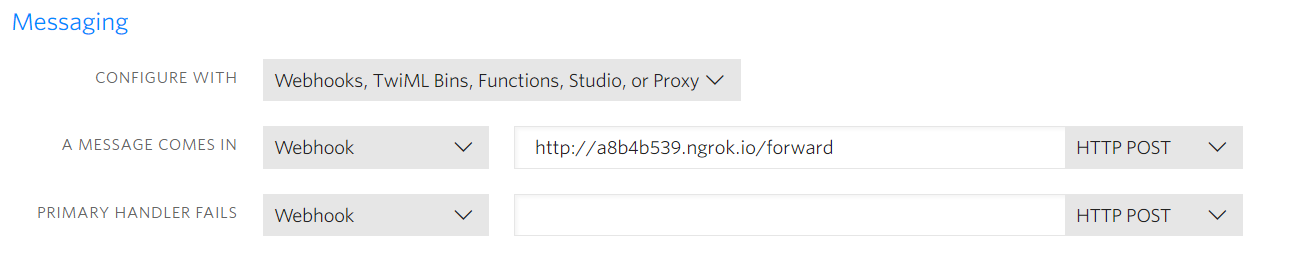
Testing a webhook
Finally, Let’s test the completed code. Send an SMS to the Twilio phone number we have just configured above.
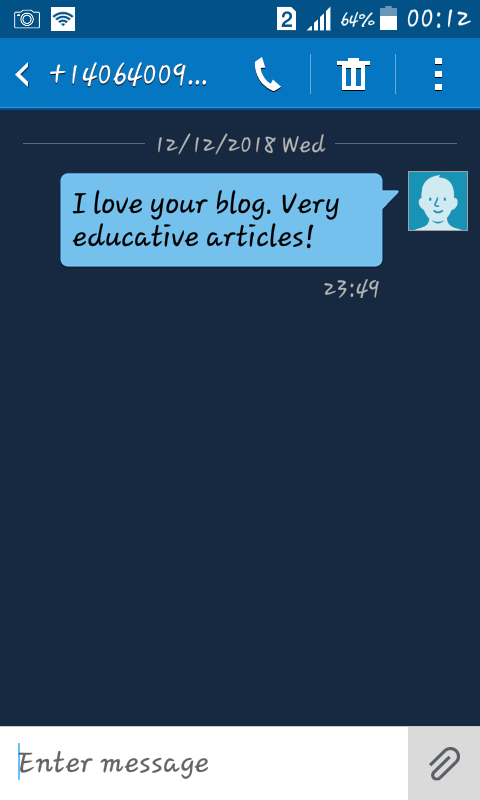
You will immediately see the message pop up in the Slack channel as shown below.

Conclusion
You can now make awesome apps to take advantage of the awesome features in both Slack and Twilio. If you can picture it, then you can implement it.
The complete code for the tutorial can be found on Github.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.