How to Queue Emails in Laravel PHP with Twilio SendGrid
Time to read:
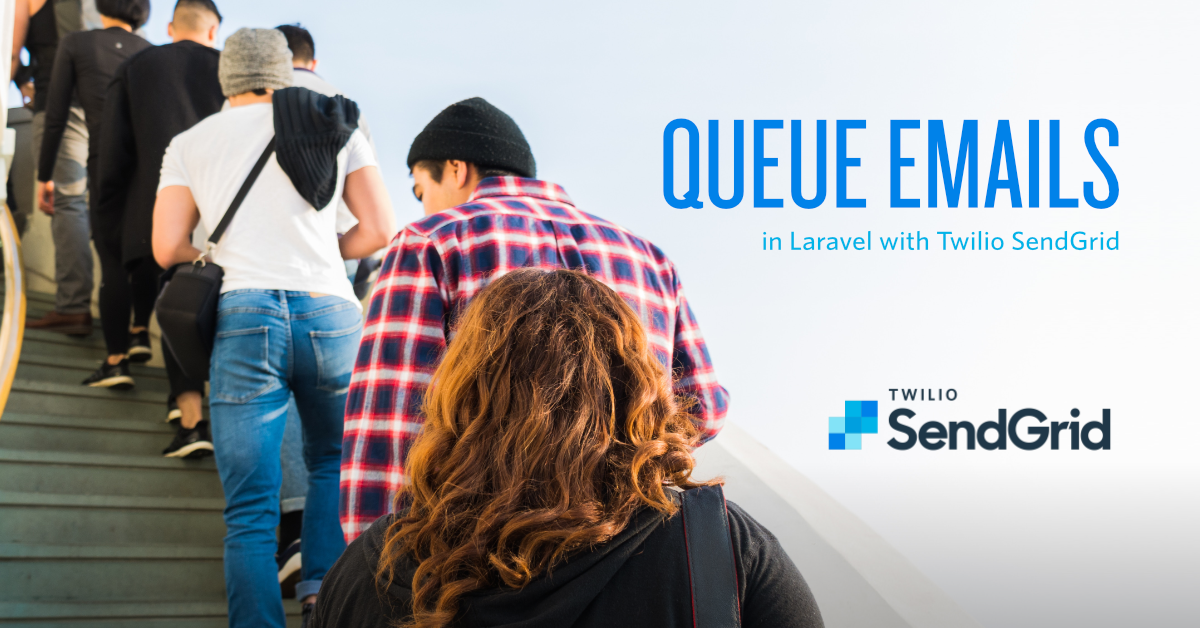
Introduction
Laravel is one of the most famous PHP MVC frameworks with a great community. It provides all the features that are required to create your project, whether it is for personal or enterprise level usage. One feature that sets Laravel apart from other frameworks is “Laravel Queue”. For any project that needs to defer time consuming tasks, Laravel Queue offers this support out of the box.
This tutorial will help you to implement the Laravel Queue to send emails. After we’re finished, you will have a running Laravel application that allows you to send emails to registered users using Laravel queue to prevent your task from timing out and increase deliverability of your emails. We will use Sendgrid for sending emails because their service will allow us to check out whether your email is read or opened by the user.
Requirements
Set Up a New Laravel Project
If you don’t have Laravel installed in your system, install it first from the Laravel Official Documentation. A guide to getting started from Laravel can be found in the Laravel docs. Let’s start with setting up a new Laravel Project named “laravel-queue”. Remember, you can give your project any name you want.
This will install Laravel v5.8 along with all the dependencies required by it. Composer post-create
scripts automatically create the .env
file for us and set the APP_KEY
environment variable. Add the APP_KEY
in the .env
file by running this command if it is not configured automatically.
Next we need to add the database connection details to our .env
file. We will use MySQL
database for this project. After you have created your local database, add the DB_USERNAME, DB_PASSWORD
and DB_DATABASE
values in the .env
file.
Create a SendGrid Account
Before going further, let’s set up a Twilio SendGrid account for emailing. Yes, SendGrid is now part of Twilio, powering the future of customer engagement on one platform.
Once we are done with account setup, let’s create a Mail driver for our Laravel application. Here’s a quick setup guide for Using SendGrid as Your SMTP Relay in Laravel PHP
Create Auth Scaffolding for Login/Registration
Laravel makes implementing authentication very simple. In fact, almost everything is configured for you out of the box. If you’re unfamiliar, you can read more about Laravel Authentication from the documentation.
Run this command to add the Auth scaffolding to our Laravel project.
This will generate the Auth scaffolding for the project. Laravel 5.7+ comes with the email verification feature by default, so we will use the default functionality first. Later on we will switch to Laravel queues so we can have an understanding of both implementations.
Use the Email Verification Feature
To use the Email verification feature we need to make sure that our App\User
model implements the Illuminate\Contracts\Auth\MustVerifyEmail
contract. Open App\User
model file and make the following changes:
Our user
migration table is already configured with the email_verified_at
column and is set to nullable by default. It stores the date and time when the email address is verified. Run our database migration by running this command.
Email verification is an optional feature that comes with Laravel. We just need to enable routes to send verification links and verify emails. This can be done simply by passing the verify
option to the Auth:routes()
method. Open the web.php
file under the routes
folder to enable this option.
Laravel comes with a verified
route middleware to allow access only to the verified users. So let's add this route to the home
route like this:
Now that we have enabled the basic email verification feature, let’s implement Laravel Queues.
Implement Laravel Queues
We have completed basic setup for email verification from Laravel. We will now implement Laravel Queue to add a layer of efficiency. Laravel Queue is used widely because it uses a queue to send emails one by one without getting them lost in delivery. We can also prioritize these emails depending on our need.
Before implementing the Laravel Queue, let’s understand how Laravel implements the email verification so that we can successfully include our queue procedure within their flow.
Laravel raises events during the registration process. Whenever a new user is registered, an event is fired which is listened to by the class SendEmailVerificationNotification
. We can find this event in the EventServiceProvider.php
file under the app/Providers
directory. This concludes what we need in order to create a listener that listens on the registration event. From there we can make our own flow of sending emails without changing the default configuration.
Creating Listener Class
In Laravel, Event listeners receive the event instance in their handle()
method. Within the handle()
method, we may perform any actions necessary to respond to the event. Let’s create a listener class using the Artisan CLI:
If it doesn’t exist, a new directory app/Listeners
is created with the EmailVerificationListener.php
file within it. We will handle the event within the handle()
function. Since we don’t need a constructor method, we can remove it. From the handle()
method we need to dispatch our mailing job. Our file will look like this:
Now add the EmailVerificationListener
to listen for the registration event in the EventServiceProvider
file and remove the SendEmailVerificationNotification
listener.
Creating Jobs
By default, all of the queueable jobs for our application are stored in the app/Jobs
directory. If the app/Jobs
directory doesn't exist, it will be created when we run the make:job
Artisan command. We can generate the EmailVerification job using the following Artisan CLI:
Let’s create a verification link through the temporarySignedRoute()
method using the URL
facade, which is sent to the user’s email. After that, we will queue mail to the user’s email address using the Mail
facade which we have under the $event
variable. Within this job, we call the EmailVerification
mailable class to build our email template and pass the verification link to it. Our EmailVerification
job will look like this:
Creating Mailable Class
In Laravel, each type of email sent by your application is represented as a “mailable” class. These classes are stored in the app/Mail
directory. Let’s create one for our email verification using the Artisan CLI:
As soon as this command is completed, a new directory app/Mail
, is generated with the EmailVerification.php
file within it.
Mailable Classes in Laravel have a build()
method along with a constructor()
in which we build the template of the email that we are going to send. Within this template, we pass along any data that should be transferred with the email. In the EmailVerification
class, we pass the token in every email, ensuring it will be passed down into an email template.
Now let’s create a template for our email. We will store the template in the email
directory under the resources/views
folder. If it doesn’t exist, create the email
folder.
Next in the resources/views/email
folder create a new file emailVerificationMail.blade.php
. Copy the code below into your emailVerificationMail.blade.php
file.
We’ll now include the view in the EmailVerification
mailable class to build out the template of the email.
We are almost done with our Listeners, Jobs, and Mailable classes. Now, we need to setup Queue Drivers so that our mailable class is recognizable by Laravel.
Setup Queue Driver
Now we will set our queue driver to “database”. Add the following settings in the .env
file and make sure to change the APP_URL
fromhttp://localhost
tohttp://localhost:8000
else the queue workers will send the incorrect verification link which results in the Invalid Signature.
After that we need to generate migrations and create tables for the queue. Let’s run the command below to generate the queue database tables:
Cool, let’s test our application and see whether it’s queueing our emails or not.
Testing
Open two terminals. One for running the Laravel development server and one for running the queue worker. Laravel includes a queue worker that will process new jobs as they are pushed into the queue. Run these commands in each terminal respectively.
Laravel Development server will run at http://localhost:8000
so let’s open the browser and browse this URL. Go to the registration and register again.
Once we registered successfully, we can see the logs of the queue worker. It will show the processing/processed jobs and emails using the queue.
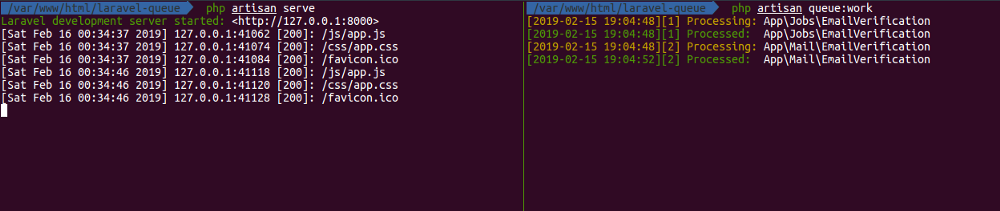
Let’s check our inbox for the email we have received through the Laravel queue.
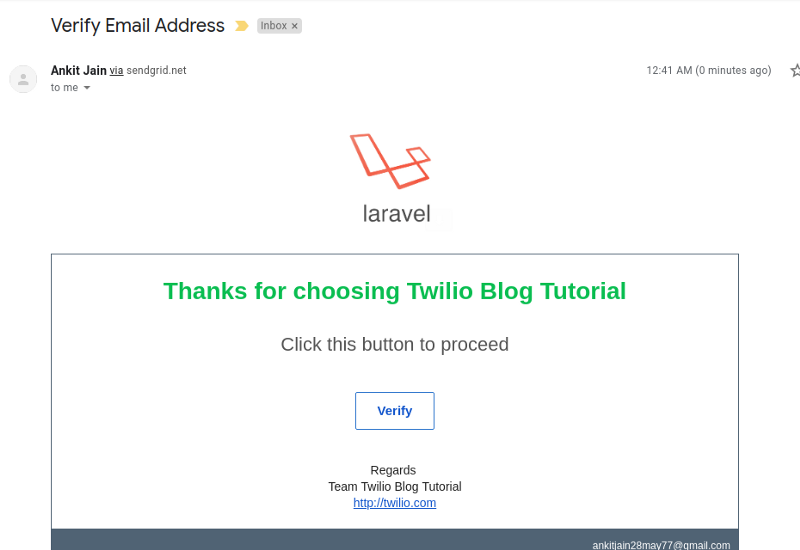
We will receive an email similar to the screenshot above which asks us to verify our email address. Once we click the Verify button, our email address will be verified and we will be redirected to the /home
page.
Let’s check the activity in our SendGrid Dashboard.
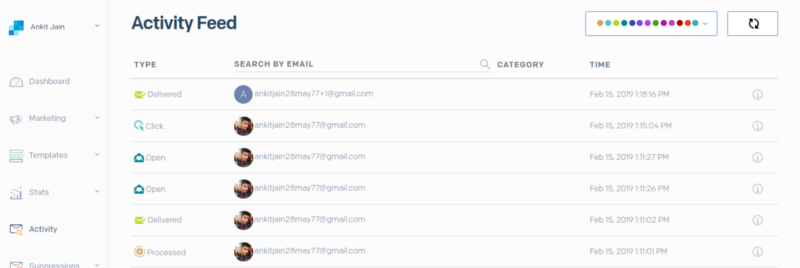
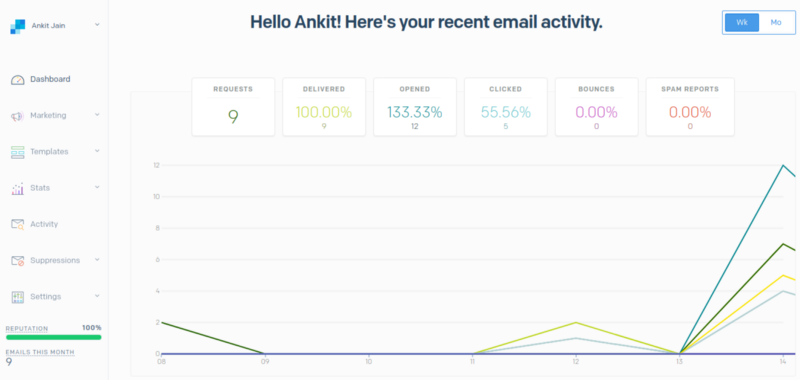
Conclusion
In this article, we implemented the Laravel Queue features and learned about listeners, jobs, mailable classes and understanding the default workflow of sending emails in Laravel. The complete code for the tutorial can be found on GitHub repo → laravel-queue-tutorial.
Wait we forget to fix the Request another email feature in Laravel as it is still sent through the default workflow that is without Laravel Queue. I left that part so you can implement that on your own and learn more about this Laravel Queues.
Tips: “Request another email” feature can be implemented through the VerificationController
under app/Http/Controllers/Auth
directory.
Laravel Queue has a lot of other good features that we should know how to make full use of like Queue Priorities, Specifying Max Job Attempts / Timeout Values, Job Chaining, Rate Limiting and many more. Read them in the official documentation of Laravel Queues.
Feel free to comment and ask me anything. You can reach me at ankitjain28may77@gmail.com and follow me on Twitter @ankitjain28may. You can also read more of my articles on Medium @ankitjain28may.
Thanks for reading!
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.