Start a Ghost Writing Career for Halloween with OpenAI's GPT-3 Engine and Java
Time to read: 5 minutes
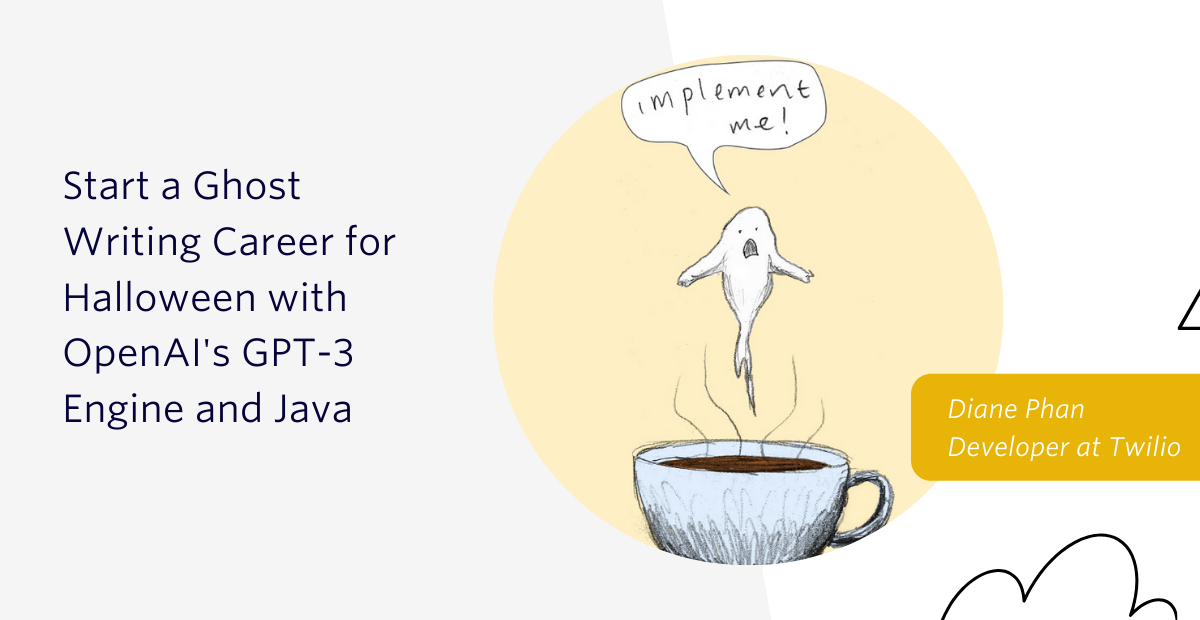
It can be tempting to stay inside with a cup of hot cocoa to read as the days get chillier. Why not challenge yourself by writing some code along with a new story this fall season?
In this article, you will learn how to navigate a Java IDE to build a ghost writing application in Java with the OpenAI GPT-3 engine.
Tutorial Requirements
- Java Development Kit (JDK) version 8 or newer.
- IntelliJ IDEA Community Edition for convenient and fast Java project development work. The community edition is sufficient for this tutorial.
- An OpenAI API key. Request beta access here.
Start a new Java project in IntelliJ IDEA
Open IntelliJ IDEA and click on Create New Project.
Choose Gradle on the left hand side, check Java in the box on the right hand side, and click Next.
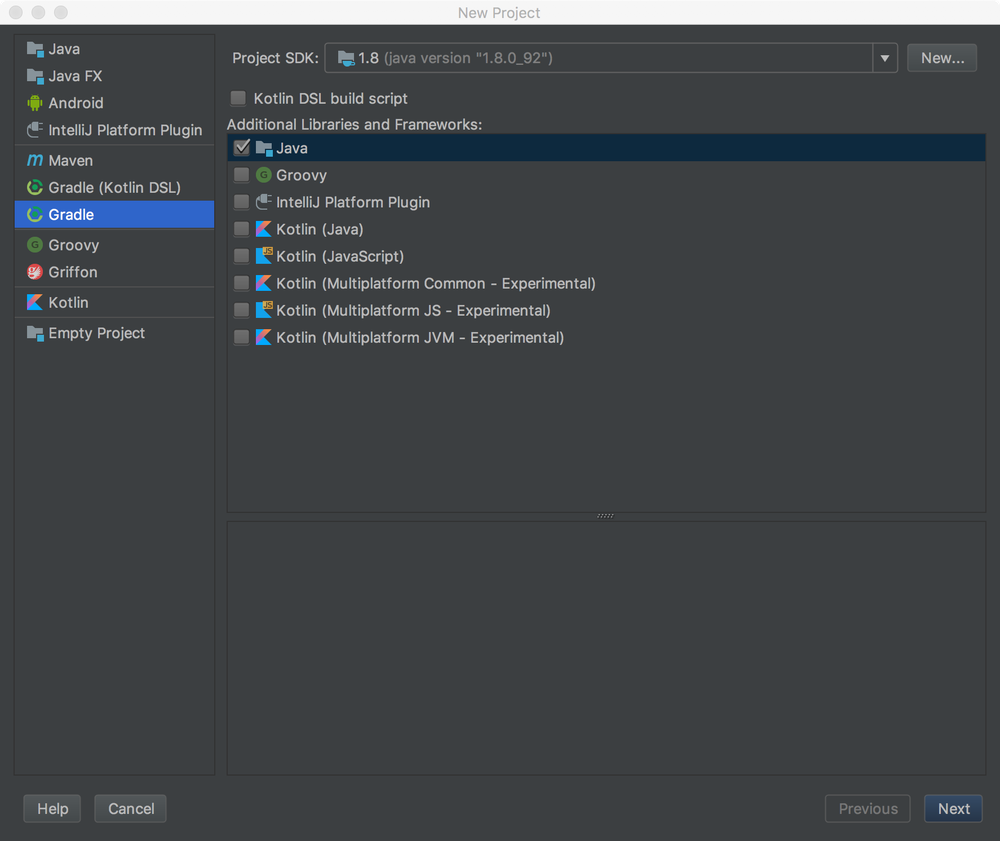
Give your project a name such as "GhostWriter" and click the Finish button.
After the project setup is complete, your project directory structure should look like the following image:
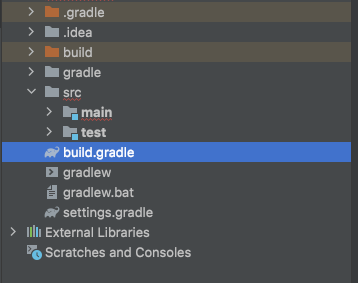
Open the build.gradle file in the IDE and add the following lines inside the dependencies
block:
Build your ghost writer story app
In the spirit of Halloween and ghosts, we'll have the prompts used in this article be related to a scary story.
The OpenAI playground allows users to explore GPT-3 (Generative Pre-trained Transformer 3), a highly advanced language model that is capable of generating written text that sounds like an actual human wrote it. This powerful model can also read a user's input and learn about the context of the prompt to determine how it should generate a response.
In this project, we will be feeding the GPT-3 engine with a sentence to create a complete scary story that will keep running on your WhatsApp device.
Create the Ghost Writer app's Java class
Expand the main subfolder under the src folder at the root of the project directory. Notice the empty java folder.
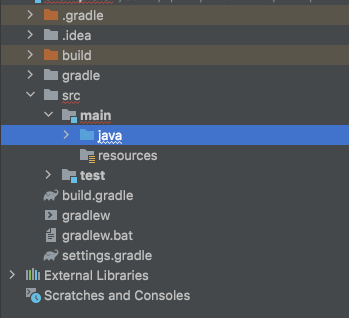
Right click on the Java folder and click on the New option, then select Java Class as seen in the image below. Clicking this option will prompt you to give the class a name. Go ahead and name it "GhostWriter".
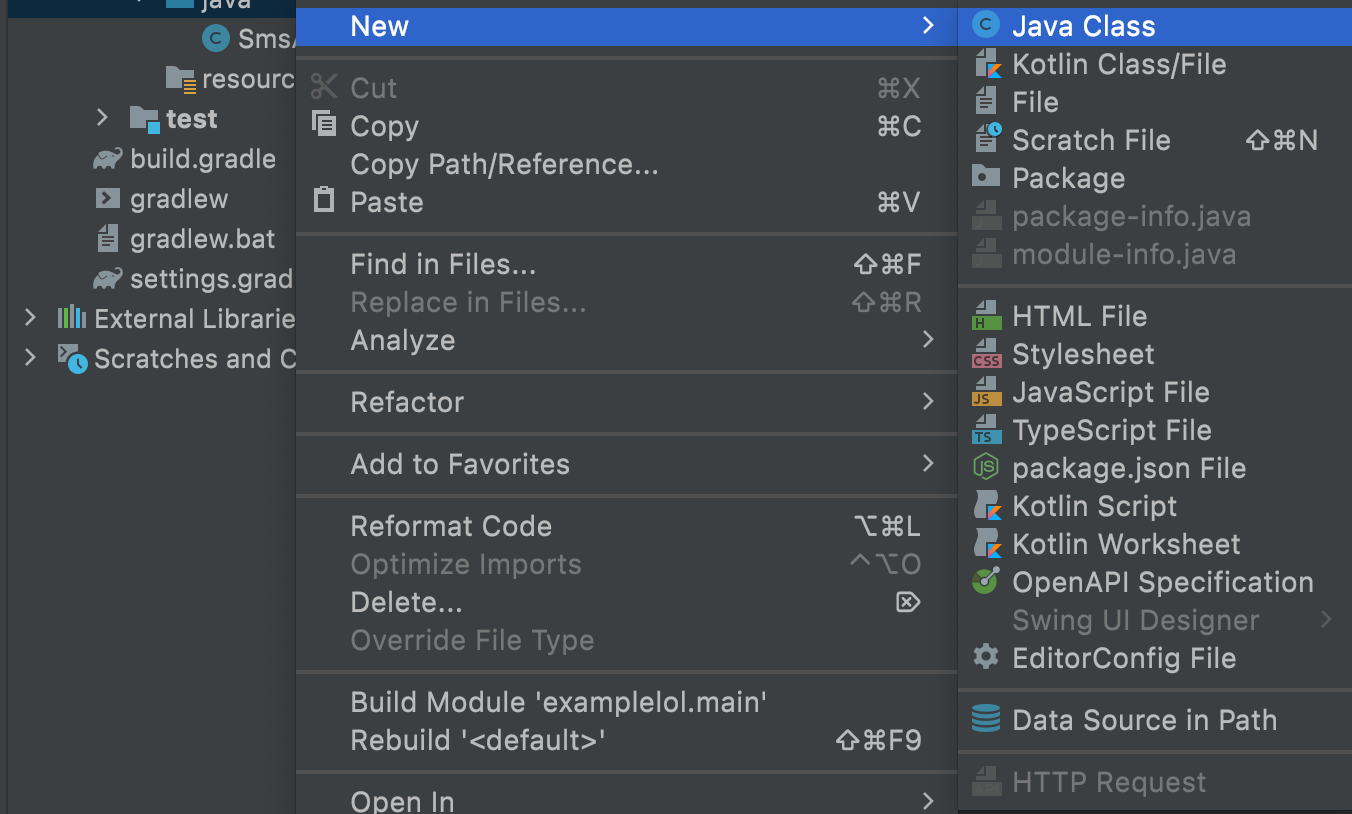
Delete the existing template code in the newly created file and then copy and paste the following code:
In order to use OpenAI in Java projects, developers must incorporate TheoKanning's openai-java community library. This library allows us to utilize the CompletionRequest
and CompletionChoice
classes to generate an appropriate response from the engine.
In the public GhostWriter
class, a main
method is invoked so that the code in the program starts once executed.
As seen above, a token
variable retrieves the OPENAI_TOKEN
that holds the API key configured in the project's environment variables. The token allows the Java project to create an OpenAiService
object where all the magic happens. This token will be set in the upcoming section.
Set the OpenAI API Key in IntelliJ IDEA
As mentioned above, this project requires an API token from OpenAI. During the time of this article, the only way to obtain the API key is by being accepted into their private beta program.
If you have access to the Beta page, the API key can be found in the Authentication tab in the Documentation.
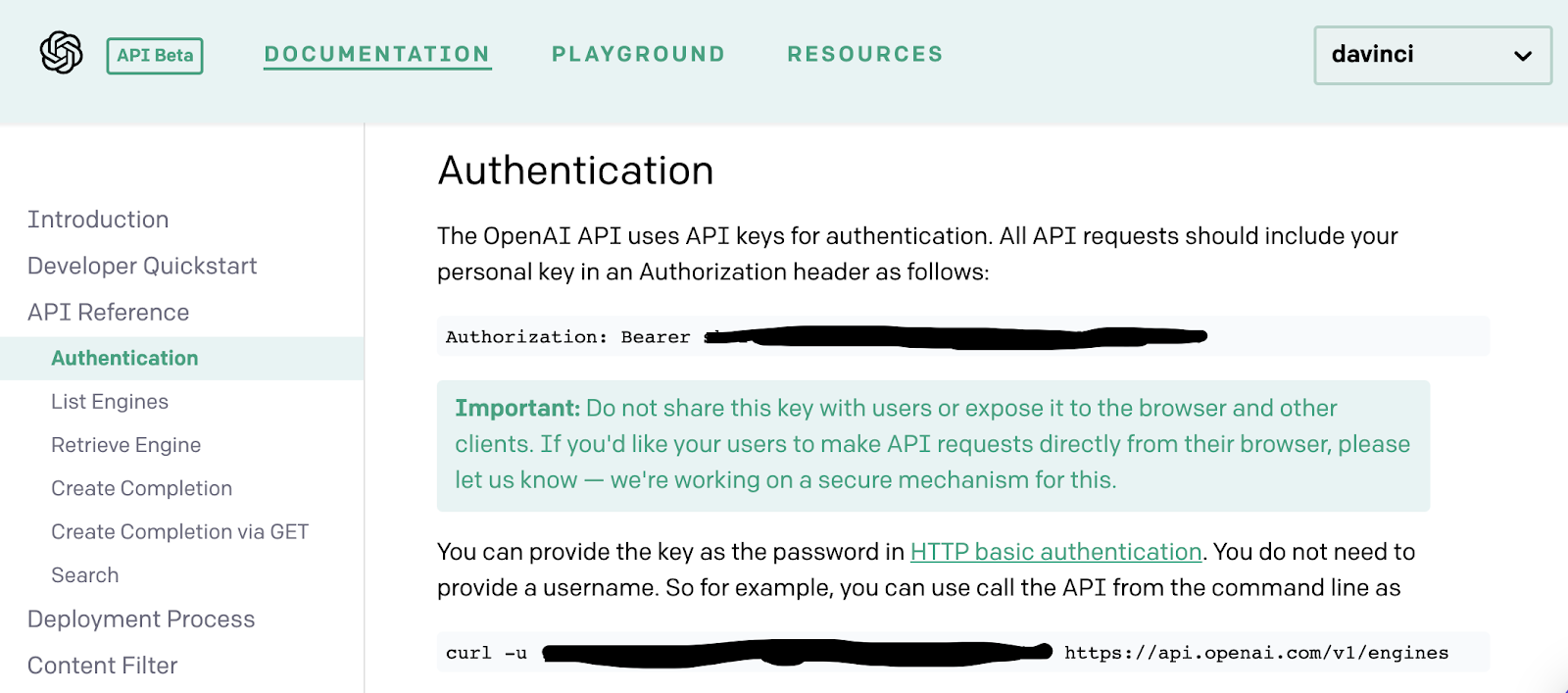
The Java application will need to have access to this key, so environment variables need to be set to safely make a connection to the OpenAI GPT-3 engine.
Locate the Run tab at the top of the IntelliJ IDEA console and select Edit Configurations… in the dropdown as seen below:
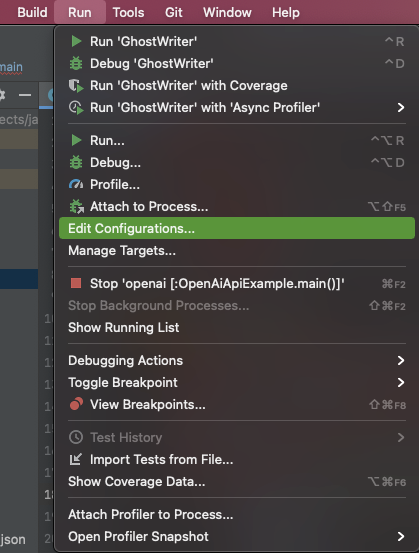
Another window displaying the "Run/Debug Configurations" will pop up with the details regarding the project.
In the Environment variables section, follow the sample format to set the relevant variables. In this example, the API key is named OPENAI_TOKEN
.
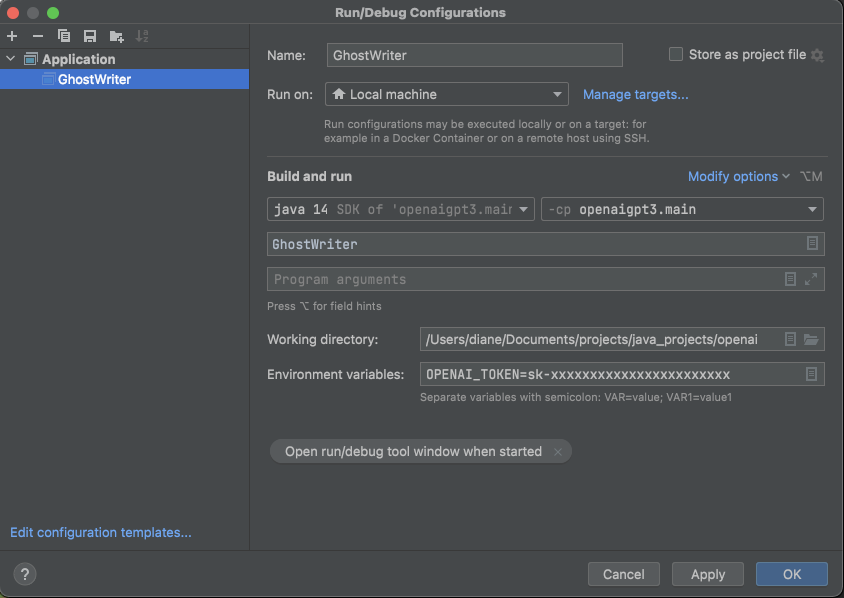
Click on the Apply button, and then OK once finished.
Establish a connection to the GPT-3 engine
There are different engines to choose from when working with GPT-3. In this article, the davinci
model is used because it is the most capable model, but feel free to explore other GPT-3 models for your project.
After creating the OpenAiService
object, copy and paste the highlighted code to the GhostWriter
class:
The generated text results are returned in an ArrayList
format consisting of values from TheoKanning's CompletionChoice class. Storing results in this ArrayList
object is encouraged so that you have the option to expand on the story later on if desired.
Teach the ghost writer app how to write
In order to make a request to the OpenAI GPT-3 engine that is relevant to our interests, a prompt and various arguments must be supplied to teach the engine, or ghost writer, how to return the desired results.
Copy and paste the following code right beneath the ArrayList
definition to create an instance of TheoKanning's CompletionRequest class:
If you played around with the OpenAI playground introduced earlier in the article, you may notice that some of the arguments here look familiar.
After setting the value for prompt
, it is recommended for the builder
function to specify values, such as the temperature
, to lessen the randomness in results. Another value that should be specified is maxTokens
, which stands for either a word or punctuation mark to be generated. This was set to 96
so that the length of the caption will be appropriate - not too long and not too short to provide a more cohesive idea on what you can start writing about.
You can read more about the GPT-3 customization options in the Ultimate Guide to OpenAI-GPT3 Language Model or explore the OpenAI Playground for yourself.
Before the build
method is executed, the echo
function is added to echo back the prompt in addition to the newly created tokens.
Each line generated by the OpenAI service
object is appended to the ArrayList
defined earlier and printed onto the console.
Run the ghost writer Java app
We've nearly reached the end of the tutorial. If you need to check your code, here's my GitHub repo.
Right click on the GhostWriter file in the project directory and find the option to Run 'GhostWriter.main()'. Wait a few seconds for the project to build and compile. When running, the Run window in the IntelliJ IDEA console should look something like this:
And just like that, your little ghost writer is ready to help you start some new spooky stories to fill the cold nights ahead! Go ahead and stop the app, then rerun it and see what other results you get.
Keep in mind that you can't control what your ghost writer decides to write so it's up to you whether you want to read what they have to say.
What’s next for OpenAI GPT-3 projects?
If you're eager to build more, try expanding on the project by feeding the generated results to the Ghost writer app so that it keeps adding onto the story for you. Otherwise, check out these ideas and incorporate Twilio:
- Convert this Ghost Writing App to Twilio SMS text
- Generate Dragon Ball Fan Fiction with OpenAI's GPT-3 and Twilio SMS
- Build a Michelin Star Chef bot Using OpenAI's GPT-3 and Twilio WhatsApp
Let me know how you are using code to be creative this holiday season by reaching out to me over email!
Diane Phan is a software developer on the Developer Voices team. She loves to help programmers tackle difficult challenges that might prevent them from bringing their projects to life. She can be reached at dphan [at] twilio.com or LinkedIn.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.