Creating a Daily Reminder WhatsApp App for Your Subscribers
Time to read:
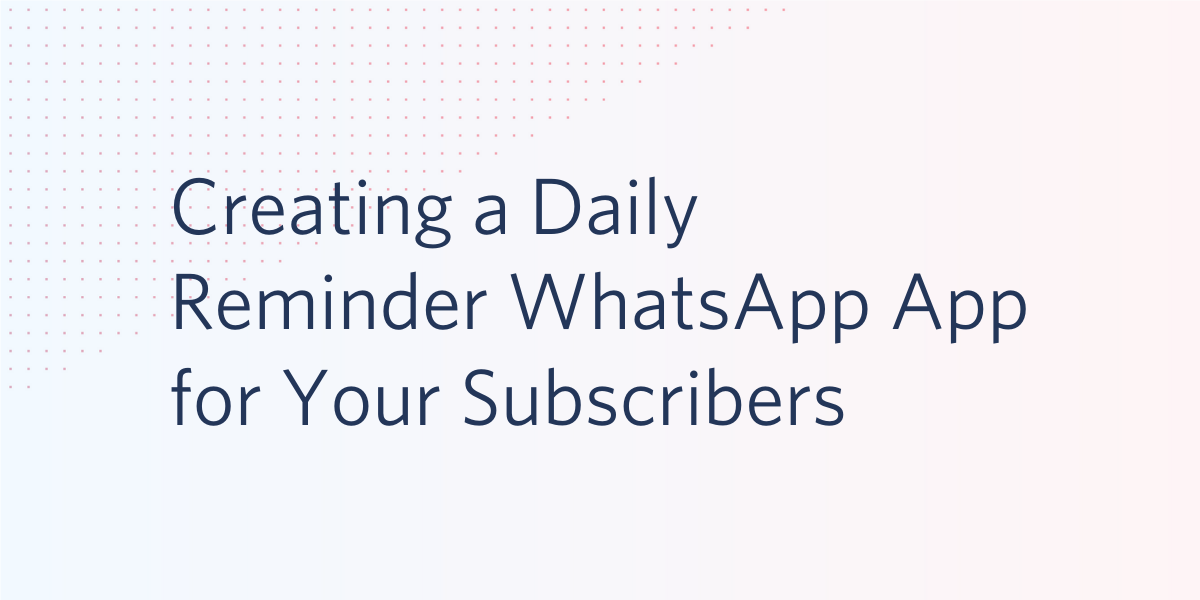
For the longest time, the ideal way to keep track of subscribers was by creating an email list of all subscribers. However, due to the tremendous increase in the number of people using smartphones, and the introduction of Whatsapp and Whatsapp business, the tides have turned.
Whatsapp, with over 1.5 billion active users in over 180 countries has revolutionized the way people communicate and consume information. Nowadays, users prefer real-time communications that are easy to consume which WhatsApp has achieved spectacularly. Most businesses have seen the potential and are now asking their users for phone numbers instead of email addresses. According to an article from Techcrunch, by mid-2018, 3 million companies were already using the WhatsApp Business app.
In this tutorial, we are going to learn how to send a daily reminder to Whatsapp subscribers. This will be achieved by creating a Reminder app using Laravel, creating a REST API that sends reminders to Whatsapp users via the Twilio’s WhatsApp API, and a sandbox phone number.
What you’ll need
To complete this tutorial, you will need the following dependencies:
- Twilio Account
- Laravel, the PHP Framework for Web Artisans
- Composer globally installed
- Guzzle, a PHP HTTP client that makes it easy to send HTTP requests
Create A New Laravel App
We will first build our reminder app and a REST API using the Laravel framework.
For more information on how to install and use Laravel, check out the official laravel documentation.
To create a new Laravel project, run the following command in your terminal:
Set Up the Development Environment
First, install the Twilio PHP SDK in your project using Composer. This will allow the application to easily connect to the Twilio API and programmatically send Whatsapp messages to our subscribers.
In your terminal, run the command below:
Set Environment Variables
Retrieve your Account SID, Auth Token, and Whatsapp sandbox number from your console dashboard and update the the .env
file as shown below:
Creating the Reminder Application
In this tutorial, we will send a scripture of the day as our reminder to subscribers. I will be using the Uncovered Treasure API from Rapid API to retrieve the Bible scriptures used.
We will create a model and extend it with custom methods to generate the scripture of the day, send WhatsApp messages with a link, and then create a controller to organize the route level logic, and finally call the logic from the model.
Create a Reminder Model
Run the command below to create the Reminder
model:
Add Reminder App Logic
Open the file app/Reminder.php
and add the code below:
The above code has two functions; the todaysScripture
and sendReminder
functions.
The todaysScripture
generates the scripture of the day while the sendReminder
functions send the scripture reminder message (with a link to a detailed breakdown of the scripture).
When using the Twilio Sandbox, you have to use one of the three pre-provisioned WhatsApp templates for one way notifications like reminders. I have opted to use the “ Your {{1}} code is {{2}}“ template.
You can get more information on how to use pre-provisioned templates from the Twilio Whatsapp docs.
Create a Reminder Controller
Run the command below to create the ReminderController
controller:
Next, create a function to handle the logic of calling the scripture of the day logic from the model and routing it to the API endpoint we will create next.
Open the file and the logic below:
Create API route/endpoint
Now that our reminder app is complete, we need an endpoint the subscribers will click on in the reminder message.
By default, all API routes are defined in routes/api.php
.
Add the code below to routes/api.php
to connect the function we created in ‘ReminderController’ to the API route.
The Reminder Artisan Command
We need a way to automate the process of sending daily reminders to the subscribers. This is where Artisan commands come in handy.
Run the artisan command below to generate the artisan command:
Open the file app/Console/Commands/sendReminder.php
and update the handle
method and the signature
variable as shown below:
Register the command in the Laravel Scheduler.
When using the scheduler, only a single Cron entry is needed on your server.
By default, the task schedule is defined in the app/Console/Kernel.php
file's schedule
method.
Update the schedule
method with this single line of code.
The command will be executed every day at 6:00 AM.
If you need help, you can use http://corntab.com/ to create cron rules.
Finally, add the following Cron entry to your server.
This Cron will call the Laravel command scheduler every minute. When the schedule:run
command is executed, Laravel will evaluate the scheduled tasks and run the tasks that are due.
Testing our App
To test the app, navigate to your project directory and run the artisan command below:
You should get a WhatsApp message similar to the one shown below:
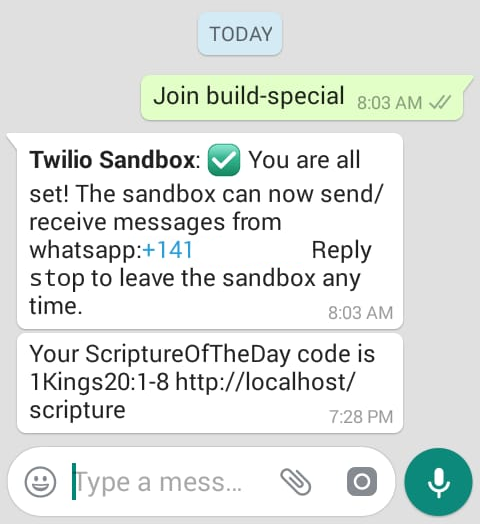
Conclusion
To improve on this reminder app, you can create a custom WhatsApp template and submit it for approval by following this guide.
The complete code used in this tutorial is available on Github.
I would love to see what you can build. Feel free to reach out to me:
- Twitter: @mikelidbary
- GitHub: mikelidbary
- Web: www.talwork.net
Additional Reading
Here are some great resources to continue building with WhatsApp:
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.