How to Initiate a Voice Call from Laravel PHP with Twilio Programmable Voice
Time to read:
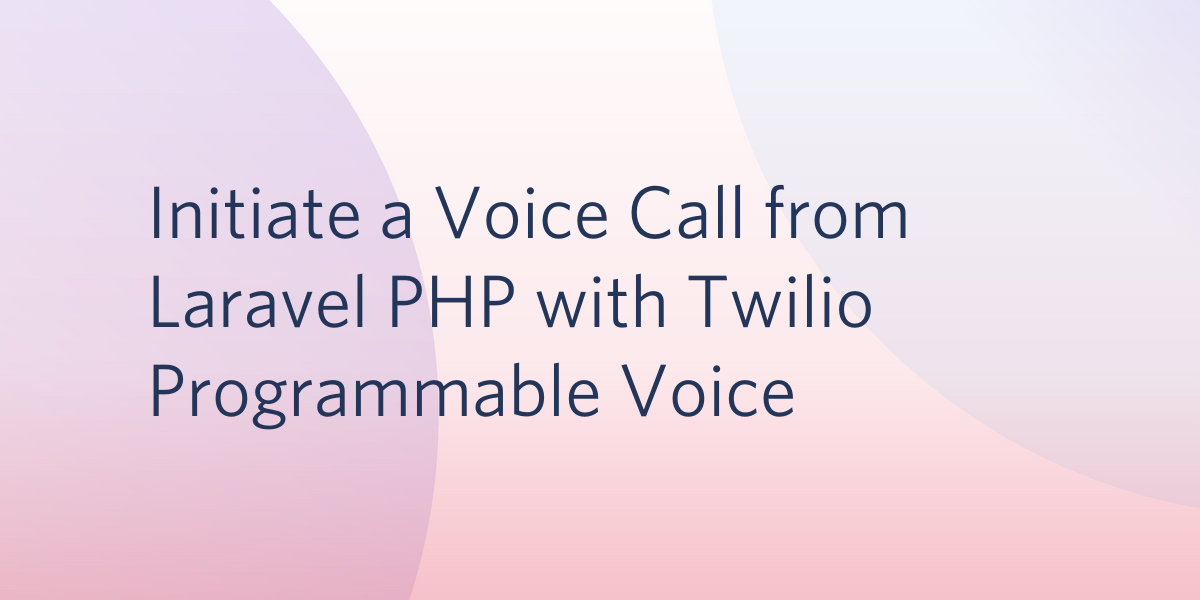
In this tutorial, we will be creating a Laravel application that will be used to make outbound voice calls. We will be making use of Twilio's Programmable Voice feature to learn how to initiate voice calls.
Technical Requirements
Completing this tutorial will require the following dependencies and a basic understanding of them.
- PHP (Version 7.1 or higher).
- Composer.
- Laravel.
- A Twilio Account.
Before we proceed with this tutorial, it is expected that you have a voice-enabled Twilio number. In case you do not have one, please proceed to purchase one here. This number will be used for the "from" number later in this tutorial.
Setting Up Your Development Environment
Let's install a new Laravel project via the Composer create-project
command. From your terminal, run the following command:
This will create a Laravel project for us in the twilio-voice
directory.
Our project will require the Twilio PHP Helper Library in order to connect to Twilio's Voice API. Using Composer, use the command below to install the SDK:
NOTE: Ensure that you are in the working directory of the Laravel application you created.
After installing the SDK, a connection between the client application and Twilio will need to be enabled. Edit your .env
file to update the parameters needed to create this connection. They can be retreived from your Twilio Dashboard.
The dotenv file allows us to securely use these credentials without exposing them to the public. To understand this better, please check out How to store your credentials securely.
Let's create a Controller that will house most of the logic for our application. Run the following command in your terminal:
This command has created a controller VoiceController.php
in the app\Http\Controllers
directory of your Laravel application.
Open the VoiceController.php file and import the Twilio Rest Client class.
Using the DRY principle as a guide, we will initialize a constructor to make the variables we need accessible by the controller, and avoid redefining them when we do need them. Add the following code to achieve that:
Creating a View
Let's proceed to create a view for the purpose of making a call. This view will allow us to create a form that captures the user's phone number. Upon submit, a call will be placed to the provided number.
In the resources/views
directory, create a file call.blade.php
. This is where our frontend design will be housed. Below is the code for this interface.
Looking Up the Phone Number
It would be really nice if we at least check to make sure that the target phone number exists or is valid before initiating the call. This line of code below performs that function.
To learn how to achieve more with this functionality, you can check out the Lookup Quickstart;
Initiating a Call
Making a call is as simple as a few lines of code with the Twilio SDK. After verifying the phone number, we can proceed to initiate the call from the client
object as defined in the constructor. The Programmable Voice API accepts the target phone number as the first parameter.
As an added benefit, calls can also be recorded and listened to on your Dashboard. If desired, you need to enable recording by setting record
to True
in your array below.
Our controller will have to be connected to a route in order to make it accessible via HTTP. In the routes/web.php
file, update the code as follows:
The completed VoiceController.php
code is below.
Testing
At this point you're probably ready to see the application in action! All you need to do is start up your development using the command below in your terminal.
Finally, using a web browser of your choice, visit http://localhost:8000. Fill in the form and submit.
Conclusion
After completing this tutorial, you should be able to see that using the Twilio Programmable Voice to make outbound calls is pretty easy. Feel free to explore other uses to build upon what we've learned today!
Additional Resources
The DRY principle and why you should use it - A top-level overview of DRY principle with PHP examples by Pety Ialimijoro Rakotoniaina
How to Lookup a phone number with the Twilio CLI - Twilio Lookup explanation and example with the new Twilio CLI by Kelly Robinson
I'd love to see what you build. Feel free to reach out to me through the following channels.
Ugendu Martins Ositadinma
Email: ugendu04@gmail.com
Twitter: https://twitter.com/ohssie_
Github: https://github.com/ohssie
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.