How to Create Text Message Alerts for PayPal Payments using Twilio, PHP, and YII 2
Time to read: 5 minutes

I was recently completing a payments integration for a recruiting firm that wanted to quickly deploy text message alerts for when buyers complete a PayPal payment flow. The business wanted the ability to instantly text payment info to the payee’s cell number. This tutorial is an introduction to integrating Twilio SDK into a Yii2 web application.
Preview of Text Alert
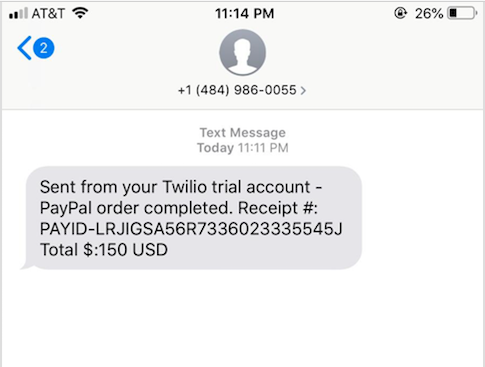
Technical Requirements
For this tutorial we’ll assume the following:
- PHP 5.4.0 or higher is required
- Twilio Account
- Paypal Account/Developer with restful application key and secret
- Composer installed
- Git installed and have a basic understanding
- Basic understanding of Yii2 PHP web framework
YII2 framework is defined as a high performance, component-based PHP framework for rapidly developing modern web applications. Personally, I love that it allows me to just focus on code instead of design patterns. I can scaffold views, data models and other trivial tasks that would normally take a great deal of time.
Cloning the sample project
The first thing we will need is a working installation of git. Open your favorite console/terminal and navigate to your local web server instance. My version is XAMPP so my server is located at ~/xamp/htdocs
. Run the following commands below.
Installing dependencies
Navigate to the folder twilio_yii2_tutorial
and open a new terminal window if you haven’t closed the previous one used for cloning the repository. We will need Composer installed at this point. If you haven't, you can follow this documentation.
Grab a cup of coffee if your Internet is slow and let it install.
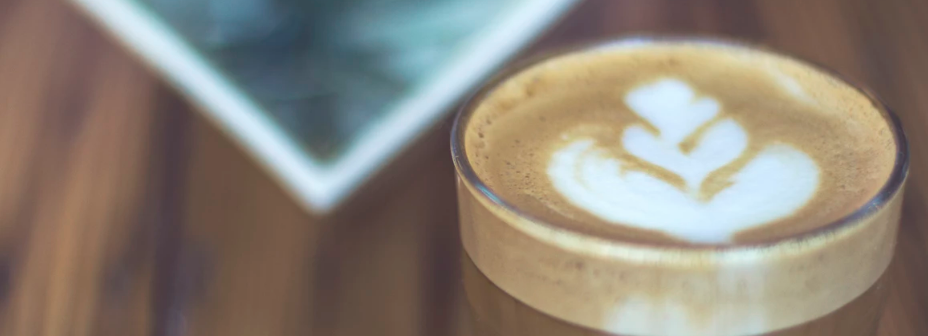
At last! The screenshot of the successful installation of project dependencies.
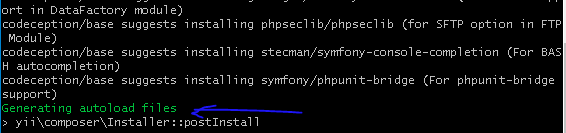
Assuming that you, cloned the project in your local PHP web server, navigate to the URL below http://localhost/twilio_yii2_tutorial/web/
The index page should load a demo product page that shows a single product and price, along with a submit button. See screenshot below.
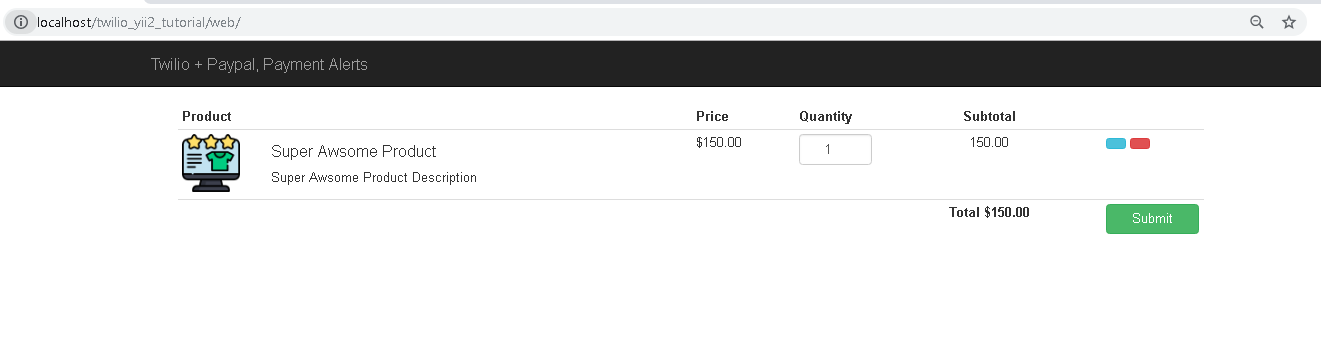
At this point you have a working YII2 web application with the Twilio and PayPal SDK installed.
Wrapping Twilio and PayPal SDKs for usage in application
Open your favorite note editor and navigate to the common folder of the application hierarchy. See screenshot below. I am using Sublime editor.
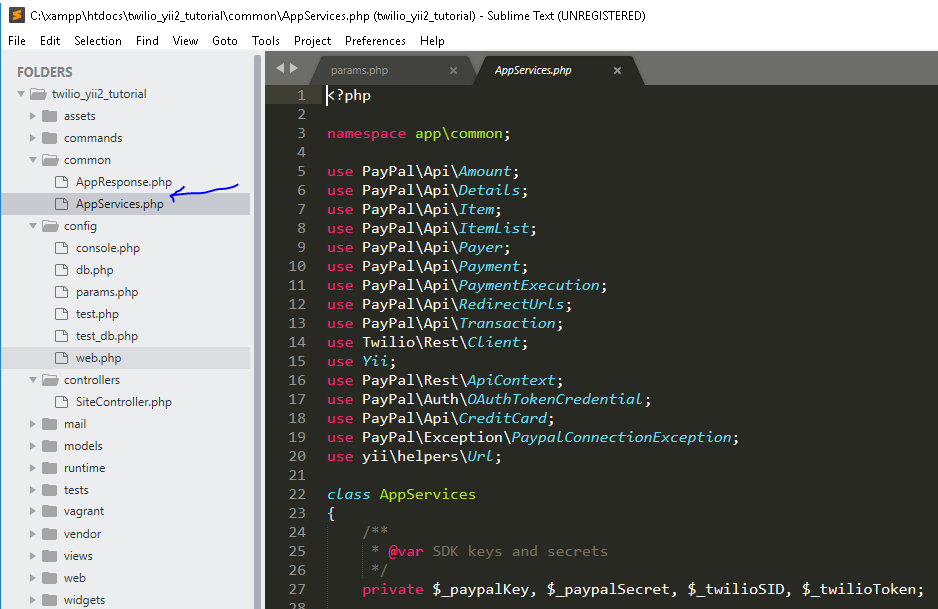
The following PHP files AppResponse.php
and AppServices.php
are classes used to conduct payments and SMS disbursement.
AppResponse.php
is a response class that holds$success
,$payload
and$message
properties for when we get a response from PayPal and Twilio servers.AppServices.php
contains the methods that actually conduct the payment and sending of text messages. Such methods include :- function
generatePaymentUrl(params, callbackUrl)
, accepts a callback URLan array of product info, ex. price, description and other relevant product information. It also returns anAppResponse
object with payload property being a redirect url to the PayPal payment gateway for the user to login and complete purchase. After payment is complete, a callback is made to a designated URL and then the methodmakePayment($payload)
is executed. - function
makePayment($payload)
accepts the redirect query string data and conducts the final step in the payment chain. Returns payment payload from PayPal SDK. - function
sendAlert(from, to, message)
accepts a Twilio verified phone number for the$from
parameter, destination number and$message
body. Returns a true or false for message sending procedure.
- function
Retrieving PayPal Client ID and Secret
A requirement of this tutorial is a PayPal restful application on the developer portal. Please follow this documentation if you haven’t done so already. Store this value in a text file for now until we ready for it.
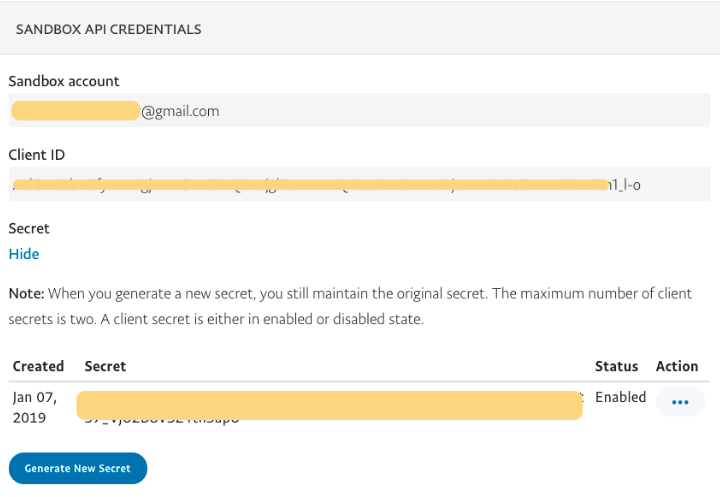
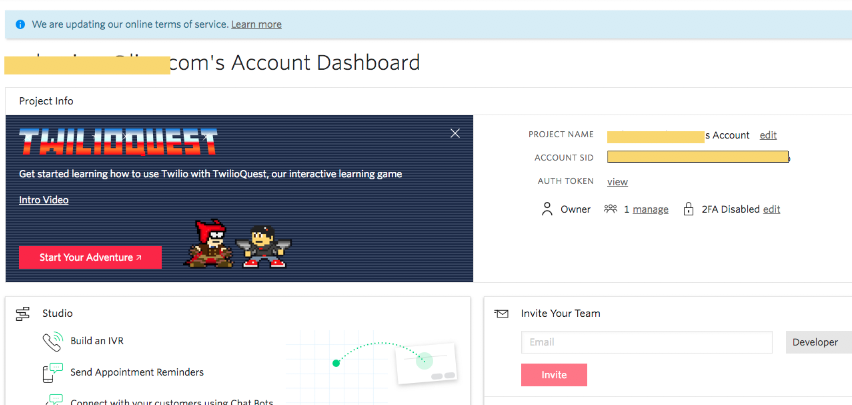
After creating a Twilio account you are given a SID and Token for your account on the dashboard page. Store this value in a text file for now until we ready for it.
Configuring Twilio managed and verified numbers
Purchase a Twilio managed phone number with SMS capabilities. If you haven’t done so, please see this complete tutorial on how to purchase one.
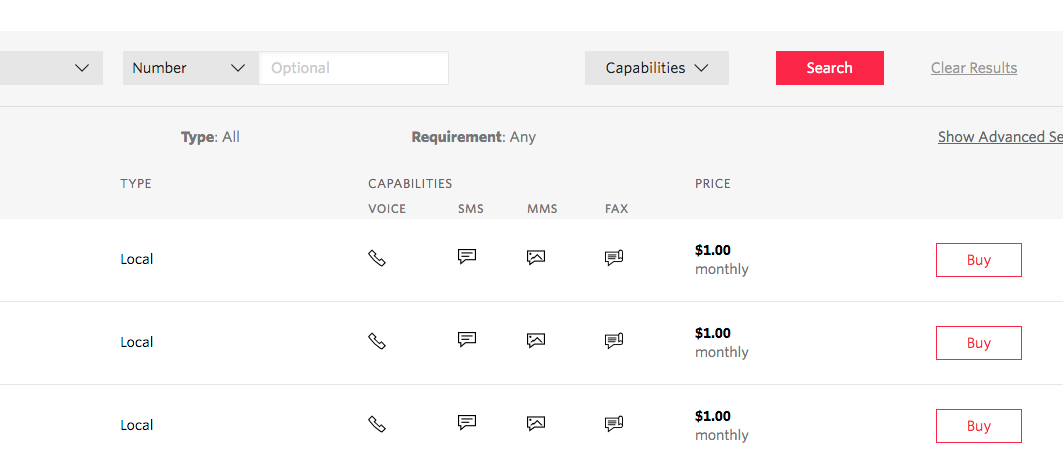
Navigate here https://www.twilio.com/console/phone-numbers/verified and enter a phone number that you want to test as a recipient.
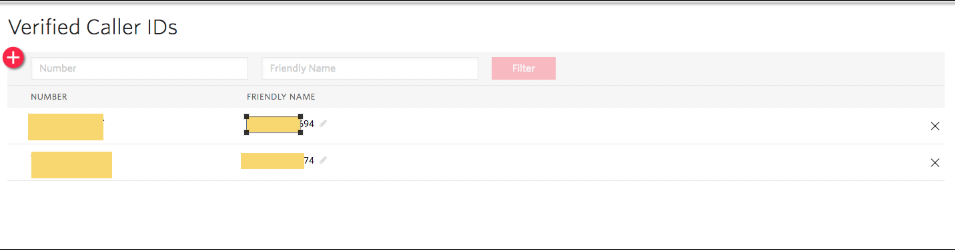
Updating parameters file in application route
Open the params.php
file in the config
folder of the tutorial
folder currently running on localhost. Open your text editor/IDE and enter the Twilio and PayPal credentials previously retrieved. See code snippet below.
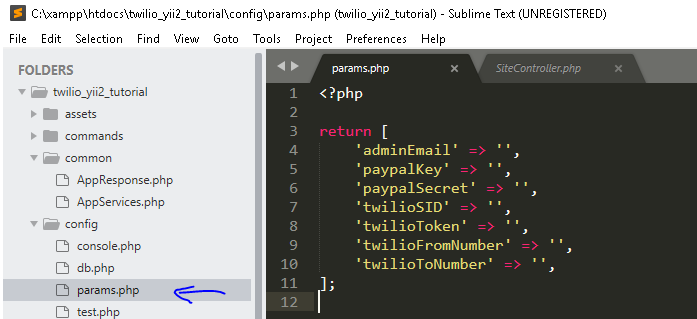
The preceding array will be used to store Twilio and PayPal credentials used by the application. See key value explanation below :
- adminEmail : Enter your email or a test email it doesn't matter
- paypalKey : Enter the PayPal client id
- paypalSecret : Enter the PayPal secret
- twilioSID : Enter the Twilio SID
- twilioToken : Enter the Twilio Token
- twilioFromNumber : Enter the verified number bought from Twilio
- twilioToNumber : Enter the verified caller id you want to send test SMS.
Dissecting method actionIndex() of SiteController class
If you are knowledgeable of MVC, the controller accepts input and converts it to commands for the model or view, thus this where we handle the actual payment after you press the submit button. The indexAction()
method holds all the code we will need to conduct payment and SMS disbursement. So let’s dissect this method.
actionIndex() HTTP Request Methods [GET,POST]
[GET] /index
- loads the demo screen showing product.
[POST] /index
- This essentially generates the redirect URL to allow for PayPal user authentication and allow the user to select their preferred method of payment.
actionPayment() HTTP Request Methods [GET,POST]
[GET] /payment
- Without params loads the index page
[GET] /payment
- This is the callback URL specified in the post request code, PayPal passes query string params :
- Success - bool
- PaymentId - unique payment id from PayPal/receipt id
- Token - id passed from PayPal to invoke the makePayment method found in the AppServices class
- PayerID - payer id defined in dashboard
On payment processed, an SMS is sent to the test destination number specified in config/params.php
file.
Conducting a payment Payment
Pressing the submit button triggers the payment flow. This action loads the PayPal hosted gateway that allows users to authenticate and pay for the item/items.
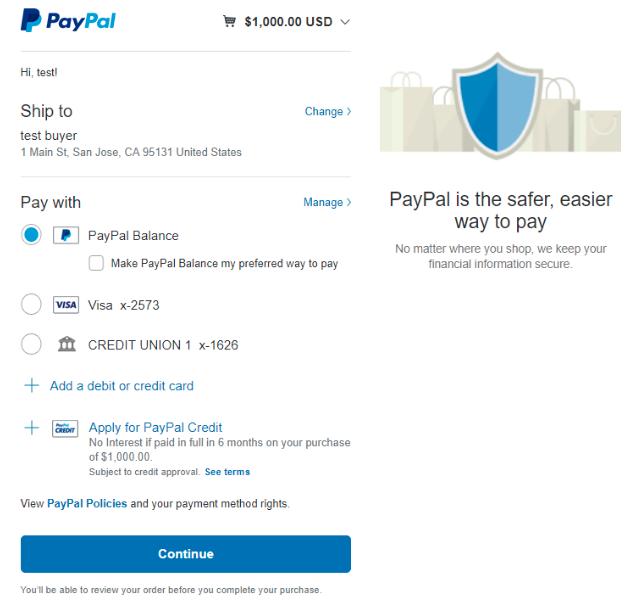
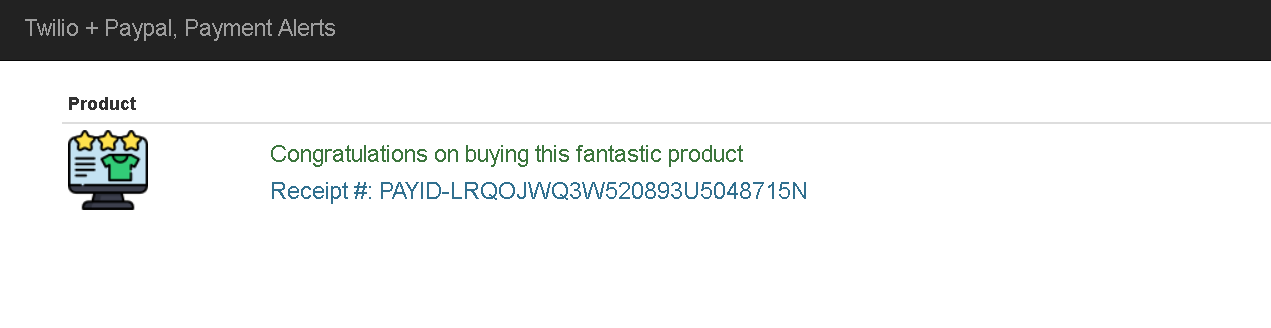
The test recipient number that was specified in the params.php
file should get a message about the payment being made. See screenshot below.
Displaying of a successful payment alert on the recipient cell phone
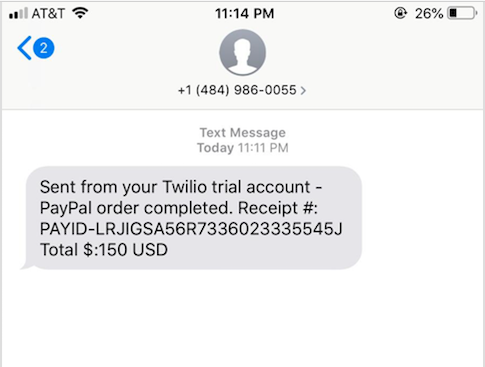
Conclusion
The example we have implemented above is just us getting our feet wet. There is a lot we can implement using Twilio SMS services. For example, we can implement a cancel payment via SMS where the account holder sends a cancel message using receipt id. Ex. cancel PAY-LRJIGSA56R7336023335545J and we kick off a cancel flow in the backend and execute PayPal restful endpoints to issue a refund.
Written By:
Name: Andre Piper
Twitter: twitter.com/andrepiper89
Email: andrepiper@gmail.com
Github: github.com/andrepiper
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.