How to send recurring emails in C# .NET using SendGrid and Quartz.NET
Time to read: 6 minutes
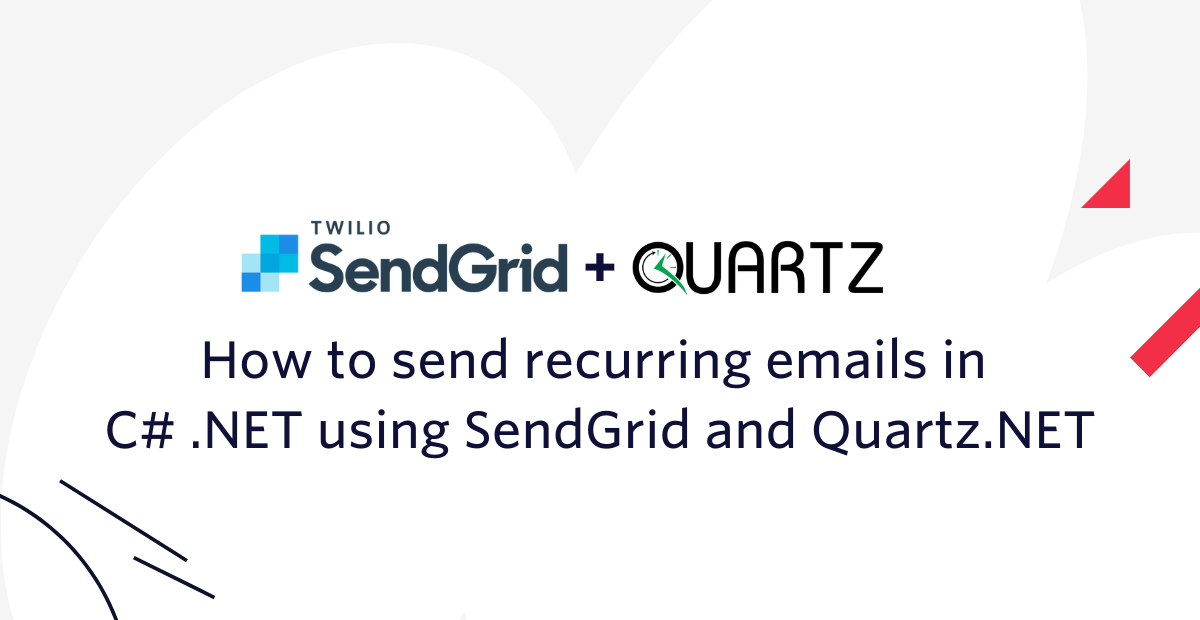
Many applications have the need to send emails on a periodic basis. A common example of this is a weekly digest, where the email recaps everything that happened that week. There are many ways you could send recurring emails using .NET. You could use the Task Scheduler on Windows, use crontab on Linux, or even develop your own job scheduling implementation. In this tutorial, you'll learn how to schedule recurring emails using Twilio SendGrid and Quartz.NET.
Prerequisites
You will need the following things to follow along:
- OS that supports .NET (Windows/Mac/Linux)
- .NET 6 SDK (download)
- A code editor or IDE (Recommended: VS Code with C# plugin, Visual Studio, JetBrains Rider)
- A Twilio SendGrid account (signup)
Everything you’ll need to do for this tutorial applies even if you’re using an older version of .NET (Core), however you’ll need to make minor adjustments.
Create your .NET application
You'll be using the .NET worker template because it is the best starting point for this type of application.
Create a new directory and change the current directory to it:
Use the .NET CLI to create a new project using the worker template:
Run the new .NET project using the .NET CLI:
Out of the box, the worker template will continuously run and print a new line to the console every second. The output of the command should look like this:
Integrate Quartz.NET into worker project
What is Quartz.NET
Quartz.NET is an open source job scheduler for .NET. You can use this library to schedule one-off jobs and jobs that should recur on a timed schedule. For this tutorial, you'll run a single instance of Quartz and store the jobs in memory, but you can also run multiple instances of Quartz and store the jobs in a database. Storing the jobs in a database and having multiple instances will help your application become more resilient and process more jobs at a time.
Add Quartz.NET to your project
Use the .NET CLI to add these two Quartz NuGet packages to your project:
The Quartz package contains the core capabilities to schedule jobs. The Quartz.Extensions.Hosting package contains extensions to integrate Quartz with .NET's generic host APIs, which the worker template is already using.
Open up the ScheduleMailUsingQuartz directory you created earlier in your preferred text editor and you will see a Program.cs file within it. Open up the file and replace the existing code with the following code:
With these changes, Quartz is now configured to run the SendMailJob
every 15 seconds.
The SendMailJob.Execute
method is where you want to add the logic of your job. For now, it logs "Greetings from SendMailJob".
Run the project again and observe the result:
The output will contain information about Quartz getting up and running. Once Quartz is running, you should see "Greetings from SendMailJob" logged every 15 seconds. The output looks like this:
Great job! You've added Quartz to your .NET application and have a job that recurs every 15 seconds.
It doesn't make sense to send an email every 15 seconds, but it makes testing a lot easier! You can use the simple schedule capabilities to run the job every X amount of seconds, minutes, or hours. You can also use CRON expressions to configure longer and more complicated schedules to run your job on.
Integrate SendGrid to send emails
Configuring your SendGrid account to send emails
There are two things you need to configure before you can send emails. First, you’ll need to set up Sender Authentication. This will verify that you own the email address or domain that you will send emails from. Second, you’ll need to create a SendGrid API Key with permission to send emails.
Sender Authentication
It is recommended to configure Domain Authentication which requires you to add a record to your DNS host. To keep things simple, you will use Single Sender Verification instead. This will verify that you own the single email address that you want to send emails from. Single Sender Verification is great for testing purposes, but it is not recommended for production.
Go to the SendGrid website and log in. Click on the Settings tab in the side menu. Once the settings tab opens up, click on Sender Authentication.
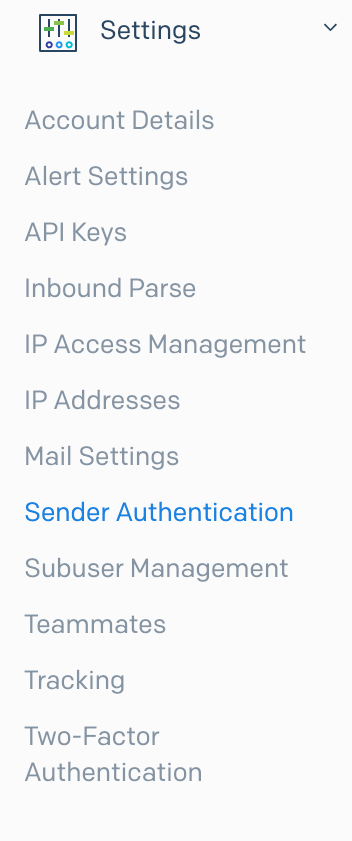
Click on Get Started under the Single Sender Verification section.
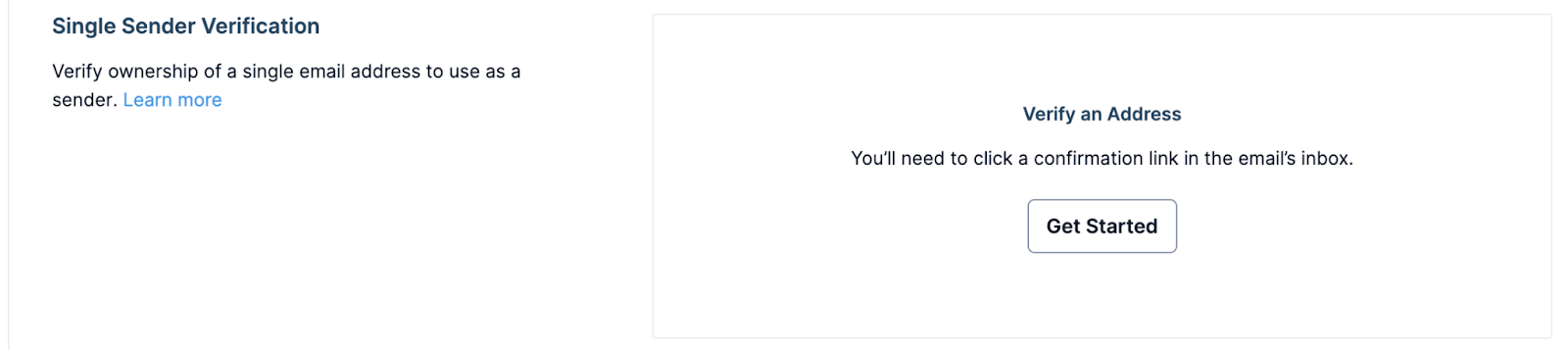
This will open a form on the right-side panel. Fill out the form with your information and email address.
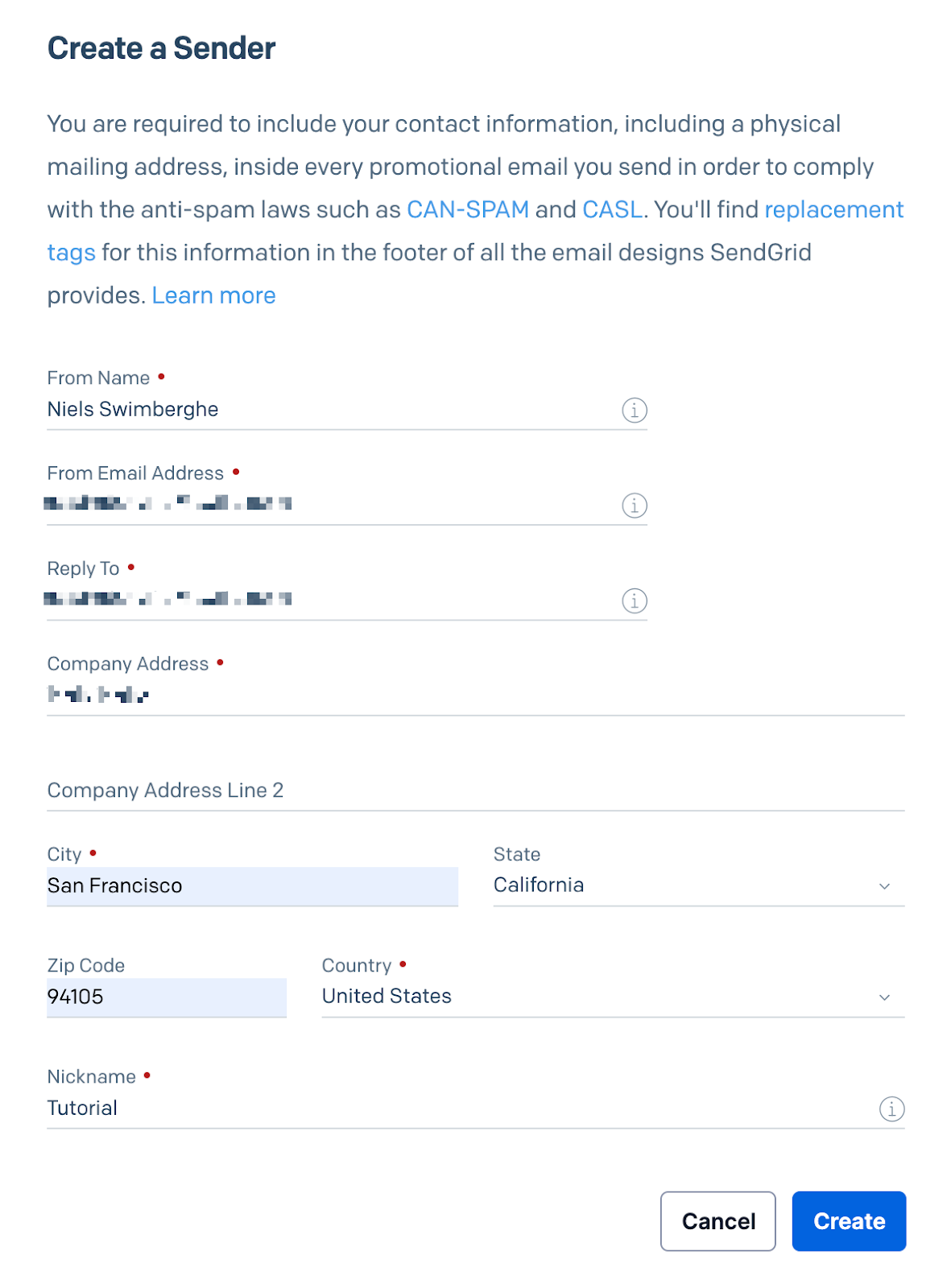
Click Create after filling out the form. Another panel will appear on the right, asking you to confirm your email address. An email has been sent to the email address you entered.
Go to your inbox, open the email from SendGrid, and click Verify Single Sender.
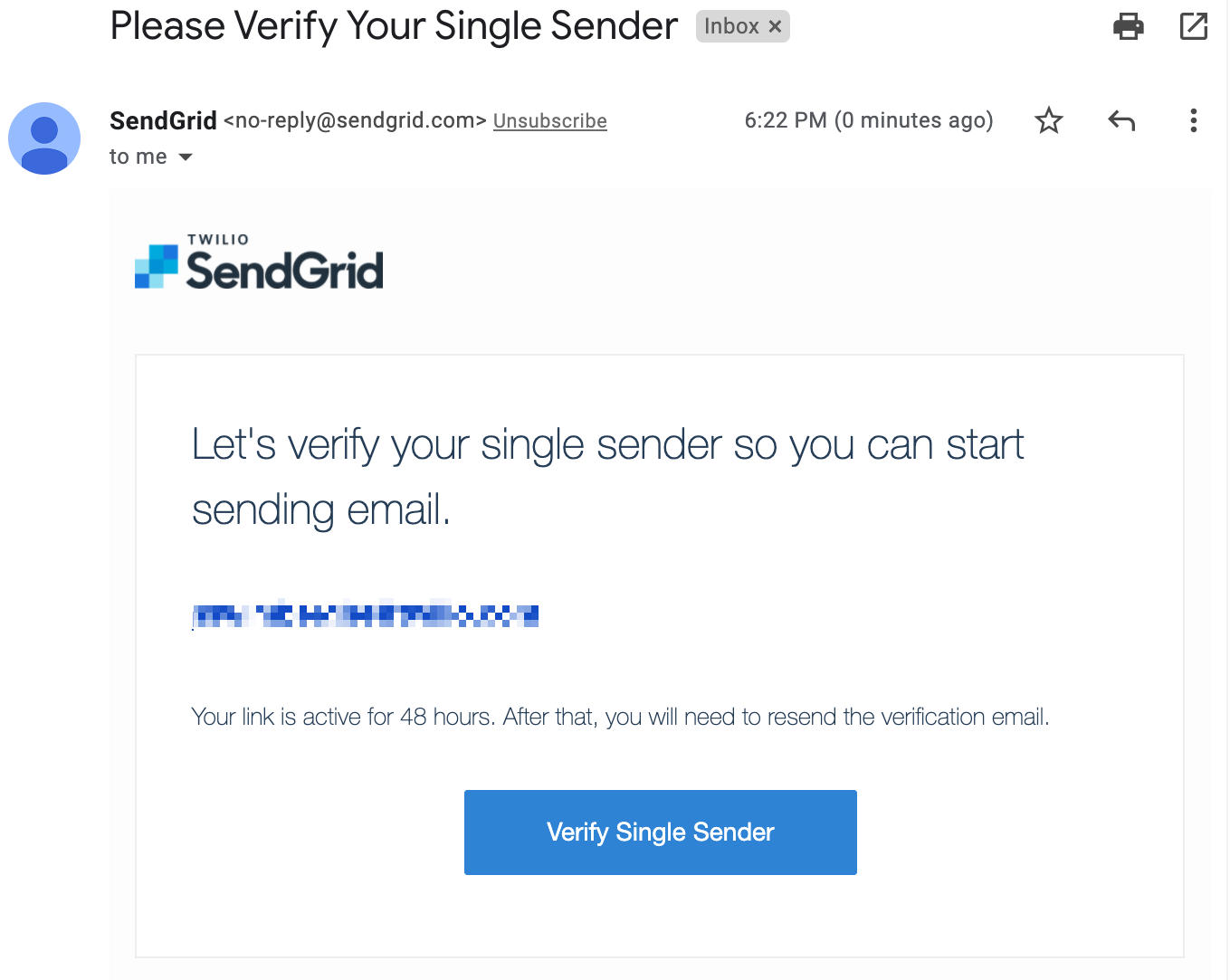
Your email address has been verified and you can now use it to send emails!
Create a SendGrid API key to send emails
Back on the SendGrid website, click on API Keys under the Settings tab. Click on Create API Key in the top right corner. This will open another form in the right-side panel. Give your API Key a useful name. You can assign different permissions to the API Key. For optimal security, you should only give the minimum amount of permissions that you need.
Click on Restricted Access.
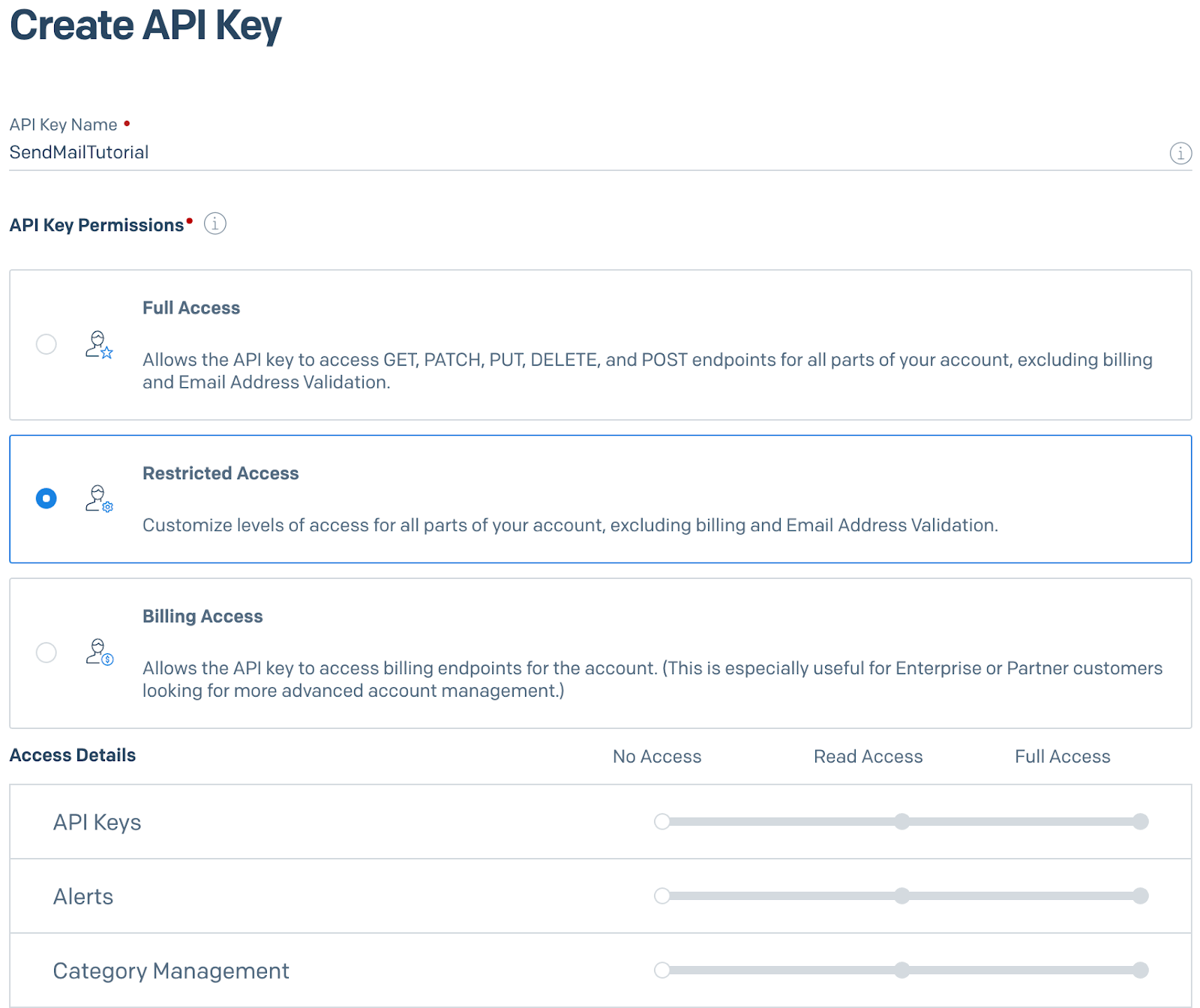
Scroll down to the Mail Send accordion item and click on it to reveal the permissions underneath. Drag the slider to the right for the Mail Send permission.
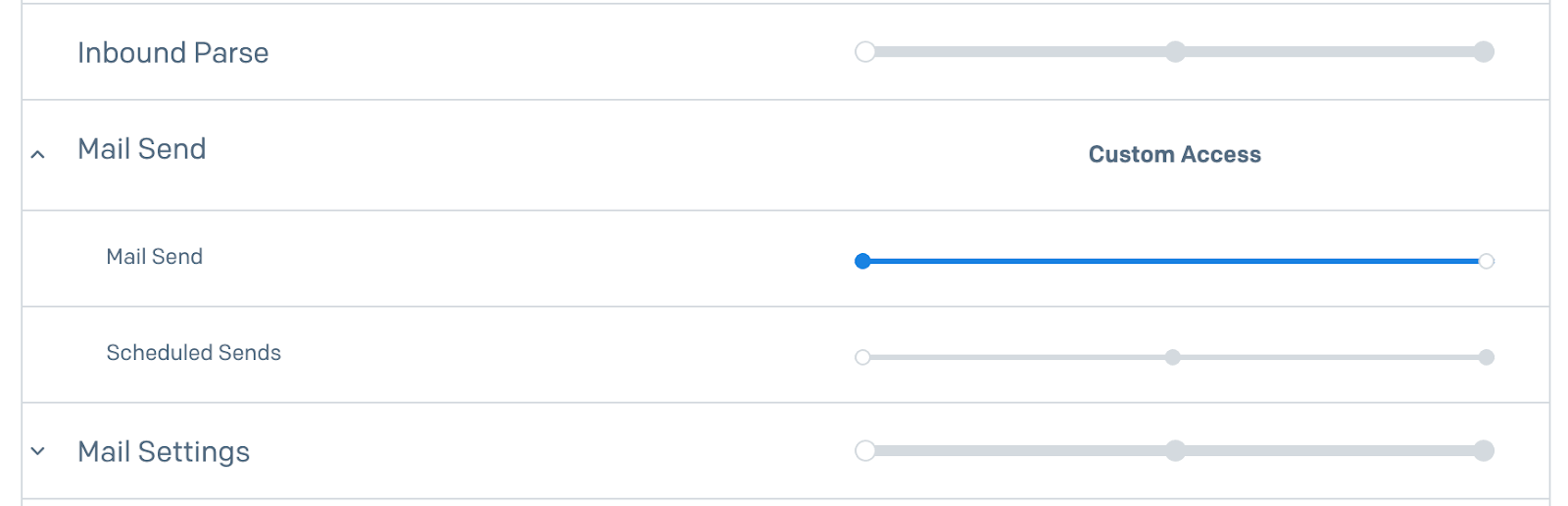
Scroll to the bottom of the form and click on Create & View. The API key will now be displayed on your screen. You will not be able to retrieve the API key again once you leave this screen.
Copy the secret somewhere safe.
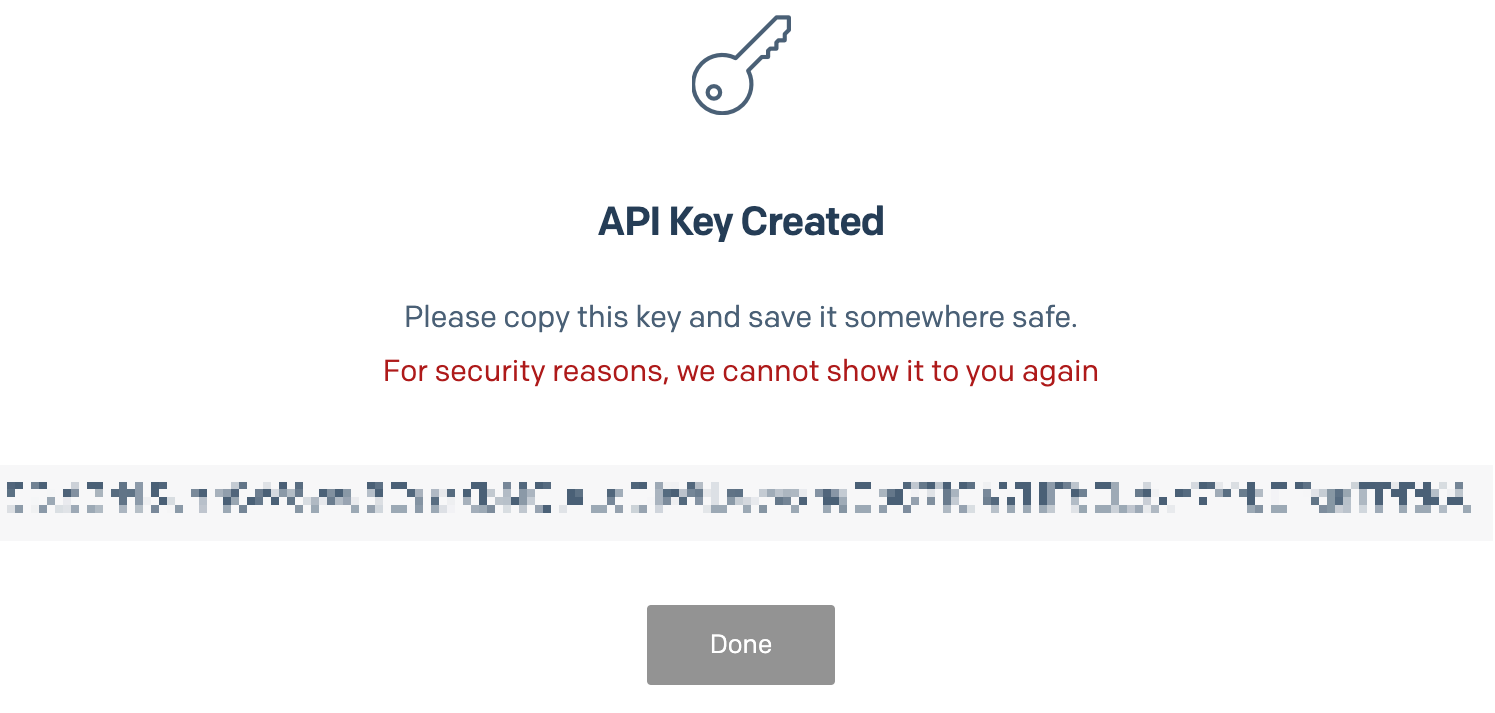
With the sender verified and the API Key created, you’re ready to write some code!
Add Twilio SendGrid to your Quartz.NET job
Add the following two SendGrid NuGet packages to your project using the .NET CLI:
The SendGrid NuGet package will add the core email capabilities. The SendGrid.Extensions.DependencyInjection NuGet package adds extensions to add the ISendGridClient
to the dependency injection container.
Update the Program.cs file with the highlighted lines:
The lambda passed into the ConfigureServices
method, now receives two parameters: context of type HostBuilderContext
and services of type IServiceCollection
. The context parameter has been added because it holds a reference to the configuration that will provide the API key for SendGrid.
services.AddSendGrid
will add the SendGrid client to the dependency injection container. A lambda is passed into the AddSendGrid
method to configure the ApiKey
option. The SendGridApiKey
is pulled from the configuration and stored into options.ApiKey
.
Now that SendGrid has been added to the dependency injection container, ISendGridClient
will be injected into the constructor of SendMailJob
and stored in a field.
The Execute
method uses the SendGrid APIs to construct an email and submit it to the SendGrid service.
Update the placeholder strings:
- replace
[REPLACE WITH YOUR EMAIL]
with the email address you verified with your SendGrid account. - replace
[REPLACE WITH YOUR NAME]
with your name. - replace
[REPLACE WITH DESIRED TO EMAIL]
with the email address you want to send an email towards. - replace
[REPLACE WITH DESIRED TO NAME]
with the recipient's name.
Save the Program.cs file.
The project now depends on the SendGridApiKey
configuration element, but it hasn't been configured anywhere. You can use .NET user secrets to configure sensitive configuration such as the API key.
Add the user secrets NuGet packages using the .NET CLI:
Initialize user secrets for your project using the .NET CLI:
Run the following command to set the API key as a user secret:
The user secrets configuration will override other configuration sources such as the appsettings.json file, but the user secrets will only be loaded when the environment is set as "Development". To do this, you can set an environment variable "DOTNET_ENVIRONMENT" to "Development". You can also pass it in as a command-line argument like this:
Run the project using the command above. The recurring output should look like this:
The program should now queue an email every 15 seconds and log "Email queued successfully!". Verify that you are receiving the email by opening your mailbox and look for the email with subject "Sending with Twilio SendGrid is Fun".
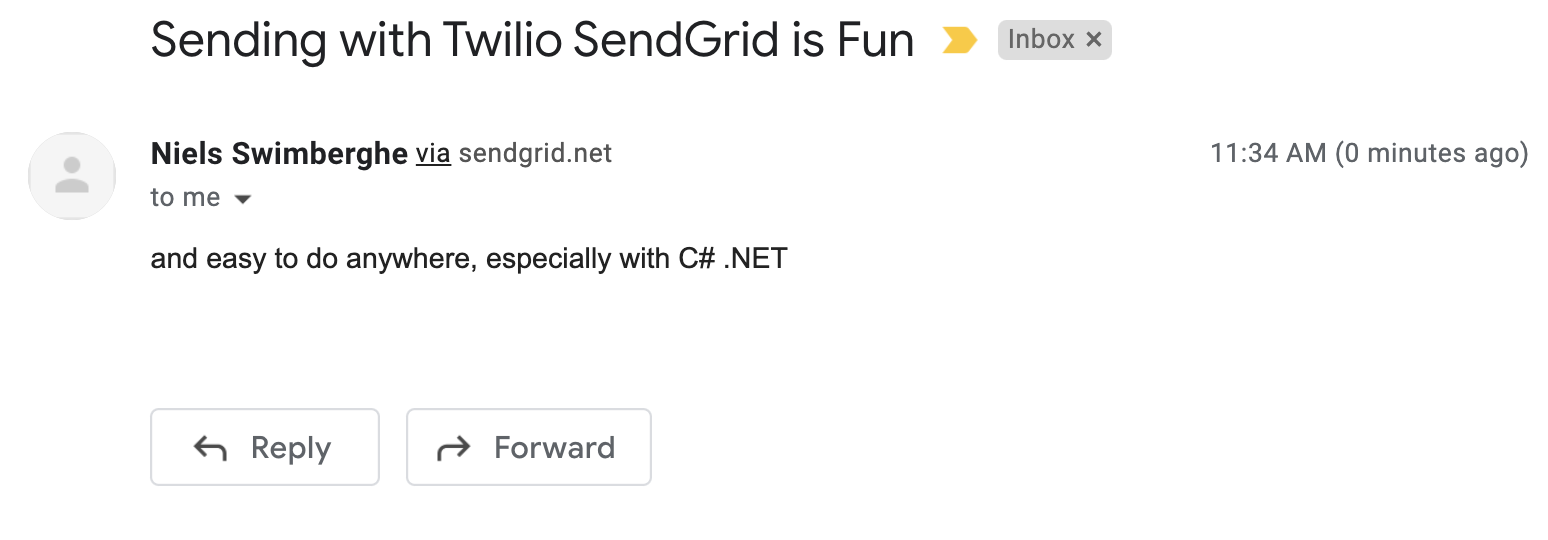
Conclusion
Quartz.NET is an open-source job scheduler for .NET. You built a .NET application that sends emails on a recurring schedule by integrating Quartz.NET and the SendGrid API.
Using what you learned here, you can also start integrating SendGrid into your ASP.NET Core applications. Twilio has a lot of other products you can integrate. Check out this tutorial on how to receive text messages using Twilio Programmable SMS and forward them using SendGrid emails with C# and ASP.NET Core.
Additional resources
Check out the following resources for more information on the topics and tools presented in this tutorial:
Quartz.NET website and documentation – You used the minimum amount of code to send emails on a recurring basis using Quartz, but there is a lot more to configure and learn at Quartz' website to get the most out of it.
SendGrid .NET SDK source code on GitHub – The SendGrid .NET SDK you used in this tutorial is open source! This GitHub repository contains helpful documentation on how to integrate with SendGrid using .NET. You can read the source code to better understand what's happening or submit issues if something isn't working as expected.
Worker Services in .NET on Microsoft Docs – Learn more about worker services and how to use it at Microsoft's documentation.
Source Code to this tutorial on GitHub - Use this source code if you run into any issues, or submit an issue on this GitHub repo if you run into problems.
Niels Swimberghe is a Belgian software engineer and technical content creator at Twilio, working from the United States. Get in touch with Niels on Twitter @RealSwimburger and follow Niels’ personal blog on .NET, Azure, and web development at swimburger.net.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.