How To Send Multiple SMS Using Spring Boot and Kotlin
Time to read: 7 minutes
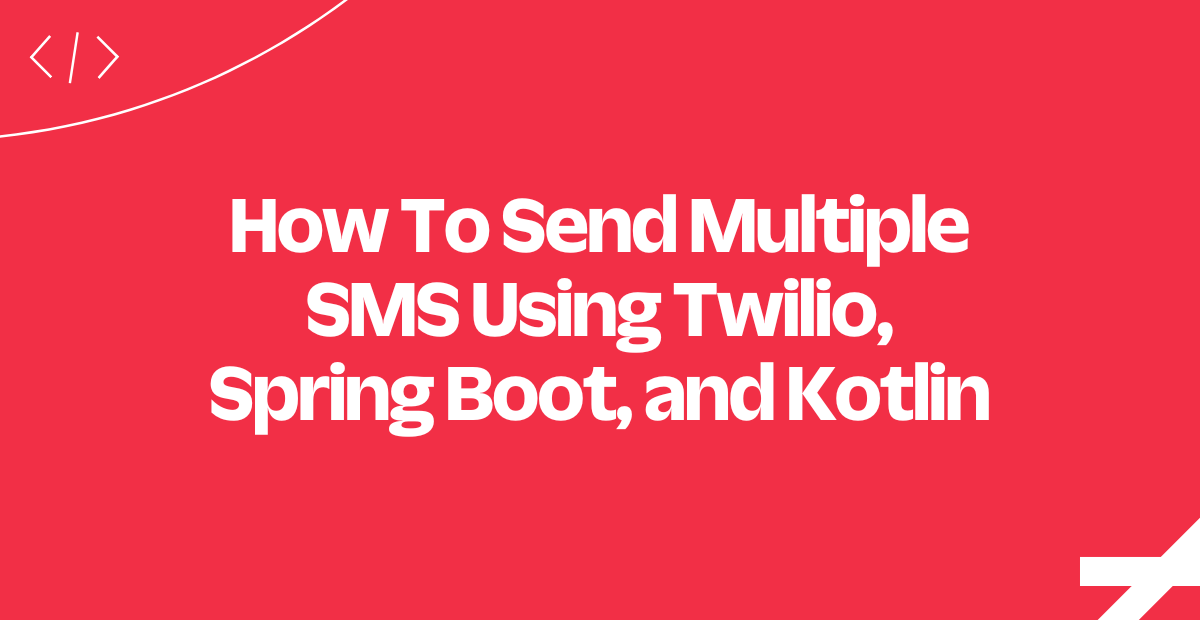
Finding a channel to communicate with your users is critical. Chances are that many of them spend a lot of time with their phones in their hands. So, notifying your users with a personalized SMS is one of the most effective techniques to get in touch with them.
In this tutorial, you will learn how to build an API in Spring Boot and Kotlin that uses Twilio’s Programmable SMS to programmatically notify multiple contacts via SMS.
Prerequisites
This is the list of prerequisites you need to meet to follow this tutorial:
- Java Development Kit (JDK) ≥ 11: any LTS version of Java greater than or equal to 11 will do. This article is based on Java 17, which is the latest LTS version of Java at the time of writing.
- Gradle ≥ 7 or Maven ≥ 3: choose whichever Java building automation tool you prefer. This guide shows the use of Gradle with Kotlin. Note that Gradle is very sensitive to Java versions, so you need to install the right version according to your Java version.
- IntelliJ IDEA: the Community Edition version of the IDE is enough to follow this tutorial.
- A Twilio account.
- A Kotlin-based Spring Boot Web 3+ project.
Click the links above and follow the official installation guides to download and install everything you need.
Now, let's set up a Twilio account and Spring Boot project with Kotlin. If you already meet these two prerequisites, you can skip to the next section.
Create and set up a Twilio account with a phone number
Follow the link to sign up for a free Twilio trial. You will be asked to fill out the form below:
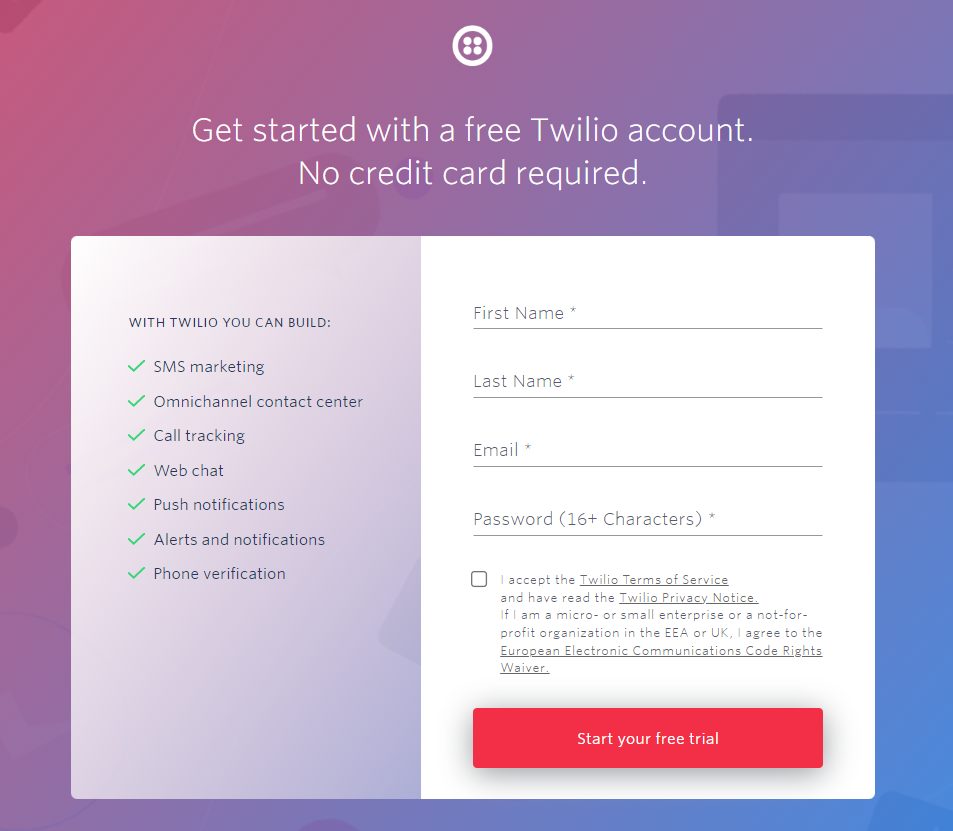
Insert your info in the form and click on “Start your free trial.” This will initialize the procedure to get your new Twilio account and start a free trial.
Within a few minutes, you will receive an email to the email address you specified in the sign-up form. Open the email from Twilio and click on the link you received in your inbox to verify your email address:
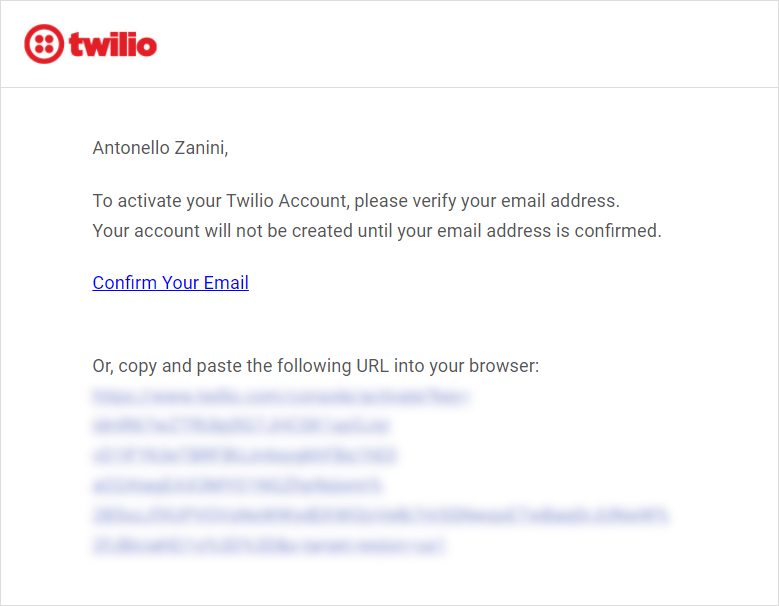
Clicking on that link will take you to the following Twilio page:
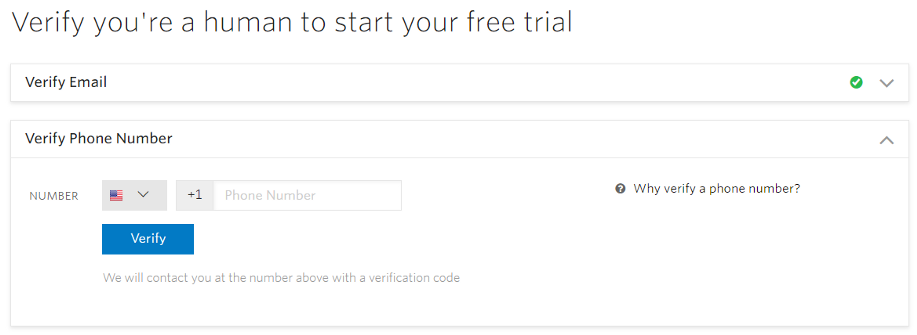
As you can see, the green checkmark indicates that you successfully verified your email. It is time to verify the phone number. Fill out the form with your phone number. Click "Verify," and wait until you receive a text message from Twilio. Enter the verification code you received via SMS to verify your phone number.
You will be redirected to the following page. Fill out the last form as shown below:
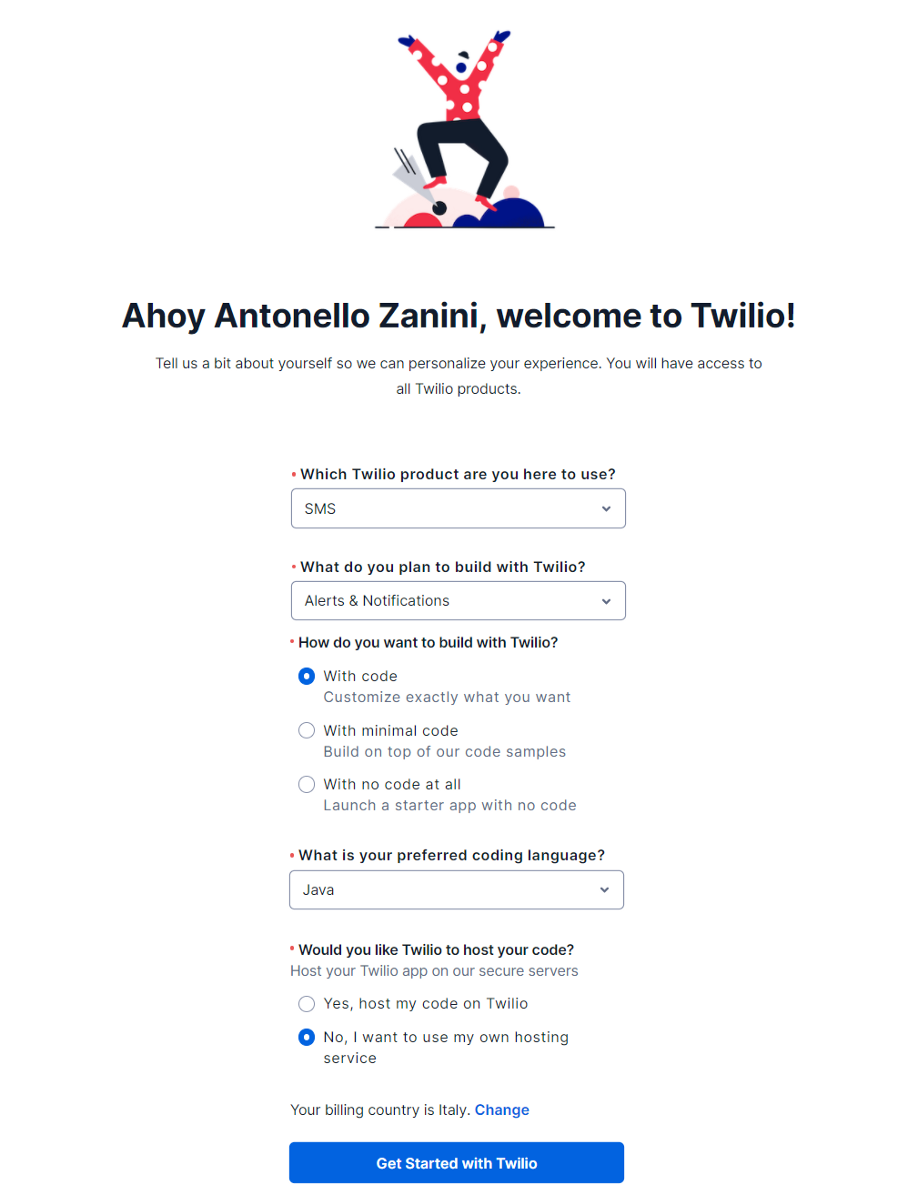
Note that Kotlin is fully interoperable with Java. So, selecting Java as your preferred programming language will be sufficient.
Click Get Started with Twilio to get access to the Twilio Console page:
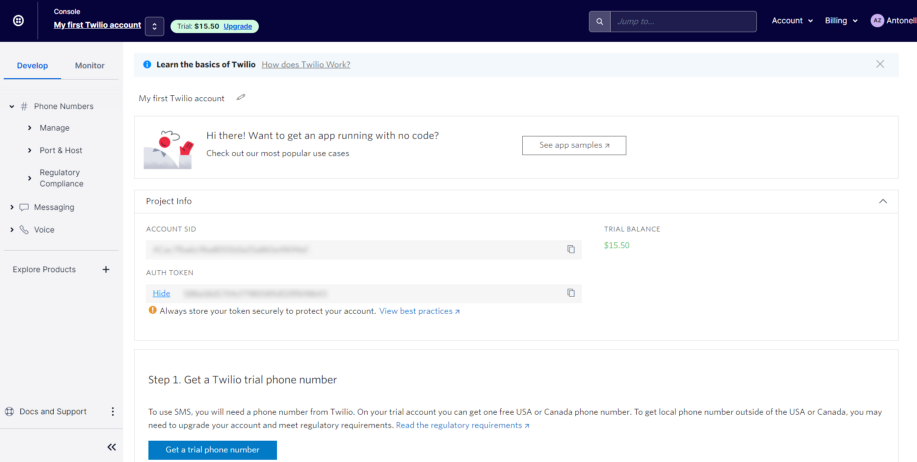
Now, you need to set up a Twilio phone number. Click “Get a trial phone number” in the “Step 1.” section. Then, select Choose this Number.
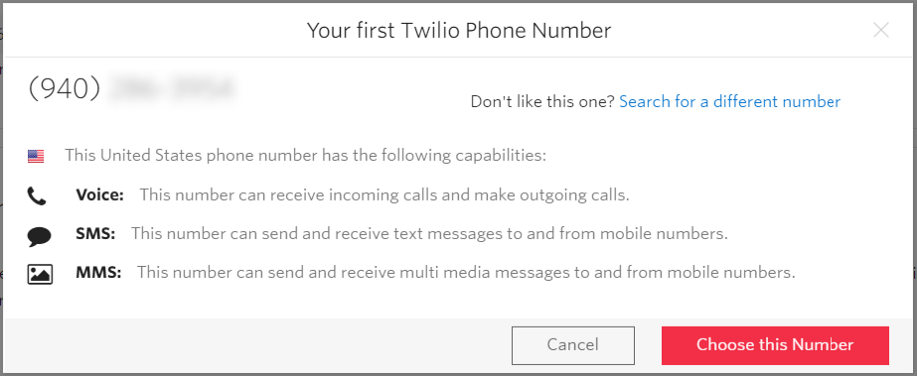
Follow the procedure, and you should now have access to a valid Twilio phone number. This is the phone number Twilio will use to send the multiple SMS. Keep in mind that during your trial, you can only set up a free Canada or USA phone number.
Now, your Twilio Console should look as follows:
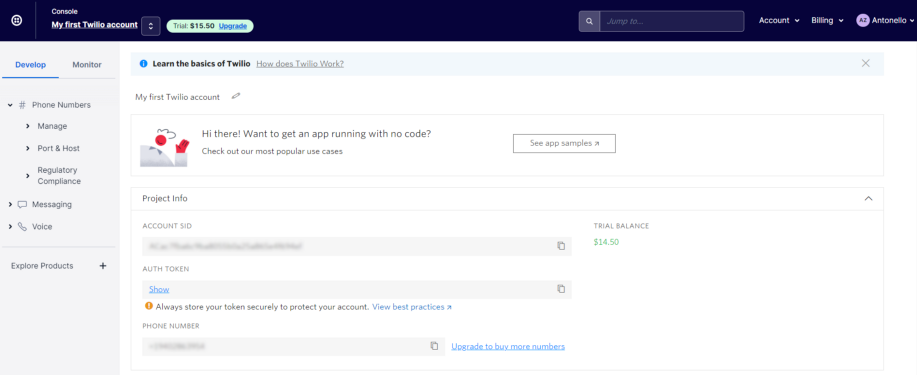
In the “Project Info” card, you can find your:
- ACCOUNT SID
- AUTH TOKEN
- PHONE NUMBER
These three values represent everything required to connect your project to your Twilio account. Copy these values and store them in a safe place. You will need them later.
Initialize a Kotlin-based Spring Boot Web project
If you do not have a Spring Boot project, you can initialize it in Spring Initializr as follows:
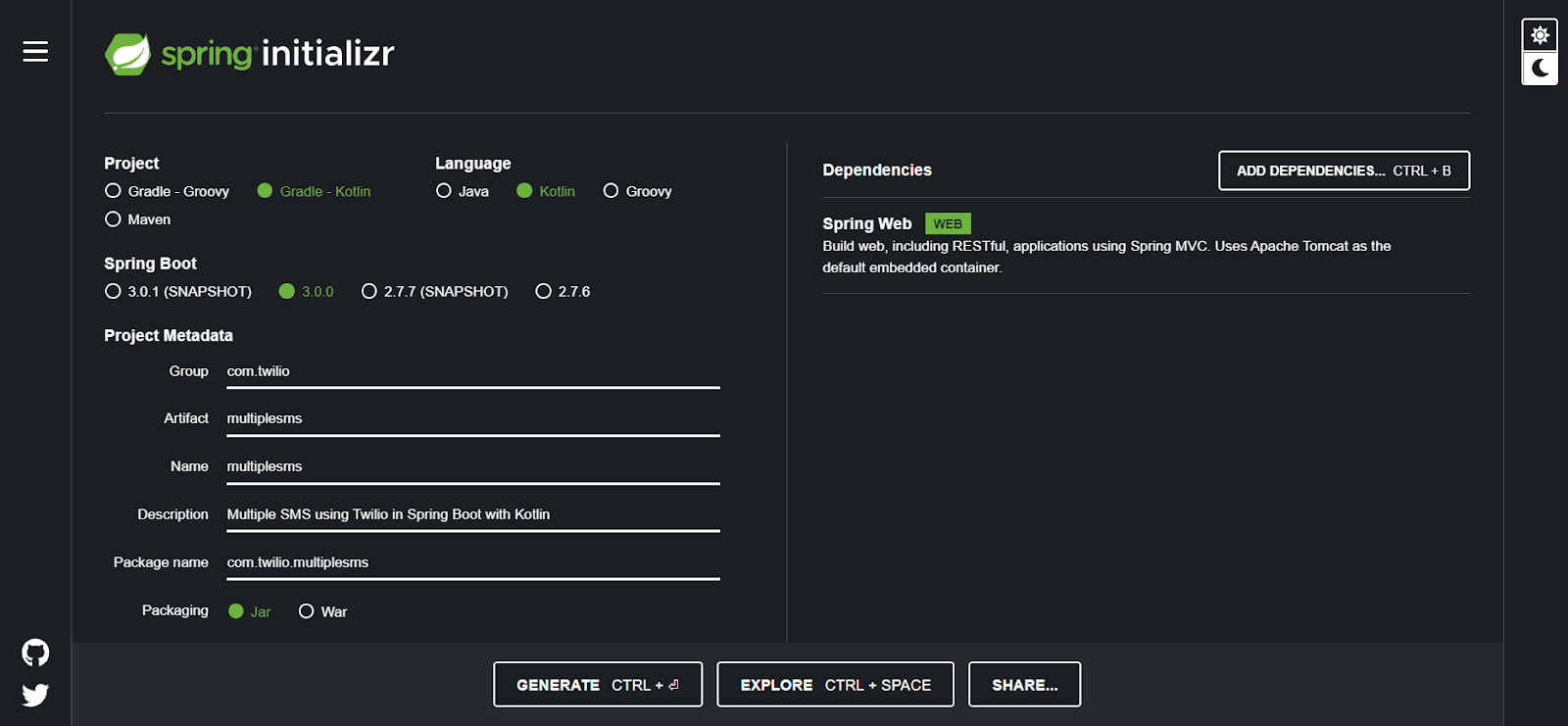
Click on ADD DEPENDENCIES… and select “Spring Web” to initialize a Spring Boot Web project. Click GENERATE to download the .zip archive containing your blank Kotlin Spring Boot project. Extract it and open the project in your IntelliJ IDE to get started.
Build an API to Send Multiple SMS in Spring Boot
Follow all the steps below to learn how to build an API in Spring Boot with Kotlin to notify several contacts via SMS programmatically.
If you want to take a look at the full codebase rather than build the application as you read, then you can clone from clone the GitHub repository that supports the article with the command below:
Now, let’s learn how to achieve the same result in a step-by-step tutorial.
Add the Twilio Java Helper Library to the project dependencies
If you are a Gradle user, open the build.gradle.kts file you can find in the root folder of your project. Add the following line in the dependencies
object:
Otherwise, if you are a Maven user, navigate to the pom.xml file in the root folder of your project. Open it and add the following lines to your dependencies
section:
These lines add the Twilio Java Helper Library to your project’s dependencies. This library is essential for the project because it allows you to use the Twilio SMS API. In detail, this Twilio feature provides you with everything you need to send multiple SMS in Spring Boot.
When you save the dependency file, IntelliJ IDEA will show the Gradle/Maven reload button on the top right corner:
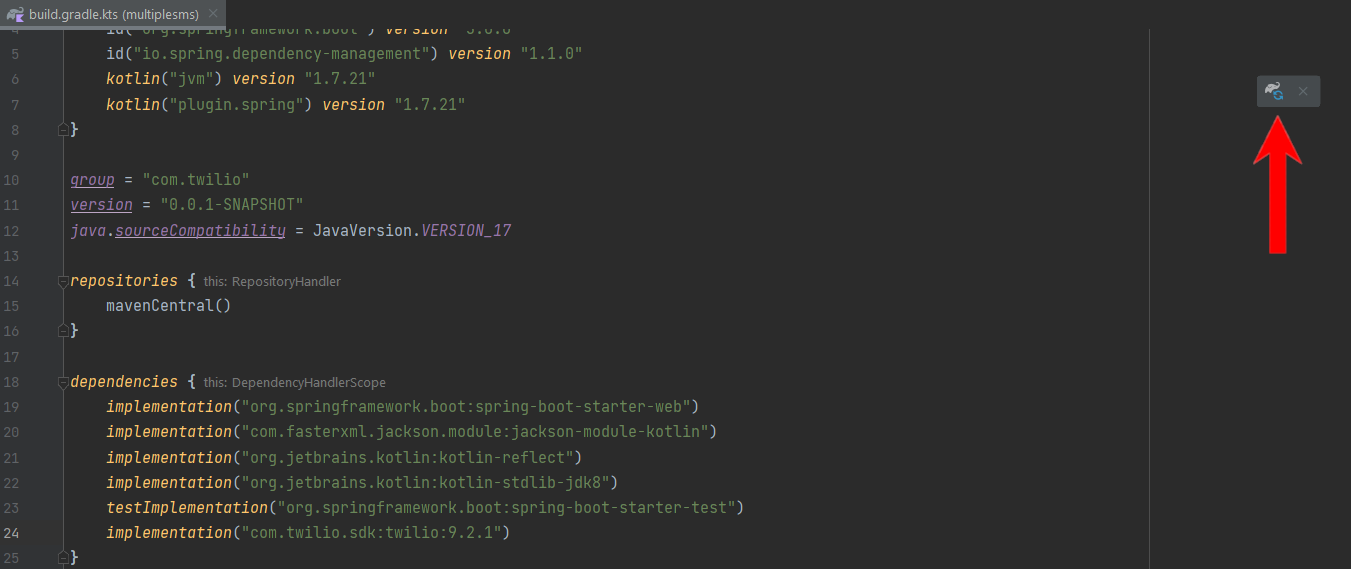
Click the button to install the Twilio Java Helper Library. Wait for the installation process to end, and you can now take advantage of the Twilio SMS API in your Kotlin Spring Boot application.
Initialize Twilio
As explained in the Twilio official documentation, you need to initialize Twilio through the Twilio.init() function. Let’s learn how to achieve this in Spring Boot.
First, paste your Twilio ACCOUNT SID, AUTH TOKEN, and PHONE NUMBER in the application.properties file as below:
This is a special Spring Boot file that contains your project configs. Note that you can configure these two fields to read the value from the environment variables.
Then, create a config package. In IntelliJ IDEA, right-click the com.twilio.multiplesms package, select New > Package type "config" and press the Enter key. This package will contain all @Configuration files that Spring Boot automatically loads at initialization time.
In the config package, create a new Kotlin file named TwilioConfig.kt and initialize it as follows:
This @Configuration
class will read the twilio.account-sid
and twilio.auth-token
properties from application.properties through the @Value annotation. Then, Spring will call the twilioInit()
method to initialize Twilio.
Note that Spring Boot calls methods annotated with @PostConstruct only once, after the fields annotated with @Value
have already been initialized.
Define a service to Send SMS
It is now time to create a SMSService
class that implements the business logic required to send multiple SMS with Twilio.
To send multiple SMS, you will need a list of contacts. Let’s assume you store the following info about your contacts:
- full name
- phone number
You need to model this data structure in a Contact
class in Kotlin. Create a data package inside the com.twilio.multiplesms package, and initialize a Contact.kt data class file as below:
Now, right-click on the com.twilio.multiplesms package. Select New > Create Kotlin Class/File and create the new class service.SMSService.kt. This will create a SMSService.kt file under the service package.
This package will contain all your @Service Spring Boot files. If you are not familiar with this concept, a Spring Boot service should contain part of your business logic. Specifically, the service package represents your business logic layer.
Now, initialize an SMSService.kt file as follows:
The sendMultipleSMS()
method takes the string message and a list of contacts. Then, it uses the parallelStream() method to iterate over contactList
in parallel and sends an SMS containing message
to each contact. This is done with the Twilio Message class.
In detail, Message.creator()
requires the following three parameters:
- the recipient's phone number.
- the sender's phone number.
- the string message.
Then, when the create()
method is called, Twilio will send the SMS.
Create an API Endpoint
Now, let’s define an API endpoint that calls the sendMultipleSMS()
defined earlier and programmatically sends multiple SMS messages.
You can define API endpoints in Spring Boot in @RestController files. First, create a controller package. This package will contain all your Spring Boot controllers.
In the controller package, create an SMSController.kt file as below:
Here, you just defined a /api/v1/sms/sendMultipleSMS
POST API endpoint to send multiple SMS in Spring Boot. Specifically, the API uses the data contained in a MultipleSMSBody
instance received as a body parameter to call the sendMultipleSMS()
method from SMSService
.
Define a MultipleSMSBody
class in a MultipleSMSBody.kt file inside the data package as follows:
This data class maps the JSON body that you need to pass to the /api/v1/sms/sendMultipleSMS
endpoint. In particular, it accepts a list of contacts and a message. This is exactly what the SMSService
sendMultipleSMS()
method needs to send multiple SMS.
Test the SMS API
In IntelliJ IDEA, click the Run button to launch your Kotlin Spring Boot app:
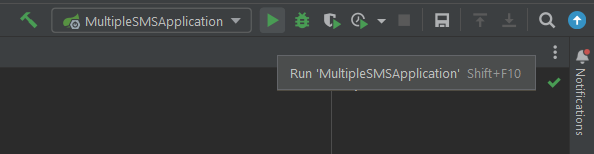
Wait for Spring Boot to start. Then, open your terminal.
If you are a Linux or macOS user, you can test the API with the following command:
Or if you are a Windows user, run the following command:
This command will reach the API made available by your Spring Boot. In detail, it will send an SMS message containing the “Welcome to my app!” text to the following numbers in the contacts
object:
- +1555000111
- +1555000222
- +1555000333
Please remember to change the phone numbers above to your own cellular device.
Congrats! You just learned how to implement an API to send multiple SMS messages in Spring Boot and Kotlin!
Conclusion
In this step-by-step tutorial, you saw how to use the Twilio Java Helper Library to build an API in Spring Boot and Kotlin to notify many contacts via SMS. In detail, this required only a handful of lines of code. This is thanks to the Twilio SMS API, which allows you to send multiple SMS messages with no effort.
All that remains is to make the system a more complex and robust system. For example, you could retrieve the contact list with a query from a database. Or, you could use the API offered by Twilio to support bulk SMS sending.
Antonello Zanini is a CEO, technical writer, and software engineer but prefers to call himself a Technology Bishop. Spreading knowledge through writing is his mission. He can be reached on LinkedIn or at antonello [at] writech.run.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.